java tutorial - How to Check file permission and Set file permission - java programming - learn java - java basics - java for beginners
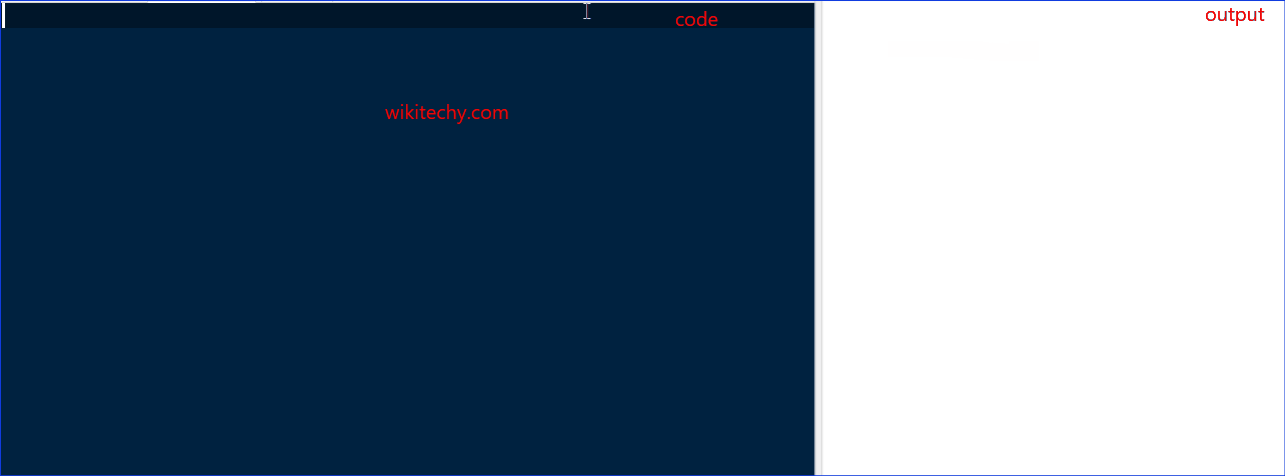
Learn java - java tutorial - check file permission and set file permission - java examples - java programs
Check file permission :
- canExecute() :
true
if and only if the abstract pathname occurs and the application is allowed to the file execute. - canWrite() :
true
if and only if the file system contains a file denoted by this abstract pathname and the application is permitted to write the file otherwise it hasfalse
to write the file. - canRead() :
true
if and only if the file identified by this abstract pathname exists and can be read by the application otherwise it hasfalse.
Set file permission :
- setExecutable() : If
true
sets the right to use permit to the executable operations; elsefalse
to stop the execute operations - setWritable() : If
true
sets the right to use permit to the writable operations; elsefalse
to stop the write operations - setReadable() : If
true
sets the right to use permit to the readable operations; elsefalse
to stop the read operations
sample code:
import java.io.File;
public class Kaashiv_infotech
{
public static void main(String[] args)
{
File myFile = new File("C:" + File.separator + "jdk" + File.separator, "kaashiv_file.java");
System.out.println("is File : " +myFile.isFile());
System.out.println("is Hidden : " +myFile.isHidden());
if(myFile.exists()) {
System.out.println("Can File Execute : " + myFile.canExecute());
System.out.println("Can File Write : " + myFile.canWrite());
System.out.println("Can File Read : " + myFile.canRead());
}
myFile.setExecutable(false);
myFile.setReadable(false);
myFile.setWritable(false);
System.out.println("Can File Execute : " + myFile.canExecute());
System.out.println("Can File Write : " + myFile.canWrite());
System.out.println("Can File Read : " + myFile.canRead());
}
}
output:
is File : false
is Hidden : false
Can File Execute : false
Can File Write : false
Can File Read : false