Android tutorial - Android checkbox - android app development - android studio - android development tutorial
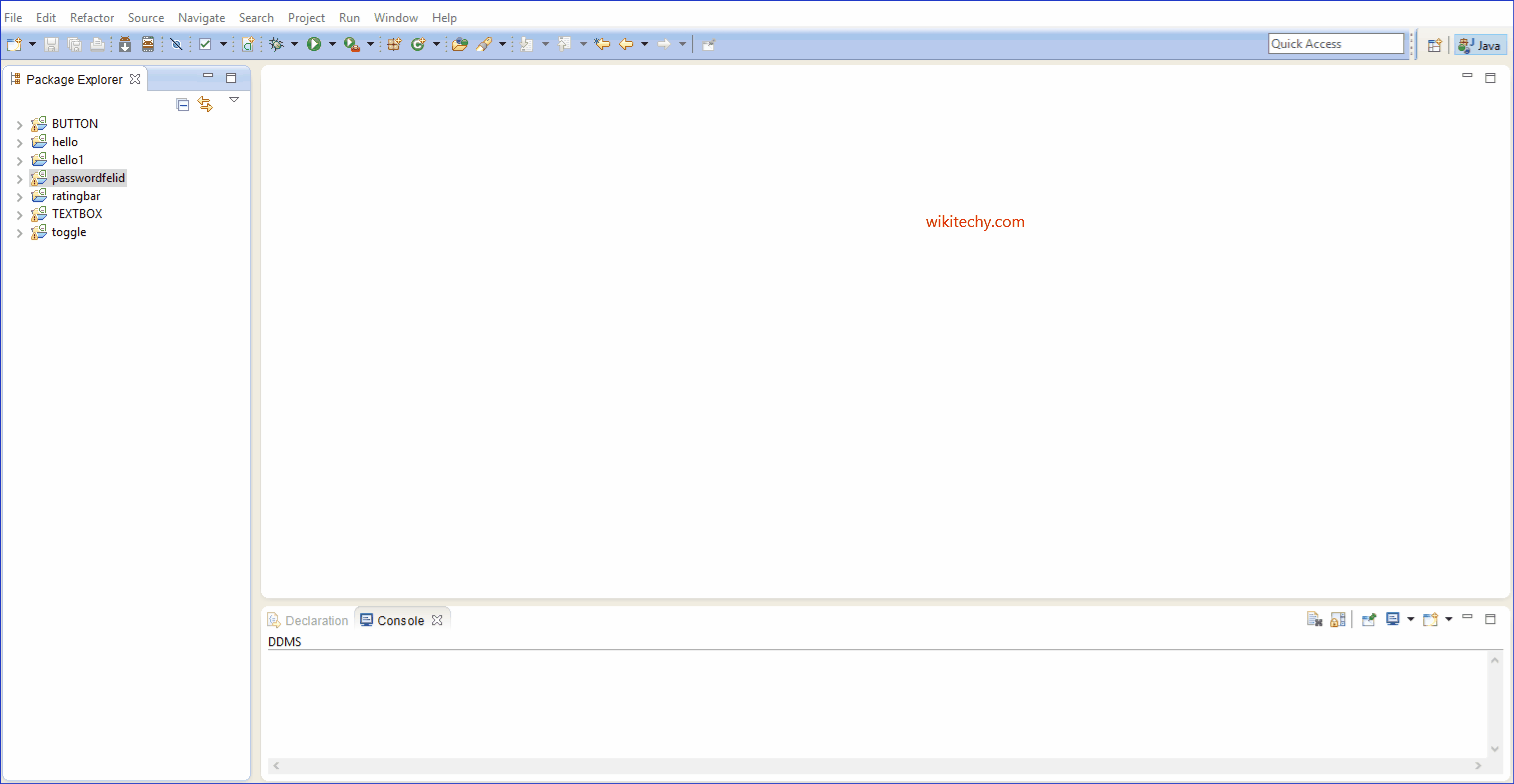
Learn android - android tutorial - Android checkbox - android examples - android programs
Checkbox
- Alternatively referred to as a checkbox, selection box, or tick box, a check box is a square box where the user can click to say that they want or have a particular setting.
- Check boxes are used when more than one option may need to be selected.
- Checking would disable it.
- The Box would enable that option and unchecking it .
- In Android, you can use “android.widget.CheckBox” class to render a checkbox.
- In this tutorial, we show you how to create 3 checkboxes in XML file, and Demonstrates the use of listener to check the checkbox state – checked or unchecked.
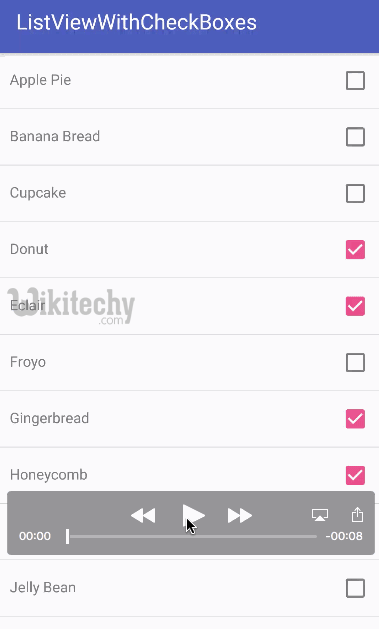
Custom String
- Open “res/values/strings.xml” file, add some user-defined string.
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="hello">Hello World, MyAndroidAppActivity!</string>
<string name="app_name">MyAndroidApp</string>
<string name="chk_ios">IPhone</string>
<string name="chk_android">Android</string>
<string name="chk_windows">Windows Mobile</string>
<string name="btn_display">Display</string>
</resources>
click below button to copy the code from android tutorial team
CheckBox
- Open “res/layout/main.xml” file, add 3 “CheckBox” and a button, inside the LinearLayout.
File : res/layout/main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<CheckBox
android:id="@+id/chkIos"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/chk_ios" />
<CheckBox
android:id="@+id/chkAndroid"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/chk_android"
android:checked="true" />
<CheckBox
android:id="@+id/chkWindows"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/chk_windows" />
<Button
android:id="@+id/btnDisplay"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/btn_display" />
</LinearLayout>
click below button to copy the code from android tutorial team
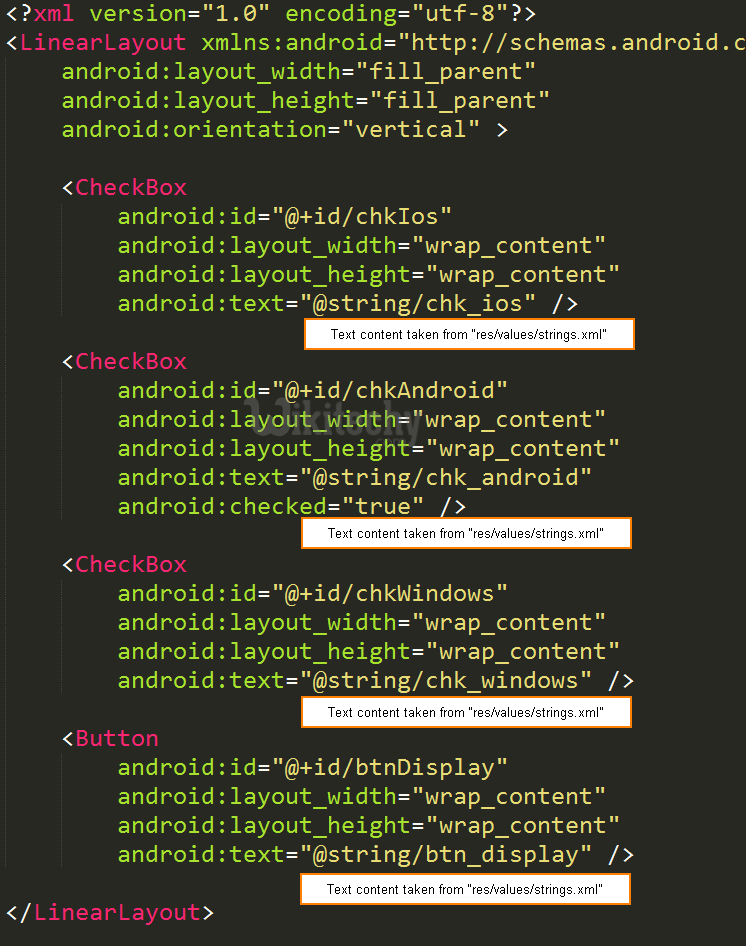
Code
- Attach listeners inside your activity “onCreate()” method, to monitor following events :
- If checkbox id : “chkIos” is checked, display a floating box with message “Wikitechy - Android Tutorial”.
- If button is is clicked, display a floating box and display the checkbox states.
File : MyAndroidAppActivity.java
package com.wikitechy.android;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.Toast;
public class MyAndroidAppActivity extends Activity {
private CheckBox chkIos, chkAndroid, chkWindows;
private Button btnDisplay;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
addListenerOnChkIos();
addListenerOnButton();
}
public void addListenerOnChkIos() {
chkIos = (CheckBox) findViewById(R.id.chkIos);
chkIos.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
//is chkIos checked?
if (((CheckBox) v).isChecked()) {
Toast.makeText(MyAndroidAppActivity.this,
"Wikitechy - Android Tutorial", Toast.LENGTH_LONG).show();
}
}
});
}
public void addListenerOnButton() {
chkIos = (CheckBox) findViewById(R.id.chkIos);
chkAndroid = (CheckBox) findViewById(R.id.chkAndroid);
chkWindows = (CheckBox) findViewById(R.id.chkWindows);
btnDisplay = (Button) findViewById(R.id.btnDisplay);
btnDisplay.setOnClickListener(new OnClickListener() {
//Run when button is clicked
@Override
public void onClick(View v) {
StringBuffer result = new StringBuffer();
result.append("IPhone check : ").append(chkIos.isChecked());
result.append("\nAndroid check : ").append(chkAndroid.isChecked());
result.append("\nWindows Mobile check :").append(chkWindows.isChecked());
Toast.makeText(MyAndroidAppActivity.this, result.toString(),
Toast.LENGTH_LONG).show();
}
});
}
}
click below button to copy the code from android tutorial team
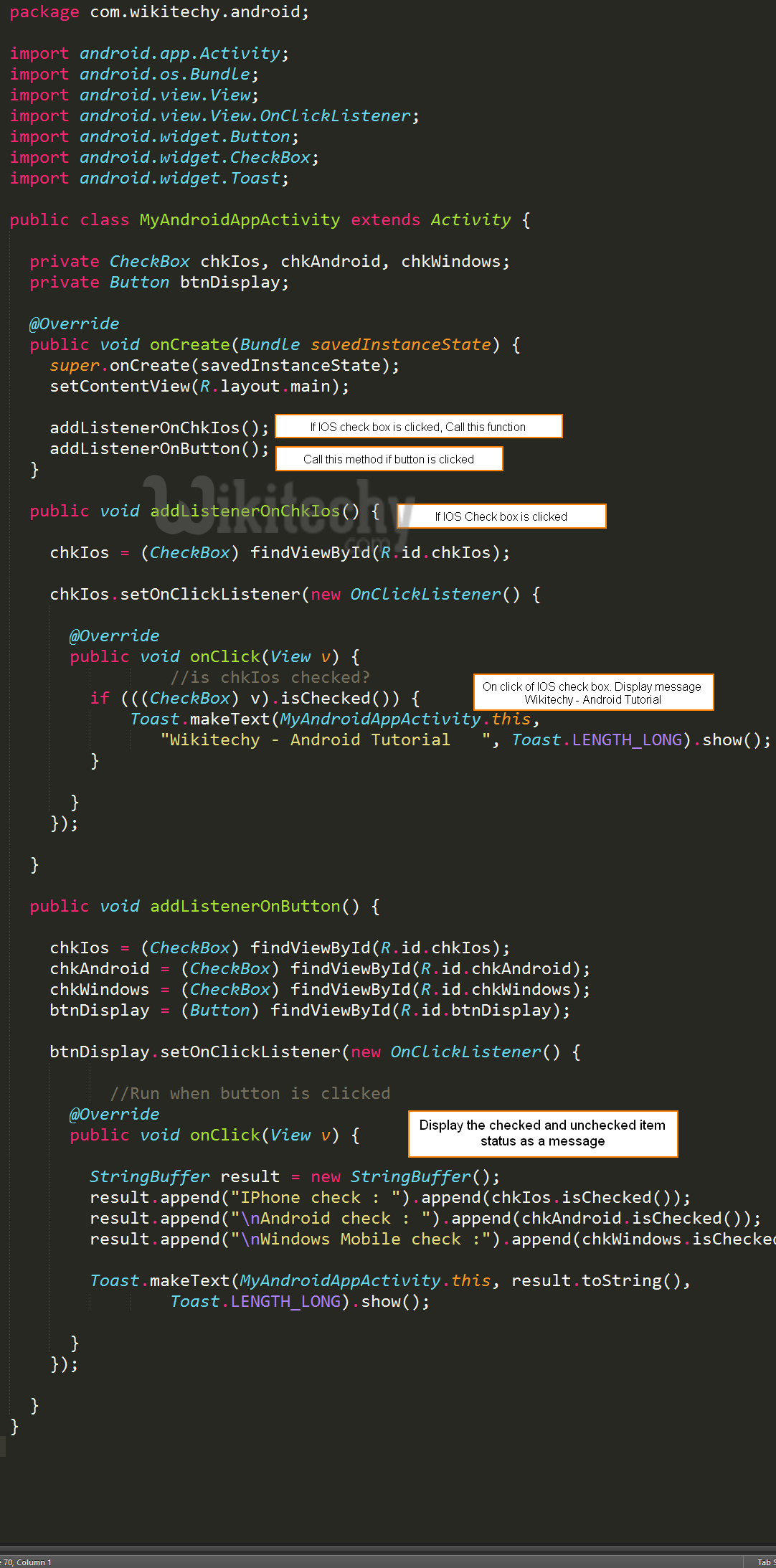
Demo - android emulator - android tutorial
- Run the application.
- Result :
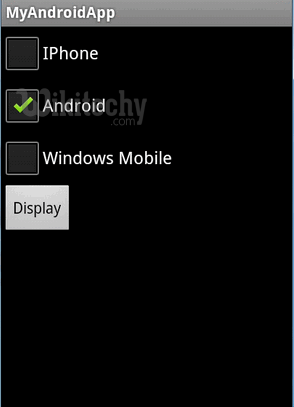
- If “IPhone” is checked :
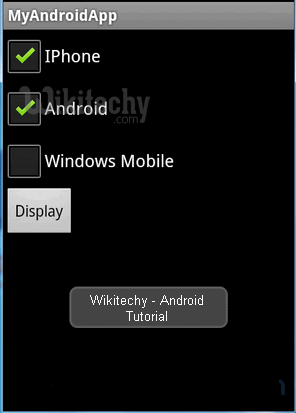
- Checked “IPhone” and “Windows Mobile”, later, click on the “display” button :
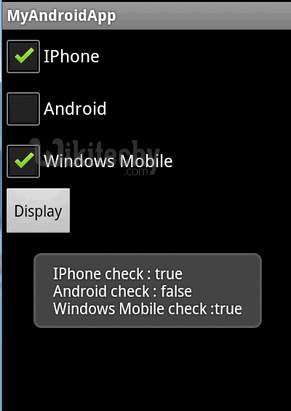