java tutorial - Variables and Data Types in java - java programming - learn java - java basics - java for beginners
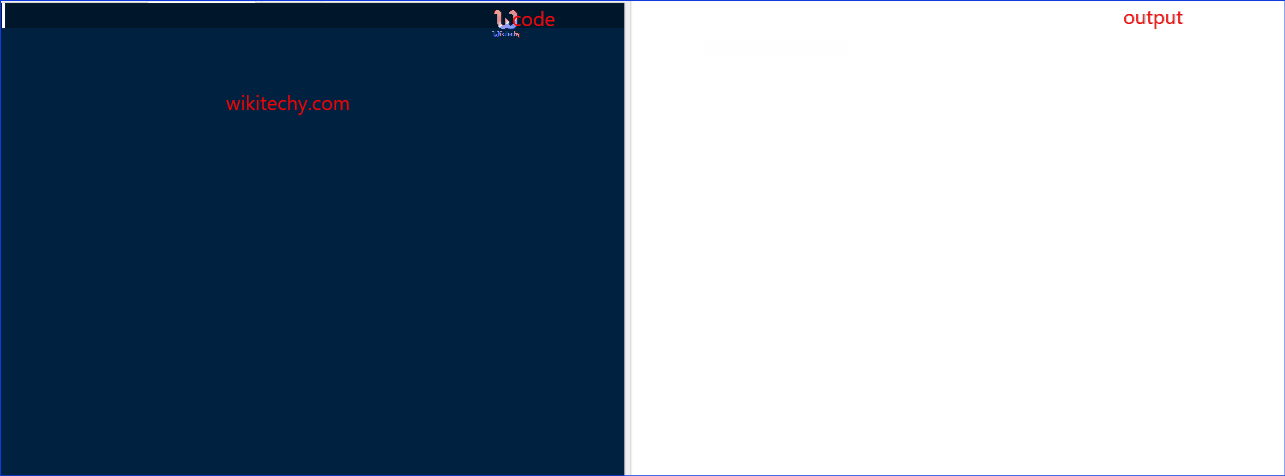
Learn java - java tutorial - Variables and Types in Java - java examples - java programs
Variables and Types in Java:
- The variable is defined as a name of memory location.
Types of Variable
- There are three types of variables in java:
- local variable
- instance variable
- static variable
Local Variable:
- The variable which is declared inside the method is known as local variable.
Instance Variable
- The variable which is declared inside the class but outside the method, is known as instance variable. It is not declared as static.
Static variable
- The variable which is declared as static is known as static variable. It cannot be local.
Data Types in Java
- Data types represent the different values to be stored in the variable. In java, there are two types of data types:
- Primitive data types
- Non-primitive data types

Learn java - java tutorial - variables and data types in Java - java examples - java programs
Data Type | Default Value | Default size |
---|---|---|
boolean | false | 1 bit |
byte | 0 | 1 bit |
char | '\u0000' | 2 byte |
short | 0 | 2 byte |
int | 0 | 4 byte |
long | 0L | 8 byte |
float | 0.0f | 4 byte |
int | 0 | 4 byte |
double | 0.0d | 8 byte |
Sample Code:
public class kaashiv_infotech
{
public static void main(String[] args)
{
int num = 30;
float dec = (float) 4.2;
double big = 217.07;
char let = 'z';
boolean value = true;
System.out.println(num);
System.out.println(dec);
System.out.println(big);
System.out.println(let);
System.out.println(value);
}
}
Output:
$javac kaashiv_infotech.java
$java kaashiv_infotech
30
4.2
217.07
z
true