java tutorial - How to implement Insertion Sort Algorithm in java - java programming - learn java - java basics - java for beginners
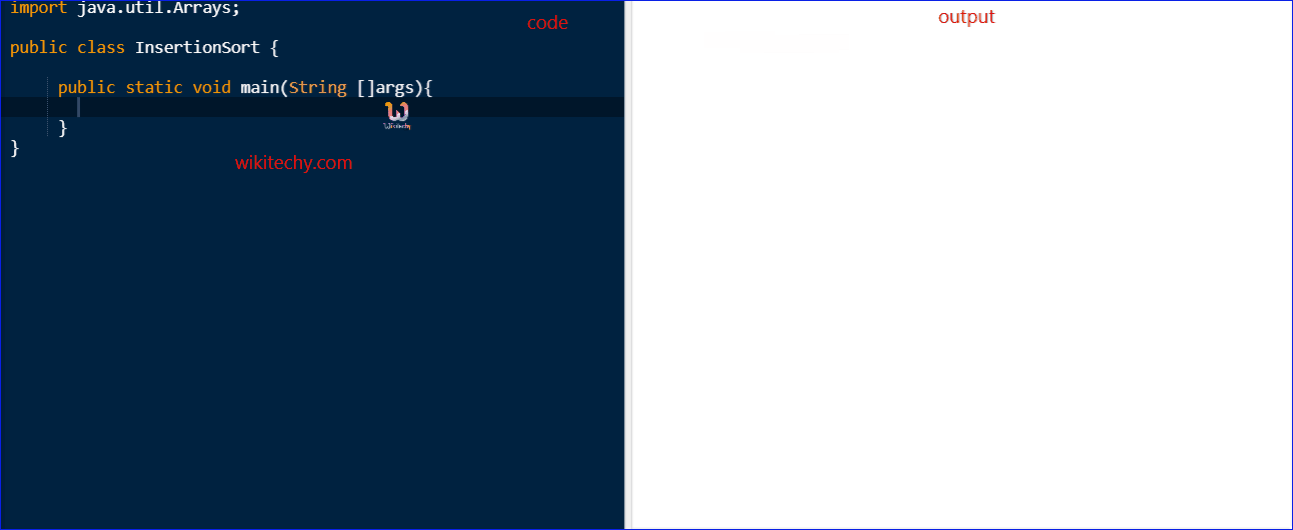
Learn Java - Java tutorial - insertion sort in Java - Java examples - Java programs
Insertion Sort Algorithm
- Insertion sort is used once the total elements is small.
- It is valuable when input array is almost sorted, only few elements are misplaced in whole big array.
- Insertion is worthy for small elements only for the purpose that it needs long time for sorting huge amount of elements.
- Insertion sort is an easy sorting algorithm that builds the final sorted array one item at a time.
- It is much less efficient on huge lists than more advanced algorithms such as quicksort, heapsort, or merge sort.
- Each replication of insertion sort eliminates an element of the input data, inserted to the exact place in the already-sorted list, up to no input elements remain.
sample code:
import java.util.Arrays;
public class InsertionSort {
public static void main (String[] args){
int[] list = {6,5,1,9,3,7,2,4};
System.out.println("Before Bubble sort\n");
System.out.println(Arrays.toString(list));
list = insertionSort(list);
System.out.println("\nAfter Bubble sort");
System.out.println(Arrays.toString(list));
}
public static int[] insertionSort(int[] list){
if(list.length <2){
return list;
}
for(int i =1; i<list.length;i++){
int j = i-1;
int current = list[i];
while(j>-1 && list[j]>current){
list[j+1] = list [j];
j--;
}
list[j+1] = current;
}
return list;
}
}
Output:
Before Bubble sort
[6, 5, 1, 9, 3, 7, 2, 4]
After Bubble sort
[1, 2, 3, 4, 5, 6, 7, 9]