java tutorial - Java Operator switchcase - java programming - learn java - java basics - java for beginners
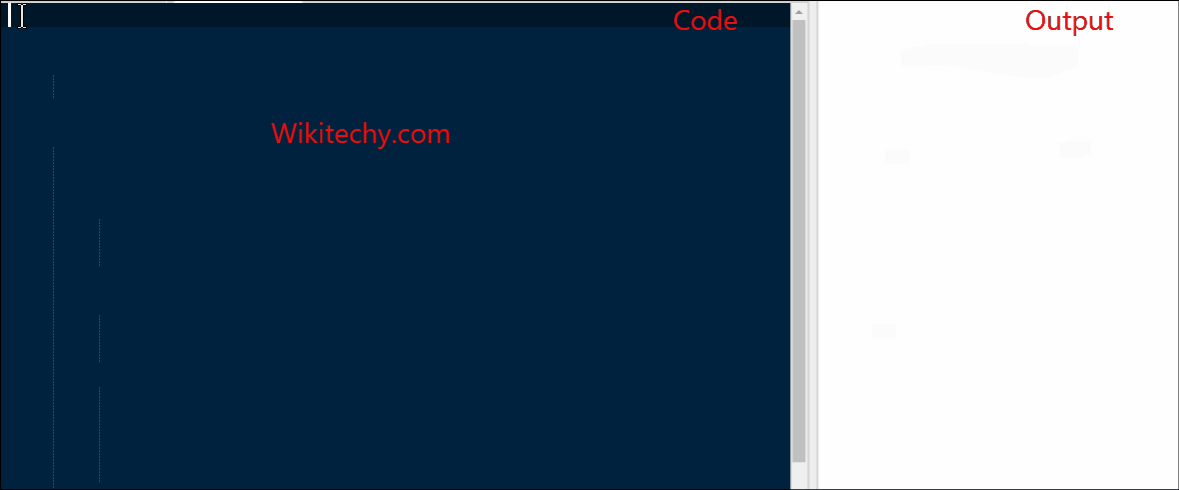
Learn Java - Java tutorial - Java operator switch case - Java examples - Java programs
Java Operator switchcase — checks the variable for equality with respect to the list of values. Each value is called case, and the variable switching is checked for each case.
Syntax
The syntax for the extended switch loop in Java is:
switch (expression) {
case value:
// Operators
break; //not necessary
case value:
// Operators
break; //not necessary
// You can have any number of case statements.
default: // optional
// Operators
}
click below button to copy the code. By - java tutorial - team
The following rules apply to the switch statement:
- Variables that are used in the switch statement can only be integers converted to integers (byte, short, char), strings and enumerations.
- You can have any number of case statements within a single switch. Each case should be followed by a value to be compared, followed by a colon.
- The case value must be the same data type as the variable in the switch, and it must be a constant or a literal.
- When the switch variable is equal to the case statement, the statements following the case will be executed until the break statement is reached.
- When the break statement is reached, then the switch statement is terminated and the control thread moves to the next line after the switch statement.
- Not every case must contain a break. If there is no break, the control thread will fall on the next case, until the break is reached.
- In Java, the switch statement has an additional default case that must be at the end of the switch.
- The default case can be used to perform a task when none of the cases is correct. Break is not required in the default case.
Process description
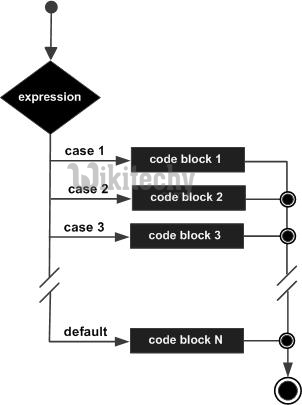
Learn java - java tutorial -switch statement - java examples - java programs
Sample Code
public class Test {
public static void main (String args []) {
// char grade = args [0] .charAt (0);
char grade = 'C';
switch (grade)
{
case 'A':
System.out.println ("Great!");
break;
case 'B':
case 'C':
System.out.println ("Completed");
break;
case 'D':
System.out.println ("You have passed");
case 'F':
System.out.println ("Better try again");
break;
default:
System.out.println ("Invalid score");
}
System.out.println ("Your rating" + grade);
}
}
click below button to copy the code. By - java tutorial - team
Output
-
Compile and run the java-program using various arguments on the command line.
The following result will be obtained:
Great
Your score A