java tutorial - Java - ByteArrayInputStream class: constructors and methods - java programming - learn java - java basics - java for beginners
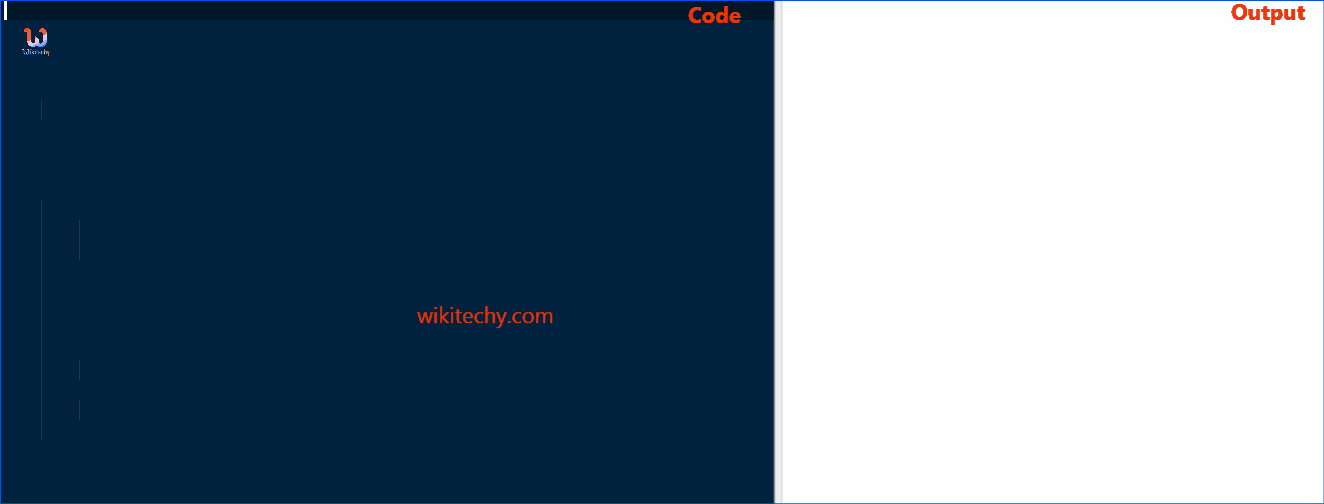
Learn Java - Java tutorial - Java array input stream - Java examples - Java programs
The ByteArrayInputStream class permits you to use the buffer in memory as an InputStream. The input source is an array of bytes.
Constructors
No. | Constructor and Description |
1 | ByteArrayInputStream (byte [] a) The constructor takes a byte array as a parameter. |
2 | ByteArrayInputStream (byte [] a, int off, int len) The constructor takes an array of bytes and two integer values, where off is used to read the first byte, and len is used to number of bytes to read. |
The ByteArrayInputStream class provides the following constructors.
Methods
If you use the ByteArrayInputStream object, then you have some handy methods that you can use to read the stream or perform other operations on the stream.
No. | Method and Description |
1 | public int read () The method reads the next byte of data from the InputStream. Returns int as a data byte. If this is the end of the file, it returns -1. |
2 | public int read (byte [] r, int off, int len) The method reads up to len the number of bytes from the off input stream to the array. Returns the total number of bytes read. If this is the end of the file, -1 will be returned. |
3 | public int available () Get the number of bytes that can be read from this file input stream. Returns int, which receives the number of bytes read. |
4 | public void mark (int read) Sets the current marked position in the stream. The parameter returns the maximum limit of bytes that can be read before the marked position becomes invalid. |
5 | public long skip (long n) Skips 'n' the number of bytes from the stream. Returns the actual number of bytes passed. |
Sample Code
- Below is a sample of ByteArrayInputStream and ByteArrayOutputStream.
import java.io.*;
public class TestByteStream {
public static void main(String args[])throws IOException {
ByteArrayOutputStream outputByte = new ByteArrayOutputStream(12);
while(outputByte.size()!= 12) {
outputByte.write("hi wikitechy".getBytes());
}
byte a [] = outputByte.toByteArray();
System.out.println("Output");
for(int i = 0 ; i < a.length; i++) {
// Display the characters
System.out.print((char)a[i] + " ");
}
System.out.println();
int b;
ByteArrayInputStream inputByte = new ByteArrayInputStream(a);
System.out.println("Converting characters to upper case:" );
for(int j = 0; j < 1; j++) {
while(( b = inputByte.read())!= -1) {
System.out.println(Character.toUpperCase((char)b));
}
inputByte.reset();
}
}
}
click below button to copy the code. By - java tutorial - team
Output
Output
h i w i k i t e c h y
Converting characters to upper case:
H
I
W
I
K
I
T
E
C
H
Y