java tutorial - Java ArrayList class - java programming - learn java - java basics - java for beginners
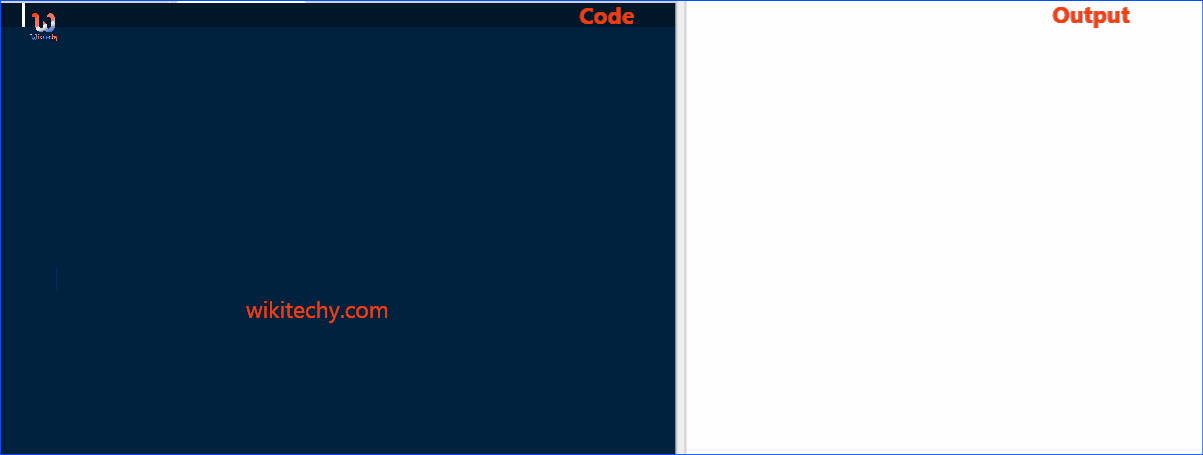
Learn Java - Java tutorial - Java arraylist - Java examples - Java programs
Java ArrayList class uses a dynamic array for storing the elements. It inherits AbstractList class and implements List interface.
The important points about Java ArrayList class are:
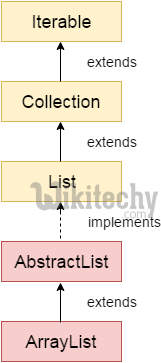
Learn java - java tutorial - arraylist - java examples - java programs
- Java ArrayList class can contain duplicate elements.
- Java ArrayList class maintains insertion order.
- Java ArrayList class is non synchronized.
- Java ArrayList allows random access because array works at the index basis.
- In Java ArrayList class, manipulation is slow because a lot of shifting needs to be occurred if any element is removed from the array list.
Hierarchy of ArrayList class
As shown in above diagram, Java ArrayList class extends AbstractList class which implements List interface. The List interface extends Collection and Iterable interfaces in hierarchical order.
ArrayList class declaration
Let's see the declaration for java.util.ArrayList class.
public class ArrayList<E> extends AbstractList<E> implements List<E>, RandomAccess, Cloneable, Serializable
click below button to copy the code. By - java tutorial - team
Constructors of Java ArrayList
Constructor | Description |
---|---|
ArrayList() | It is used to build an empty array list. |
ArrayList(Collection c) | It is used to build an array list that is initialized with the elements of the collection c. |
ArrayList(int capacity) | It is used to build an array list that has the specified initial capacity. |
Methods of Java ArrayList
Method | Description |
---|---|
void add(int index, Object element) | It is used to insert the specified element at the specified position index in a list. |
boolean addAll(Collection c) | It is used to append all of the elements in the specified collection to the end of this list, in the order that they are returned by the specified collection's iterator. |
void clear() | It is used to remove all of the elements from this list. |
int lastIndexOf(Object o) | It is used to return the index in this list of the last occurrence of the specified element, or -1 if the list does not contain this element. |
Object[] toArray() | It is used to return an array containing all of the elements in this list in the correct order. |
Object[] toArray(Object[] a) | It is used to return an array containing all of the elements in this list in the correct order. |
boolean add(Object o) | It is used to append the specified element to the end of a list. |
boolean addAll(int index, Collection c) | It is used to insert all of the elements in the specified collection into this list, starting at the specified position. |
Object clone() | It is used to return a shallow copy of an ArrayList. |
int indexOf(Object o) | It is used to return the index in this list of the first occurrence of the specified element, or -1 if the List does not contain this element. |
void trimToSize() | It is used to trim the capacity of this ArrayList instance to be the list's current size. |
Java Non-generic Vs Generic Collection
Java collection framework was non-generic before JDK 1.5. Since 1.5, it is generic. Java new generic collection allows you to have only one type of object in collection. Now it is type safe so typecasting is not required at run time. Let's see the old non-generic example of creating java collection.
ArrayList al=new ArrayList();//creating old non-generic arraylist
click below button to copy the code. By - java tutorial - team
Let's see the new generic example of creating java collection.
ArrayList<String> al=new ArrayList<String>();//creating new generic arraylist
click below button to copy the code. By - java tutorial - team
- In generic collection, we specify the type in angular braces. Now ArrayList is forced to have only specified type of objects in it. If you try to add another type of object, it gives compile time error.
Java ArrayList Example
import java.util.*;
public class TestCollection1{
public static void main(String args[]){
ArrayList<String> list=new ArrayList<String>();//Creating arraylist
list.add("Raji");//Adding object in arraylist
list.add("Vijay");
list.add("Raju");
list.add("Ajith");
//Traversing list through Iterator
Iterator itr=list.iterator();
while(itr.hasNext()){
System.out.println(itr.next());
}
}
}
click below button to copy the code. By - java tutorial - team
Output
Raji
Vijay
Raju
Ajith
Two ways to iterate the elements of collection in java
There are two ways to traverse collection elements:
- By Iterator interface.
- By for-each loop.
In the above example, we have seen traversing ArrayList by Iterator. Let's see the example to traverse ArrayList elements using for-each loop
Iterating Collection through for-each loop
import java.util.*;
public class TestCollection2{
public static void main(String args[]){
ArrayList<String> al=new ArrayList<String>();
al.add("Raji");
al.add("Vijay");
al.add("Raju");
al.add("Ajith");
for(String obj:al)
System.out.println(obj);
}
}
click below button to copy the code. By - java tutorial - team
Output
Raji
Vijay
Raju
Ajith
User-defined class objects in Java ArrayList
Let's see an example where we are storing Student class object in array list.
class Student{
int rollno;
String name;
int age;
Student(int rollno,String name,int age){
this.rollno=rollno;
this.name=name;
this.age=age;
}
}
click below button to copy the code. By - java tutorial - team
import java.util.*;
public public class TestCollection3{
public static void main(String args[]){
//Creating user-defined class objects
Student s1=new Student(101,"Sonu",23);
Student s2=new Student(102,"Raja",21);
Student s2=new Student(103,"anu",25);
//creating arraylist
ArrayList<Student> al=new ArrayList<Student>();
al.add(s1);//adding Student class object
al.add(s2);
al.add(s3);
//Getting Iterator
Iterator itr=al.iterator();
//traversing elements of ArrayList object
while(itr.hasNext()){
Student st=(Student)itr.next();
System.out.println(st.rollno+" "+st.name+" "+st.age);
}
}
}
click below button to copy the code. By - java tutorial - team
Output
101 Sonu 23
102 Raja 21
103 anu 25
Example of addAll(Collection c) method
import java.util.*;
public class TestCollection4{
public static void main(String args[]){
ArrayList<String> al=new ArrayList<String>();
al.add("Ravi");
al.add("jay");
al.add("Ajay");
ArrayList<String> al2=new ArrayList<String>();
al2.add("Sonu");
al2.add("anu");
al.addAll(al2);//adding second list in first list
Iterator itr=al.iterator();
while(itr.hasNext()){
System.out.println(itr.next());
}
}
}
click below button to copy the code. By - java tutorial - team
Output
Ravi
jay
Ajay
Sonu
anu
Example of removeAll() method
import java.util.*;
public class TestCollection5{
public static void main(String args[]){
ArrayList<String> al=new ArrayList<String>();
al.add("Ravi");
al.add("Vijay");
al.add("Ajay");
ArrayList<String> al2=new ArrayList<String>();
al2.add("Raji");
al2.add("anu");
al.removeAll(al2);
System.out.println("iterating the elements after removing the elements of al2...");
Iterator itr=al.iterator();
while(itr.hasNext()){
System.out.println(itr.next());
}
}
}
click below button to copy the code. By - java tutorial - team
Output
iterating the elements after removing the elements of al2...
Vijay
Ajay
Example of retainAll() method
import java.util.*;
public class TestCollection6{
public static void main(String args[]){
ArrayList<String> al=new ArrayList<String>();
al.add("Ravi");
al.add("Vijay");
al.add("Ajay");
ArrayList<String> al2=new ArrayList<String>();
al2.add("Raji");
al2.add("anu");
al.retainAll(al2);
System.out.println("iterating the elements after retaining the elements of al2...");
Iterator itr=al.iterator();
while(itr.hasNext()){
System.out.println(itr.next());
}
}
}
click below button to copy the code. By - java tutorial - team
Output
iterating the elements after retaining the elements of al2...
Raji
Java ArrayList Example: Book
Let's see an ArrayList example where we are adding books to list and printing all the books.
import java.util.*;
public class Book {
int id;
String name,author,publisher;
int quantity;
public Book(int id, String name, String author, String publisher, int quantity) {
this.id = id;
this.name = name;
this.author = author;
this.publisher = publisher;
this.quantity = quantity;
}
}
public class ArrayListExample {
public static void main(String[] args) {
//Creating list of Books
List<Book> list=new ArrayList<Book>();
//Creating Books
Book b1=new Book(101,"Let us C","Yashwant","BPB",8);
Book b2=new Book(102,"Data Communications ","Forouzan","Mc Graw Hill",4);
Book b3=new Book(103,"Operating System","Galvin","Wiley",6);
//Adding Books to list
list.add(b1);
list.add(b2);
list.add(b3);
//Traversing list
for(Book b:list){
System.out.println(b.id+" "+b.name+" "+b.author+" "+b.publisher+" "+b.quantity);
}
}
}
click below button to copy the code. By - java tutorial - team
Output
101 Let us C Yashwant BPB 8
102 Data Communications Forouzan Mc Graw Hill 4
103 Operating System Galvin Wiley 6