java tutorial - Reverse a string in java - java programming - learn java - java basics - java for beginners
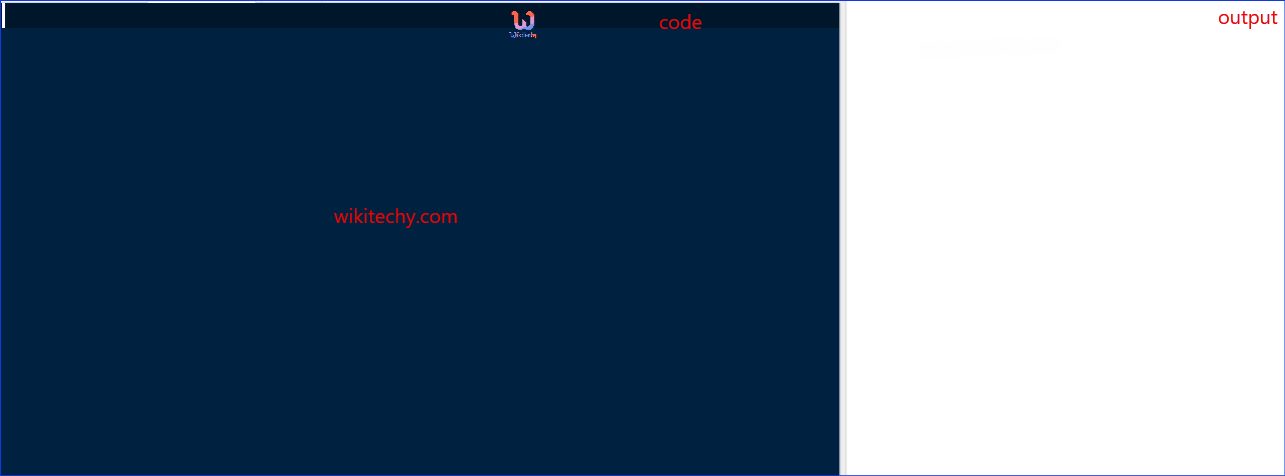
Learn java - java tutorial - Reverse a string in java - java examples - java programs
Reverse String Array :
- There are fundamentally two ways in Reverse String Array, first is to use temporary array and physically loop over the elements of an Array then swap them or second way is to use Arrays as well as Collections classes.
Syntax :
public static void Reverse(
Array array
)
Three Ways to Reverse a String in JavaScript:
- Reverse a String With Built-In Functions
- Reverse a String With a Decrementing for Loop
- Reverse a String With Recursion
The given below are several interesting facts about String and also StringBuffer classes :
- Objects of String are immutable.
- String class in Java does not have reverse() method, however StringBuilder class has built in reverse() method.
- StringBuilder class don’t have toCharArray() method, whereas String class does have toCharArray() method.
sample code:
import java.util.Collections;
import java.util.List;
import java.util.Arrays;
public class kaashiv_infotech
{
public static void main(String args[])
{
String[] strvalues = new String[]{"mani", "Tiger", "kishore", "Jana"};
List<String> arrlist = Arrays.asList(strvalues);
Collections.reverse(arrlist);
strvalues = (String[]) arrlist.toArray();
System.out.println("reversed String array ");
for(int i=0; i < strvalues.length; i++)
{
System.out.println(strvalues[i]);
}
}
}
Output:
reversed String array
Jana
kishore
Tiger
mani