Identifiers in Java
What is a Java Identifier ?
- A Java identifier is a name given to a package, class, interface, method, or variable. It allows a programmer to refer to the item from other places in the program.
- To make the most out of the identifiers you choose, make them meaningful and follow the standard Java naming conventions.
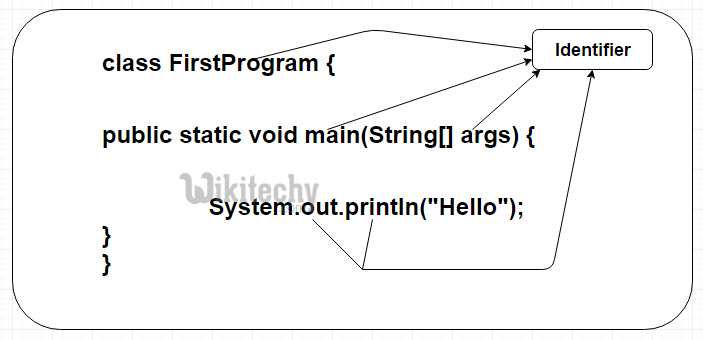
Learn java - java tutorial - Identifiers in java - java examples - java programs
What is a Valid Identifier in Java ?
- In Java, all identifiers must begin with a letter, an underscore, or a Unicode currency character.
- Any other symbol, such as a number, is not valid. An identifier cannot have the same spelling as one of Java's reserved words.
Examples of Java Identifiers :
- If you have variables that hold the name, height, and weight of a person.
String name = "John";
int weight = 300;
double height = 6;
System.out.printf("My name is %s, my height is %.0f foot and my weight is %d pounds. D'oh!%n", name, height, weight);
About Java Identifiers
- Reserved words like class, continue, void, else, and if cannot be used. Check that link for even more reserved words to avoid
- "Java letters" is the term given to the acceptable letters that can be used for an identifier. This includes not only regular alphabet letters but also symbols, which just includes, without exception, the underscore (_) and dollar sign ($)
- "Java digits" include the numbers 0-9
- An identifier can begin with a letter, dollar sign, or underscore, but not a digit. However, it's important to realize that digits can be used so long as they exist after the first character, likee8xmple
- Java letters and digits can be anything from the Unicode character set, which means characters in Chinese, Japanese, and other languages can be used
- Spaces are not acceptable, so an underscore can be used instead
- The length does not matter, so you can have a really long identifier if you choose to
- A compile-time error will occur if the identifier uses the same spelling as a keyword, the null literal, or boolean literal
- Since the list of SQL keywords may, at some point in the future, include other SQL words (and identifiers can't be spelled the same as a keyword), it's usually not recommended that you use an SQL keyword as an identifier
- It's recommended to use identifiers that are related to their values so they're easier to remember
- Variables are case-sensitive, which means myvalue does not mean the same as MyValue
- Following the rules above, these identifiers would be considered legal:
- _variablename
- _3variable
- $testvariable
- VariableTest
- variabletest
- this_is_a_variable_name_that_is_long_but_still_valid_because_of_the_underscores
- max_value
- Here are some examples of identifiers that are not valid because they disobey the rules mentioned above:
- 8example(this starts off with a digit)
- exa+ple (the plus sign isn't allowed)
- variable test (spaces are not valid)
- this_long_variable_name_is_not_valid_because_of_this-hyphen (while the underscores are acceptable like in the example from above, even the one hyphen in this identifier renders it invalid)