java tutorial - Java HashSet class - java programming - learn java - java basics - java for beginners
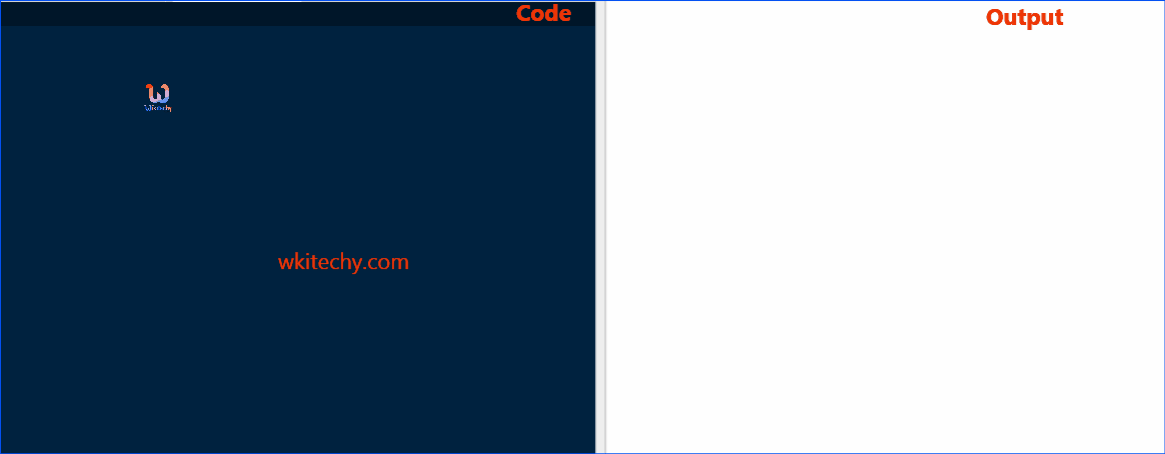
Learn Java - Java tutorial - Java hashset - Java examples - Java programs
Java HashSet class is used to create a collection that uses a hash table for storage. It inherits the AbstractSet class and implements Set interface.
The important points about Java HashSet class are:
- HashSet stores the elements by using a mechanism called hashing.
- HashSet contains unique elements only.
Difference between List and Set
- List can contain duplicate elements whereas Set contains unique elements only.
Hierarchy of HashSet class
- The HashSet class extends AbstractSet class which implements Set interface. The Set interface inherits Collection and Iterable interfaces in hierarchical order.
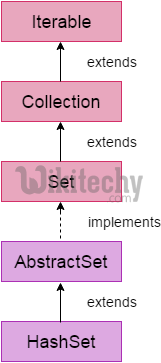
Learn java - java tutorial - hashset - java examples - java programs
HashSet class declaration
- Let's see the declaration for java.util.HashSet class.
public class HashSet<E> extends AbstractSet<E> implements Set<E>, Cloneable, Serializable
click below button to copy the code. By - java tutorial - team
Constructors of Java HashSet class:
Constructor | Description |
---|---|
HashSet() | It is used to construct a default HashSet. |
HashSet(Collection c) | It is used to initialize the hash set by using the elements of the collection c. |
HashSet(int capacity) | It is used to initialize the capacity of the hash set to the given integer value capacity. The capacity grows automatically as elements are added to the HashSet. |
Methods of Java HashSet class:
Method | Description |
---|---|
void clear() | It is used to remove all of the elements from this set. |
boolean contains(Object o) | It is used to return true if this set contains the specified element. |
boolean add(Object o) | It is used to adds the specified element to this set if it is not already present. |
boolean isEmpty() | It is used to return true if this set contains no elements. |
boolean remove(Object o) | It is used to remove the specified element from this set if it is present. |
Object clone() | It is used to return a shallow copy of this HashSet instance: the elements themselves are not cloned. |
Iterator iterator() | It is used to return an iterator over the elements in this set. |
int size() | It is used to return the number of elements in this set. |
Java HashSet Example
import java.util.*;
public class TestCollection9{
public static void main(String args[]){
//Creating HashSet and adding elements
HashSet<String> set=new HashSet<String>();
set.add("Ravi");
set.add("Vijay");
set.add("Ravi");
set.add("Ajay");
//Traversing elements
Iterator<String> itr=set.iterator();
while(itr.hasNext()){
System.out.println(itr.next());
}
}
}
click below button to copy the code. By - java tutorial - team
Output
Ajay
Vijay
Ravi
Java HashSet Example: Book
- Let's see a HashSet example where we are adding books to set and printing all the books.
import java.util.*;
public class Book {
int id;
String name,author,publisher;
int quantity;
public Book(int id, String name, String author, String publisher, int quantity) {
this.id = id;
this.name = name;
this.author = author;
this.publisher = publisher;
this.quantity = quantity;
}
}
public class HashSetExample {
public static void main(String[] args) {
HashSet<Book> set=new HashSet<Book>();
//Creating Books
Book b1=new Book(101,"Let us C","Yashwant Kanetkar","BPB",8);
Book b2=new Book(102,"Data Communications & Networking","Forouzan","Mc Graw Hill",4);
Book b3=new Book(103,"Operating System","Galvin","Wiley",6);
//Adding Books to HashSet
set.add(b1);
set.add(b2);
set.add(b3);
//Traversing HashSet
for(Book b:set){
System.out.println(b.id+" "+b.name+" "+b.author+" "+b.publisher+" "+b.quantity);
}
}
}
click below button to copy the code. By - java tutorial - team
Output:
101 Let us C Yashwant Kanetkar BPB 8
102 Data Communications & Networking Forouzan Mc Graw Hill 4
103 Operating System Galvin Wiley 6