java tutorial - Java List Interface - java programming - learn java - java basics - java for beginners
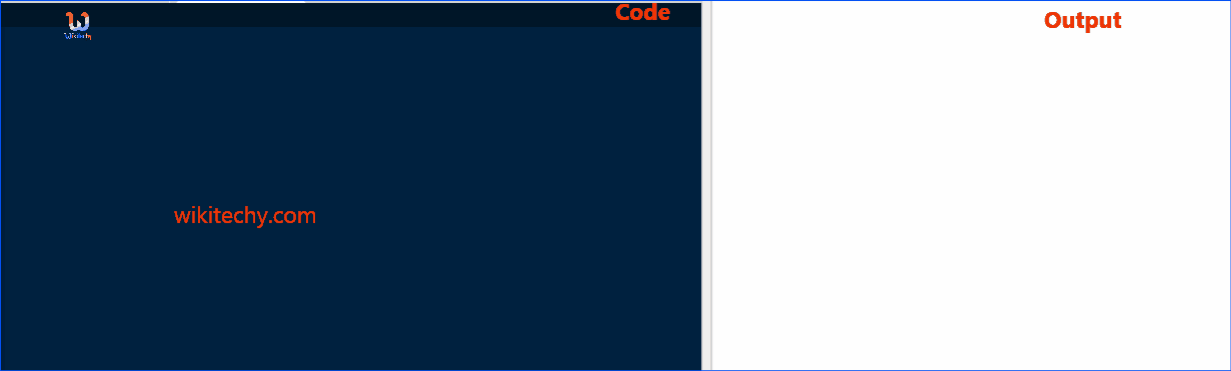
Learn Java - Java tutorial - Java list - Java examples - Java programs
List Interface is the subinterface of Collection.It contains methods to insert and delete elements in index basis.It is a factory of ListIterator interface.
List Interface declaration
public interface List<E> extends Collection<E>
click below button to copy the code. By - java tutorial - team
Methods of Java List Interface
Method | Description |
---|---|
void add(int index,Object element) | It is used to insert element into the invoking list at the index passed in the index. |
boolean addAll(int index,Collection c) | It is used to insert all elements of c into the invoking list at the index passed in the index. |
object get(int index) | It is used to return the object stored at the specified index within the invoking collection. |
object set(int index,Object element) | It is used to assign element to the location specified by index within the invoking list. |
object remove(int index) | It is used to remove the element at position index from the invoking list and return the deleted element. |
ListIterator listIterator() | It is used to return an iterator to the start of the invoking list. |
ListIterator listIterator(int index) | It is used to return an iterator to the invoking list that begins at the specified index. |
Java List Example
import java.util.*;
public class ListExample{
public static void main(String args[]){
ArrayList<String> al=new ArrayList<String>();
al.add("Amit");
al.add("Vijay");
al.add("Kumar");
al.add(1,"Sachin");
System.out.println("Element at 2nd position: "+al.get(2));
for(String s:al){
System.out.println(s);
}
}
}
click below button to copy the code. By - java tutorial - team
Output
Element at 2nd position: Vijay
Amit
Sachin
Vijay
Kumar
Java ListIterator Interface
ListIterator Interface is used to traverse the element in backward and forward direction.
ListIterator Interface declaration
public interface ListIterator<E> extends Iterator<E>
click below button to copy the code. By - java tutorial - team
Methods of Java ListIterator Interface:
Method | Description |
---|---|
boolean hasNext() | This method return true if the list iterator has more elements when traversing the list in the forward direction. |
Object next() | This method return the next element in the list and advances the cursor position. |
boolean hasPrevious() | This method return true if this list iterator has more elements when traversing the list in the reverse direction. |
Object previous() | This method return the previous element in the list and moves the cursor position backwards. |
Example of ListIterator Interface
import java.util.*;
public class TestCollection8{
public static void main(String args[]){
ArrayList<String> al=new ArrayList<String>();
al.add("Amit");
al.add("Vijay");
al.add("Kumar");
al.add(1,"Sachin");
System.out.println("element at 2nd position: "+al.get(2));
ListIterator<String> itr=al.listIterator();
System.out.println("traversing elements in forward direction...");
while(itr.hasNext()){
System.out.println(itr.next());
}
System.out.println("traversing elements in backward direction...");
while(itr.hasPrevious()){
System.out.println(itr.previous());
}
}
}
click below button to copy the code. By - java tutorial - team
Output:
element at 2nd position: Vijay
traversing elements in forward direction...
Amit
Sachin
Vijay
Kumar
traversing elements in backward direction...
Kumar
Vijay
Sachin
Amit
Example of ListIterator Interface: Book
import java.util.*;
public class Book {
int id;
String name,author,publisher;
int quantity;
public Book(int id, String name, String author, String publisher, int quantity) {
this.id = id;
this.name = name;
this.author = author;
this.publisher = publisher;
this.quantity = quantity;
}
}
public class ListExample {
public static void main(String[] args) {
//Creating list of Books
List<Book> list=new ArrayList<Book>();
//Creating Books
Book b1=new Book(101,"Let us C","Yashwant Kanetkar","BPB",8);
Book b2=new Book(102,"Data Communications & Networking","Forouzan","Mc Graw Hill",4);
Book b3=new Book(103,"Operating System","Galvin","Wiley",6);
//Adding Books to list
list.add(b1);
list.add(b2);
list.add(b3);
//Traversing list
for(Book b:list){
System.out.println(b.id+" "+b.name+" "+b.author+" "+b.publisher+" "+b.quantity);
}
}
}
click below button to copy the code. By - java tutorial - team
Output:
101 Let us C Yashwant Kanetkar BPB 8
102 Data Communications & Networking Forouzan Mc Graw Hill 4
103 Operating System Galvin Wiley 6