java tutorial - Java Math Operators - java programming - learn java - java basics - java for beginners
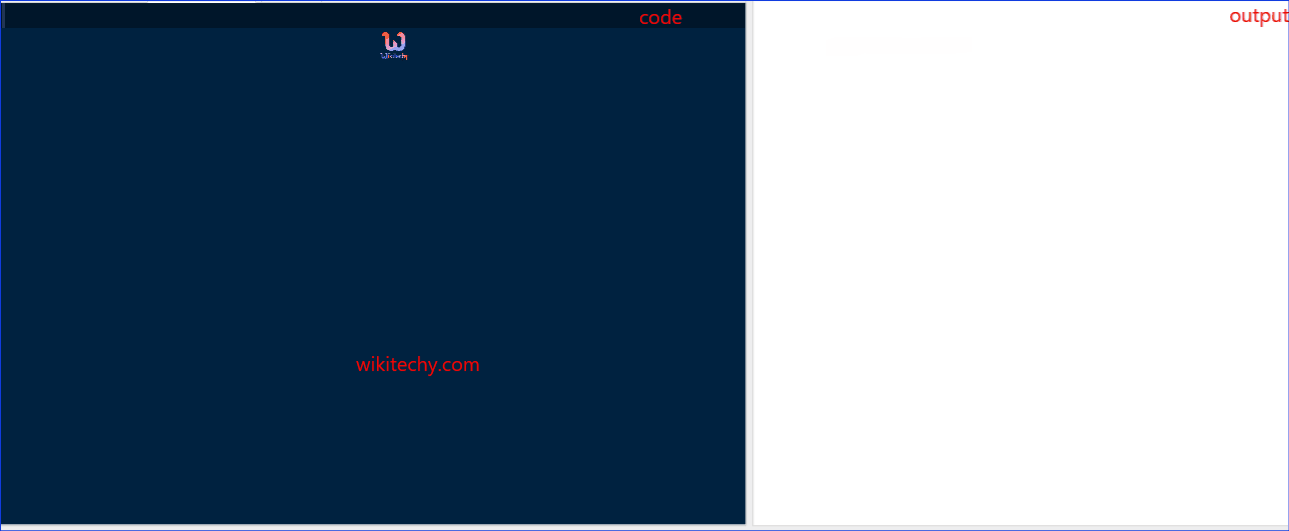
Learn java - java tutorial - Java Math Operators - java examples - java programs
Assignment Operator:
- Assignment operators are used in Java to assign values to variables.
- The assignment operator assigns the value on its right to the variable on its left. Here, 5 is assigned to the variable age using = operator.
Arithmetic Operators :
- Arithmetic operators are used to perform mathematical operations like addition, subtraction, multiplication etc
Operator | Meaning |
---|---|
+ | Addition (also used for string concatenation) |
- | Subtraction Operator |
* | Multiplication Operator |
/ | Division Operator |
% | Remainder Operator |
Unary Operators :
- An equality and a relational operator determines the relationship among two operands. It checks if an operand is greater than, less than, equal to, not equal to and so on. Depending on the relationship, it results to either
true
orfalse.
Logical Operators :
- The logical operators conditional-OR (||) and conditional-AND (&&) operates on boolean expressions.
Ternary Operator :
- The conditional operator or ternary operator (?:) is shorthand for if-then-else statement. The syntax of conditional operator is shorthand for
if-then-else
statement. The syntax of conditional operator is
Syntax:
variable = Expression ? expression1 : expression2
Bitwise and Bit Shift Operators
- To perform bitwise and bit shift operators in Java, these operators are used.
Operator | Description |
---|---|
~ | Bitwise Complement |
<< | Left Shift |
>> | Right Shift |
>>> | Unsigned Right Shift |
& | Bitwise AND |
^ | Bitwise exclusive OR |
| | Bitwise inclusive OR |
- These operators are not commonly used. Visit this page to learn more about bitwise and bit shift operators.
Sample code:
public class kaashiv_infotech
{
public static void main(String[] args)
{
int a = 32;
int b = 27;
double c = 45.375;
double d = 3.62;
System.out.println("Variable values...");
System.out.println(" a = " + a);
System.out.println(" b = " + b);
System.out.println(" c = " + c);
System.out.println(" d = " + d);
System.out.println("Adding...");
System.out.println(" a + b = " + (a + b));
System.out.println(" c + d = " + (c + d));
System.out.println("Subtracting...");
System.out.println(" a - b = " + (a - b));
System.out.println(" c - d = " + (c - d));
System.out.println("Multiplying...");
System.out.println(" a * b = " + (a * b));
System.out.println(" c * d = " + (c * d));
System.out.println("Dividing...");
System.out.println(" a / b = " + (a / b));
System.out.println(" c / d = " + (c / d));
System.out.println("Computing the remainder...");
System.out.println(" a % b = " + (a % b));
System.out.println(" c % d = " + (c % d));
System.out.println("Mixing types...");
System.out.println(" a + d = " + (b + d));
System.out.println(" b * c = " + (a * c));
}
}
Output:
$javac kaashiv_infotech.java
$java kaashiv_infotech
Variable values...
a = 32
b = 27
c = 45.375
d = 3.62
Adding...
a + b = 59
c + d = 48.995
Subtracting...
a - b = 5
c - d = 41.755
Multiplying...
a * b = 864
c * d = 164.2575
Dividing...
a / b = 1
c / d = 12.53453038674033
Computing the remainder...
a % b = 5
c % d = 1.9349999999999987
Mixing types...
a + d = 30.62
b * c = 1452.0