Android tutorial - Screen Navigation in Android - android app development - android studio - android development tutorial
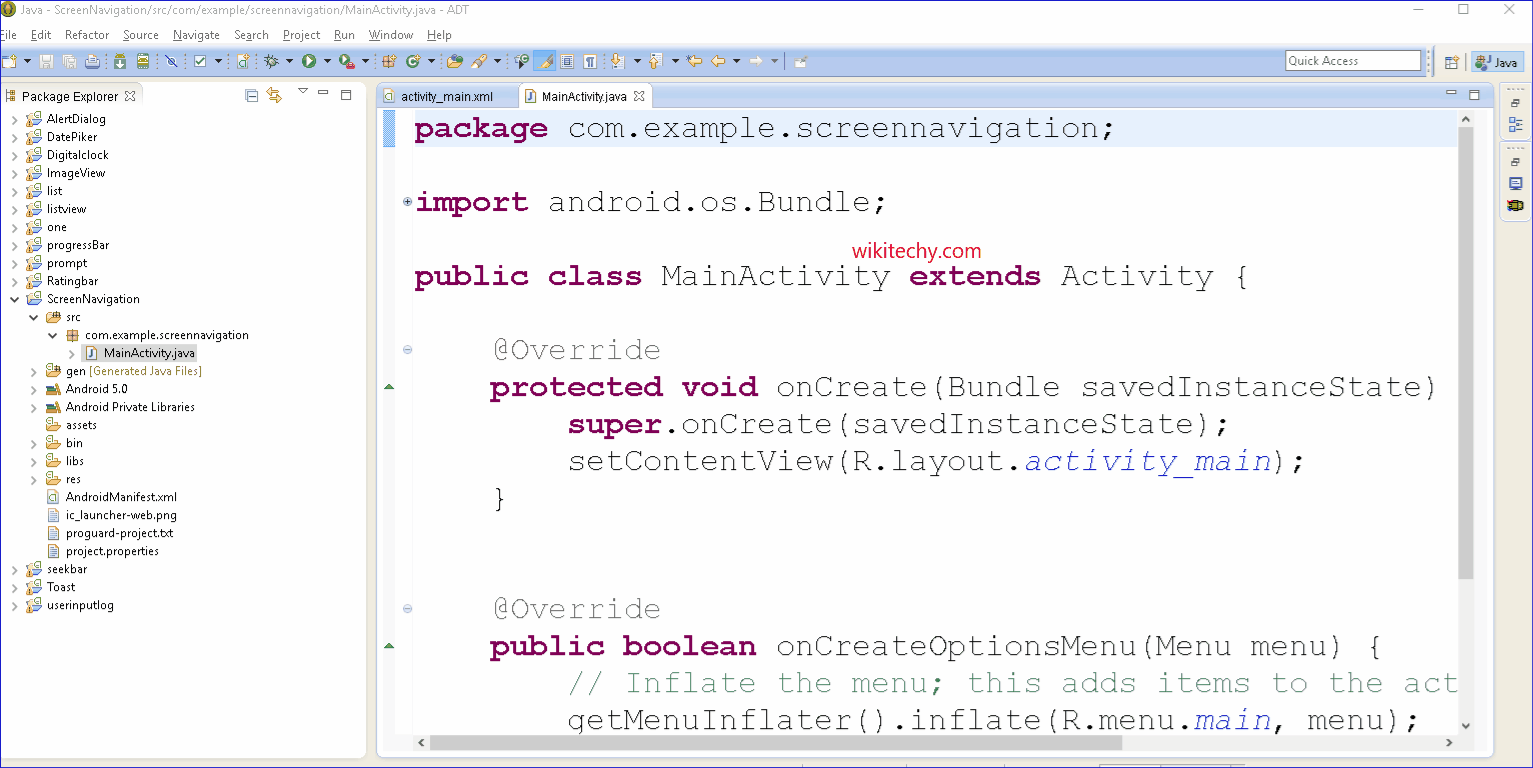
Learn android - android tutorial - Screen navigation in android - android examples - android programs
Android activity - Navigation from one screen to another screen
- In Android, an activity is representing a single screen.
- Most applications have multiple activities to represent different screens, for example, one activity to display a list of the application settings, another activity to display the application status.
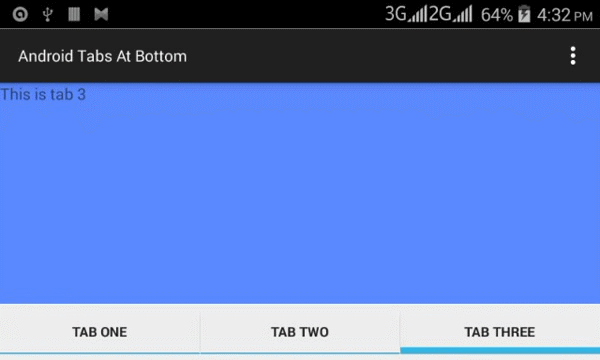
Functionality
- How to interact with activity, when a button is clicked, navigate from current screen (current activity) to another screen (another activity).
1. XML Layouts
Create following two XML layout files in “res/layout/” folder:
- res/layout/main.xml - Represent screen 1
- res/layout/main2.xml - Represent screen 2
Code for the File : res/layout/main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="I'm screen 1 (main.xml)"
android:textAppearance="?android:attr/textAppearanceLarge" />
<Button
android:id="@+id/button1"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Click me to another screen" />
</LinearLayout>
click below button to copy the code from android tutorial team
Code for the File : res/layout/main2.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="I'm screen 2 (main2.xml)"
android:textAppearance="?android:attr/textAppearanceLarge" />
</LinearLayout>
click below button to copy the code from android tutorial team
2. Activities
Create two activity classes :
- AppActivity.java -> main.xml
- App2Activity.java -> main2.xml
To navigate from one screen to another screen, use following code :
Intent intent = new Intent(context, anotherActivity.class);
startActivity(intent);
click below button to copy the code from android tutorial team
File : AppActivity.java
package com.wikitechy.android;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.os.Bundle;
import android.widget.Button;
import android.view.View;
import android.view.View.OnClickListener;
public class AppActivity extends Activity {
Button button;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
addListenerOnButton();
}
public void addListenerOnButton() {
final Context context = this;
button = (Button) findViewById(R.id.button1);
button.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
Intent intent = new Intent(context, App2Activity.class);
startActivity(intent);
}
});
}
}
click below button to copy the code from android tutorial team
File : App2Activity.java
package com.wikitechy.android;
import android.app.Activity;
import android.os.Bundle;
import android.widget.Button;
public class App2Activity extends Activity {
Button button;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main2);
}
}
click below button to copy the code from android tutorial team
3. AndroidManifest.xml
- Declares above two activity classes in AndroidManifest.xml.
File : AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.wikitechy.android"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk android:minSdkVersion="10" />
<application
android:icon="@drawable/ic_launcher"
android:label="@string/app_name" >
<activity
android:label="@string/app_name"
android:name=".AppActivity" >
<intent-filter >
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity
android:label="@string/app_name"
android:name=".App2Activity" >
</activity>
</application>
</manifest>
click below button to copy the code from android tutorial team
4. Demo - android emulator - android tutorial
- Run application.
- AppActivity.java (main.xml) screen is display.
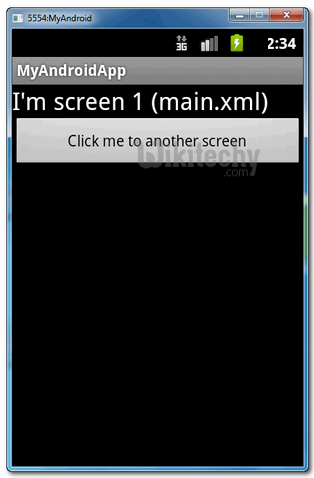
- When abovebutton is clicked, it will navigate to another screen App2Activity.java (main2.xml).
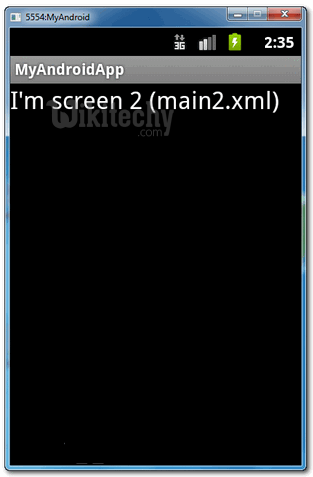