java tutorial - Java Collections class - java programming - learn java - java basics - java for beginners
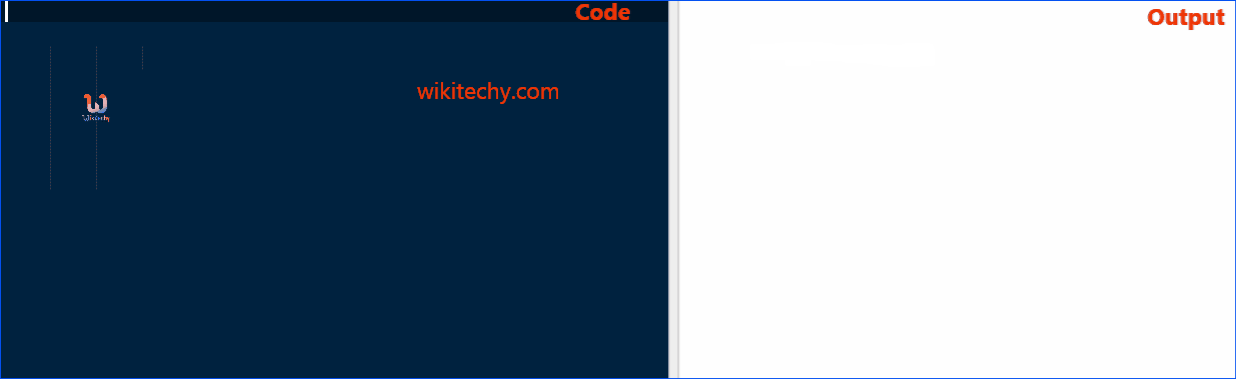
Learn Java - Java tutorial - Java collections class - Java examples - Java programs
Java collection class is used exclusively with static methods that operate on or return collections. It inherits Object class.
The important points about Java Collections class are
- Java Collection class supports the polymorphic algorithms that operate on collections.
- Java Collection class throws a NullPointerException if the collections or class objects provided to them are null.
Collections class declaration
Let's see the declaration for Java.util.Collections class.
public class Collections extends Object
click below button to copy the code. By - java tutorial - team
Methods of Java Collections class
Method | Description |
---|---|
static <T> boolean addAll(Collection<? super T> c, T... elements) | It is used to add all of the specified elements to the specified collection. |
static <T> Queue<T> asLifoQueue(Deque<T> deque) | It is used to return a view of a Deque as a Last-In-First-Out (LIFO) Queue. |
static <T> int binarySearch(List<? extends T> list, T key, Comparator<? super T< c) | It is used to search the specified list for the specified object using the binary search algorithm. |
static <E> List<E> checkedList(List<E> list, Class<E> type) | It is used to return a dynamically typesafe view of the specified list. |
static <E> Set<E> checkedSet(Set<E> s, Class<E> type) | It is used to return a dynamically typesafe view of the specified set. |
static <E> SortedSet<E>checkedSortedSet(SortedSet<E> s, Class<E> type) | It is used to return a dynamically typesafe view of the specified sorted set |
static void reverse(List<?> list) | It is used to reverse the order of the elements in the specified list. |
static <T> T max(Collection<? extends T> coll, Comparator<? super T> comp) | It is used to return the maximum element of the given collection, according to the order induced by the specified comparator. |
static <T extends Object & Comparable<? super T>>T min(Collection<? extends T> coll) | It is used to return the minimum element of the given collection, according to the natural ordering of its elements. |
static |
It is used to replace all occurrences of one specified value in a list with another. |
Java Collections Example
import java.util.*;
public class CollectionsExample {
public static void main(String a[]){
List<String> list = new ArrayList<String>();
list.add("C");
list.add("Core Java");
list.add("Advance Java");
System.out.println("Initial collection value:"+list);
Collections.addAll(list, "Servlet","JSP");
System.out.println("After adding elements collection value:"+list);
String[] strArr = {"C#", ".Net"};
Collections.addAll(list, strArr);
System.out.println("After adding array collection value:"+list);
}
}
click below button to copy the code. By - java tutorial - team
Initial collection value:[C, Core Java, Advance Java]
After adding elements collection value:[C, Core Java, Advance Java, Servlet, JSP]
After adding array collection value:[C, Core Java, Advance Java, Servlet, JSP, C#, .Net]
Java Collections Example: max()
import java.util.*;
public class CollectionsExample {
public static void main(String a[]){
List<Integer> list = new ArrayList<Integer>();
list.add(46);
list.add(67);
list.add(24);
list.add(16);
list.add(8);
list.add(12);
System.out.println("Value of maximum element from the collection: "+Collections.max(list));
}
}
click below button to copy the code. By - java tutorial - team
Value of maximum element from the collection: 67
Java Collections Example: min()
import java.util.*;
public class CollectionsExample {
public static void main(String a[]){
List<Integer> list = new ArrayList<Integer>();
list.add(46);
list.add(67);
list.add(24);
list.add(16);
list.add(8);
list.add(12);
System.out.println("Value of minimum element from the collection: "+Collections.min(list));
}
}
click below button to copy the code. By - java tutorial - team
Value of minimum element from the collection: 8