AngularJS Input Checkbox
- input [checkbox] is one of the input directive in module ng.
- Checkbox means when a user selected by the box which shows that a particular feature has been enabled.
- It can be used with <input type=”checkbox”>
- The default value of input [checkbox] is true and false.
- This directive executes at priority level is 0.
Syntax for input[checkbox] directive in AngularJs:
<input type="checkbox"
ng-model="string"
[name="string"]
[ng-true-value="expression"]
[ng-false-value="expression"]
[ng-change="string"]>
Parameter Values:
Parameter | Description |
---|---|
ngModel | Defines angular expression to data-bind to. |
name (optional) | Name of the form under which the control is available. |
ngTrueValue (optional) | ng-true-value to which the expression must be set when selected. |
ngFalseValue (optional) | ng-false-value to which the expression must be set when not selected. |
ngChange(optional) | An expression of Angular to be executed when input changes due to user interaction with the input element. |
Sample coding for input[checkbox] directive in AngularJS
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<form ng-app="myApp" ng-controller="checkboxCtrl">
<h3>input[checkbox] directive example</h3>
<label>Checkbox1:
<input type="checkbox" ng-model="value1">
</label><br/>
<tt>Checkbox1 value = {{value1}}</tt><br/><br/>
<label>Checkbox2:
<input type="checkbox" ng-model="value2"
ng-true-value=" 'Checked' " ng-false-value=" 'Unchecked' ">
</label><br/>
<tt>Checkbox2 value = {{value2}}</tt><br/>
</form>
<script>
var app = angular.module("myApp", []);
app.controller('checkboxCtrl', ['$scope', function($scope) {
$scope.value1 = true;
$scope.value2 = 'Checked';
}]);
</script>
</body>
</html>
Code Explanation for Create input[checkbox] Directive in AngularJS:
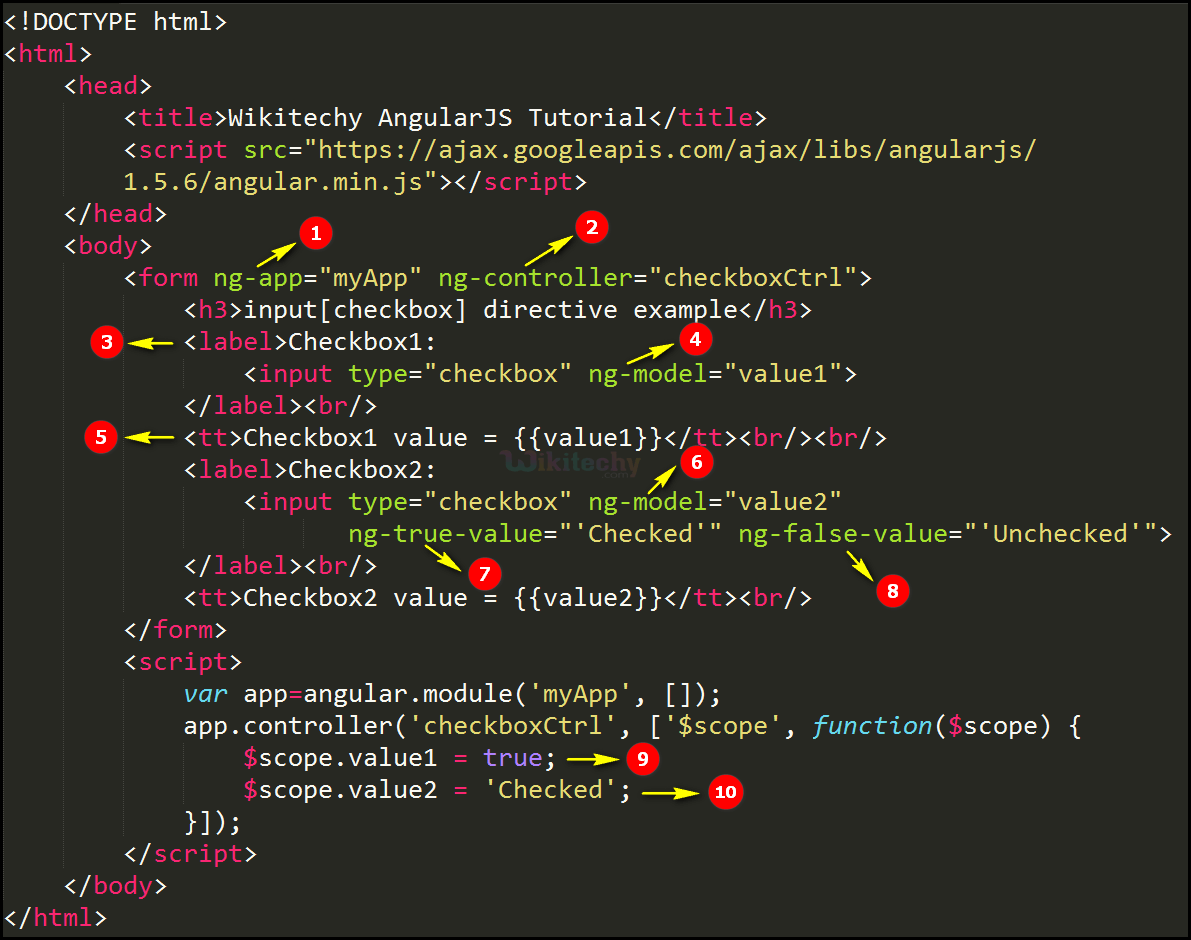
- The ng-app specifies the root element (“myapp”) to define AngularJS application.
- ng-controller specifies the application controller in AngularJS the controller value is given as “checkboxctrl”.
- <label> tag is used label for an input element.
- The ng-model bind an input field value to AngularJS application variable (“value1”).
- <tt> tag is used for text in teletype text.
- The ng-model bind an input field value to AngularJS application variable (“value2”).
- ng-true-value is used to “checked” expression should be set when user click the checkbox and the output will displays the ‘checked’.
- Ng-false-value is used to “unchecked” expression should be set when user unclick the checkbox and the output will displays “unchecked”.
- $scope.value1 is used to declare the value as “true”.
- $scope.value2 is used to declare the value as “checked”.
Sample Output input[checkbox] directive in AngularJS:
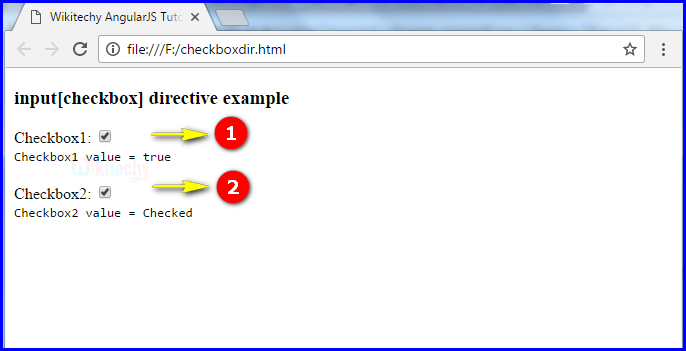
- When user click the checkbox1 and the checkbox1 value will be displays true.
- when user click the checkbox2 and the checkbox2 value will be displays checked.
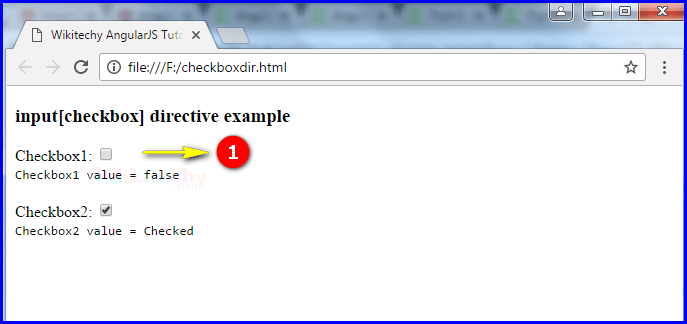
- If the user unclick the checkbox1 then the output will be taken as a default value false.
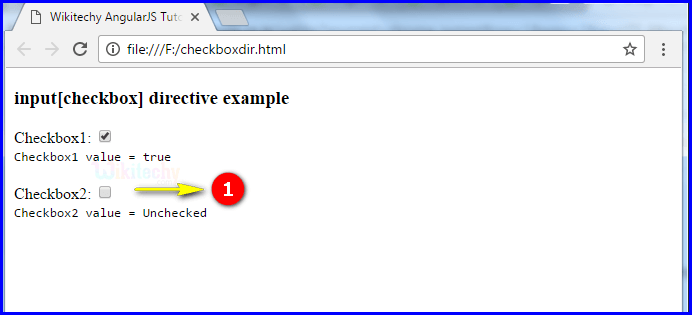
- If the user unclick the checkbox2 button then the output will be displays “unchecked”.