Android tutorial - Android Sensor - android app development - android studio - android development tutorial
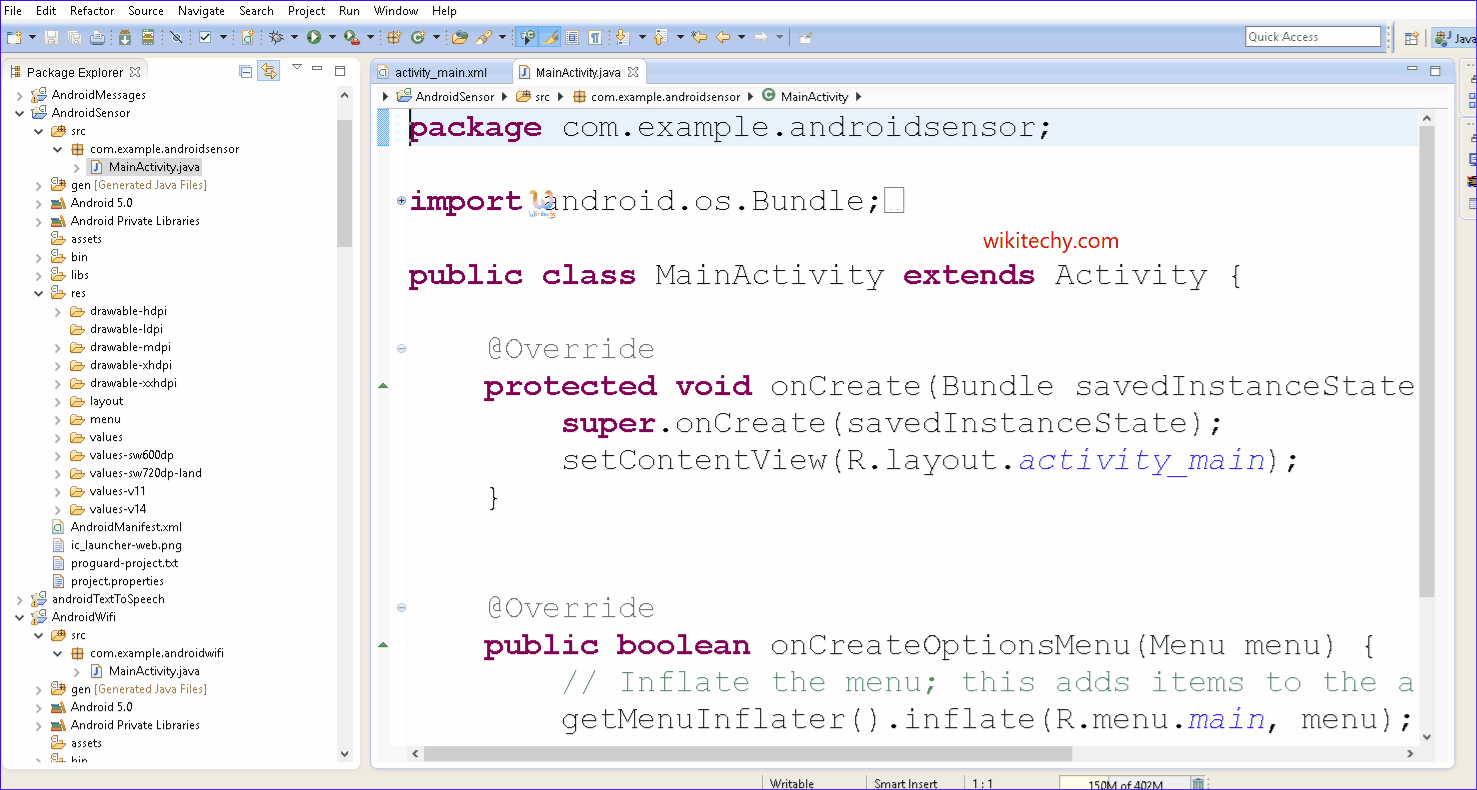
Learn android - android tutorial - Android sensor - android examples - android programs
- Sensors can be used to monitor the three-dimensional device movement or change in the environment of the device.
- Most of the android devices have built-in sensors that measure motion, orientation, and various environmental condition.
- The android platform supports three wide categories of sensors.
- Some of the sensors are hardware based and another some are software based sensors. Whatever the sensor is, android permits us to get the raw data from these sensors and uses it in our application.
- For this android provides us with some classes.
- Android provides SensorManager and Sensor classes to use the sensors in our application.
- In order to use sensors, first thing you need to do is to instantiate the object of SensorManager class.
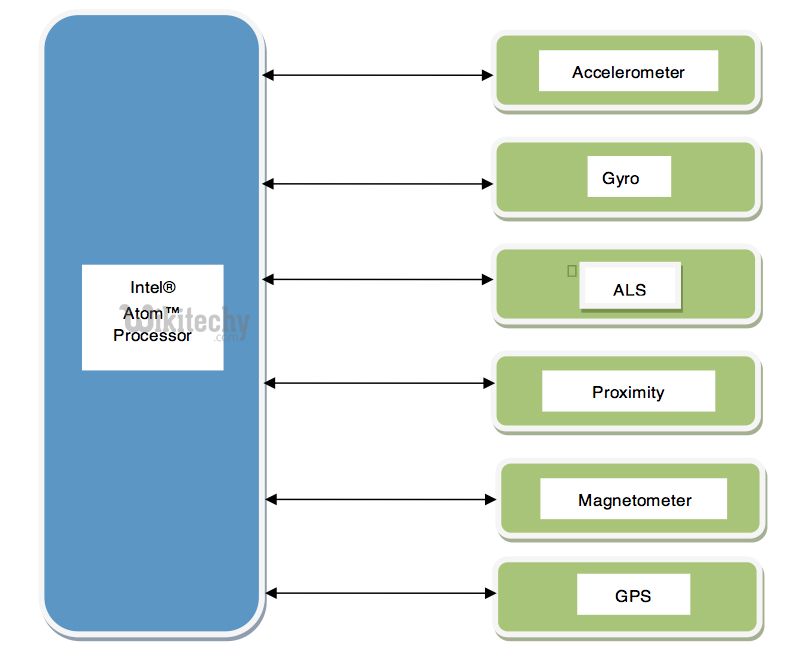
- Android provides sensor api to work with various types of sensors.
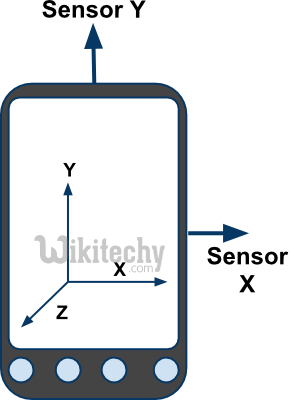
Types of Sensors
- Android supports three types of sensors:
1) Motion Sensors
- Motion Sensors is used to measure acceleration forces & rotational forces along with three axes.
2) Position Sensors
- Position Sensors is used to measure the physical position of device.
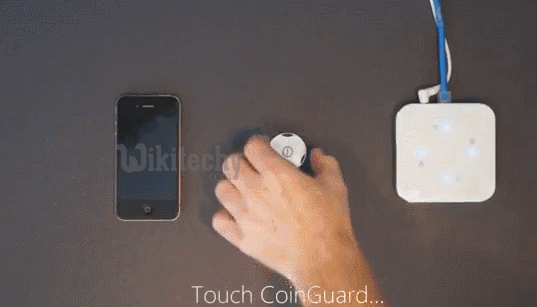
3) Environmental Sensors
- Environmental sensors is used to measure the environmental changes such as temperature, humidity etc.
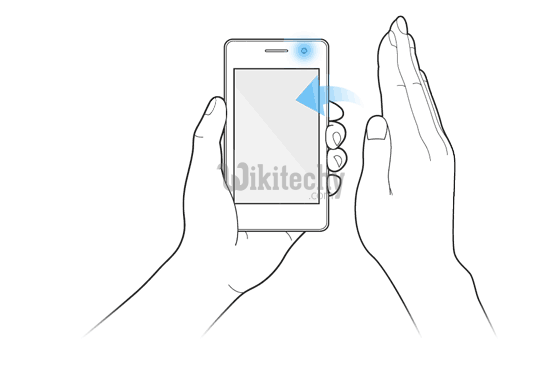
Android Sensor API
- Android sensor api provides various classes & interface. The important classes and interfaces of sensor api are as follows:
1) SensorManager class
- The android.hardware.SensorManager class provides methods :
- to get sensor instance,
- to access and list sensors,
- to register and unregister sensor listeners etc.
- You can get the instance of SensorManager by calling the method getSystemService() & passing the SENSOR_SERVICE constant in it.
SensorManager sm = (SensorManager)getSystemService(SENSOR_SERVICE);
click below button to copy the code from android tutorial team
2) Sensor class
- The android.hardware.Sensor class provides methods to get data of the sensor such as sensor name, sensor type, sensor resolution, sensor type etc.
3) SensorEvent class
- Its instance is created by the system. It provides information about the sensor.
4) SensorEventListener interface
- It provides two call back methods to get information when sensor values (x,y and z) change or sensor accuracy changes.
Android simple sensor app example
- Let's see the two sensor ex’s.
- A sensor example that prints x, y and z axis values. Here, we are going to see that.
- A sensor example that changes the background color when device is shuffled. Click for changing background color of activity sensor example
activity_main.xml
- There is only one text view in this file.
File: activity_main.xml
<RelativeLayout xmlns:androclass="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginLeft="92dp"
android:layout_marginTop="114dp"
android:text="TextView" />
</RelativeLayout>
click below button to copy the code from android tutorial team
Activity class
- Write the code that prints values of x-axis, y-axis and z-axis.
File: MainActivity.java
package com.example.sensorsimple;
import android.app.Activity;
import android.os.Bundle;
import android.widget.TextView;
import android.widget.Toast;
import android.hardware.SensorManager;
import android.hardware.SensorEventListener;
import android.hardware.SensorEvent;
import android.hardware.Sensor;
import java.util.List;
public class MainActivity extends Activity {
SensorManager sm = null;
TextView textView1 = null;
List list;
SensorEventListener sel = new SensorEventListener(){
public void onAccuracyChanged(Sensor sensor, int accuracy) {}
public void onSensorChanged(SensorEvent event) {
float[] values = event.values;
textView1.setText("x: "+values[0]+"\ny: "+values[1]+"\nz: "+values[2]);
}
};
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
/* Get a SensorManager instance */
sm = (SensorManager)getSystemService(SENSOR_SERVICE);
textView1 = (TextView)findViewById(R.id.textView1);
list = sm.getSensorList(Sensor.TYPE_ACCELEROMETER);
if(list.size()>0){
sm.registerListener(sel, (Sensor) list.get(0), SensorManager.SENSOR_DELAY_NORMAL);
}else{
Toast.makeText(getBaseContext(), "Error: No Accelerometer.", Toast.LENGTH_LONG).show();
}
}
@Override
protected void onStop() {
if(list.size()>0){
sm.unregisterListener(sel);
}
super.onStop();
}
}
click below button to copy the code from android tutorial team
Output:
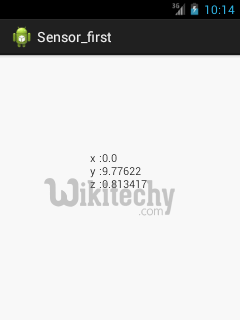