- In Java programming, the character preceded by a backslash character() is called a control sequence and has a special meaning for the compiler.
- The newline character (\ n) is often used in our examples in System .out.println(), the operator moves to the next line after the typed string. li>
- The following table shows the escape sequences used in Java:
java tutorial - Java - Characters - java tutorial - java programming - learn java - java basics - java for beginners
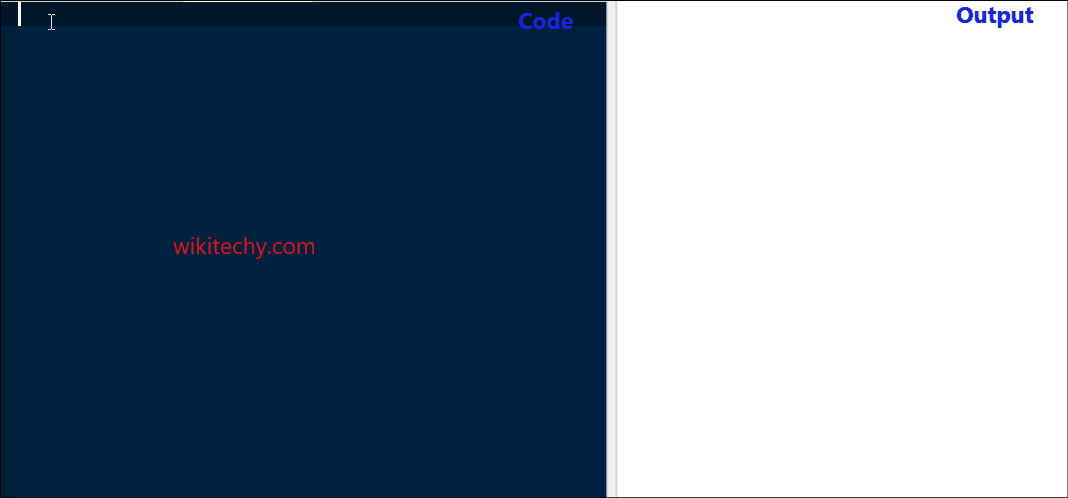
Learn Java - Java tutorial - Java characters - Java examples - Java programs
Example 1
char ch = 'a';
char uniChar = '\u039A';
// Array of characters
char[] charArray ={ 'a', 'b', 'c', 'd', 'e' };
click below button to copy the code. By - java tutorial - team
- In development, we encounter situations where we need to use objects instead of primitive data types.
- To succeed this, Java provides a wrapper class for primitive char data.
- In Java, the symbol class deals a number of useful (for example, static) methods for manipulating characters. A symbol object is created using a character constructor (Character):
Character ch = new Character('a');
click below button to copy the code. By - java tutorial - team
- The Java compiler will also create a symbol object for you in some circumstances. For example, if a primitive char data type is passed to a method that expects an object, the compiler automatically converts the char to a Character object for you.
- This function is called automatic packing or unpacking, if the conversion goes a different way.
Example 2
// Below is the following primitive char 'a'
// wrapped in Character ch object
Character ch = 'a';
// Below, the primitive 'x' is packaged for the test method,
// returns to the unpacked char 'c'
char c = test ('x');
click below button to copy the code. By - java tutorial - team
Control sequences
Control Sequence | Description |
\t | The tab character. |
\b | A character that returns a text back one step or deletes one character in a line (backspace). |
\n | The newline character. |
\r | The carriage return character. |
\f | Paging the page. |
\' | The single-quote character. |
\" | The double-quote character. |
\\ | The backslash character (\). |
When the escape sequence encounters a print statement, the java compiler interprets this appropriately.
Example of control sequences
public class Test {
public static void main (String args []) {
System.out.println ("welcome \twikitechy.com - > \\ t - tabulation");
System.out.println ("welcome \bwikitechy.com - > \\ b - go back one step");
System.out.println ("welcome \nwikitechy.com - > \\ n - new line");
System.out.println ("welcome \rwikitechy.com - > \\ r - carriage return");
System.out.println ("welcome \fwikitechy.com - > \\ f - page run");
System.out.println ("welcome \'wikitechy.com - > \\' - single quotation mark");
System.out.println ("welcome \"wikitechy.com - > \\\ "- double quote");
System.out.println ("welcome \\wikitechy.com -> \\\\ - backslash");
}
}
click below button to copy the code. By - java tutorial - team
Output
Welcome wikitechy.com - > \ t - tab
Welcome wikitechy.com - > \ b - return one step back
Welcome wikitechy.com - > \ n - new line
wikitechy.com - > \ r - carriage return
Welcome wikitechy.com - > \ f - page run
Welcome'wikitechy.com - > \ '- single quotation mark
Welcome "wikitechy.com - > \"- double quote
Welcome \ wikitechy.com - > \\ - backslash
An example of inserting a double-quote character into a string
To insert a double-quote character into a string, use the escape sequence \". If you want to take quotes a word or phrase - insert \" 2 times, one at the beginning, the other at the end:
public class Test {
public static void main (String args []) {
System.out.println ("Hi Welcome to wikitechy");
}
}
click below button to copy the code. By - java tutorial - team
Output
Hi Welcome to wikitechy.
An example of inserting a double quotation character into Java character class methods
A list of methods that implements subclasses of the character class:
NO | Methods | Description |
---|---|---|
1 | isLetter() | Determines whether the value of the specified type char is a letter. |
2 | isDigit() | Determines whether the value of the specified type char is a digit. |
3 | isWhitespace() | Determines whether the value of the specified char type is an empty space. |
4 | isUpperCase() | Determines whether the value of the specified char type is uppercase. |
5 | isLowerCase() | Determines whether the value of the specified char type is in lowercase. |
6 | toUpperCase() | Returns the uppercase value as the specified char type. |
7 | toLowerCase() | Returns a lowercase value of the specified char type. |
8 | toString() | Returns a string object denote the specified character value, string is one character. |