java tutorial - Java LinkedHashSet class - java programming - learn java - java basics - java for beginners
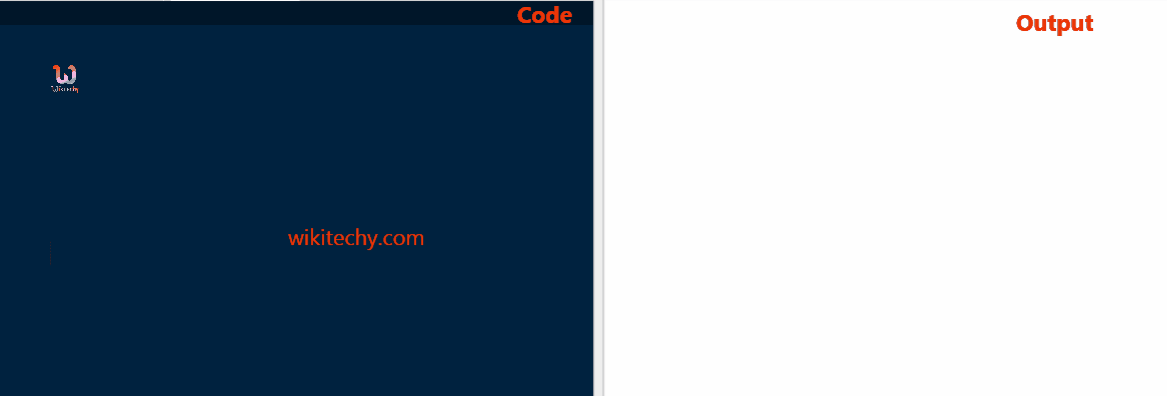
Learn Java - Java tutorial - Java linked hashset - Java examples - Java programs
Java LinkedHashSet class is a Hash table and Linked list implementation of the set interface. It inherits HashSet class and implements Set interface.
The important points about Java LinkedHashSet class are:
- Contains unique elements only like HashSet.
- Provides all optional set operations, and permits null elements.
- Maintains insertion order.
Hierarchy of LinkedHashSet class
- The LinkedHashSet class extends HashSet class which implements Set interface. The Set interface inherits Collection and Iterable interfaces in hierarchical order.
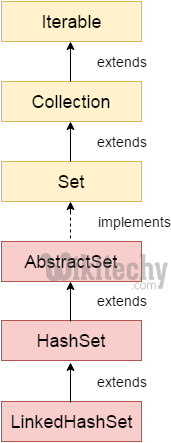
Learn java - java tutorial - linkedhashset - java examples - java programs
LinkedHashSet class declaration
- Let's see the declaration for java.util.LinkedHashSet class.
public class LinkedHashSet<E> extends HashSet<E> implements Set<E>, Cloneable, Serializable
click below button to copy the code. By - java tutorial - team
Constructors of Java LinkedHashSet class
Constructor | Description |
---|---|
HashSet() | It is used to construct a default HashSet. |
HashSet(Collection c) | It is used to initialize the hash set by using the elements of the collection c. |
LinkedHashSet(int capacity) | It is used initialize the capacity of the linkedhashset to the given integer value capacity. |
LinkedHashSet(int capacity, float fillRatio) | It is used to initialize both the capacity and the fill ratio (also called load capacity) of the hash set from its argument. |
Example of LinkedHashSet class:
import java.util.*;
public class TestCollection10{
public static void main(String args[]){
LinkedHashSet<String> al=new LinkedHashSet<String>();
al.add("Ravi");
al.add("Vijay");
al.add("Ravi");
al.add("Ajay");
Iterator<String> itr=al.iterator();
while(itr.hasNext()){
System.out.println(itr.next());
}
}
}
click below button to copy the code. By - java tutorial - team
Output
Ravi
Vijay
Ajay
Java LinkedHashSet Example: Book
import java.util.*;
public class Book {
int id;
String name,author,publisher;
int quantity;
public Book(int id, String name, String author, String publisher, int quantity) {
this.id = id;
this.name = name;
this.author = author;
this.publisher = publisher;
this.quantity = quantity;
}
}
public class LinkedHashSetExample {
public static void main(String[] args) {
LinkedHashSet<Book> hs=new LinkedHashSet<Book>();
//Creating Books
Book b1=new Book(101,"Let us C","Yashwant Kanetkar","BPB",8);
Book b2=new Book(102,"Data Communications & Networking","Forouzan","Mc Graw Hill",4);
Book b3=new Book(103,"Operating System","Galvin","Wiley",6);
//Adding Books to hash table
hs.add(b1);
hs.add(b2);
hs.add(b3);
//Traversing hash table
for(Book b:hs){
System.out.println(b.id+" "+b.name+" "+b.author+" "+b.publisher+" "+b.quantity);
}
}
}
click below button to copy the code. By - java tutorial - team
Output:
101 Let us C Yashwant Kanetkar BPB 8
102 Data Communications & Networking Forouzan Mc Graw Hill 4
103 Operating System Galvin Wiley 6