Android tutorial - Android Start Activity For Result Example - android app development - android studio - android development tutorial
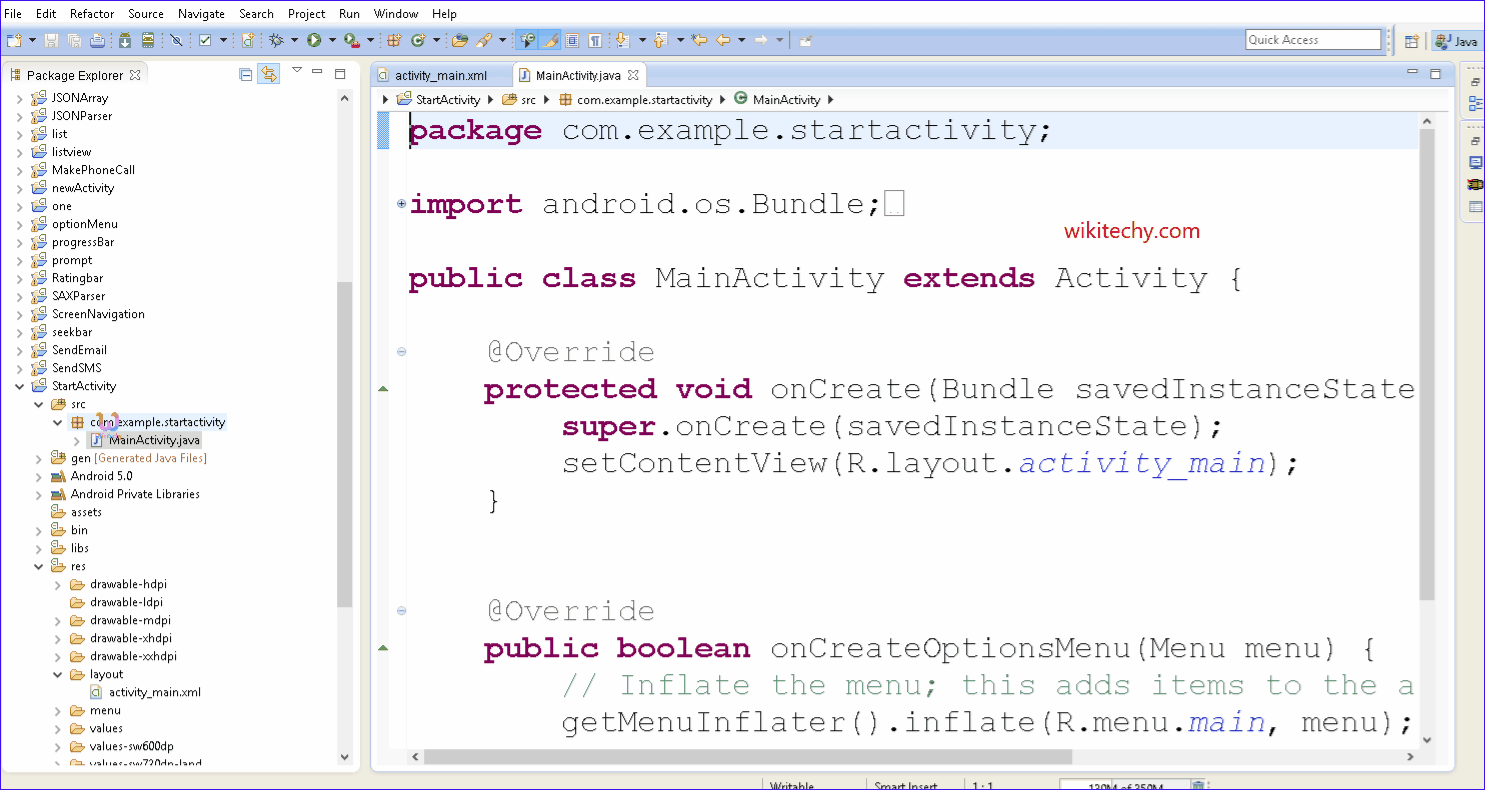
Learn android - android tutorial - Android start activity - android examples - android programs
What is Start Activity For Result
- Start Activity For Result is used to receive result back from android activity like if your starting camera application into your mobile phone then as the final result you want to display your captured photo on mobile phone screen.
- In Android, the start Activity(Intent) method is used to start a new activity, which will be placed at the top of the activity stack.
- It takes a single argument, an Intent, which describes the activity to be executed.
- However, sometimes we might want to get a result back from an activity when it ends.
- For example, you may start an activity that lets the user pick a person in a list of contacts; when it ends, it returns the person that was selected.
- To do this, you call the start Activity For Result(Intent, 'extraValue') version with a second parameter identifying the call.
- The result will come back through your Extra values, from getIntent().
- By the help of android startActivityForResult() method, we can get result from another activity.
- By the help of android startActivityForResult() method, we can send information from one activity to another and vice-versa. The android startActivityForResult method, requires a result from the second activity (activity to be invoked).
- In such case, we need to override the onActivityResult method that is invoked automatically when second activity returns result.
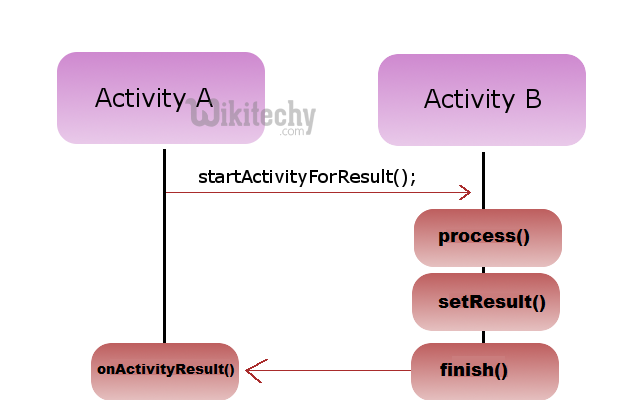
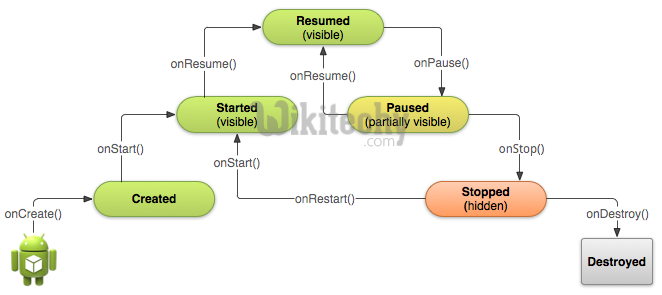
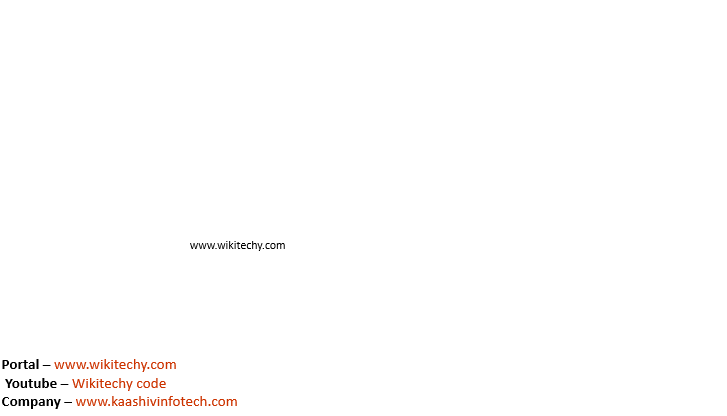
Method Signature
There are two variants of startActivityForResult() method.
- public void startActivityForResult (Intent intent, int requestCode)
- public void startActivityForResult (Intent intent, int requestCode, Bundle options)
Let's see the simple example of android startActivityForResult method.
activity_main.xml
- Drag one textview and one button from the pallete, now the xml file will look like this.
File: activity_main.xml
<RelativeLayout xmlns:androclass="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/button1"
android:layout_alignParentTop="true"
android:layout_marginTop="48dp"
android:text="Default Message" />
<Button
android:id="@+id/button1"
android:layout_width="wrap_content" android:layout_height="wrap_content"
android:layout_below="@+id/textView1"
android:layout_centerHorizontal="true"
android:layout_marginTop="42dp"
android:text="GetMessage" />
</RelativeLayout>
click below button to copy the code from android tutorial team
second_main.xml
- This xml file is created automatically when you create another activity. To create new activity Right click on the package inside the src -> New -> Other ->Android Activity.
- Now drag one editText, one textView and one button from the pallete, now the xml file will look like this:
File: second_main.xml
<RelativeLayout xmlns:androclass="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".SecondActivity" >
<EditText
android:id="@+id/editText1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_marginTop="61dp"
android:layout_toRightOf="@+id/textView1"
android:ems="10" />
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/editText1"
android:layout_alignBottom="@+id/editText1"
android:layout_alignParentLeft="true"
android:text="Enter Message:" />
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/editText1"
android:layout_centerHorizontal="true"
android:layout_marginTop="34dp"
android:text="Submit" />
</RelativeLayout>
click below button to copy the code from android tutorial team
Activity class
- Now let's write the code that invokes another activity and get result from that activity.
File: MainActivity.java
package com.wikitechy.startactivityforresult;
import android.os.Bundle;
import android.app.Activity;
import android.content.Intent;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends Activity {
TextView textView1;
Button button1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textView1=(TextView)findViewById(R.id.textView1);
button1=(Button)findViewById(R.id.button1);
button1.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
Intent intent=new Intent(MainActivity.this,SecondActivity.class);
startActivityForResult(intent, 2);// Activity is started with requestCode 2
}
});
}
// Call Back method to get the Message form other Activity
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data)
{
super.onActivityResult(requestCode, resultCode, data);
// check if the request code is same as what is passed here it is 2
if(requestCode==2)
{
String message=data.getStringExtra("MESSAGE");
textView1.setText(message);
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
click below button to copy the code from android tutorial team
Second Activity class
- Let's write the code that displays the content of second activity layout file.
File: SecondActivity.java
package com.wikitechy.startactivityforresult;
import android.os.Bundle;
import android.app.Activity;
import android.content.Intent;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
public class SecondActivity extends Activity {
EditText editText1;
Button button1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
editText1=(EditText)findViewById(R.id.editText1);
button1=(Button)findViewById(R.id.button1);
button1.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
String message=editText1.getText().toString();
Intent intent=new Intent();
intent.putExtra("MESSAGE",message);
setResult(2,intent);
finish();//finishing activity
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.second, menu);
return true;
}
}
click below button to copy the code from android tutorial team
Output:
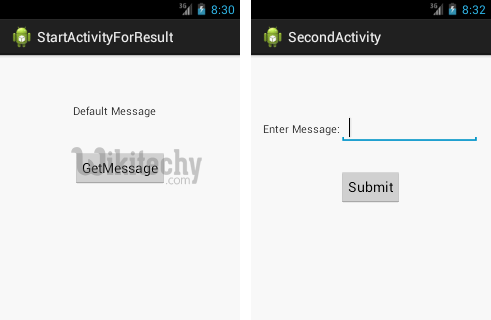
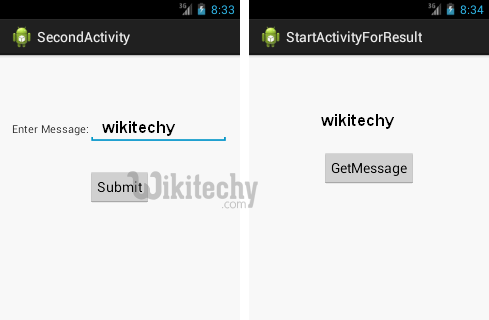