java tutorial - java operators | Basic Language Operators - java programming - learn java - java basics - java for beginners
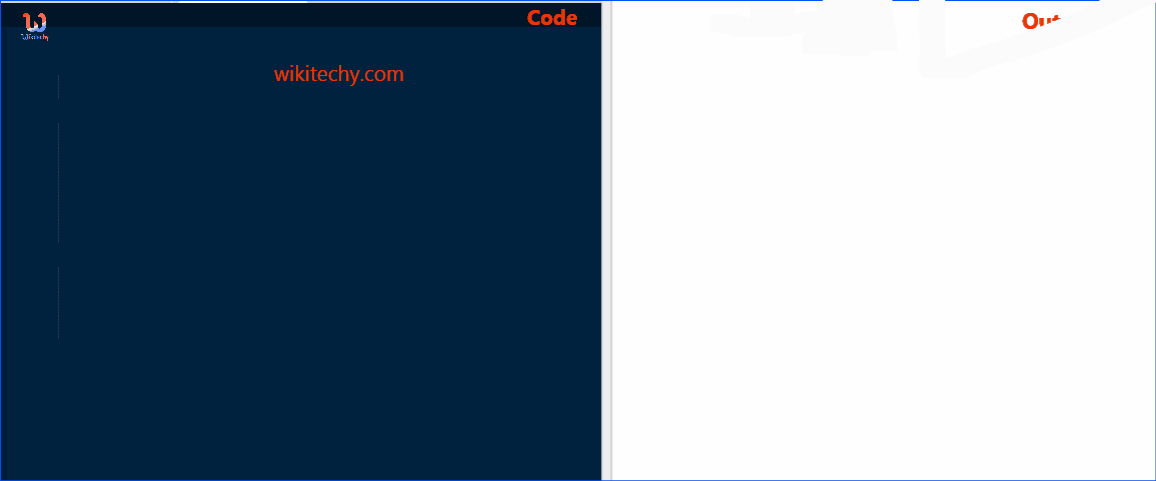
Learn java - java tutorial - java operators - java examples - java programs
The Java provides a rich set of operators for managing variables. Every statements can be separated into the following groups:
- arithmetic operators;
- comparison operators;
- bitwise operators;
- logical operators;
- assignment operators;
- other operators.
Arithmetic Operators
The Java Arithmetic operators are used in mathematical expressions in the same way as they are used in algebra also. Suppose the integer variable A is 10, and the variable B is 20. The given below table lists the arithmetic operators in Java:
Operator | Description | Example |
---|---|---|
+ | Adds values on both sides of the Operator | A + B will give 30 |
- | Subtracts right operand from left operand | A-B gives -10 |
* | Multiply values on both the sides of the Operator | A * B will give 200 |
/ | The division operator divides left operand by right operand | A / B gives 2 |
% | Divides the left operand by the right operand and returns the remainder | A% B gives 0 |
++ | The increment increases the value of the operand by 1 | B ++ will give 21 |
- | The decrement decreases the value of the operand by 1 | B-- will give 19 |
Sample Code
The following example shows software arithmetic operators. Copy and paste the following java code into the test.java file compile and then run this program:
public class Test {
public static void main(String args[]) {
int a = 10;
int b = 20;
int c = 25;
int d = 25;
System.out.println("a + b = " + (a + b));
System.out.println("a - b = " + (a - b));
System.out.println("a * b = " + (a * b));
System.out.println("b / a = " + (b / a));
System.out.println("b % a = " + (b % a));
System.out.println("c % a = " + (c % a));
System.out.println("a++ = " + (a++));
System.out.println("b-- = " + (a--));
// Check the difference in d ++ and ++ d
System.out.println("d++ = " + (d++));
System.out.println("++d = " + (++d));
}
}
click below button to copy the code. By - java tutorial - team
This will produce the following result:
a + b = 30
a - b = -10
a * b = 200
b / a = 2
b % a = 0
c % a = 5
a++ = 10
b-- = 11
d++ = 25
++d = 27
Comparison Operators
Here the following comparison operators supported in Java language. Suppose the variable A is 10 and the variable B is 20. The given below table lists relational operators or comparison operators in Java:
Operator | Description | Example |
---|---|---|
== | Checks whether two operands are equal or not, if so, then the condition becomes true | (A == B) - not valid |
! = | Checks if two operands are equal or not; if the values are not equal, then the condition becomes true | (A! = B) - the value is true |
& gt; | Checks if the given value of left operand is greater than value of right operand, if yes, then the given condition becomes true | (A & gt; B) - not true |
Checks if the value of left operand is less than value of right operand, if yes, then the given condition becomes true | (A & lt; B) - the value is true | |
& gt; = | Checks if the value of left operand is greater than or equal to value of right operand, if yes, then the given condition becomes true | (A & gt ; = B) - the value is not valid |
& lt; = | Checks if the value of left operand is less than or equal to the value of right operand, if yes, then the given condition becomes true | (A & lt; = B) - the value is true |
Sample Code
The following example shows the software comparison operators in Java. Copy and paste the following java code into the test.java file compile and then run this program:
public class Test {
public static void main(String args[]) {
int a = 10;
int b = 20;
System.out.println("a == b = " + (a == b) );
System.out.println("a != b = " + (a != b) );
System.out.println("a > b = " + (a > b) );
System.out.println("a < b = " + (a < b) );
System.out.println("b >= a = " + (b >= a) );
System.out.println("b <= a = " + (b <= a) );
}
}
click below button to copy the code. By - java tutorial - team
Output
a == b = false
a != b = true
a > b = false
a < b = true
b >= a = true
b <= a = false
Bitwise Operators
Java defines several bitwise operators that can be applied to integer types: int, long, short, char, and byte. In Java, a bitwise operator is working on bits and performs a bit-by-bit operation. Suppose, if a = 60; and b = 13; then in the binary format they will be the following:
a = 0011 1100
b = 0000 1101
-------
a & b = 0000 1100
a | b = 0011 1101
a ^ b = 0011 0001
~ a = 1100 0011
Assume the integer variable A is 60, and the variable B is 13. The following table lists the bitwise operators in Java:
Operator | Description | Example |
---|---|---|
& | The "AND" operator, copies the bit to the outcome if it exists in both of the operands | (A & B) will give 12, which is 0000 1100 |
| | The "OR" operator copies the bit if it exists in any operand | (A | B) will give 61 which is 0011 1101 |
^ | The binary "XOR" operator copies the bit if it is set in one operand, but not in both | (A ^ B) will give 49, which is 0011 0001 |
~ | The binary complement operator and has the effect of "reflection" bits | (~ A) will give -61, which is a complement 1100 0011 in the binary notation |
< < | The binary shift operator to the left. The value of the left operand moves to the left by the number of bits specified in the right operand | A < < 2 will give 240, which is 1111 0000 |
> > | The binary shift operator to the right. The value of the left operand moves to the right by the number of bits specified in the right operand | A > > 2 will give 15, which is 1111 |
>>> | The shift operator is the right of zero fill. The value of the left operand moves to the right by the number of bits specified in the right operand and the offset values are filled with zeros | A >>> 2 will give 15, which is 0000 1111 |
Sample Code
The following example shows program-by-bit operators in Java. Copy and paste the following java code into the test.java file compile and then run this program:
public class Test {
public static void main(String args[]) {
int a = 60; /* 60 = 0011 1100 */
int b = 13; /* 13 = 0000 1101 */
int c = 0;
c = a & b; /* 12 = 0000 1100 */
System.out.println("a & b = " + c );
c = a | b; /* 61 = 0011 1101 */
System.out.println("a | b = " + c );
c = a ^ b; /* 49 = 0011 0001 */
System.out.println("a ^ b = " + c );
c = ~a; /*-61 = 1100 0011 */
System.out.println("~a = " + c );
c = a << 2; /* 240 = 1111 0000 */
System.out.println("a << 2 = " + c );
c = a >> 2; /* 215 = 1111 */
System.out.println("a >> 2 = " + c );
c = a >>> 2; /* 215 = 0000 1111 */
System.out.println("a >>> 2 = " + c );
}
}
click below button to copy the code. By - java tutorial - team
Output
a & b = 12
a | b = 61
a ^ b = 49
~a = -61
a << 2 = 240
a >> 15
a >>> 15
Logical Operators
Suppose the logical variable A is true and the variable B stores false. The following table lists the logical operators in Java:
Operator | Description | Example |
---|---|---|
&& | The logical operator "AND" is called. If both operands are not equal to zero, then the condition becomes true | (A && B) - false |
|| | The logical operator "OR" is called. If either of the two operands is not equal to zero, then the condition becomes true | (A || B) - true |
! | The logical operator "NOT" is called. Use changes the logical state of its operand. If the condition is true, then the logical "NOT" operator will do false | ! (A && B) - true |
Sample Code
The following simple example shows the software logical operators in Java. Copy and paste the following java code into the test.java file compile and run this program:
public class Test {
public static void main(String args[]) {
boolean a = true;
boolean b = false;
System.out.println("a && b = " + (a&&b));
System.out.println("a || b = " + (a||b) );
System.out.println("!(a && b) = " + !(a && b));
}
}
click below button to copy the code. By - java tutorial - team
This will produce the following result:
a && b = false
a || b = true
!(a && b) = true
Assignment Operators
The given below assignment operators are supported by the Java:
Operator | Description | Example |
---|---|---|
= | A simple assignment operator, assigns the values from right side of the operands to left operand | C = A + B, assigns A + B to C |
+ = | The addition operator assigns the right-hand value to the left-hand operand | C + = A, is equivalent to C = C + A |
- = | The "Subtraction" assignment operator, it subtracts the left operand | C - = A from the right operand, is equivalent to C = C - A |
* = | Assignment operator "Multiplication", it multiplies the right operand by the left operand | C * = A is equivalent to C = C * A |
/ = | The assignment operator "division", it divides the left operand by the right operand | C / = A is equivalent to C = C / A |
% = | Assignment statement "Module", it takes a module with two operands and assigns its result to the left operand | C% = A, is equivalent to C = C% A |
< < = | The assignment operator "Shift left" | C < < = 2, it's like C = C < < 2 |
>> = | The "Right Shift" assignment Operator | C >> = 2, it's like C = C >> 2 |
& = | Bit assignment operator AND (AND) | C&= 2, it's like C = C& 2 |
^ = | The assignment operator is a bitwise exclusive "OR" ("XOR") | C ^ = 2, it's like C = C ^ 2 |
| = | Bit assignment operator "OR" | C | = 2, this is like C = C | 2 |
Sample Code
The following simple example shows the software logical operators in Java. Copy and paste the following java code into the test.java file compile and run this program:
public class Test {
public static void main(String args[]) {
int a = 10;
int b = 20;
int c = 0;
c = a + b;
System.out.println("c = a + b = " + c );
c += a ;
System.out.println("c += a = " + c );
c -= a ;
System.out.println("c -= a = " + c );
c *= a ;
System.out.println("c *= a = " + c );
a = 10;
c = 15;
c /= a ;
System.out.println("c /= a = " + c );
a = 10;
c = 15;
c %= a ;
System.out.println("c %= a = " + c );
c <<= 2 ;
System.out.println("c <<= 2 = " + c );
c >>= 2 ;
System.out.println("c >>= 2 = " + c );
c >>= 2 ;
System.out.println("c >>= a = " + c );
c &= a ;
System.out.println("c &= 2 = " + c );
c ^= a ;
System.out.println("c ^= a = " + c );
c |= a ;
System.out.println("c |= a = " + c );
}
}
click below button to copy the code. By - java tutorial - team
Output
c = a + b = 30
c += a = 40
c -= a = 30
c *= a = 300
c /= a = 1
c %= a = 5
c <<= 2 = 20
c >>= 2 = 5
c >>= 2 = 1
c &= a = 0
c ^= a = 10
c |= a = 10
Other operators
There are some other operators supported by the Java.
Ternary operator or Conditional operator (? :)
- A ternary operator that consists of three operands and it is used to evaluate expressions like boolean. A ternary operator in Java is also called as a conditional statement.
- The goal of a ternary operator or conditional statement is to decide what value should be assigned to a variable. The operator is written as:
variable x = (expression)? if true: if false
click below button to copy the code. By - java tutorial - team
Sample Code
Below is an example:
public class Test {
public static void main (String args []) {
int a, b;
a = 10;
b = (a == 1)? 20: 30;
System.out.println ("Value b:" + b);
b = (a == 10)? 20: 30;
System.out.println ("Value b:" + b);
}
}
click below button to copy the code. By - java tutorial - team
Output
Value of b: 30
Value of b: 20
The instanceof operator
The instanceof operator checks whether an object is of a specific type (type of class or interface type) and is used only for referenced object variables. The instanceof operator is written as:
(Reference object variable) instanceof (class / interface type)
click below button to copy the code. By - java tutorial - team
If the reference object variable on the left side of the statement is tested for the class / interface type on the right side, the result is true. Below is an example and description of the instanceof operator:
public class Test {
public static void main (String args []) {
String name = "Oleg";
// The following will return true, because the String type
boolean result = name instanceof String;
System.out.println (result);
}
}
click below button to copy the code. By - java tutorial - team
Output
true
This statement will still return true if the compared object is compatible with the type to the destination right. Below is another example:
class Vehicle {}
public class Car extends Vehicle {
public static void main(String args[]){
Vehicle a = new Car();
boolean result = a instanceof Car;
System.out.println( result );
}
}
click below button to copy the code. By - java tutorial - team
Output
true
Operator precedence in Java
- For example, x = 7 + 3 * 2. Here x is assigned a value of 13, not 20, because the operator "*" has a higher priority than "+", so first multiply "3 * 2", and then add "7 ".
- In the table, operators with the highest priority are placed at the top, and the priority level is reduced to the bottom of the table. In the expression, the high priority of operators in Java will be evaluated from left to right.
Category | Operator | Associativity |
---|---|---|
Postfix | () []. (point) | From left to right |
Unary | ++ - -! ~ | Right to left |
Multiplicative | * /% | From left to right |
Additive | + - | From left to right |
Shift | >> >>> < < | From left to right |
Relational | >> = <<= | From left to right |
Equality | ==! = | From left to right |
Bitwise AND (AND) | & | From left to right |
Bitwise exclusive "OR" ("XOR") | ^ | From left to right |
Bitwise OR (OR) | | | From left to right |
Logical "AND" | && | From left to right |
Logical OR (OR) | || | From left to right |
Conditional | ?: | Right to Left |
Assignment | = + = - = * = / =% = >> = < < = & = ^ = | = | Right to left |
Comma | , | From left to right |