Spring Boot Tutorial
Spring Boot Tutorial
Download latest version of “Eclipse IDE for Java Developers”.
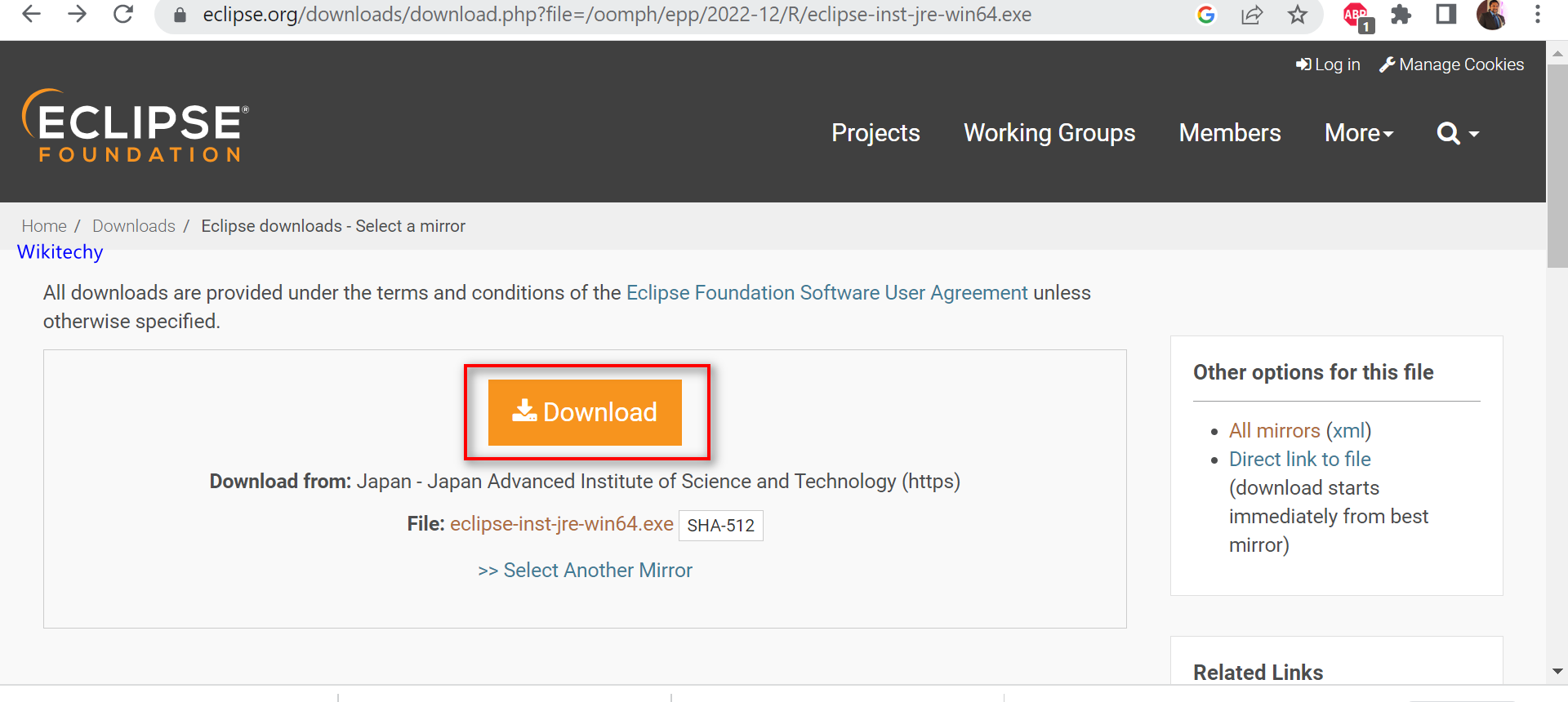
- Open the Spring initializr https://start.spring.io
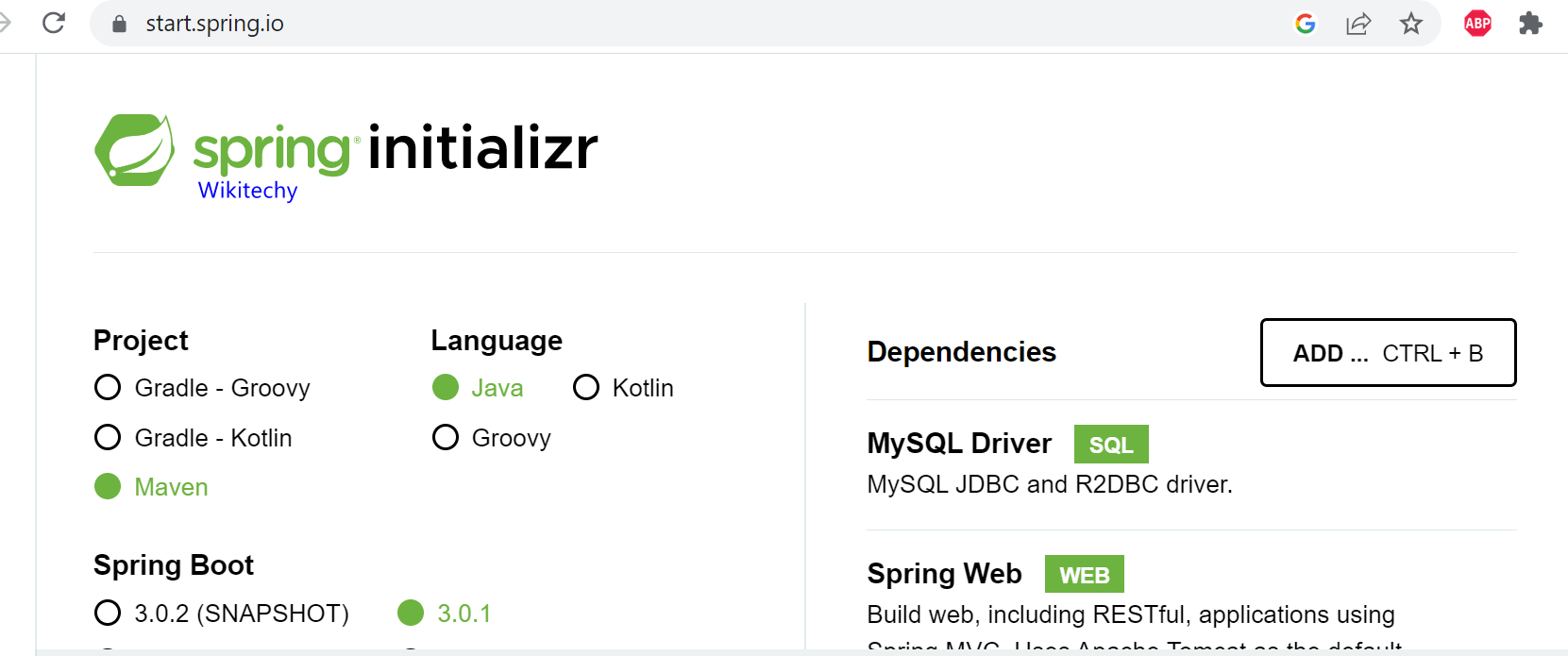
- Provide the Group and Artifact name. Now click on the Generate button.
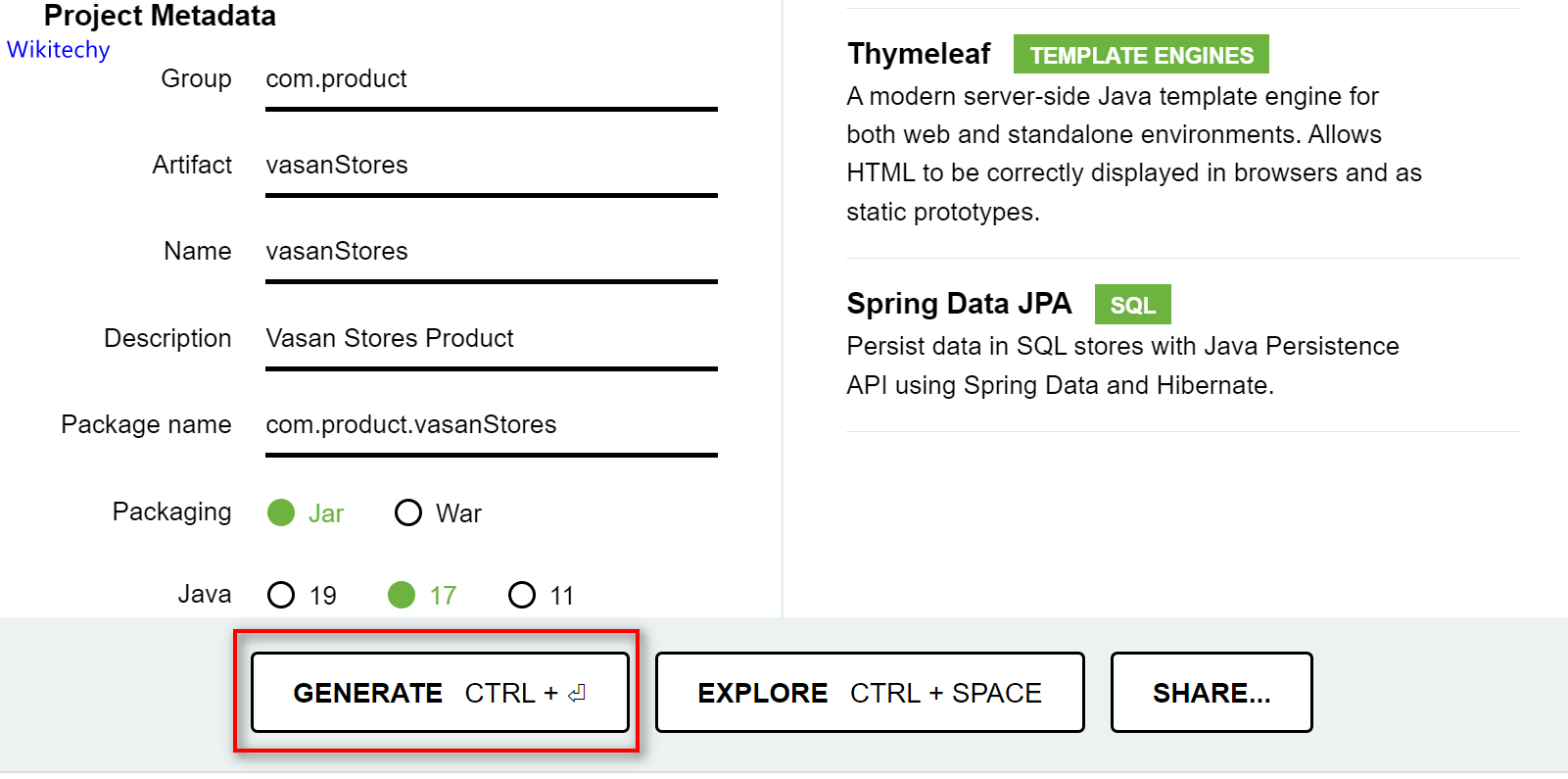
When we click on the Generate button, it starts packing the project in a .rar file and downloads the project. Extract the RAR file.
Double-click on file to Install the Eclipse. A new window will open. Click Eclipse IDE for Enterprise Java and Web Developers.
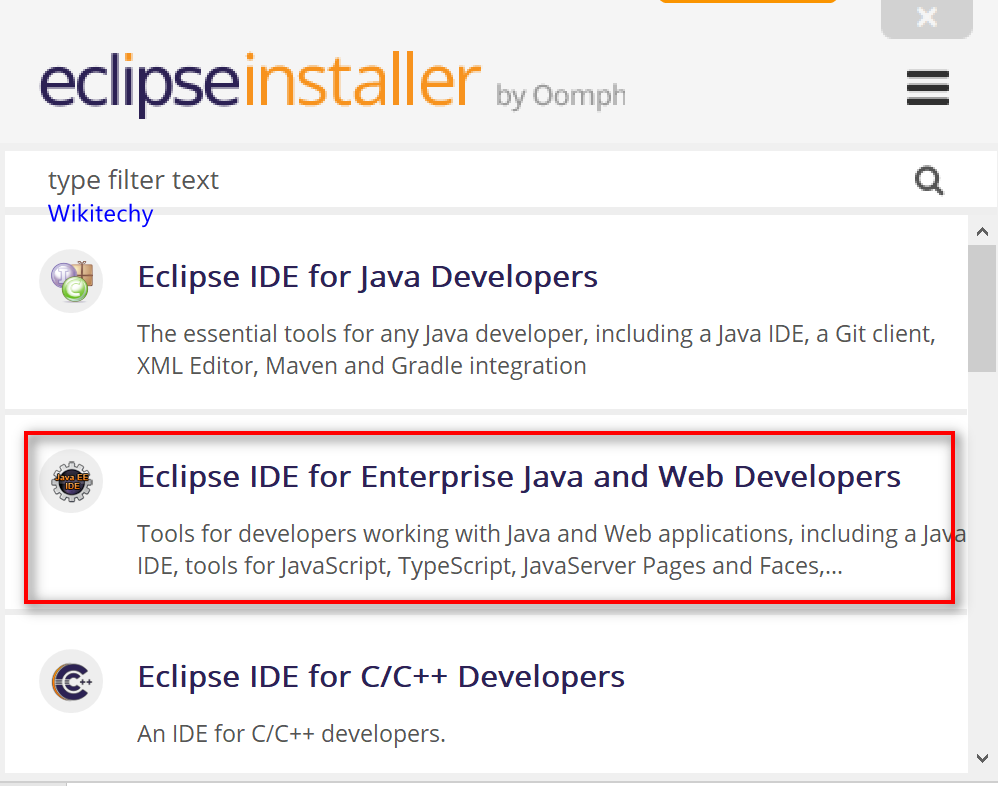
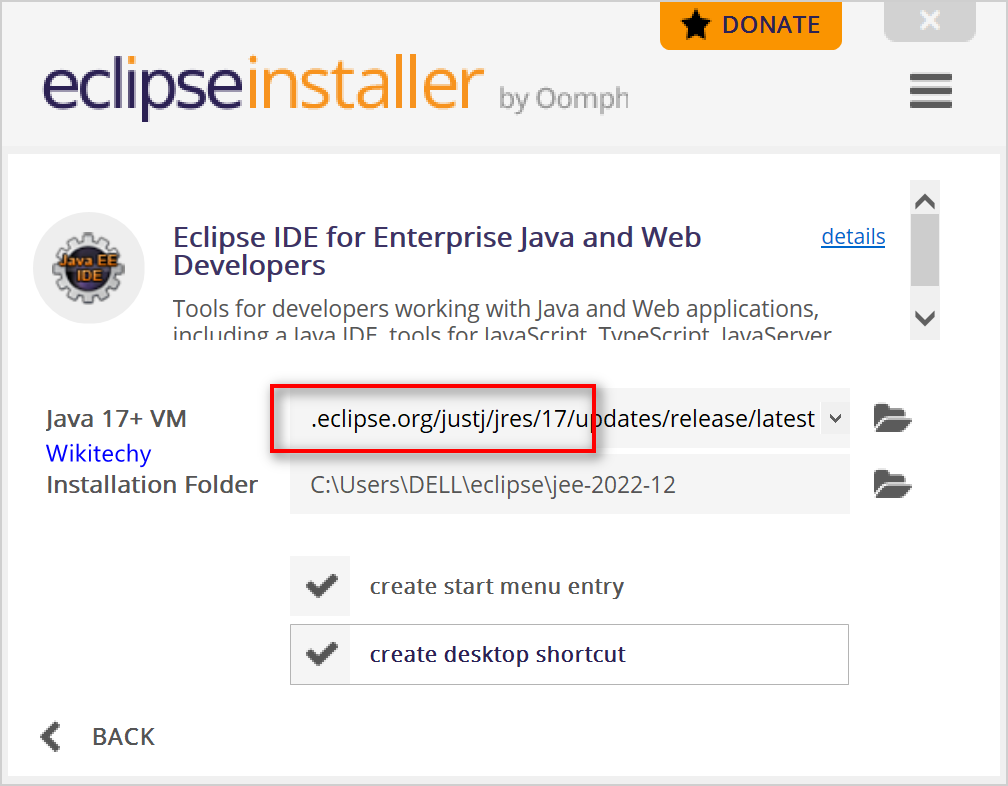
Now click on the Install button. By accepting the Agreement you can complete installation. It will take some time to complete the installation.
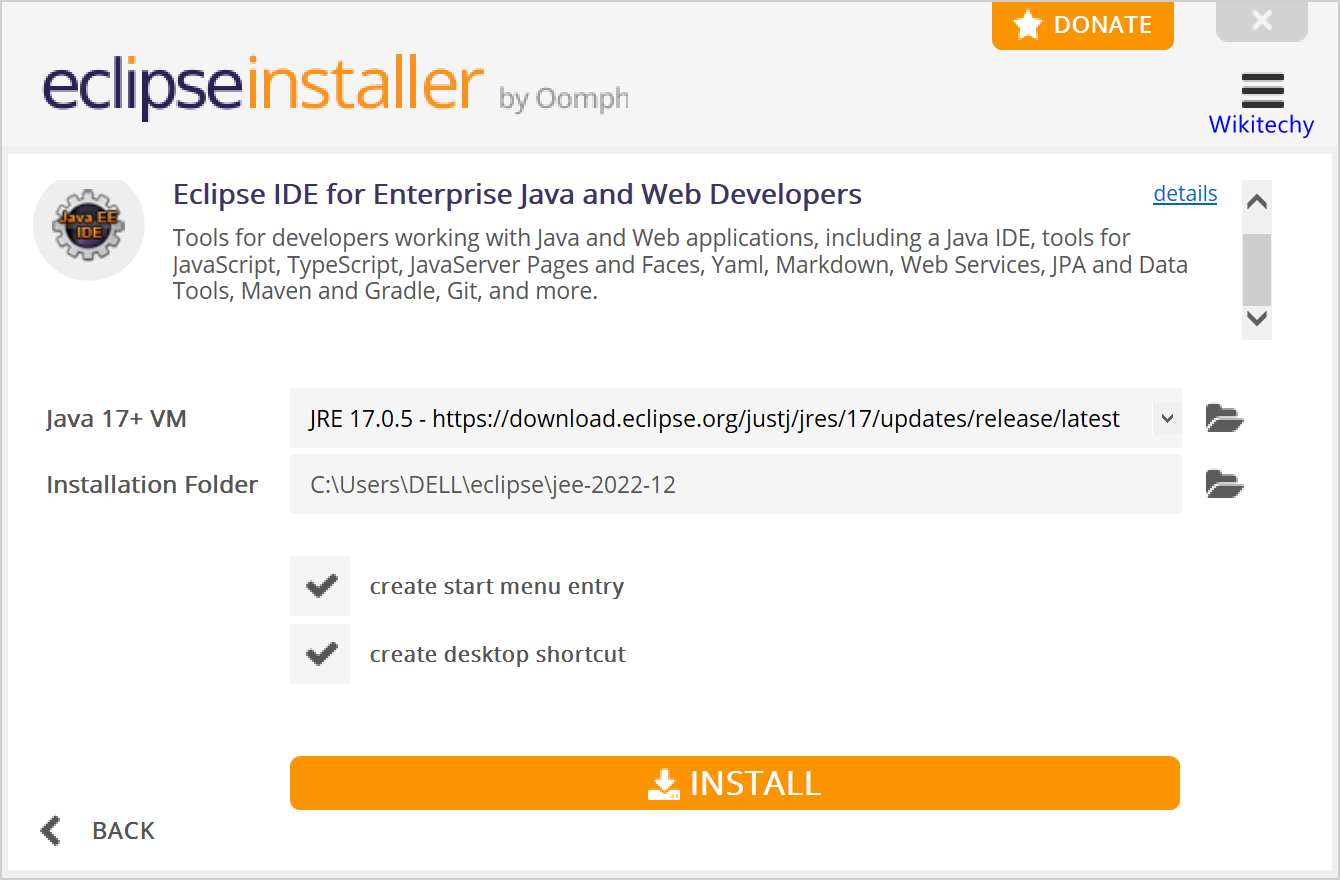
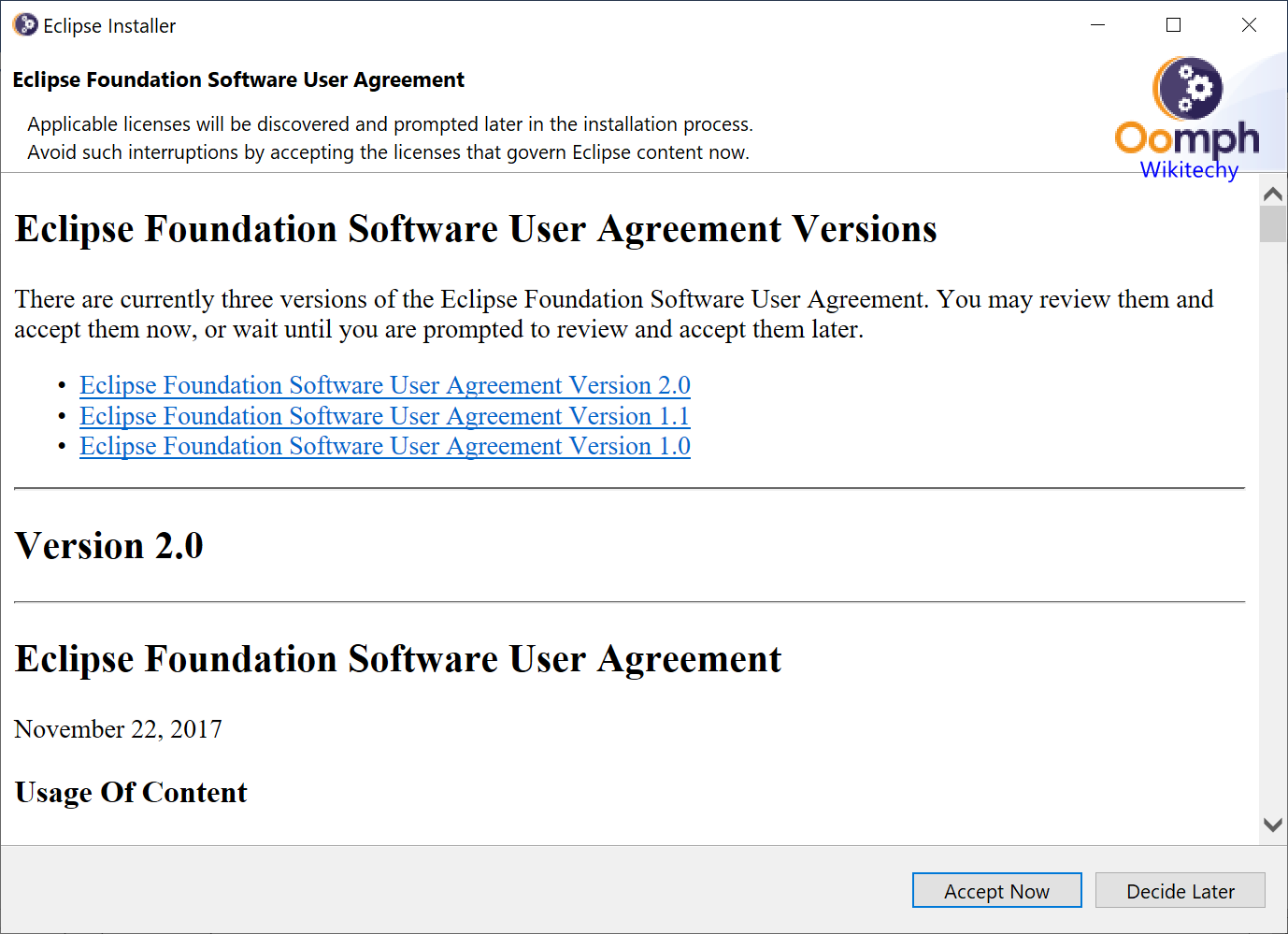
After successfully installed now click on the LUNCH button as shown below.
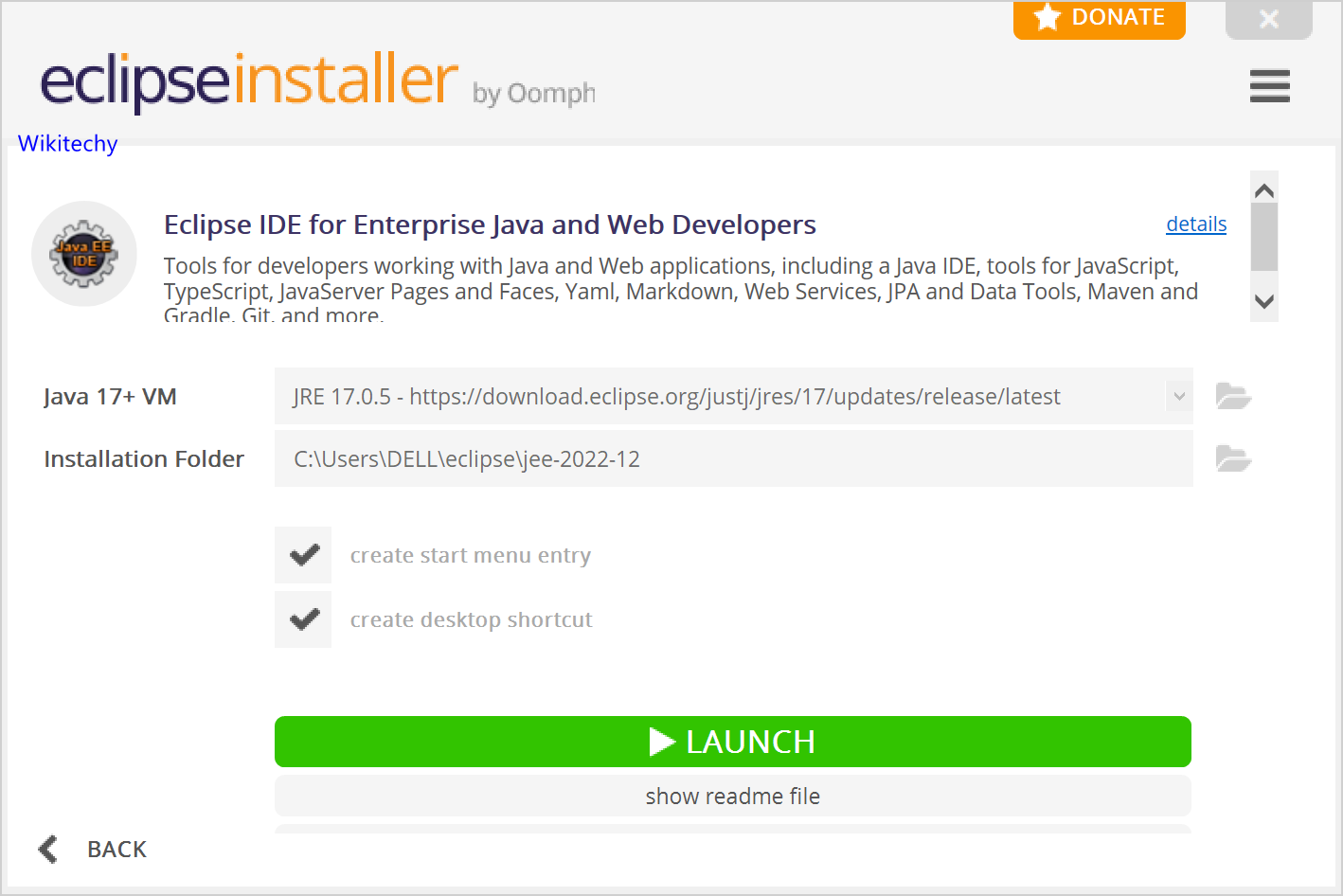
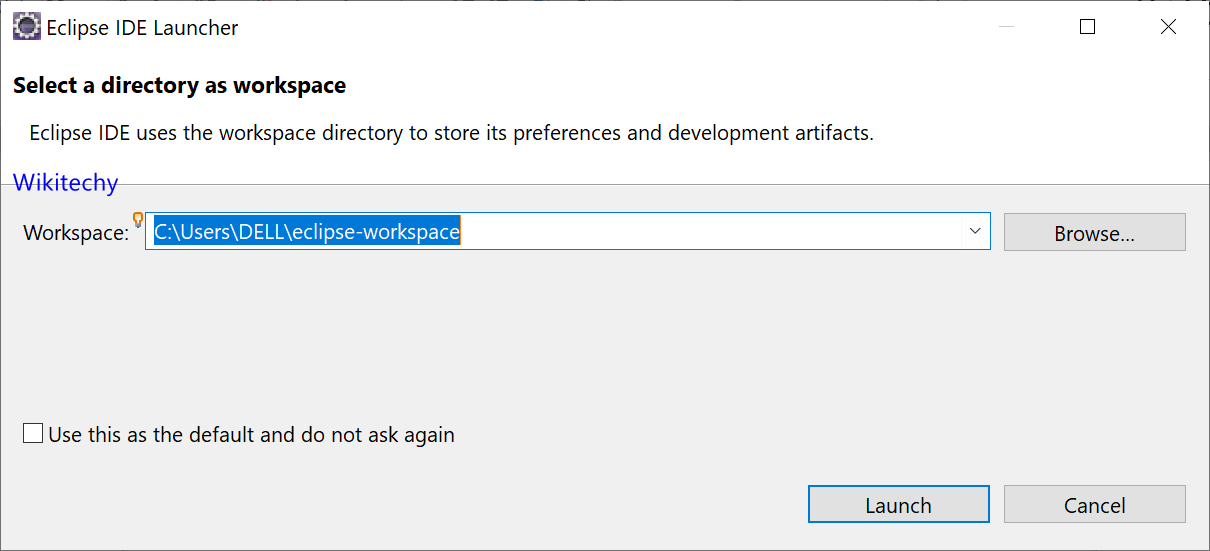
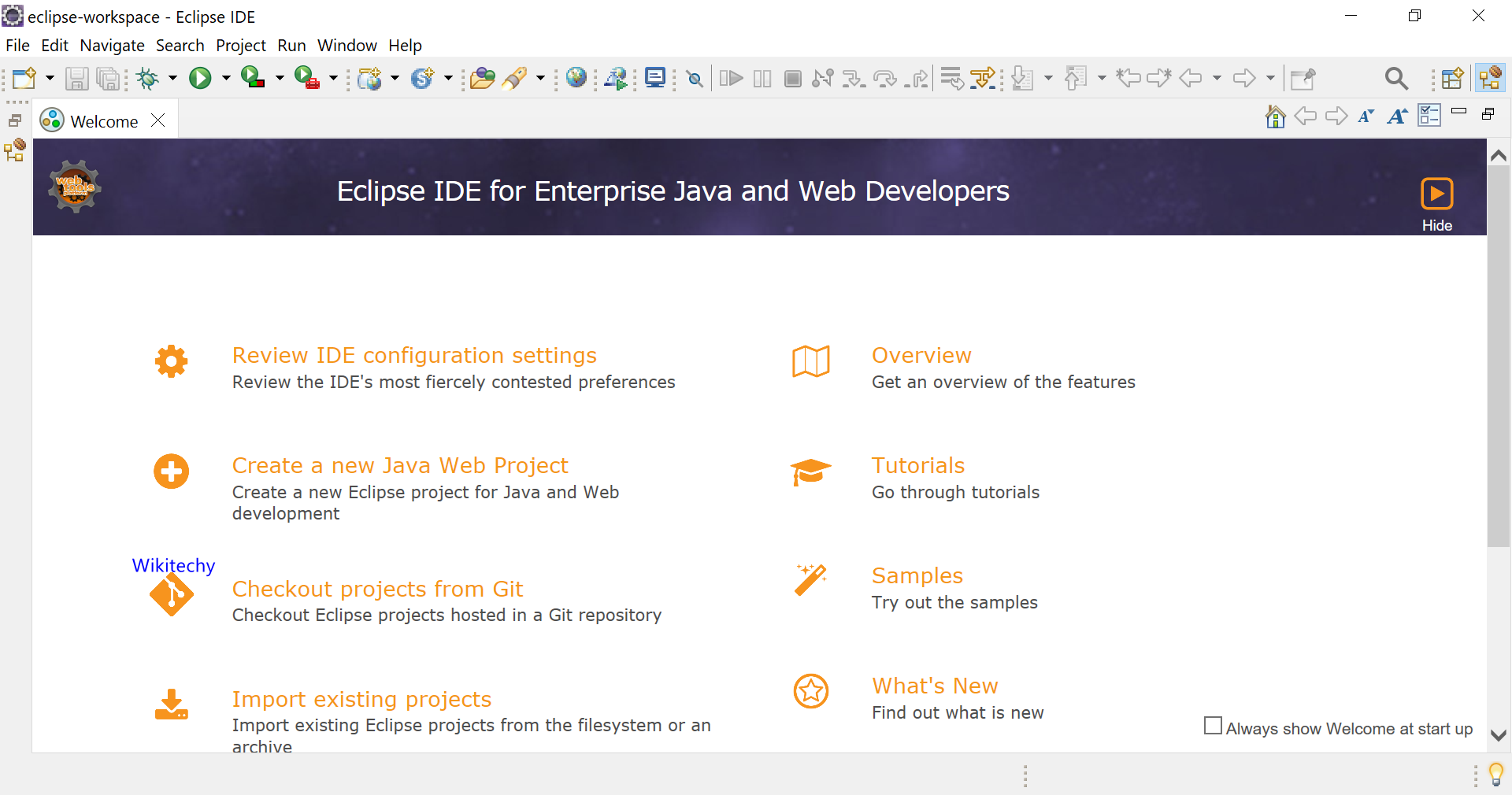
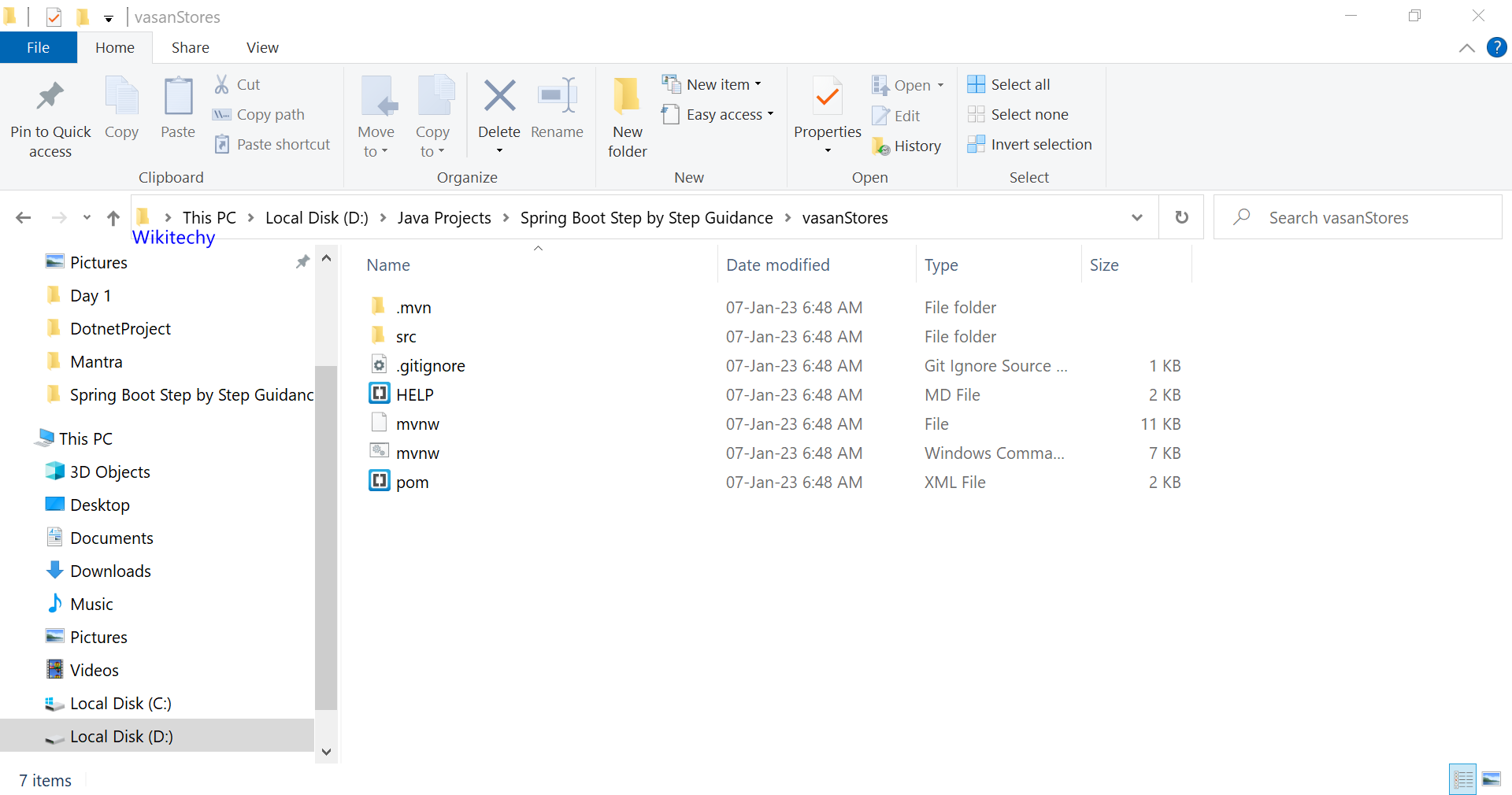
File -> Open Projects from file System
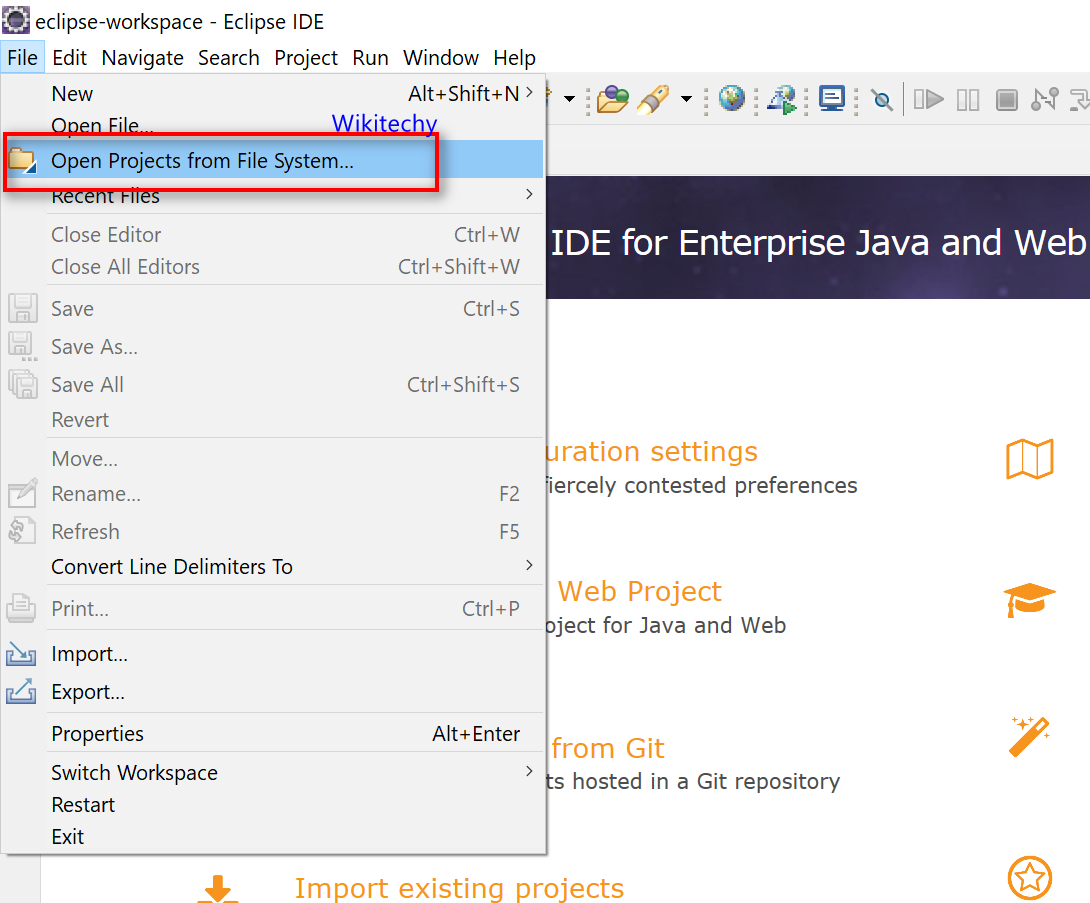
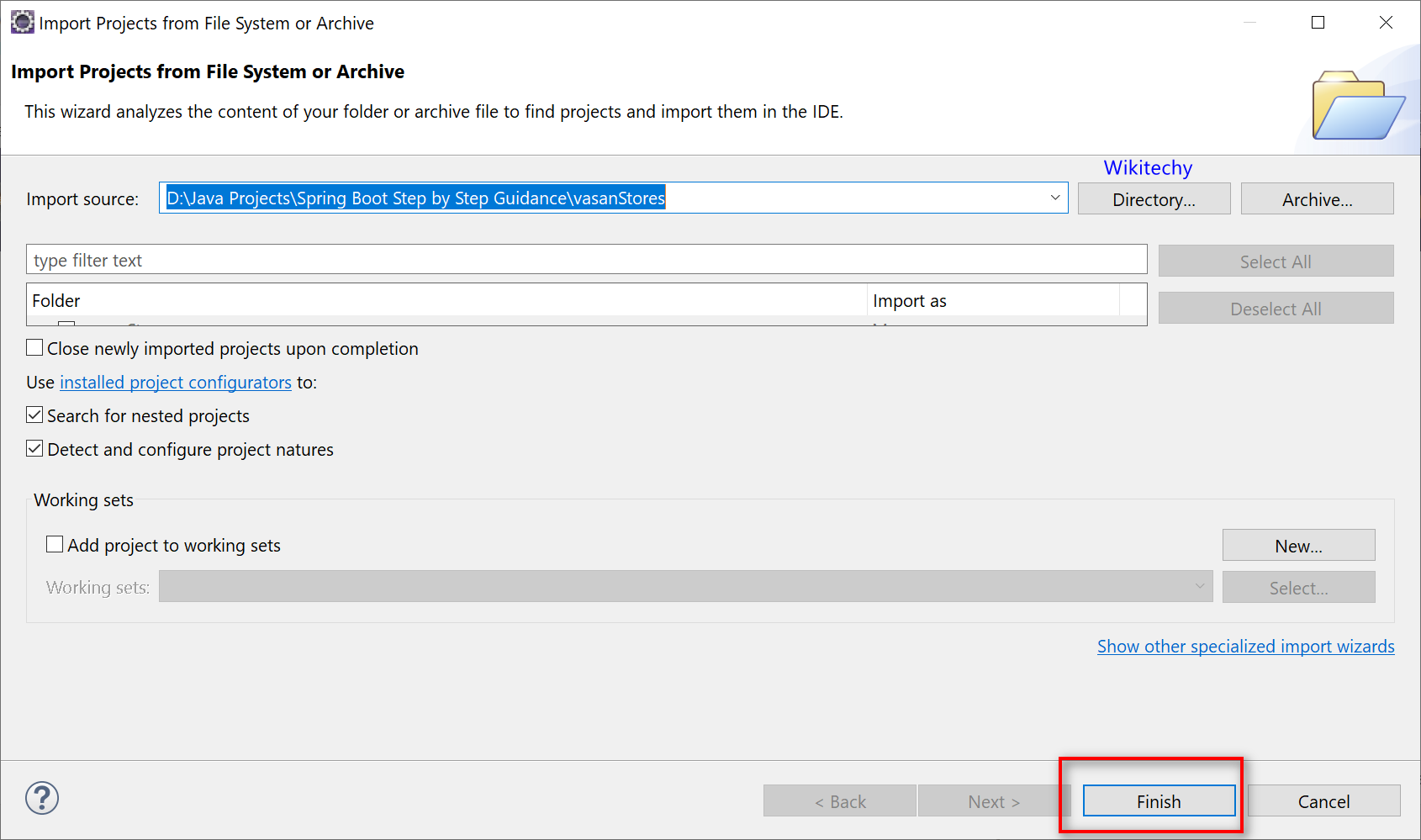
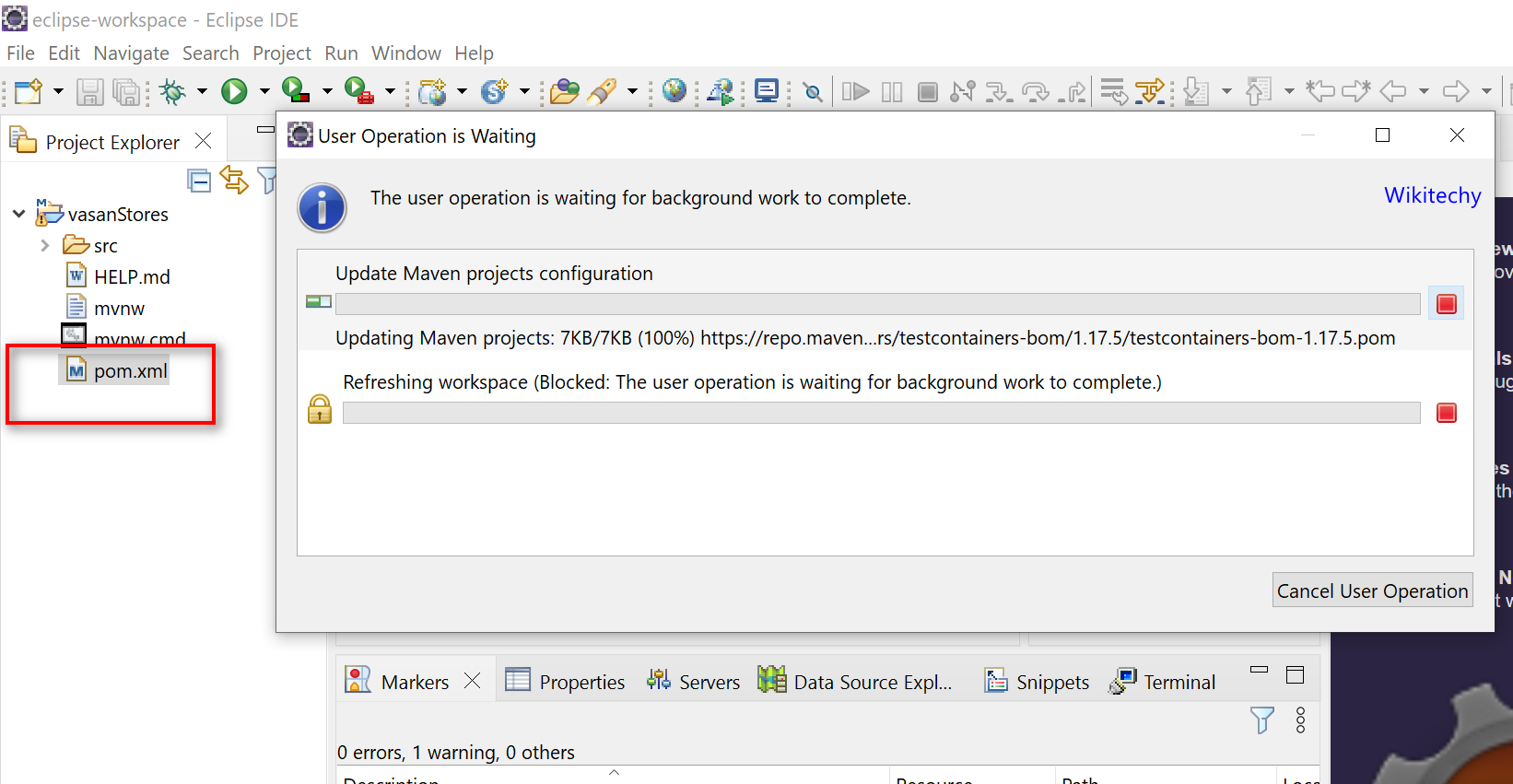
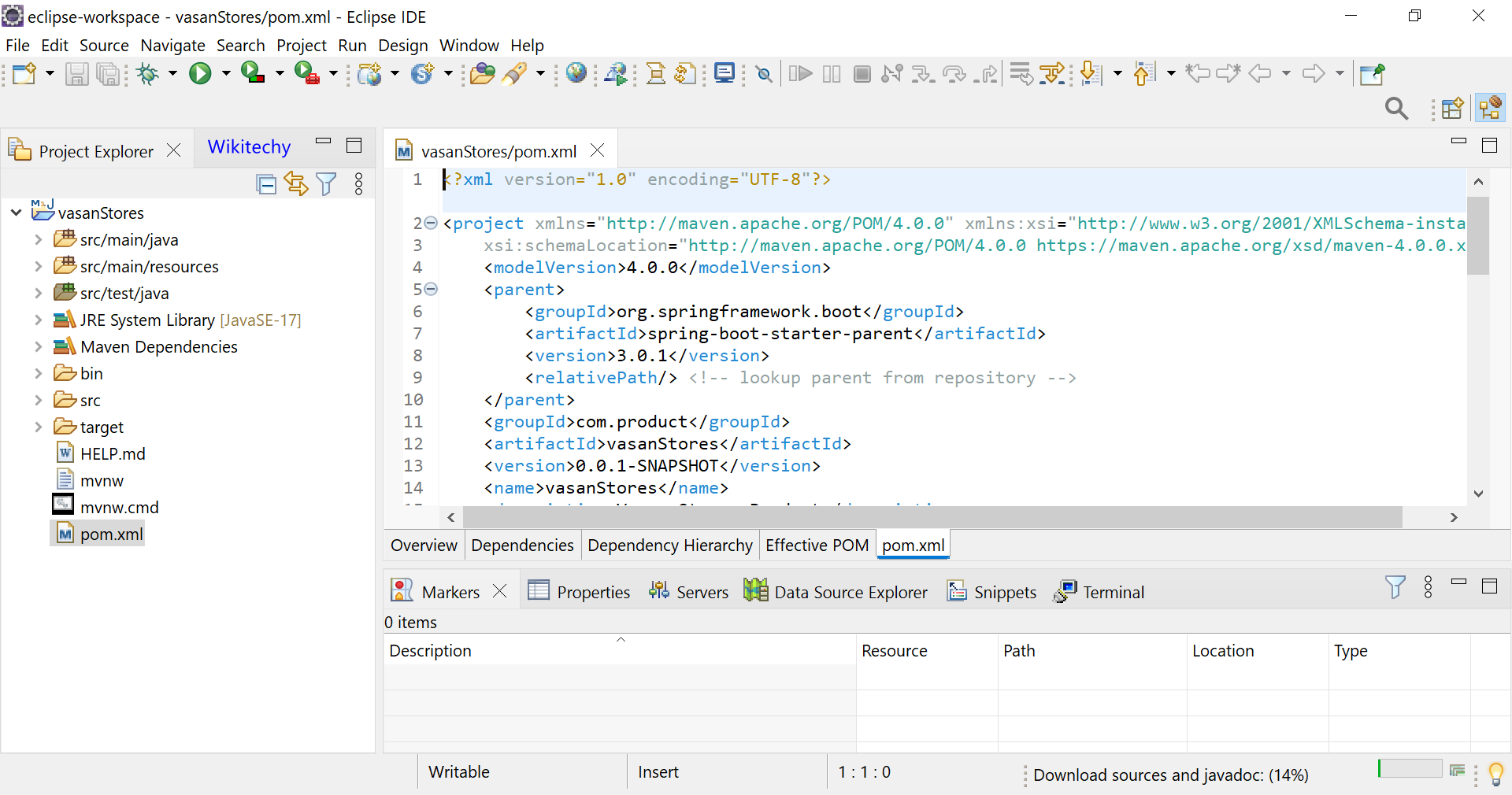

Right Click on Vasan Stores -> New -> Class [create a Java class]
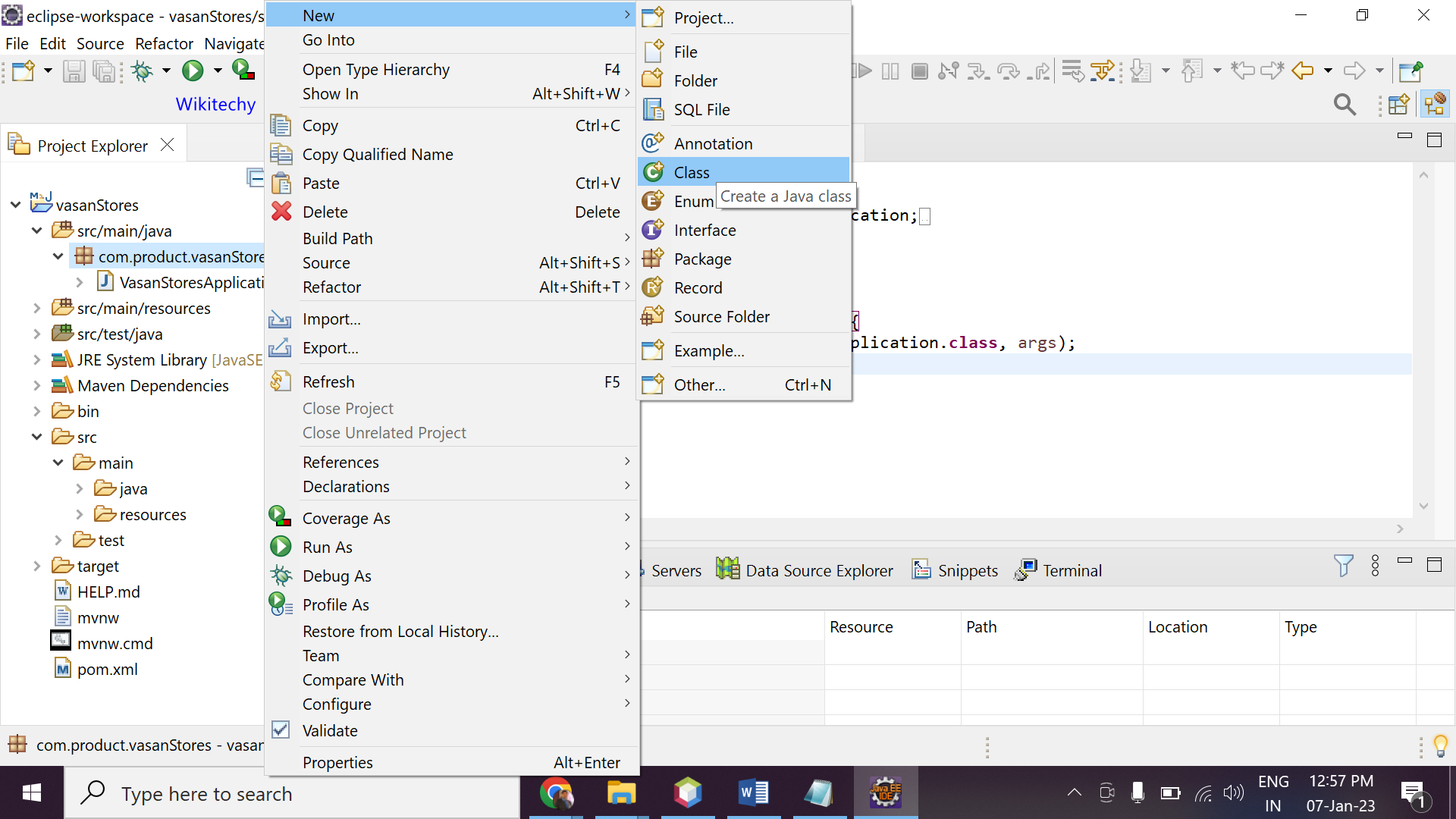
Create Class Name -> Click on Next
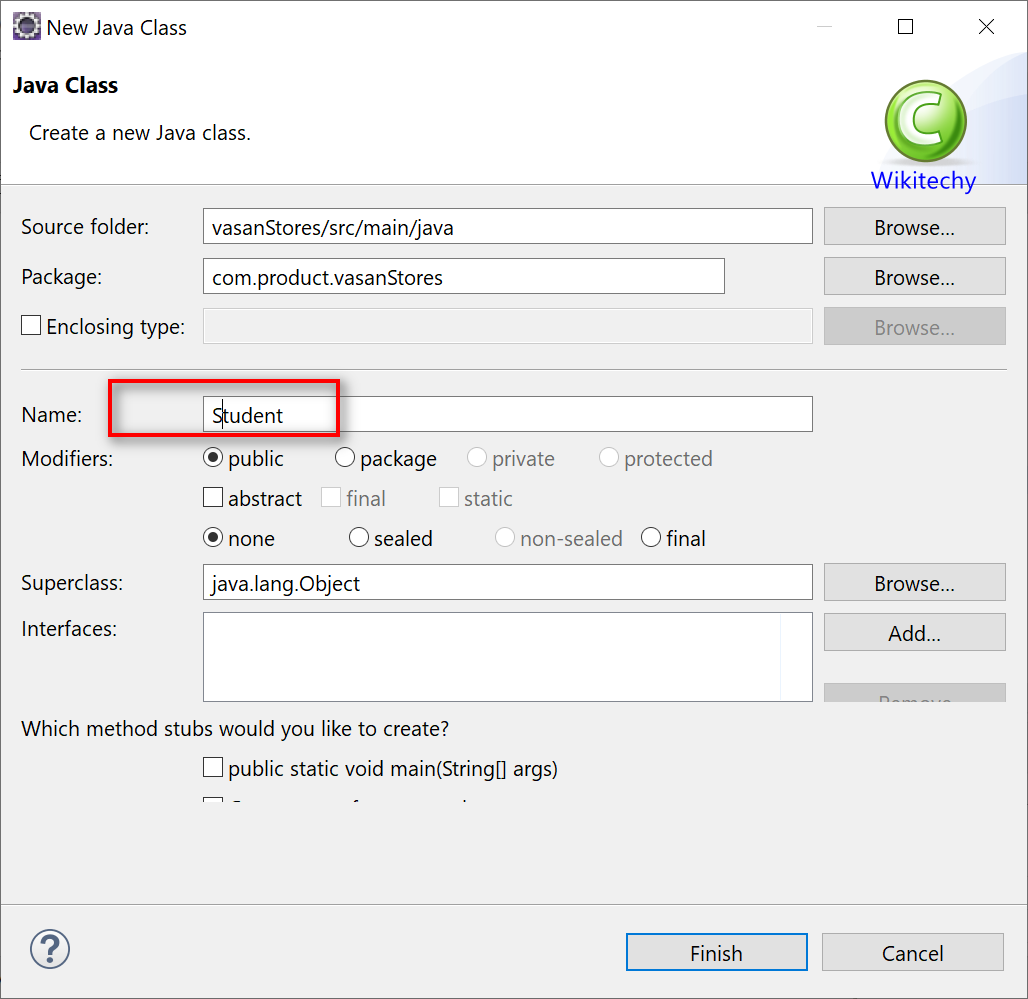
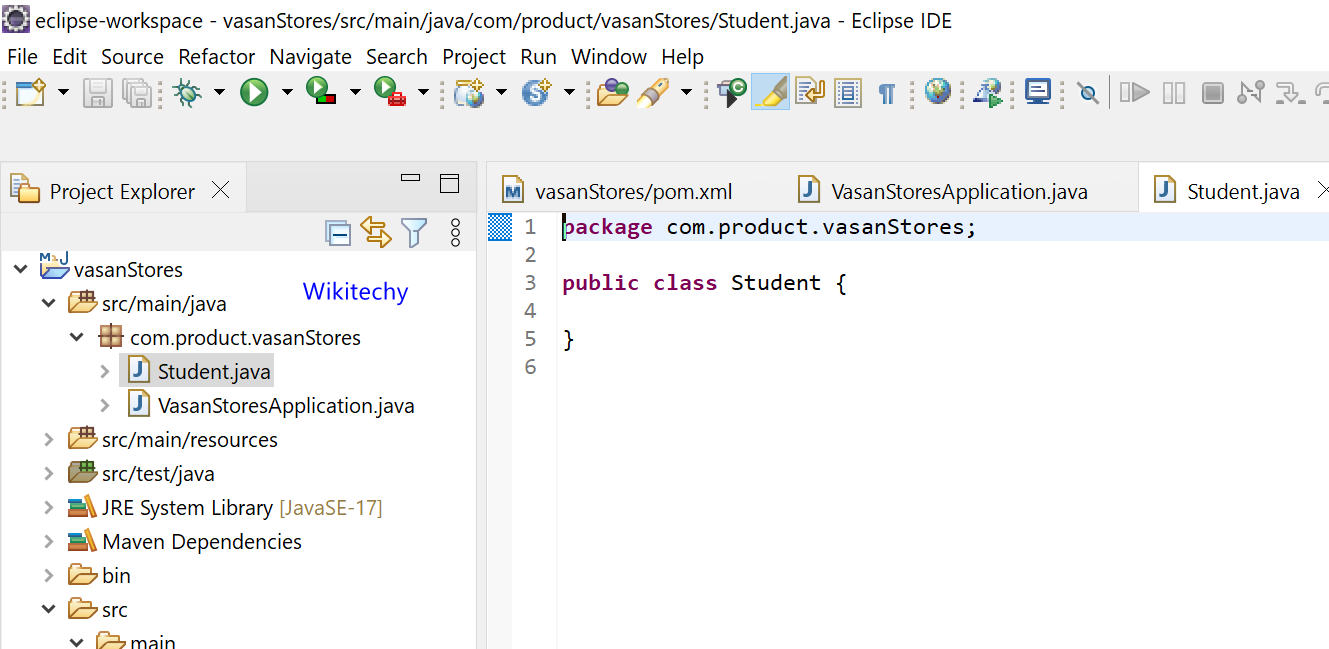
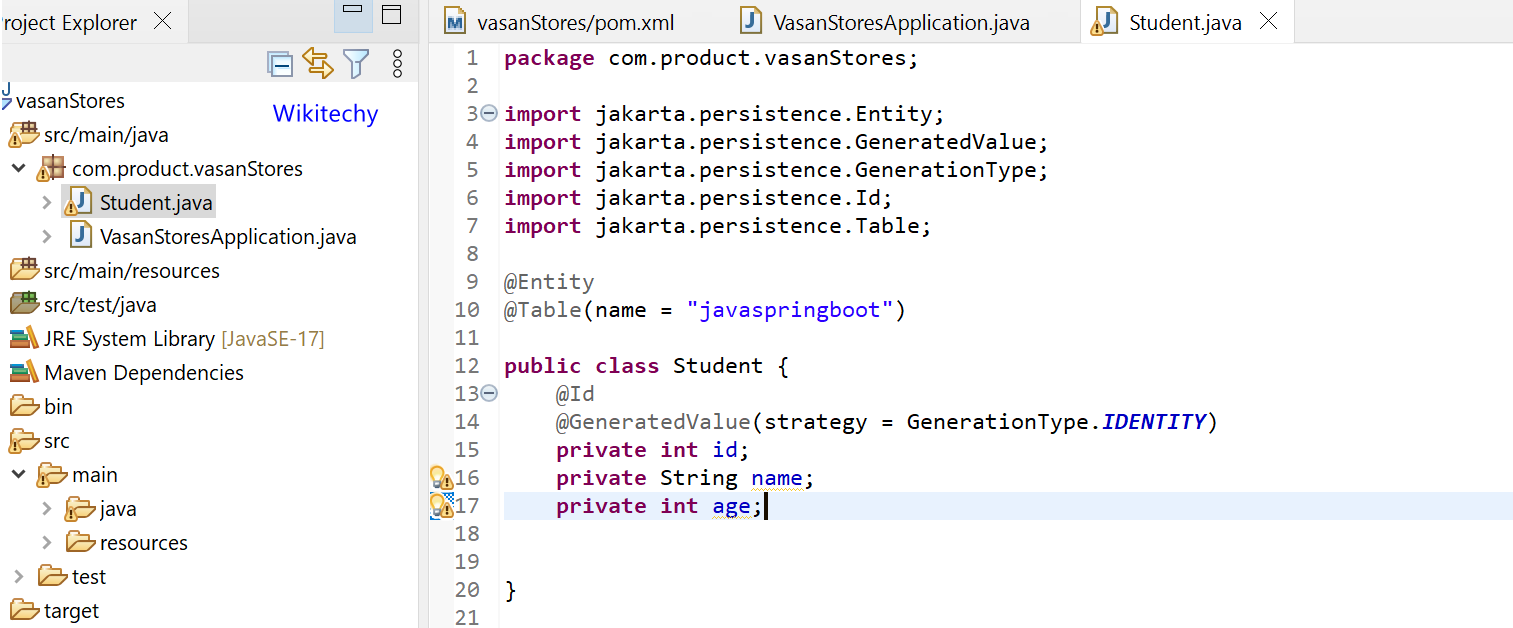
In coding Window, Right Click on Source -> Generate Getter and Setter Methods
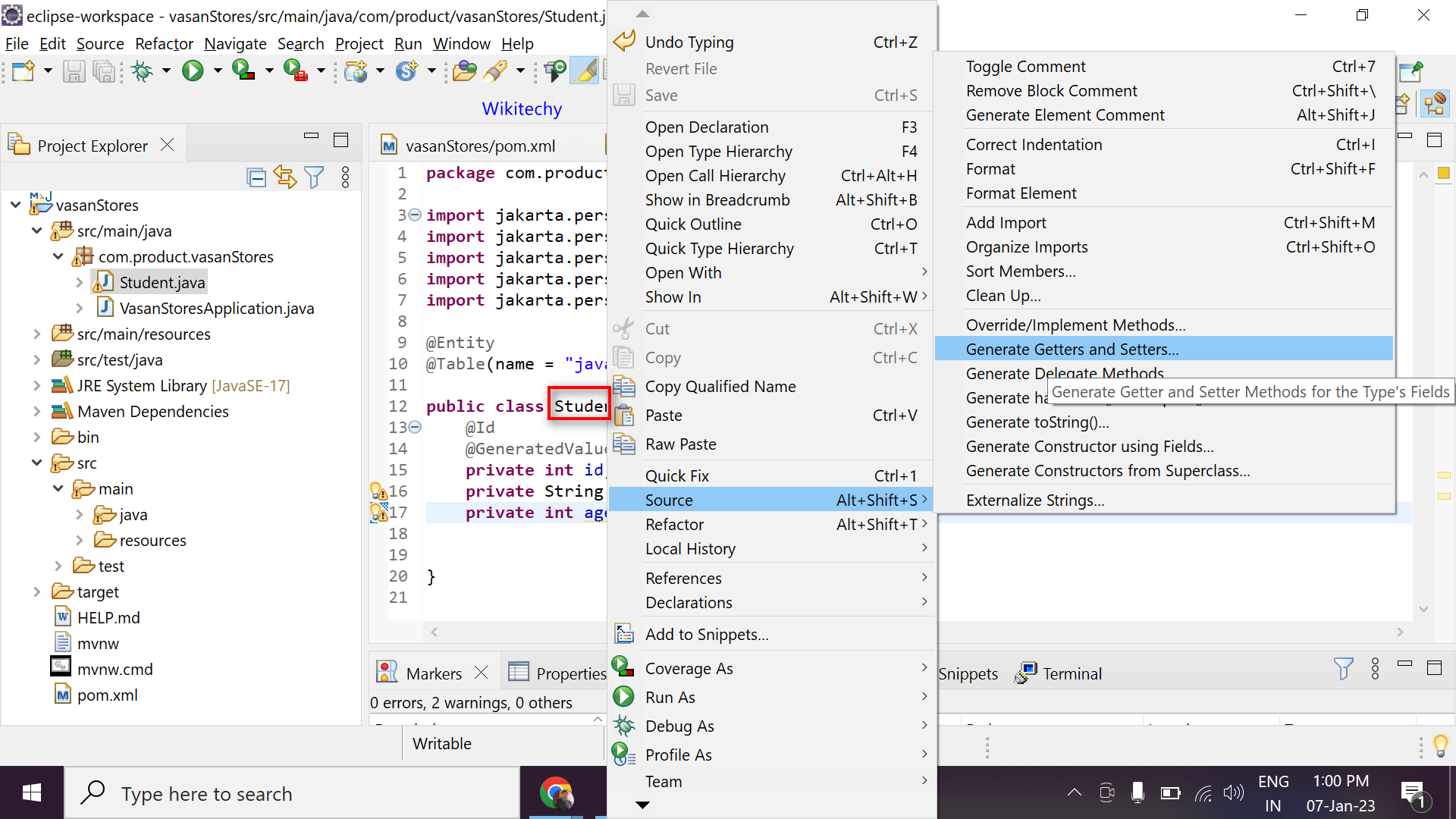
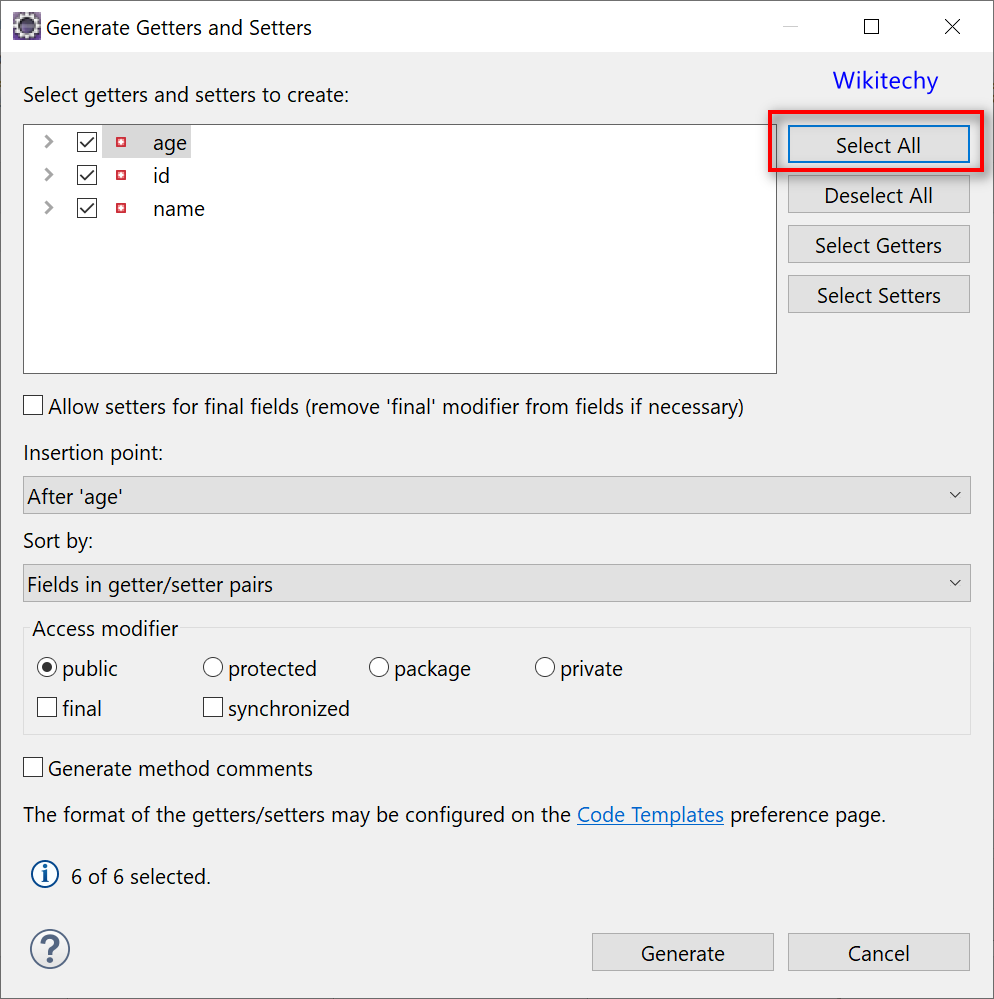
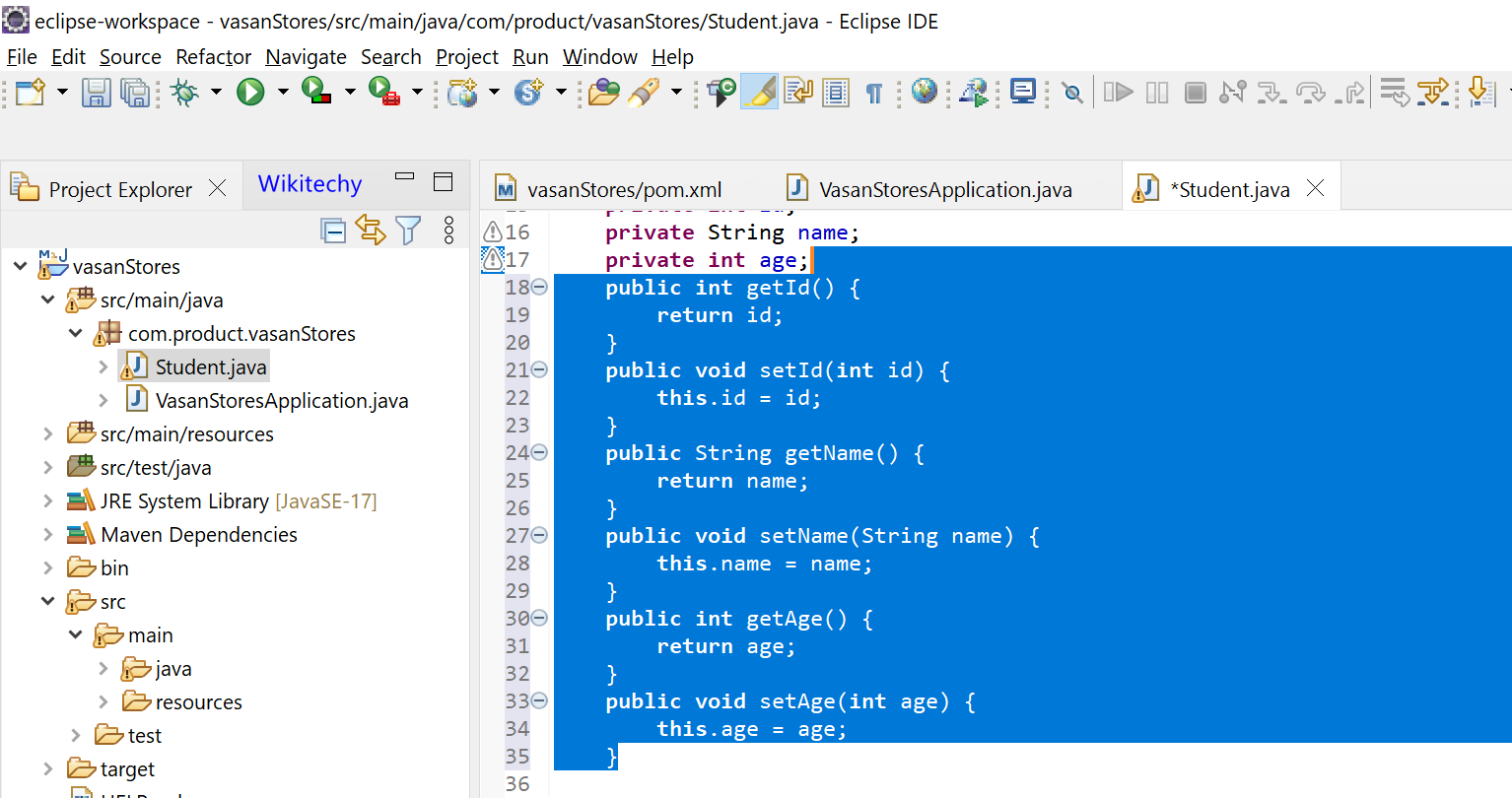
In coding Window, Right Click on Source -> Generate Constructors from Super Class
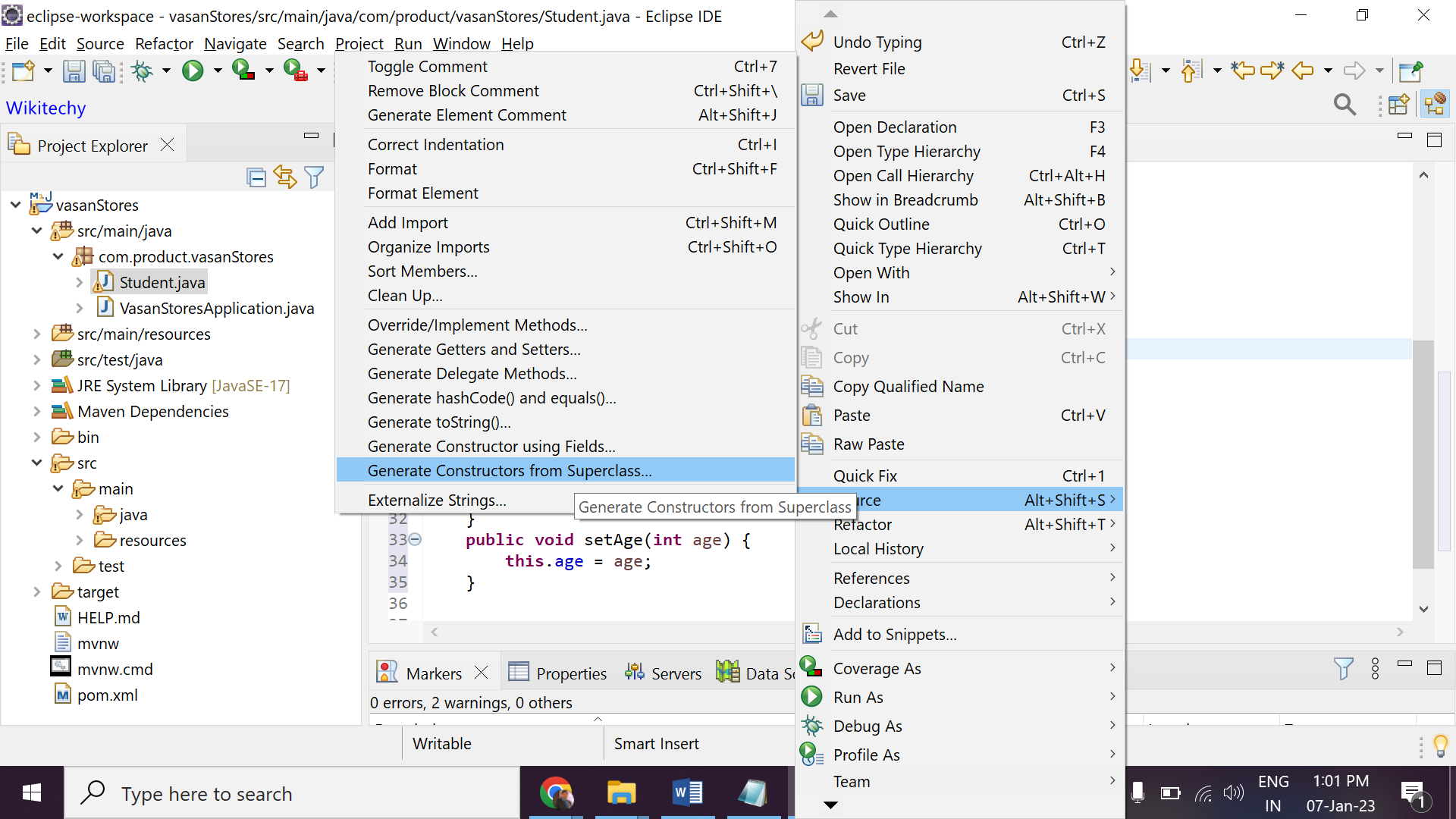
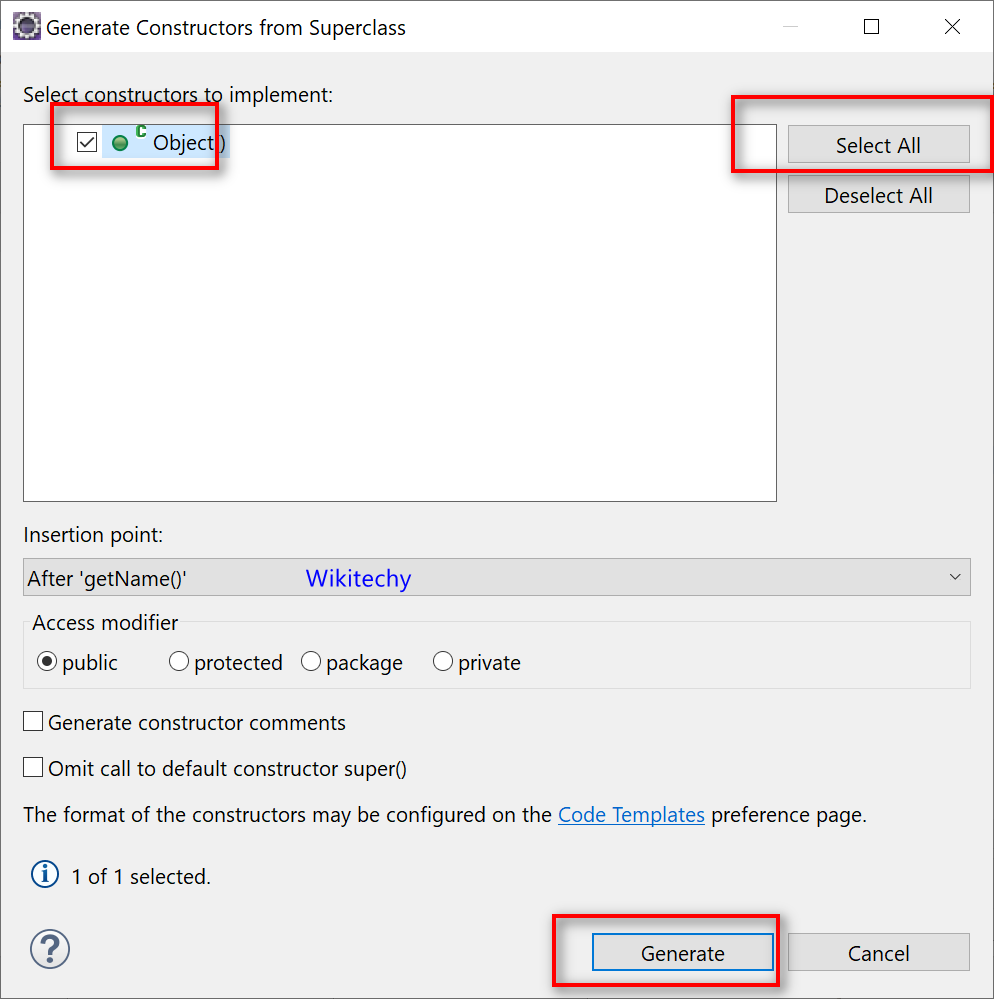
Spring Boot Login Registeration Page
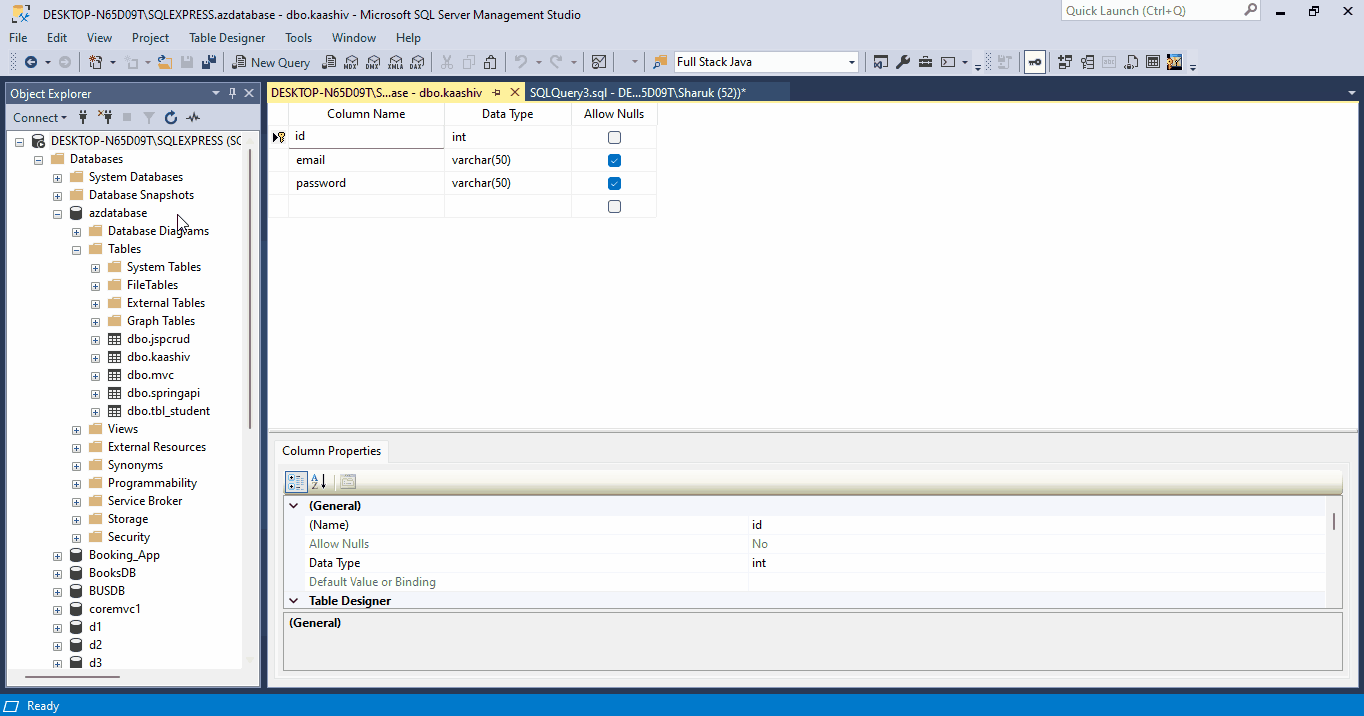
SQL Database Create
CREATE DATABASE azdatabase
SQL Table Create
USE azdatabase
Create Table kaashiv (
id int PRIMARY KEY IDENTITY(1,1) NOT NULL,
email varchar(50) NULL,
password varchar(50) NULL
)
SQL Store Procedure
create proc student_login(
@eamil varchar(50),
@password varchar(50)
)
as begin
select * from kaashiv where email = @eamil AND password = @password
end
create proc student_register(
@eamil varchar(50),
@password varchar(50)
)
as begin
insert into kaashiv values(@eamil, @password)
end
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.2.0</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.kaashiv</groupId>
<artifactId>signup.login</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>signup.login</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- Declar Your JDK Mssql Driver -->
<dependency>
<groupId>com.microsoft.sqlserver</groupId>
<artifactId>mssql-jdbc</artifactId>
<version>11.2.0.jre18</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
application.properties
spring.datasource.driver-class-name=com.microsoft.sqlserver.jdbc.SQLServerDriver
spring.datasource.url=jdbc:sqlserver://127.0.0.1:1433;databaseName=azdatabase;TrustServerCertificate=True
spring.datasource.username=YOUR_USER_NAME
spring.datasource.password=YOUR_PASSWORD
spring.jpa.show-sql=true
server.port=5000
StudentModel.java
package com.kaashiv.signup.login;
import jakarta.persistence.Entity;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.GenerationType;
import jakarta.persistence.Id;
import jakarta.persistence.Table;
@Entity
@Table(name = "kaashiv")
public class StudentModel {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private long id;
private String email;
private String password;
public StudentModel() {
super();
// TODO Auto-generated constructor stub
}
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
StudentRepository.java
package com.kaashiv.signup.login;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.Modifying;
import org.springframework.data.jpa.repository.Query;
import org.springframework.data.repository.query.Param;
import org.springframework.stereotype.Repository;
import org.springframework.transaction.annotation.Transactional;
@Repository
public interface StudentRepository extends JpaRepository<StudentModel, Integer> {
@Transactional
@Modifying
@Query(nativeQuery = true, value = "student_register :email , :password")
public void Student_Register(@Param("email") String email,@Param("password") String password);
@Query(nativeQuery = true, value = "student_login :email , :password")
public StudentModel Student_Login(@Param("email") String email,@Param("password") String password);
}
StudentController.java
package com.kaashiv.signup.login;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
@Controller
public class StudentController {
@Autowired
private StudentRepository repo;
@GetMapping("/register")
public String Register() {
return "register";
}
@PostMapping("/register-post")
public String RegisterPost(@ModelAttribute StudentModel std) {
repo.Student_Register(std.getEmail(),std.getPassword());
return "redirect:/";
}
@GetMapping("/")
public String Login() {
return "login";
}
@PostMapping("/login-post")
public String LoginPost(@ModelAttribute StudentModel std) {
StudentModel check = repo.Student_Login(std.getEmail(),std.getPassword());
if(check != null) {
return "index";
}
return "redirect:/";
}
}
login.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<title>Kaashiv - Login</title>
<style>
body {
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
}
</style>
</head>
<body>
<div class="container col-4 shadow" style="padding:30px">
<form th:action="@{/login-post}" method="post">
<h1 class="text-center">Kaashiv - Login</h1><br>
<label>Email :</label><br>
<input type="email" name="email" placeholder="Enter Email" class="form-control" required><br>
<label>Password :</label><br>
<input type="password" name="password" placeholder="Enter Password" class="form-control" required><br><br>
<input type="submit" value="Login" class="btn btn-outline-primary"><br><br>
<p>Don't have accounct <a href="/register"> Signup?</a></p>
</form>
</div>
</body>
</html>
register.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<title>Kaashiv - Register</title>
<style>
body {
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
}
</style>
</head>
<body>
<div class="container col-4 shadow" style="padding:30px">
<form th:action="@{/register-post}" method="post">
<h1 class="text-center">Kaashiv - Register</h1><br>
<label>Email :</label><br>
<input type="email" name="email" placeholder="Enter Email" class="form-control" required><br>
<label>Password :</label><br>
<input type="password" name="password" placeholder="Enter Password" class="form-control" required><br><br>
<input type="submit" value="Register" class="btn btn-outline-primary"><br><br>
<p>Already an accounct <a href="/"> Login </a></p>
</form>
</div>
</body>
</html>
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body {
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
}
</style>
</head>
<body>
<h1>Welcome to Kaashiv Infotech !</h1>
</body>
</html>