java tutorial - Java - Classes and Objects - java programming - learn java - java basics - java for beginners
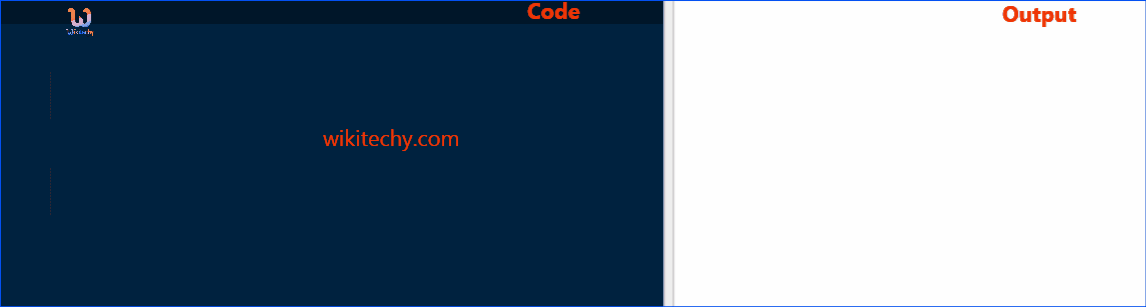
Learn Java - Java tutorial - Java classes and objects - Java examples - Java programs
Java is an object-oriented programming language. As a language that has an object-oriented function, it supports the following basic concepts:
- polymorphism;
- inheritance;
- encapsulation;
- abstraction;
- classes;
- objects;
- copy;
- method;
- parsing.
In this lesson, we'll look at Java objects and classes , their concepts.
- A class can be defined as a template (indicated in green), which describes the behavior of the object, which in turn has state and behavior. It is an instance of the class.
- For example: a dog can have a state - color, name, as well as behavior - nodding, barking, eating.
Objects in Java
- Let us now look deep into what is an object. If we look at the real world, we will find many objects around us, cars, dogs, people, etc. All of them have a state and a way of life.
- If you consider a dog, then its condition is a name, breed, color, and a way of life - barking, tail wagging, running.
- If you compare a software object in Java with objects from the real world, then they have very similar characteristics, they also have a state and behavior. Program state is stored in fields, and behavior is displayed through methods.
- Thus, in software development, methods work on the internal state of the object, and communication with others is accomplished through methods.
Classes in Java
The class from which individual objects are created is indicated in green. An example of creating a class in Java is shown below:
public class Dog{
String breed;
int age;
String color;
void barking(){
}
void hungry(){
}
void sleeping(){
}
}
click below button to copy the code. By - java tutorial - team
A class can contain any of the following kinds of variables:
- Local variables defined within methods, constructors, or blocks. They will be declared and initialized in the method, and will be destroyed when the method is completed.
- Instance variables are variables within the class, but also outside any method. They are initialized at boot time. Instance variables can be accessed from within any method, constructor, or blocks of this particular class.
- Class variables or static class variables in Java are declared in the class outside of any method using a static keyword.
In Java, classes can have any number of methods for accessing the value of different kinds of methods. In the above example, barking (), hungry (), and sleeping () are methods. Further, some of the important topics that should be considered for understanding the meaning of classes and objects in a programming language are mentioned
Class constructor
- When discussing a class issue, one of the most important subtopics in Java is the constructor. Each class has a constructor. If we do not write it or, for example, forget it, the compiler will create it by default for this class.
- Each time a new object is created in Java, at least one constructor will be called. The main rule is that they must have the same name as the class, which can have more than one constructor.
An example of the constructor is shown below:
public class Puppy{
public Puppy(){
}
public Puppy(String name){
// So the constructor in Java looks like and it has one parameter, name.
}
}
click below button to copy the code. By - java tutorial - team
Note: in the following sections we will discuss in more detail if we have two different types of constructors.
Create an object
The options for creating an object in the class are:
- Announcement : declaration of a variable with the name of a variable with the type of the object.
- Instantiation : a "new" keyword is used.
- Initialization : A "new" keyword is followed by a call to the constructor. This call initializes a new object.
An example is given below:
public class Puppy{
public Puppy(String name){
// This is a constructor and has one parameter, name.
System.out.println("Passed Name:" + name );
}
public static void main(String []args){
// Create an object myPuppy.
Puppy myPuppy = new Puppy( "Baguette" );
}
}
click below button to copy the code. By - java tutorial - team
If you compile and run the above program, it will produce the following result:
Passed Name: Baguette
Accessing Instance Variables and Methods in Java
Variables and methods are available through the created objects. To access the instance variable, the full path should look like this:
/ * First create an object * /
ObjectReference = new Constructor ();
/ * Now call the variable as follows * /
ObjectReference.variableName;
/ * Now you can call the class method * /
ObjectReference.MethodName ();
click below button to copy the code. By - java tutorial - team
Sample Code
This example explains how to access the instance variables and class methods in Java:
public class Puppy {
int puppyAge;
public Puppy (String name) {
// This is a constructor and it has one parameter, name.
System.out.println ("Transmitted Name:" + name);
}
public void setAge (int age) {
puppyAge = age;
}
public int getAge () {
System.out.println ("Age of the puppy:" + puppyAge);
return puppyAge;
}
public static void main (String [] args) {
/ * Create an object. * /
Puppy myPuppy = new Puppy ("Baguette");
/ * Call the class method to set the age of the puppy. * /
myPuppy.setAge (2);
/ * Call another class method to get the age of the puppy. * /
myPuppy.getAge ();
/ * Get the instance variable of the class. * /
System.out.println ("Value of the variable:" + myPuppy.puppyAge);
}
}
click below button to copy the code. By - java tutorial - team
If you compile and run the above program, it will produce the following result:
Passed Name: Baguette
Age of puppy: 2
Value of variable: 2
The rules for declaring classes, import statements, and packages in the source file
In the last part of this section, let's look at the declaration rules for the source file. These rules in Java are important when declaring classes, import statements, and package operators in the source file.
- The source file can have only one public class.
- The source file can have several "nonpublic" classes.
- The public class name must match the name of the source file, which must have the .java extension at the end. For example: class name public class Employee {} , then the source file must be Employee.java .
- If a class is defined inside a package, then the package operator must be the first operator in the source file.
- If there are import statements, then they must be written between the package operators and the class declaration. If there are no package operators, the import statement must be the first line in the source file.
- The import and package operators will be the same for all classes that are present in the source file. In Java, it is not possible to declare various import and / or package operators to different classes in the source file.
- Classes have several levels of access and there are different types of classes: abstract classes, final classes, etc. We'll discuss this in the lesson with access modifiers .
- In addition to the above class types, Java also has some special classes called Inner class and Anonymous classes.
Java package
When developing applications, hundreds of classes and interfaces will be written, so the categorization of these classes is mandatory, and it also makes life much easier.
Import statements
- If you specify a full name that includes the package and the class name, the compiler can easily find the source code or classes. In Java import, this is the way to specify the correct place for the compiler to find a particular class.
- For example, the given line will request the compiler to load every class obtainable in the "java_installation / java / io" directory:
import java.io.*;
click below button to copy the code. By - java tutorial - team
A simple example from the above described
- For our preparation, we create two classes. The classes are the Employee and EmployeeTest.
- Initially, open the notepad and insert the following code. Recall that this Employee class is an open class or a public class. Now save the source file named Employee.java.
- The Employee class has four instance, age, designation, and salary variables. It has one explicitly defined constructor that takes a parameter.
import java.io. *;
public class Employee {
String name;
int age;
String designation;
double salary;
// This is the constructor of the Employee class.
public Employee (String name) {
this.name = name;
}
// Assign the age of the employee to the variable age.
public void empAge (int empAge) {
age = empAge;
}
/ * Assignment of the variable designation. * /
public void empDesignation (String empDesig) {
designation = empDesig;
}
/ * Assigning variable salary. * /
public void empSalary (double empSalary) {
salary = empSalary;
}
/ * Output of detailed information. * /
public void printEmployee () {
System.out.println ("Name:" + name);
System.out.println ("Age:" + age);
System.out.println ("Name:" + designation);
System.out.println ("Wages:" + salary);
}
}
click below button to copy the code. By - java tutorial - team
- As mentioned above, processing begins with the basic method. Therefore, for us to start the Employee class, there must be a main method and created objects. Create a separate class for these tasks.
- Below is the class EmployeeTest, in which two instances of the Employee class are created and call methods for each object, to assign values to each variable.
- Save the given below code in the file "EmployeeTest.java":
import java.io. *;
public class EmployeeTest {
public static void main (String args []) {
/ * Create two objects using the constructor. * /
Employee empOne = new Employee ("Oleg Olegov");
Employee empTwo = new Employee ("Ivanov Ivanov");
// Call the method for each created object.
empOne.empAge (26);
empOne.empDesignation ("Senior Software Engineer");
empOne.empSalary (1000);
empOne.printEmployee ();
empTwo.empAge (21);
empTwo.empDesignation ("Software Engineer");
empTwo.empSalary (500);
empTwo.printEmployee ();
}
}
click below button to copy the code. By - java tutorial - team
Currently, after compiling both the classes, run EmployeeTest and obtain the following result:
C:> javac Employee.java
C:> vi EmployeeTest.java
C:> javac EmployeeTest.java
C:> java EmployeeTest
First name: Oleg Olegov
Age: 26 years old
Title: Senior Software Engineer
Salary: 1000.0
First name: Ivan Ivanov
Age: 21 years old
Title: Software Engineer
Salary: 500.0