Android tutorial - Android Media Player - android app development - android studio - android development tutorial
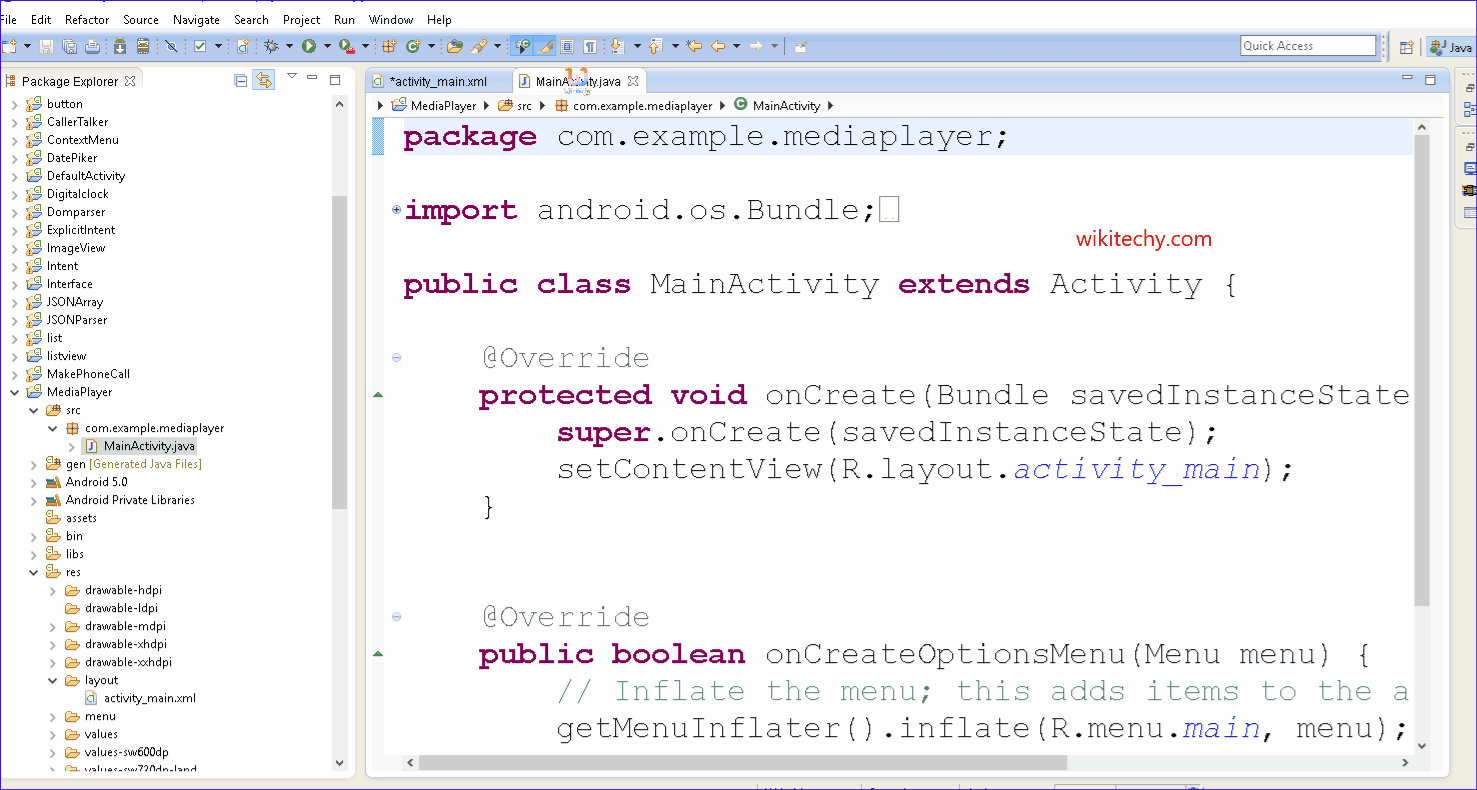
Learn android - android tutorial - Android media player - android examples - android programs
Android Media Player:
- Android provides many ways to control playback of audio/video files and streams. One of this way is through a class called MediaPlayer.
- Android is providing MediaPlayer class to access built-in mediaplayer services like playing audio,video etc.
- In order to use MediaPlayer, we have to call a static Method create () of this class. This method returns an instance of MediaPlayer class. Its syntax is as follows −
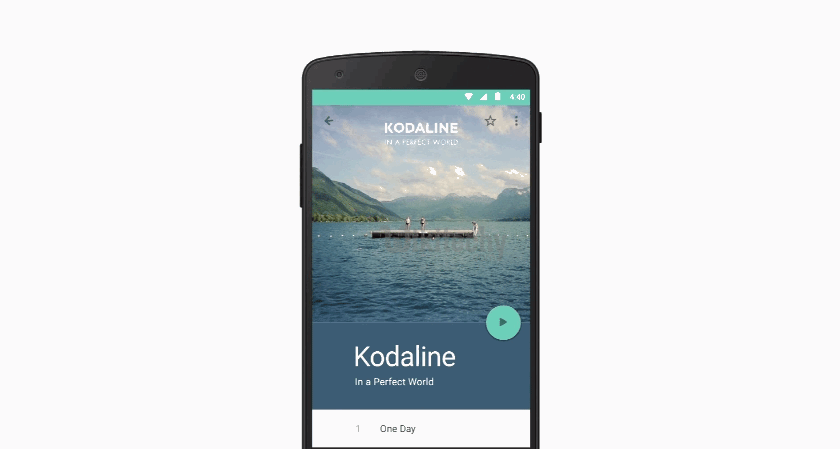
MediaPlayer mediaPlayer = MediaPlayer.create(this, R.raw.song);
click below button to copy the code from android tutorial team
- The second parameter is the name of the song that you want to play. You have to make a new folder under your project with name raw and place the music file into it.
- Once you have created the Mediaplayer object you can call some methods to start or stop the music. These methods are listed below.
mediaPlayer.start();
mediaPlayer.pause();
click below button to copy the code from android tutorial team
- On call to start() method, the music will start playing from the beginning. If this method is called again after the pause() method, the music would start playing from where it is left and not from the beginning.
- In order to start music from the beginning, you have to call reset() method. Its syntax is given below.
mediaPlayer.reset();
click below button to copy the code from android tutorial team
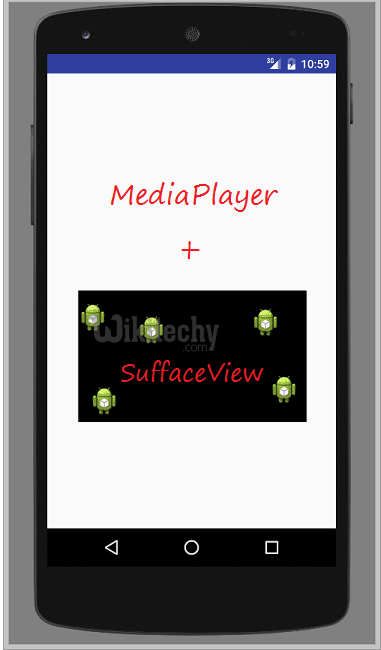
MediaPlayer class:
- The android.media.MediaPlayer class is used to control the audio or video files.
Methods of MediaPlayer class
- There are many methods of MediaPlayer class. Some of them are as follows:
Method | Description |
---|---|
public void setDataSource(String path) | sets the data source (file path or http url) to use. |
public void prepare() | prepares the player for playback synchronously. |
public void start() | it starts or resumes the playback. |
public void stop() | it stops the playback. |
public void pause() | it pauses the playback. |
public boolean isPlaying() | checks if media player is playing. |
public void seekTo(int millis) | seeks to specified time in miliseconds. |
public void setLooping(boolean looping) | sets the player for looping or non-looping. |
public boolean isLooping() | checks if the player is looping or non-looping. |
public void selectTrack(int index) | it selects a track for the specified index. |
public int getCurrentPosition() | returns the current playback position. |
public int getDuration() | returns duration of the file. |
public void setVolume(float leftVolume,float rightVolume) | sets the volume on this player. |
Activity class
- Let's write the code of to play the audio file. Here, we are going to play maine.mp3 file located inside the sdcard/Music directory.
File: MainActivity.java
package com.example.audiomediaplayer1;
import android.media.MediaPlayer;
import android.net.Uri;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.widget.MediaController;
import android.widget.VideoView;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
MediaPlayer mp=new MediaPlayer();
try{
mp.setDataSource("/sdcard/Music/maine.mp3");//Write your location here
mp.prepare();
mp.start();
}catch(Exception e){e.printStackTrace();}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
click below button to copy the code from android tutorial team
Android MediaPlayer Example of controlling the audio
- Let's see a simple example to start, stop and pause the audio play.
activity_main.xml
- Drag three buttons from pallete to start, stop and pause the audio play. Now the xml file will look like this:
File: MainActivity.java
<RelativeLayout xmlns:androclass="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_marginTop="30dp"
android:text="Audio Controller" />
<Button
android:id="@+id/button1"
style="?android:attr/buttonStyleSmall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/textView1"
android:layout_below="@+id/textView1"
android:layout_marginTop="48dp"
android:text="start" />
<Button
android:id="@+id/button2"
style="?android:attr/buttonStyleSmall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignTop="@+id/button1"
android:layout_toRightOf="@+id/button1"
android:text="pause" />
<Button
android:id="@+id/button3"
style="?android:attr/buttonStyleSmall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignTop="@+id/button2"
android:layout_toRightOf="@+id/button2"
android:text="stop" />
</RelativeLayout>
click below button to copy the code from android tutorial team
Activity class
- Let's write the code to start, pause and stop the audio player.
File: MainActivity.java
package com.example.audioplay;
import android.media.MediaPlayer;
import android.os.Bundle;
import android.os.Environment;
import android.app.Activity;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
public class MainActivity extends Activity {
Button start,pause,stop;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
start=(Button)findViewById(R.id.button1);
pause=(Button)findViewById(R.id.button2);
stop=(Button)findViewById(R.id.button3);
//creating media player
final MediaPlayer mp=new MediaPlayer();
try{
//you can change the path, here path is external directory(e.g. sdcard) /Music/maine.mp3
mp.setDataSource(Environment.getExternalStorageDirectory().getPath()+"/Music/maine.mp3");
mp.prepare();
}catch(Exception e){e.printStackTrace();}
start.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
mp.start();
}
});
pause.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
mp.pause();
}
});
stop.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
mp.stop();
}
});
}
}
click below button to copy the code from android tutorial team
Output:
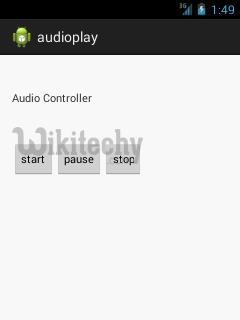