java tutorial - How to connect database in java - java programming - learn java - java basics - java for beginners
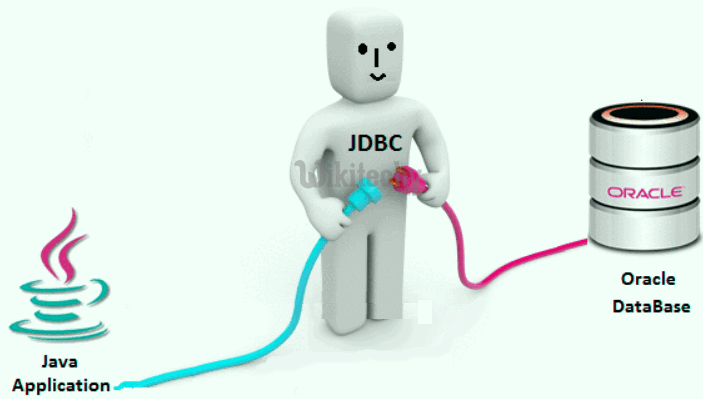
Learn Java - Java tutorial - jdbc connect with oracle - Java examples - Java programs
- To connect any java application with the database in java using JDBC.We are using 5 steps,
- Register the driver class
- Creating connection
- Creating statement
- Executing queries
- Closing connection
Register the driver class
- The forName() method of Class is used to register the driver class. This method is used to dynamically load the driver class.
Syntax
public static void forName(String className)throws ClassNotFoundException
click below button to copy the code. By - java tutorial - team
Create the connection object
- The getConnection() method of DriverManager class is used to establish connection with the database.
Syntax
public static Connection getConnection(String url)throws SQLException
public static Connection getConnection(String url,String name,String password)
throws SQLException
click below button to copy the code. By - java tutorial - team
Create the Statement object
- The createStatement() method of Connection interface is used to create statement.
- The object of statement is responsible to execute queries with the database.
Syntax
public Statement createStatement()throws SQLException
click below button to copy the code. By - java tutorial - team
Execute the query
- The executeQuery() method of Statement interface is used to execute queries to the database.
- Method returns the object of ResultSet that can be used to get all the records of a table.
Syntax
public ResultSet executeQuery(String sql)throws SQLException
click below button to copy the code. By - java tutorial - team
Close the connection object
- By closing connection object statement and ResultSet will be closed automatically.
- The close() method of Connection interface is used to close the connection.
Syntax
public void close()throws SQLException
click below button to copy the code. By - java tutorial - team
Sample Code
- In this sample we are using Oracle 10g as the database. So we need to know following information for the oracle database.
Properties of oracle thin driver.
Properties | Description |
---|---|
Driver Class: | Oracle.Jdbc.OracleDriver |
Connection url: | jdbc:oracle:thin:@localhost:1521:xe |
Username: | The default username for the oracle database is system. |
password | Password is given by the user at the time of installing the oracle database. |
jdbc:oracle:thin:@localhost:1521:x
- jdbc is the API(Application Program Interface)
- oracle is the database
- thin is the driver
- localhost is the server name on which oracle is running, we may also use IP address
- 1521 is the port number and
- XE is the Oracle service name.
Create a table in oracle database
create table student(roll number(10),name varchar2(40));
click below button to copy the code. By - java tutorial - team
Sample Code
import java.sql.*;
public class OracleCon
{
public static void main(String args[]){
try
{
//step1 load the driver class
Class.forName("oracle.jdbc.driver.OracleDriver");
//step2 create the connection object
Connection con=DriverManager.getConnection(
"jdbc:oracle:thin:@localhost:1521:xe","system","oracle");
//step3 create the statement object
Statement stmt=con.createStatement();
//step4 execute query
ResultSet rs=stmt.executeQuery("select * from student");
while(rs.next())
System.out.println(rs.getInt(1)+" "+rs.getString(2)+" "+rs.getString(3));
//step5 close the connection object
con.close();
}
catch(Exception e)
{
System.out.println(e);
}
}
}