AngularJS Input Time
- input [time] is one of the AngularJS input directive in module ng.
- AnguarJS directive input [time] is used to create a standard HTML text input with time validation and transformation.
- The input must be entered in the format ISO-8601. ( i.e. local time format (HH : mm : ss)
- In browsers does not yet support the HTML5 time input, instead text element will be used in a valid ISO-8601 local time format.
- The data model must be date object.
- The timezones is used to read/write the Date instance in the model by using ngModelOptions.
- This directive executes at priority level 0.
For ex 17:00:25
Syntax for input [text] directive in AngularJS:
<input type="time"
ng-model="string"
[name="string"]
[min ="string"]
[max ="string"]
[ng-min=" "]
[ng-max=" "]
[required ="string"]
[ng-required ="string"]
[ng-change ="string"]>
Parameter Values:
Parameters | Type | Description |
---|---|---|
ngModel | String | Defines angular expression to data-bind to. |
name (optional) | String | Name of the form under which the control is available |
min(optional) | String | To set the min validation error key if the value entered is shorter than min. (e.g “{{ minTime | date :’HH:mm:ss’ }}”) |
max(optional) | String | To set the max validation error key if the value entered is greater than max. (e.g “{{ maxTime | date :’HH:mm:ss’ }}”) |
ngMin (optional) | date, string | To set the min validation constraint to the Date/ISO week string the ngMin expression calculates to. Reminds that it does not set the min attribute. |
ngMax(optional) | date, string | To set the max validation constraint to the Date/ISO week string the ngMax expression calculates to. Reminds that it does not set the max attribute. |
required (optional) | string | Denotes the required validation error key if the value is not entered. |
ngRequired(optional) | String | Sets the required attribute and required validation constraint to the element when the ngRequired expression sets to true. Instead of required use ngRequired when we want data-bind to the required attribute. |
ngChange(optional) | String | An expression of Angular to be executed when input changes due to user interaction with the input element. |
Sample coding for input[time] directive in AngularJS
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<form ng-app="myApp" name="Form" ng-controller="TimeCtrl">
<h3>input[time] directive example in AngularJS</h3>
Display Time
<input type="time" id="Input" name="input" ng-model="value"
placeholder="HH:mm:ss" min="07:00:00" max="15:00:00" required/>
<span ng-show="Form.input.$error.required">
Required!</span>
<span ng-show="Form.input.$error.time"><br/ ><br/ >
Not a valid date!</span>
<p>value = {{value | date: "HH:mm:ss"}}</p>
<p>Form.input.$valid = {{Form.input.$valid}}</p>
<p>Form.input.$error = {{Form.input.$error}}</p>
<p>Form.$valid = {{ Form.$valid }}</p>
<p>Form.$error.required = {{ !!Form.$error.required }}</p>
</form>
<script>
var app = angular.module("myApp", []);
app.controller('TimeCtrl', function($scope) {
}]);
</script>
</body>
</html>
Code Explanation input [range] directive in AngularJS:
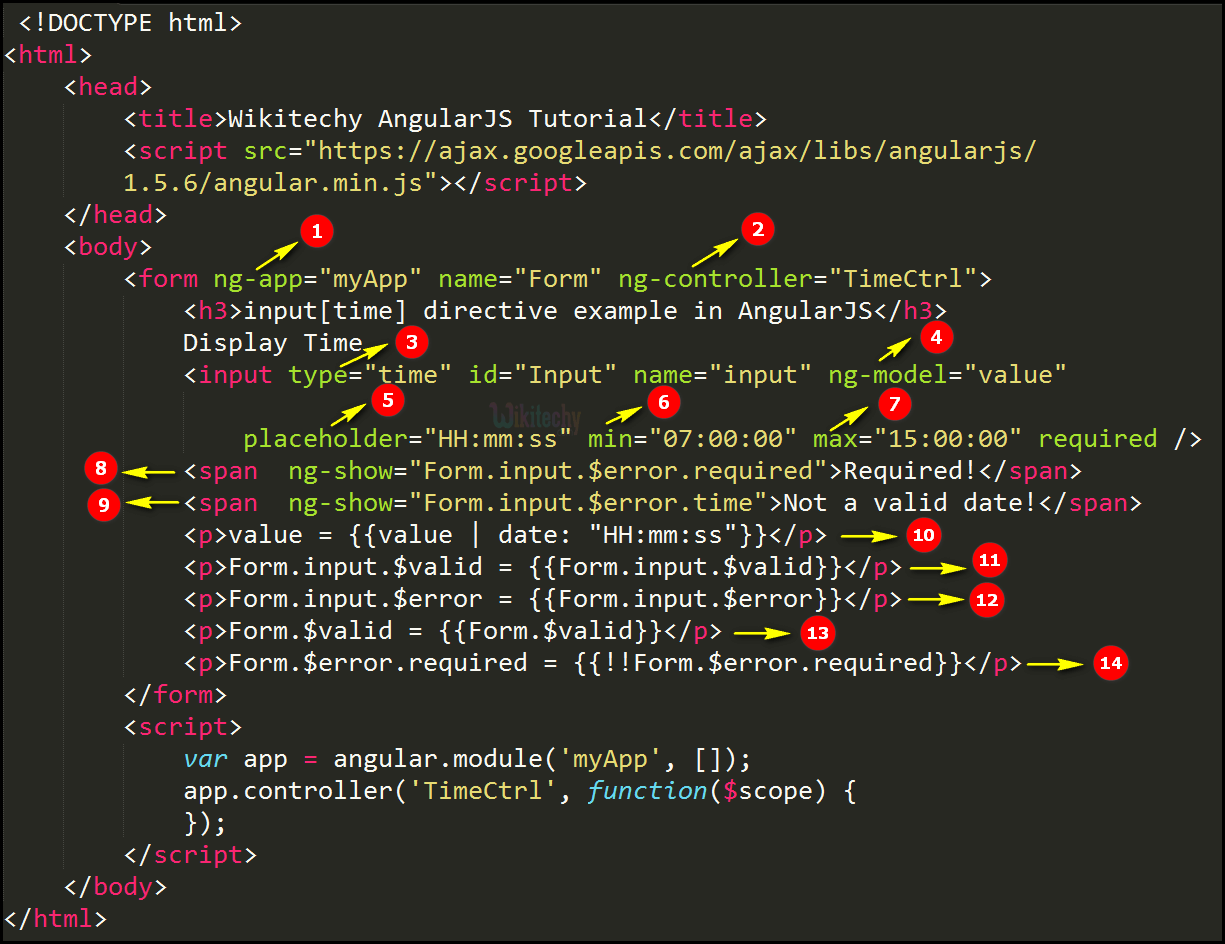
- The ng-app specifies the root element (“myapp”) to define AngularJS application.
- ng-controller specifies the application controller in AngularJS the controller value is given as “TimeCtrl”.
- “text” is declare the type value of the <input> tag.
- The ng-model bind an input field value to AngularJS application variable (“value”).
- Placeholder is used to declare the time format ( Like HH:mm:ss).
- min parameter is used to declare the min time value(07:00:00)
- max parameter is used to declare the max time value(15:00:00)
- ng-show directive is used to hides HTML elements. If the required directive shows an error the content is displayed like “Required”
- ng-show directive is used to hides HTML elements. If the required directive shows an error the content is displayed like “Not a Valid date!”.
- Here the date filter formats a date into specified format (HH:mm:ss) and the output will be updated in the <p> tag.
- Form.input.$valid to checks the correct time format or not. If the time in the input field then the output will be displays as true otherwise false.
- Form.input.$error to check whether the valid time format or not .If the time specified in error it through the exception (Like “required”=”true) otherwise it is an empty curly braces ( { } ).
- Form.$valid is used to check whether the form is valid or not and output will be displayed in <p> tag.
- Form.error.$required is used to time is required or not. If the time is required and output will be displays as false otherwise true.
Sample Output input[time] directive in AngularJS:
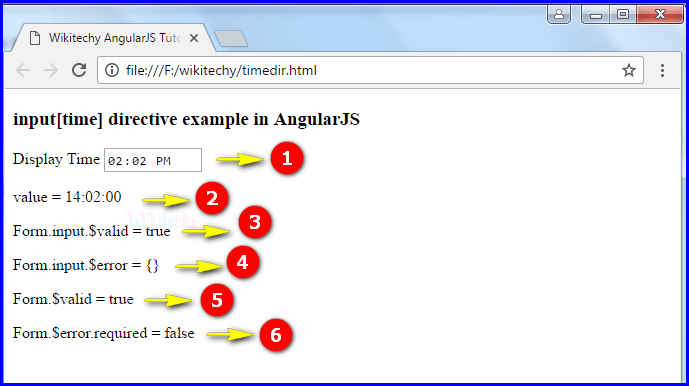
- If the user scroll the time in the input field.
- The output will be displays as value=14:02:02
- The output displays true because it is consider as a time.
- The output displays empty curly braces it means does not thrown any error.
- The output displays empty curly braces it means does not thrown any error.
- The output displays true it is valid time.
- If the text box does not empty so the output displays as false.
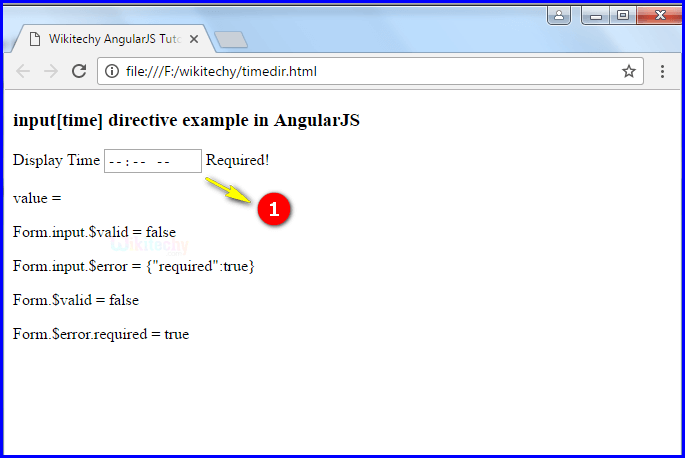
- If the user does not type any time in the input field and the output shows an error “Required”.
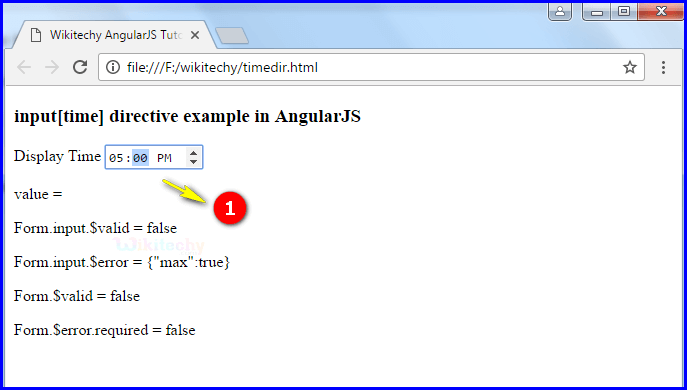
- If the user scroll the time 5.00PM ( Max=”15:00:00”) then the output displays as empty value(i.e. value=).