AngularJS Input Month
- input [month] is one of the AngularJS input directive in module ng.
- AnguarJS directive input [month] is used to create an HTML element with month validation and transformation.
- The input value text must be entered in the format ISO-8601 ( i.e. yyyy-MM)
- This directives executes at priority level 0
- The model should be a Date object or else Angular will throw an error.
- Invalid Date objects ( getTime( ) ) will be reflected as an empty string.
- The model is not set to the first of the month, then the next view to model update will set into the first of the month.
For ex: 2016-12.
Syntax for input [month] directive in AngularJs:
<input type="month"
ng-model="string"
[name="string"]
[min ="string"]
[max ="string"]
[ng-min="date or string"]
[ng-max=" date or string "]
[required ="string"]
[ng-required ="string"]
[ng-change ="string"]>
Parameter Values:
Parameter | Description |
---|---|
ngModel | Defines angular expression to data-bind to. |
name (optional) | Name of the form under which the control is available. |
min(optional) | To set the min validation error key if the value entered is shorter than min. (e.g {{ minmonth | date :’yyyy-MM’}}) |
max(optional) | To set the max validation error key if the value entered is greater than max. (e.g {{ maxmonth | date :’yyyy-MM’}}) |
ngMin (optional) | To set the min validation constraint to the Date/ISO week string the ngMin expression calculates to. Reminds that it does not set the min attribute. |
ngMax (optional) | To set the max validation constraint to the Date/ISO week string the ngMax expression calculates to. Reminds that it does not set the max attribute. |
required (optional) | Denotes the required validation error key if the value is not entered. |
ngRequired (optional) | Sets the required attribute and required validation constraint to the element when the ngRequired expression sets to true. Instead of required use ngRequired when we want data-bind to the required attribute. |
ngChange (optional) | An expression of Angular to be executed when input changes due to user interaction with the input element. |
Sample coding for input[month] directive in AngularJS
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<form ng-app="myApp" name="Form" ng-controller="DateCtrl">
<h3>input[month] directive example</h3>
Pick a Month in 2016:
<input type="month" name="input" ng-model="value"
placeholder="yyyy-MM" min="2016-01" max="2016-12"
required/>
<span ng-show="Form.input.$error.required">
Required!</span>
<span ng-show="Form.input.$error.month">
Not a valid month!</span>
<p>value = {{value | date: "yyyy-MM"}}</p>
<p>Form.input.$valid = {{ Form.input.$valid }}</p>
<p>Form.input.$error = {{ Form.input.$error }}</p>
<p>Form.$valid = {{ Form.$valid }}</p>
<p>Form.$error.required = {{ !!Form.$error.required }}</p>
</form>
<script>
var app = angular.module("myApp", []);
app.controller('DateCtrl', ['$scope', function($scope) {
}]);
</script>
</body>
</html>
Code Explanation input[email] directive in AngularJS:
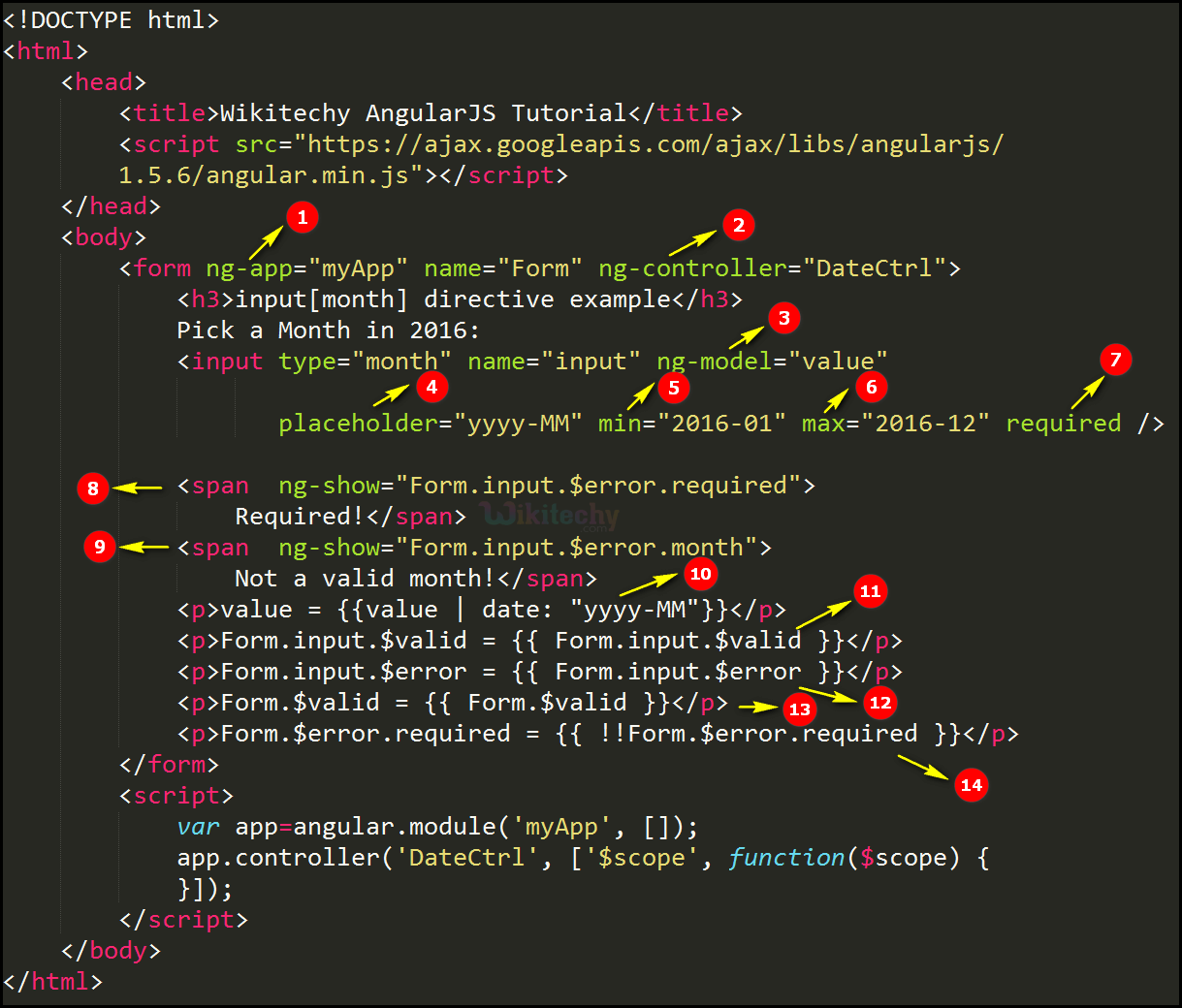
- The ng-app specifies the root element (“myapp”) to define AngularJS application.
- ng-controller specifies the application controller in AngularJS the controller value is given as “DateCtrl”.
- The ng-model bind an input field value to AngularJS application variable (“value”).
- Placeholder is used to declare the month format ( Like yyyy-MM).
- min is used to set the min validation key starts from 2016-1
- max parameter is used to declare the max validation key (i.e. 2016-12)
- If the value is not entered into the text field then the required parameter is used.
- ng-show directive is used to hides HTML elements. If the required directive shows an error the content is displayed like “Required”
- ng-show directive is used to hides HTML elements. If the required directive shows an error the content is displayed like “Not a valid month”
- Here the date filter formats a date into specified format (2016-12) and the output will be updated in the <p> tag.
- Form.input.$valid to checks the valid month format or not. If the month is correct and the output displays true otherwise false.
- Form.input.$error to check whether the month is error or not .If the month specified in error it throw an exception (Like “required”=”true) otherwise it is an empty curly braces ( { } ).
- Form.$valid is used to check whether the form is valid or not and output will be displayed in <p> tag.
- Form.$error.required is used to month is required or not. If the month is required and output will be displayed as false otherwise true.
Sample Output input[month] directive in AngularJS:
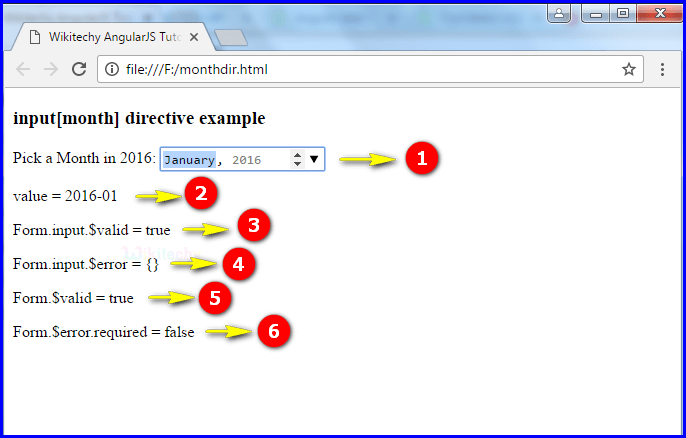
- If the user scroll the month in the input field.
- The output will be displays as value=2016-01.
- The output displays true because it is consider as a specified month format.
- The output displays empty curly braces it means there is no error.
- The output displays true it is valid month.
- If the text box does not empty so the output displays as false.
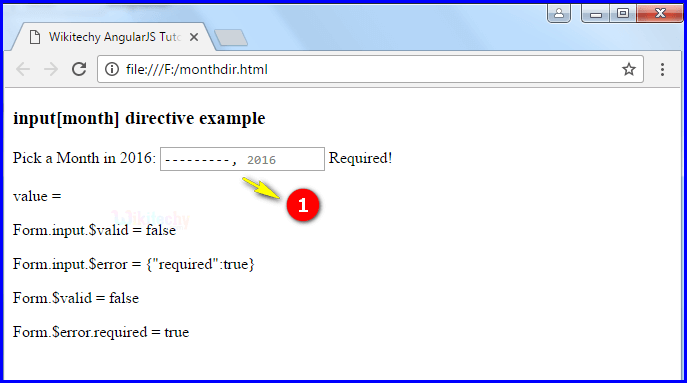
- If user does not specify any month in the input field and output displays as “Required”.