Android tutorial - ratingbar in android - android app development - android studio - android development tutorial
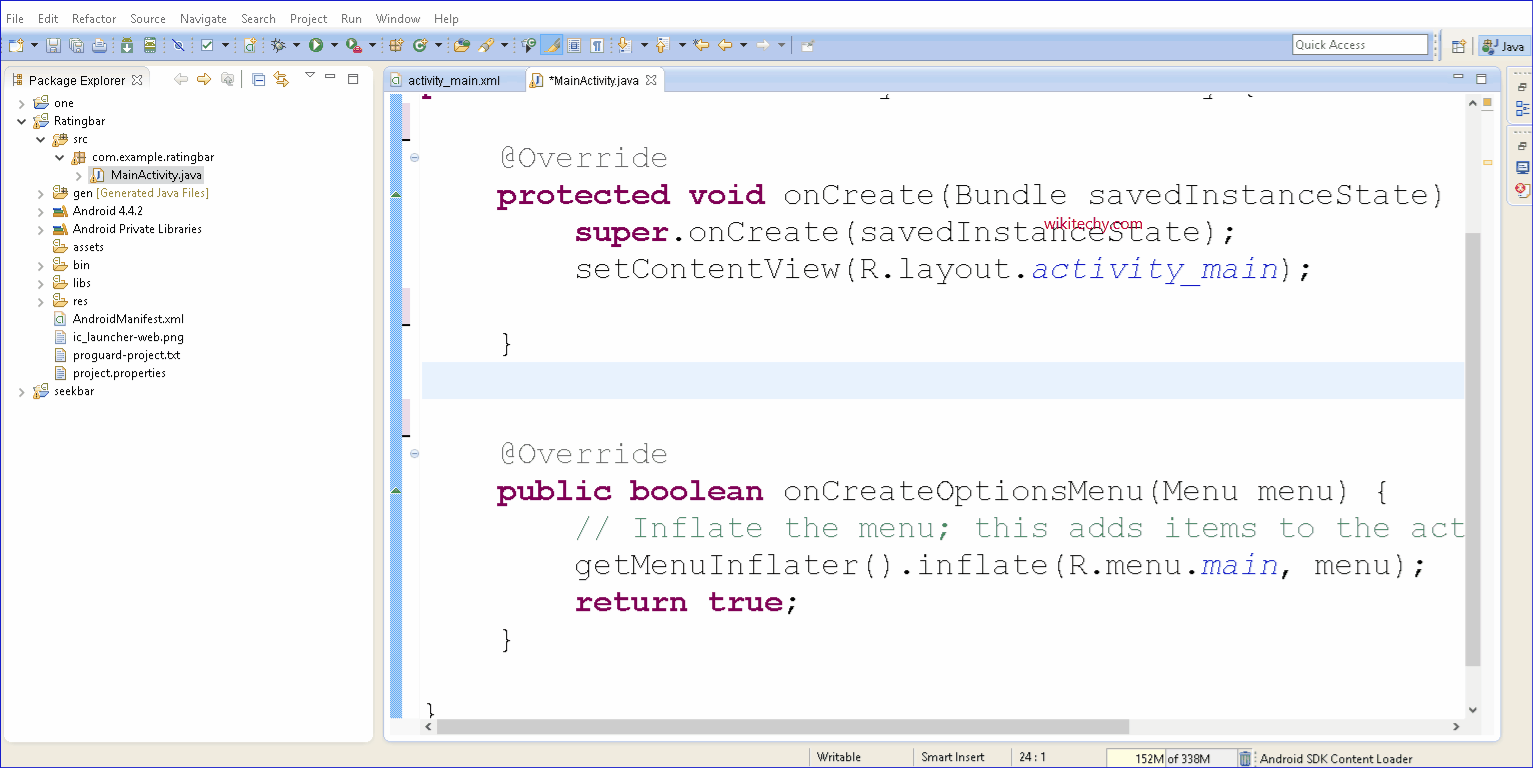
Learn android - android tutorial - Ratingbar in android - android examples - android programs
RatingBar:
- A RatingBar is an extension of SeekBar and ProgressBar that shows a rating in stars. The user can touch/drag or use arrow keys to set the rating when using the default size RatingBar.
- The smaller RatingBar style (ratingBarStyleSmall) and the larger indicator-only style (ratingBarStyleIndicator) do not support user interaction and should only be used as indicators.
- When using a RatingBar that supports user interaction, placing widgets to the left or right of the RatingBar is discouraged.
- The number of stars set (via setNumStars(int) or in an XML layout) will be shown when the layout width is set to wrap content (if another layout width is set, the results may be unpredictable).
- The secondary progress should not be modified by the client as it is used internally as the background for a fractionally filled star.
- A rating is the evaluation or assessment of something, in terms of quality, quantity, or some combination of both.
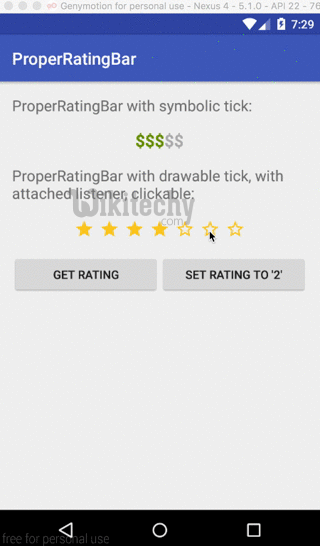
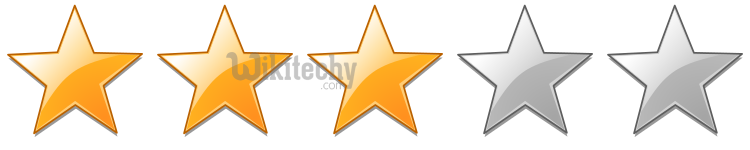
- Rating may also refer to:
- Credit rating, estimating the credit worthiness of an individual, corporation or country
- Fire-resistance rating, the duration for a passive fire protection to withstand a standard fire resistance test
- Naval rating, an enlisted member of a country's Navy not conferred by commission or warrant
- Health care provider ratings
- Performance Rating, in computing, used by AMD
- Ranally city rating, a tool used to classify U.S. cities based on economic function
- Power rating, defined as the highest power input allowed to flow through particular equipment
- Content rating, like the following:
- Web content voting, a system where users rate Web content
- Rating site, website that allows rating
- Reputation system, a score for a set of objects within the community based on a collection of opinions
- Telecommunications rating, the calculated cost of a phone call
- United States presidential approval rating, a polling term which reflects the approval of the President of the United States
1. Rating Bar
- Open “res/layout/main.xml” file,
- add a rating bar component,
- few textviews and a button.
Note
- The rating bar contains many configurable values. In this case, the rating bar contains 4 stars, each increase 1.0 value, so, it contains the minimum of 1.0 (1 star) and maximum value of 4.0 (4 stars). In addition, it made the 2nd star (2.0) selected by default.
- File : res/layout/main.xml
<?xml version="1.0" encoding="utf-8"?>
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:id="@+id/lblRateMe"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Rate Me"
android:textAppearance="?android:attr/textAppearanceMedium" />
<RatingBar
android:id="@+id/ratingBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:numStars="4"
android:stepSize="1.0"
android:rating="2.0" />
<Button
android:id="@+id/btnSubmit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Submit" />
<LinearLayout
android:id="@+id/linearLayout1"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/lblResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Result : "
android:textAppearance="?android:attr/textAppearanceLarge" />
<TextView
android:id="@+id/txtRatingValue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text=""
android:textAppearance="?android:attr/textAppearanceSmall" />
</LinearLayout>
</LinearLayout>
click below button to copy the code from android tutorial team
2. Code Code
- Inside activity “onCreate()” method,
- attach a listener on rating bar,
- fire when rating value is changed.
- Another listener on button, fire when button is clicked.
- File : MyAndroidAppActivity.java
package com.wikitechy.android;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.RatingBar;
import android.widget.RatingBar.OnRatingBarChangeListener;
import android.widget.TextView;
import android.widget.Toast;
public class MyAndroidAppActivity extends Activity {
private RatingBar ratingBar;
private TextView txtRatingValue;
private Button btnSubmit;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
addListenerOnRatingBar();
addListenerOnButton();
}
public void addListenerOnRatingBar() {
ratingBar = (RatingBar) findViewById(R.id.ratingBar);
txtRatingValue = (TextView) findViewById(R.id.txtRatingValue);
//if rating value is changed,
//display the current rating value in the result (textview) automatically
ratingBar.setOnRatingBarChangeListener(new OnRatingBarChangeListener() {
public void onRatingChanged(RatingBar ratingBar, float rating,
boolean fromUser) {
txtRatingValue.setText(String.valueOf(rating));
}
});
}
public void addListenerOnButton() {
ratingBar = (RatingBar) findViewById(R.id.ratingBar);
btnSubmit = (Button) findViewById(R.id.btnSubmit);
//if click on me, then display the current rating value.
btnSubmit.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(MyAndroidAppActivity.this,
String.valueOf(ratingBar.getRating()),
Toast.LENGTH_SHORT).show();
}
});
}
}
click below button to copy the code from android tutorial team
3. Demo - android emulator - android tutorial
- Run the application.
- Result, 2nd star is selected by default.
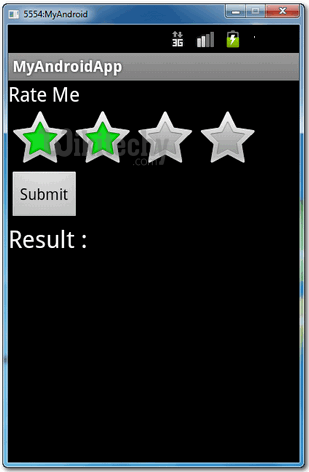
- Touch on the 3rd star, rating value is changed,
- display the current selected value in the result (textview).
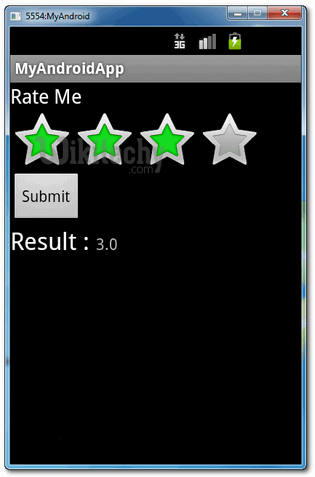
- Touch on the 1st star,
- click on the submit button, the current selected value is displayed as floating message.
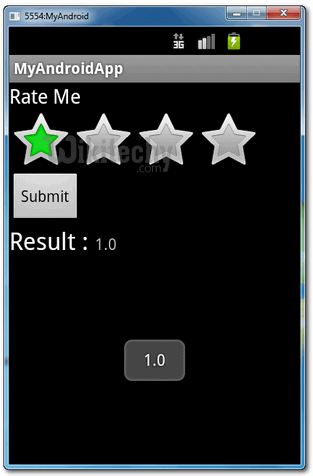