Android tutorial - Android Textbox- android app development - android studio - android development tutorial
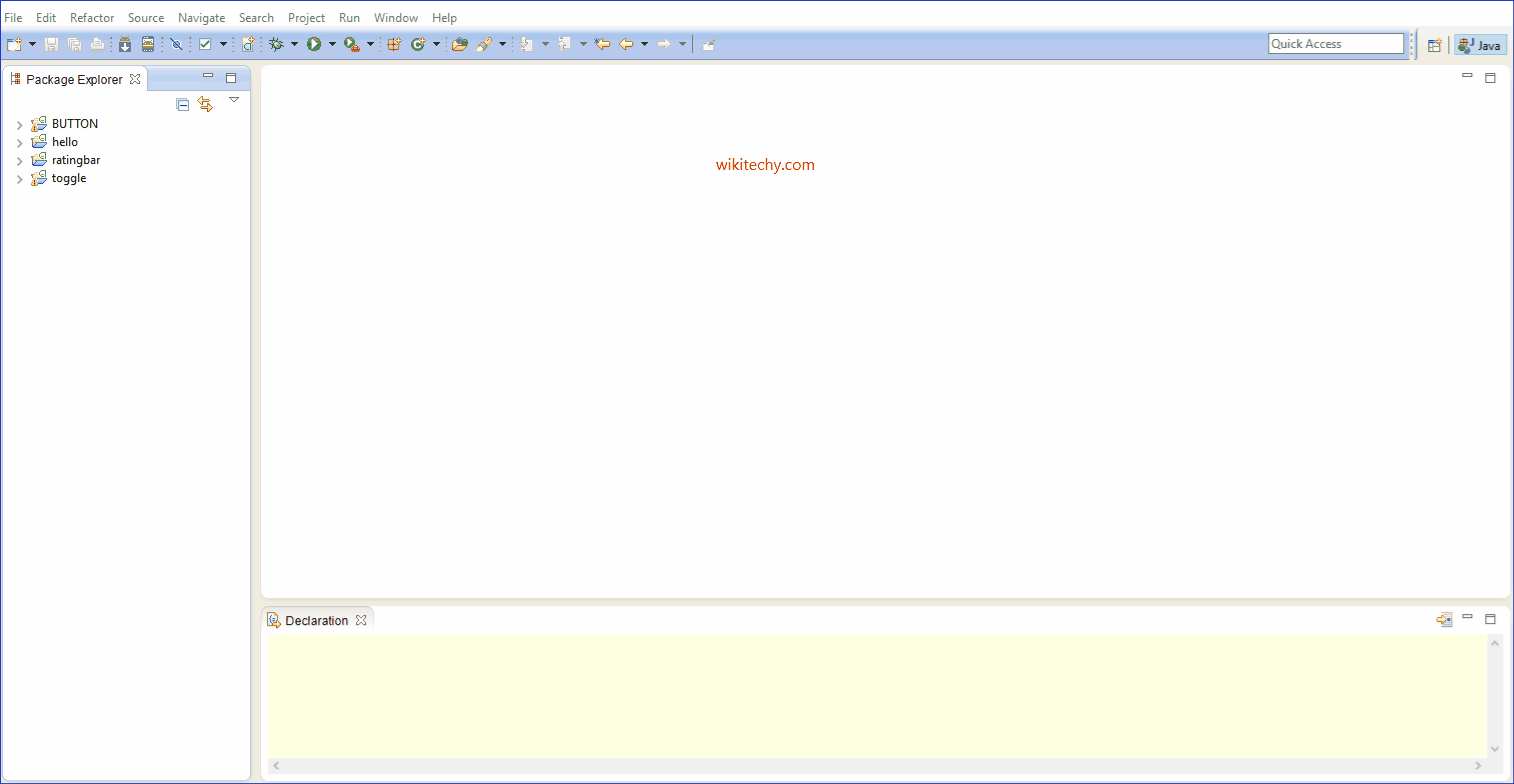
Learn android - android tutorial - Android textbox - android examples - android programs
TextBox:
- On the Insert tab, in the Text group, click Text Box, and then click Draw Text Box.
- Click in the document, and then drag to draw the text box the size that you want.
- To add text to a text box, click inside the text box, and then type or paste text.
- In Android, you can use “EditText” class to create an editable textbox to accept user input.
- This tutorial show you how to create a textbox in XML file, and Demonstrates the use of key listener to display message typed in the textbox.
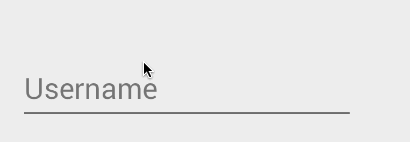
- .
- Open “res/layout/main.xml” file, add a “EditText” component.
1. EditText
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
<requestFocus />
</EditText>
</LinearLayout>
click below button to copy the code from android tutorial team
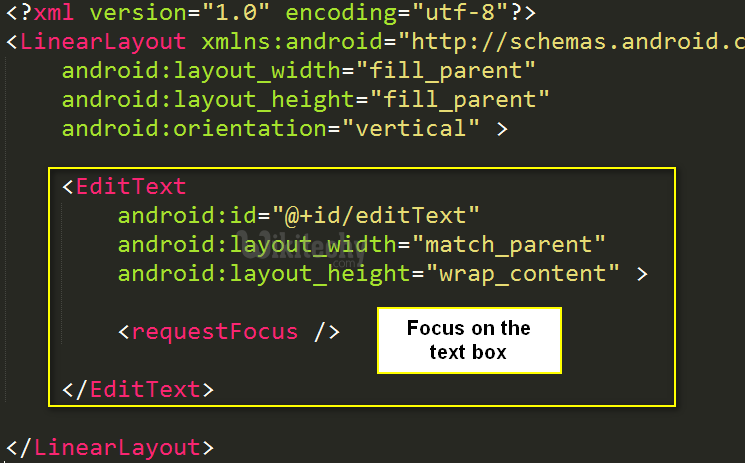
- Attach a key listener inside your activity “onCreate()” method, to monitor following events :
- If “enter” is pressed, display a floating box with the message typed in the “EditText” box.
- If “Number 9” is pressed, display a floating box with message “Number 9 is pressed!”.
2. EditText Listener
package com.wikitechy.android;
import android.app.Activity;
import android.os.Bundle;
import android.view.KeyEvent;
import android.view.View;
import android.view.View.OnKeyListener;
import android.widget.EditText;
import android.widget.Toast;
public class MyAndroidAppActivity extends Activity {
private EditText edittext;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
addKeyListener();
}
public void addKeyListener() {
// get edittext component
edittext = (EditText) findViewById(R.id.editText);
// add a keylistener to keep track user input
edittext.setOnKeyListener(new OnKeyListener() {
public boolean onKey(View v, int keyCode, KeyEvent event) {
// if keydown and "enter" is pressed
if ((event.getAction() == KeyEvent.ACTION_DOWN)
&& (keyCode == KeyEvent.KEYCODE_ENTER)) {
// display a floating message
Toast.makeText(MyAndroidAppActivity.this,
edittext.getText(), Toast.LENGTH_LONG).show();
return true;
} else if ((event.getAction() == KeyEvent.ACTION_DOWN)
&& (keyCode == KeyEvent.KEYCODE_7)) {
// display a floating message
Toast.makeText(MyAndroidAppActivity.this,
"Number 7 - Pressed", Toast.LENGTH_LONG).show();
return true;
}
return false;
}
});
}
}
click below button to copy the code from android tutorial team
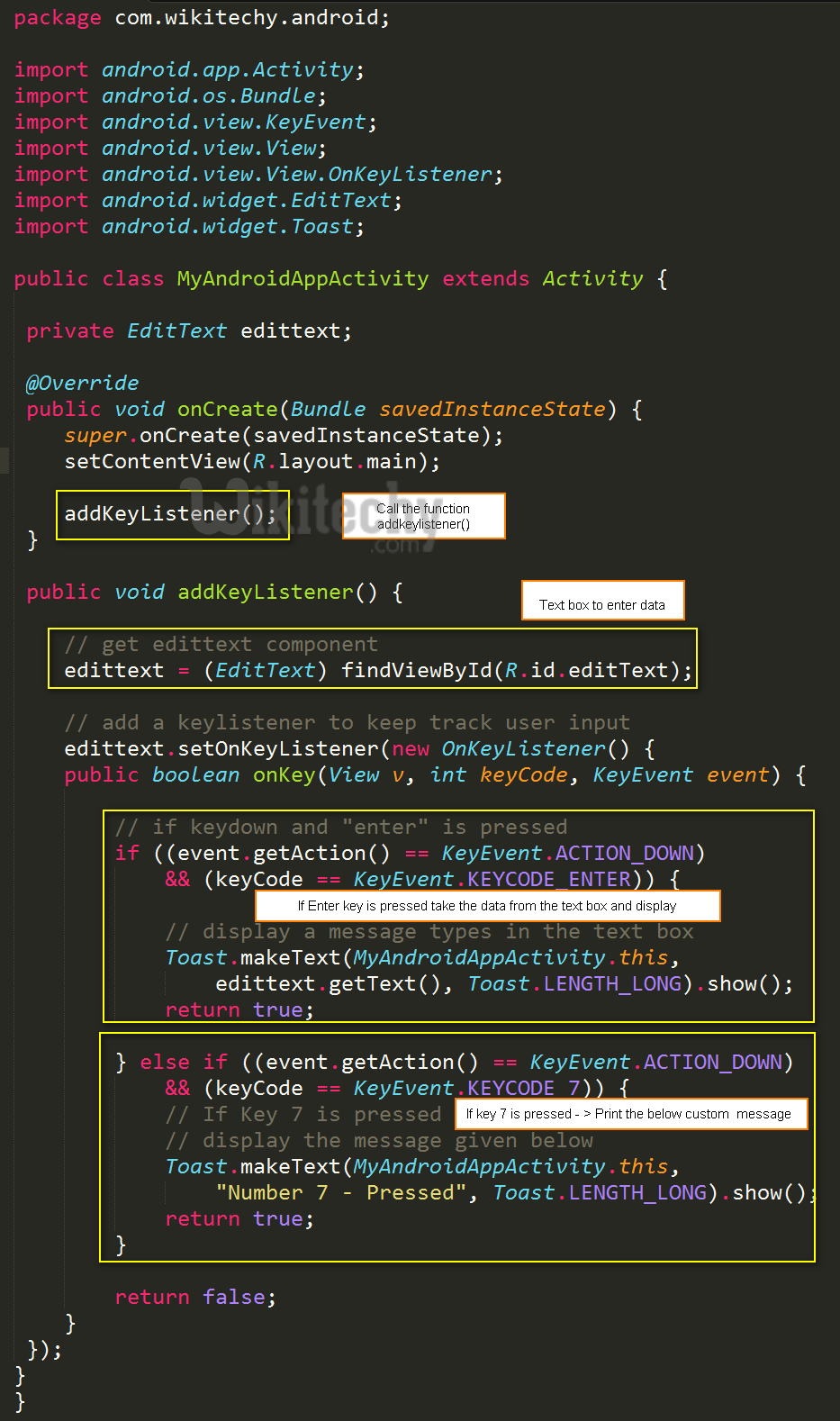
3. Demo - android emulator - android tutorial
- Run the application.
- Type something inside the textbox, and press on the “enter” key :
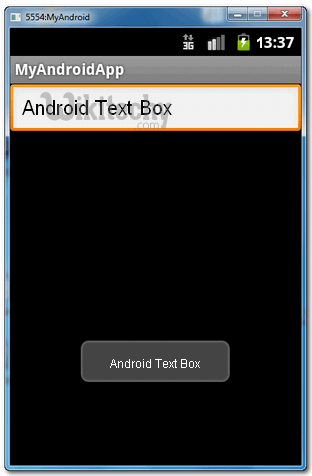
- If key “Number 7” is pressed
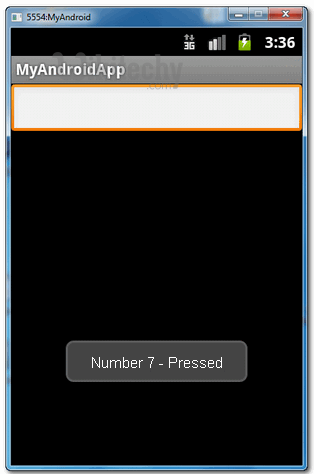