java tutorial - How to implement Selection Sort Algorithm in java - java programming - learn java - java basics - java for beginners
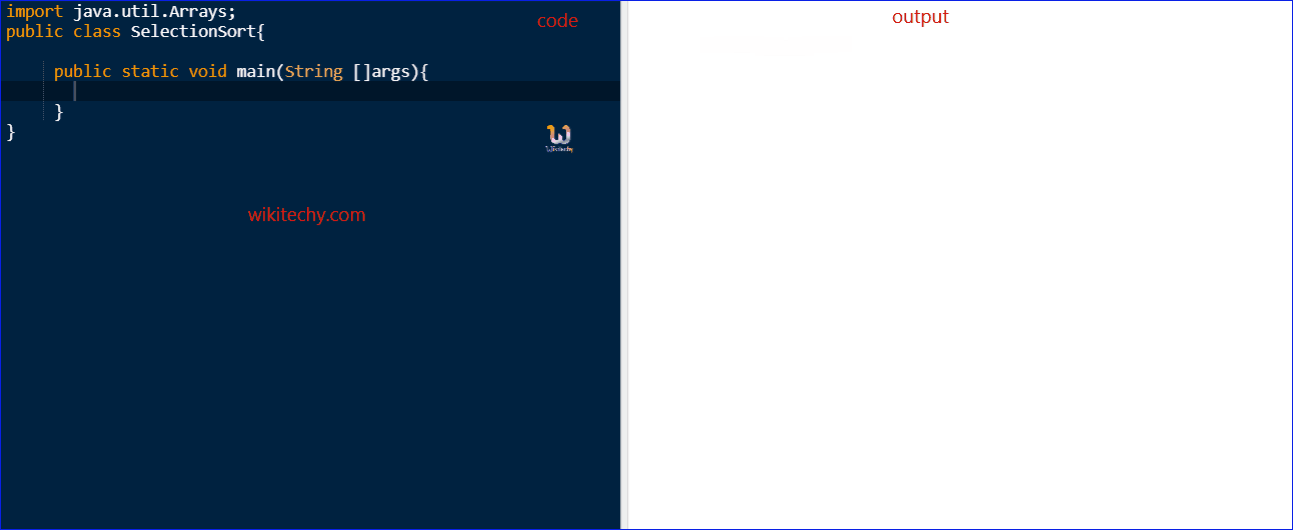
Learn Java - Java tutorial - selection sort in java - Java examples - Java programs
Selection Sort Algorithm
- The selection sort algorithm sorts an array by repetitively finding the lowest element from unsorted part and placing it at the beginning.
- In each iteration of selection sort, the lowest element from the unsorted subarray is chosen and moved to the sorted subarray.
- The good thing about selection sort is it never makes more than O(n) swaps and can be valuable when memory write is an expensive operation.
sample code:
import java.util.Arrays;
public class SelectionSort {
public static int[] doSelectionSort(int[] arr){
for (int i = 0; i < arr.length - 1; i++)
{
int index = i;
for (int j = i + 1; j < arr.length; j++)
if (arr[j] < arr[index])
index = j;
int smallestNumber = arr[index];
arr[index] = arr[i];
arr[i] = smallestNumber;
}
return arr;
}
public static void main(String a[]){
int[] list = {4,3,2,5,9,6,3,21,42,4,3,6};
System.out.println("Before Selection sort\n");
System.out.println(Arrays.toString(list));
list = doSelectionSort(list);
System.out.println("\nAfter Selection sort");
System.out.println(Arrays.toString(list));
}
}
Output:
Before Selection sort
[4, 3, 2, 5, 9, 6, 3, 21, 42, 4, 3, 6]
After Selection sort
[2, 3, 3, 3, 4, 4, 5, 6, 6, 9, 21, 42]