Web Services in C# - ASP.Net Web Services
Web Services in C#
- Web Service is otherwise known as software program and In the simple term, we use the Web Service to interact with the objects over the internet.
- Those services use the XML to exchange the information with the other software with the help of the common internet Protocols.
- Web Service is not dependent on any particular language and it is a protocol Independent.
- Web Service is platform-independent and it is also scalable.
Technology Used in Web Service
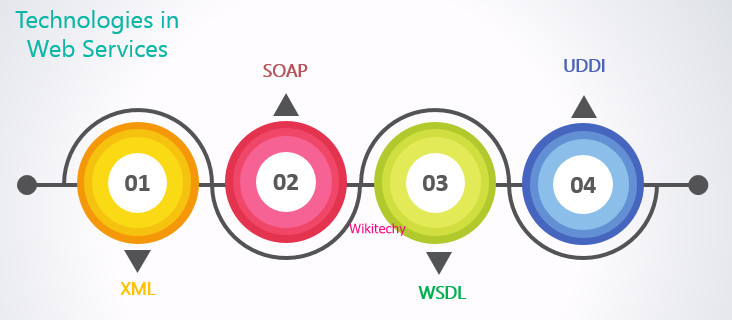
XML
- Web Service specifies only the data and so, the application which understands the XML regarding the programming language or the platform can format the XML in different ways.
SOAP
- SOAP is used to set up the communication between the Services and the application.
WSDL
- WSDL gives us a uniform method that is helpful to specify the Web Services to the other programs.
UDDI
- We can search the Web Service registries with the help of UDDI.
Advantages of Web Services
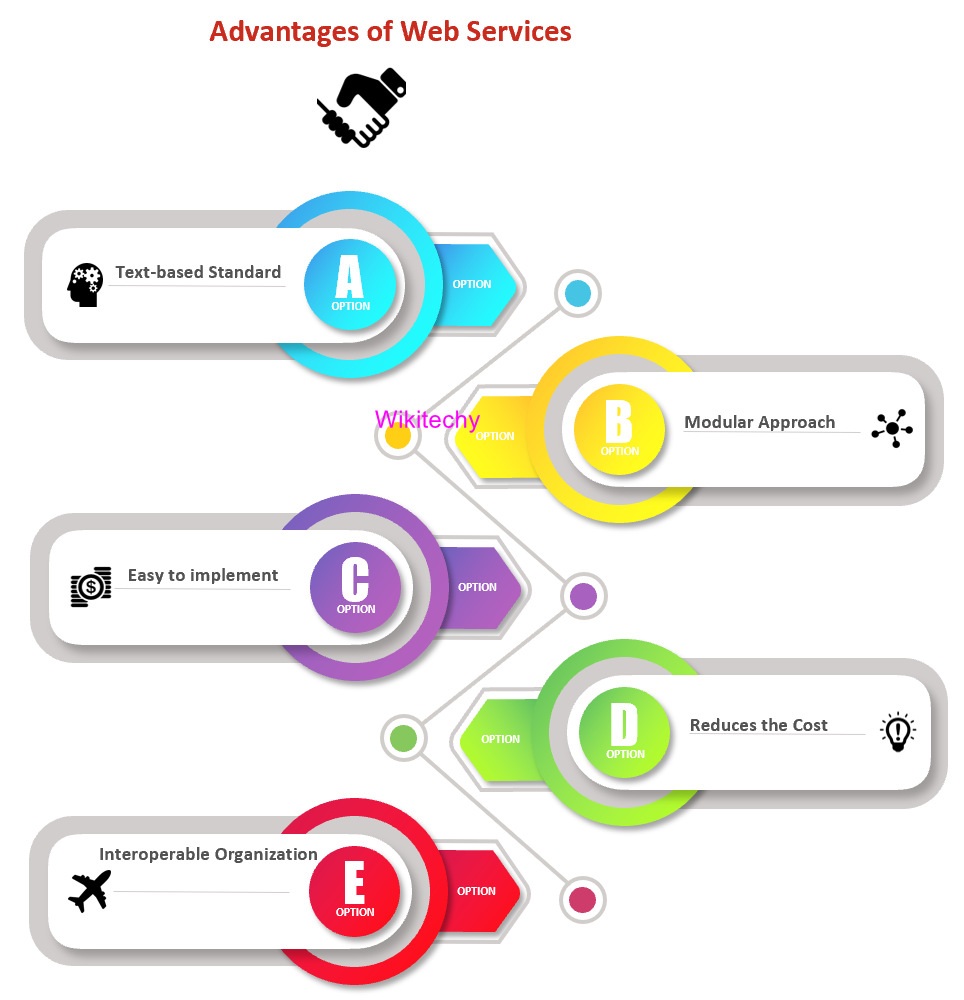
- Web Services always use the open, text-based standard and it uses all those components even they are written in various languages for different platforms.
- With same web service, it promotes the modular approach of the programming so that the multiple organizations can communicate.
- They are easy to implement but expensive because they use the existing infrastructure, and we can repackage most of the applications as the Web Service.
- It reduces the cost of enterprise application integration and B2B communications.
- They are the interoperable Organization that contains the 100 vendors and promote them interoperability.
Limitations of Web Services
- One of the limitations of the Web Services is that the WSDL, SOAP, UDDI requires the development.
- Supports to the interoperability is also the limitation of the Web Service.
- The use of web service increases the traffic on the network and security level for Web Services is low.
- We use the standard procedure to describe the quality of particular web services and standard for the Web Service is in the draft form.
- Whenever we want to use web services for the high-performance situation, in that case, web service will be slow.
Example of the Web Service
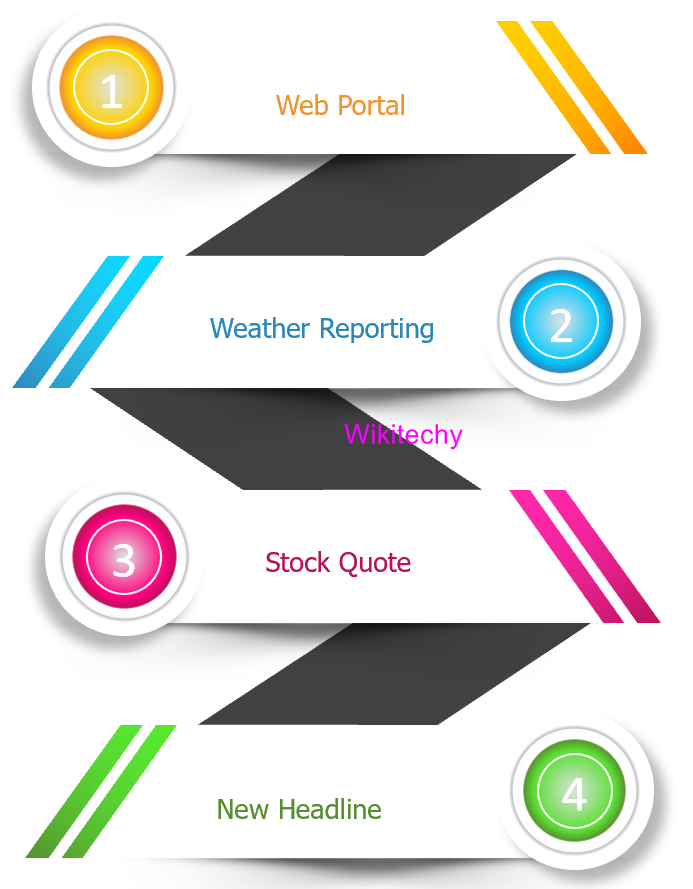
Web Portal
- It is used to fetch the headline news from the linked web service.
Weather Reporting
- We will use Weather Reporting Web Service for displaying the information about the weather on our website, for the reporting of weather.
Stock Quote
- The latest information about the share market with the Stock Quote can display on our website.
News Headline
- We can show the latest update of the news on our website, by using the news headline Web Service.
Data Types supported by the Web Service
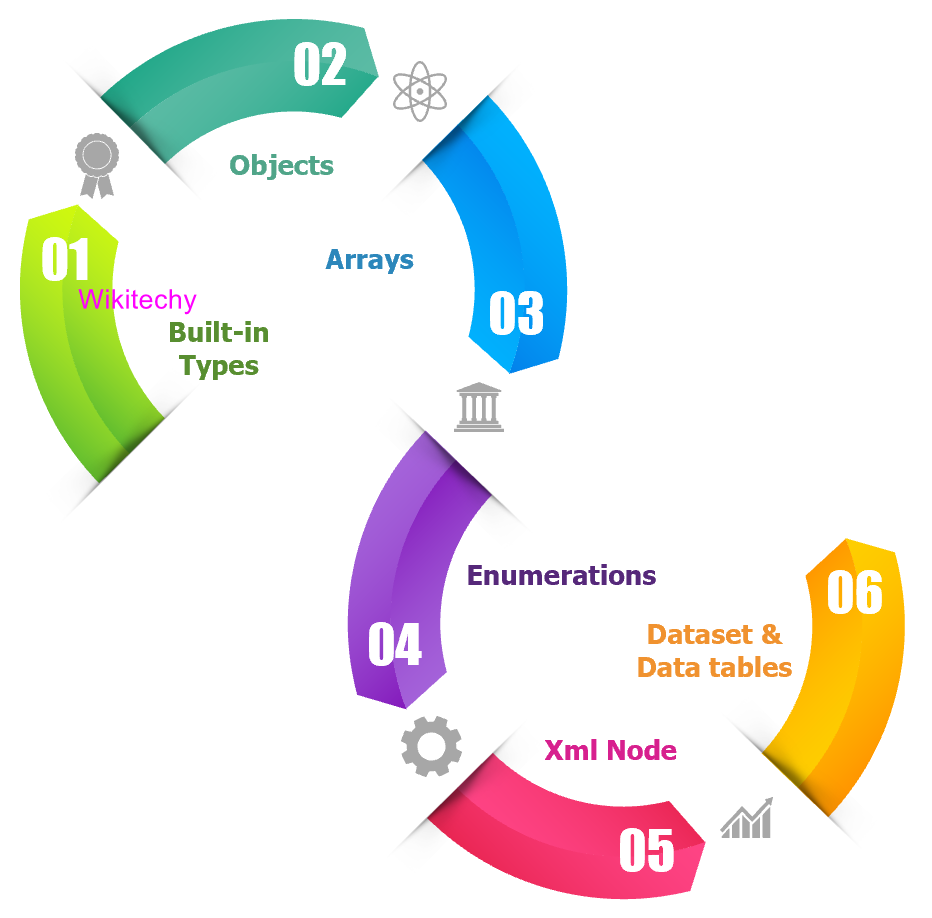
Built-in Types (Basic)
- In C#, web services use the built-in data types like short, int, long, short, float, char, byte, string, and Date Time.
Objects
- WPF uses the object of the user-defined class.
- The class will not be possible to transmit to the client, in spite of this thing that the class contains the methods.
Arrays
- WPF uses the arrays of any supported data type like built-in or customs and We can also use the Array List in WPF.
Enumerations
- WPF supports enum and it will not use integer.
- WPF uses the string name, for the value of the enumeration.
XML Node
- Objects which are based on the System.Xml.XmlNode represents the portion of the XML document.
- Here we can use the Xml Node to send the arbitrary XML.
Data Set and Data Table
- WPF supports data Set and Data Table, but the WPF does not support the ADO.NET data objects like Data Columns and Data Rows.
How to create Web Service
Step->1
- First, we will create a Web Application for creating the Web Service.
- For that, we will click on the File-> Select Project as shown in the below screenshot:
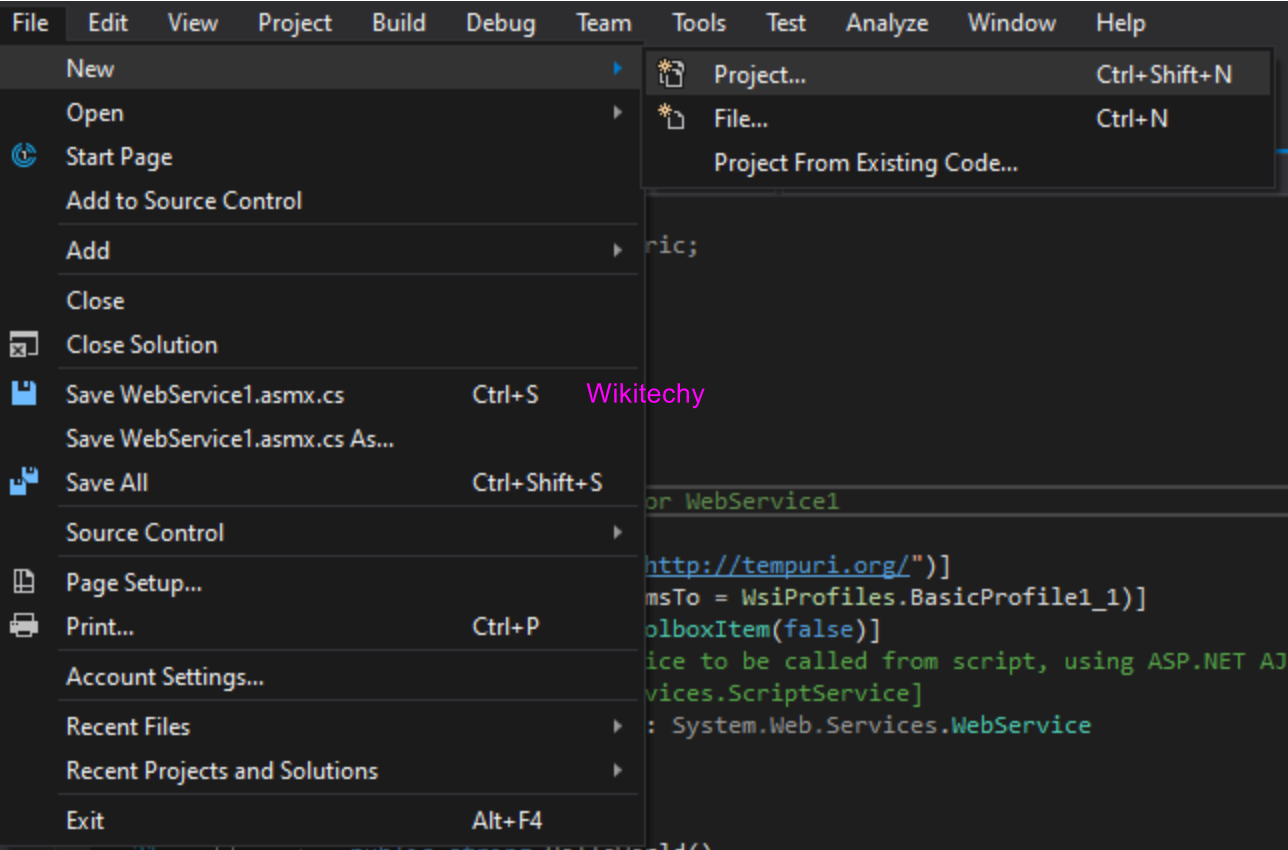
Step->2
- We will select the Web->Asp.Net Web Application->Name of the Web Application-> Click on OK.
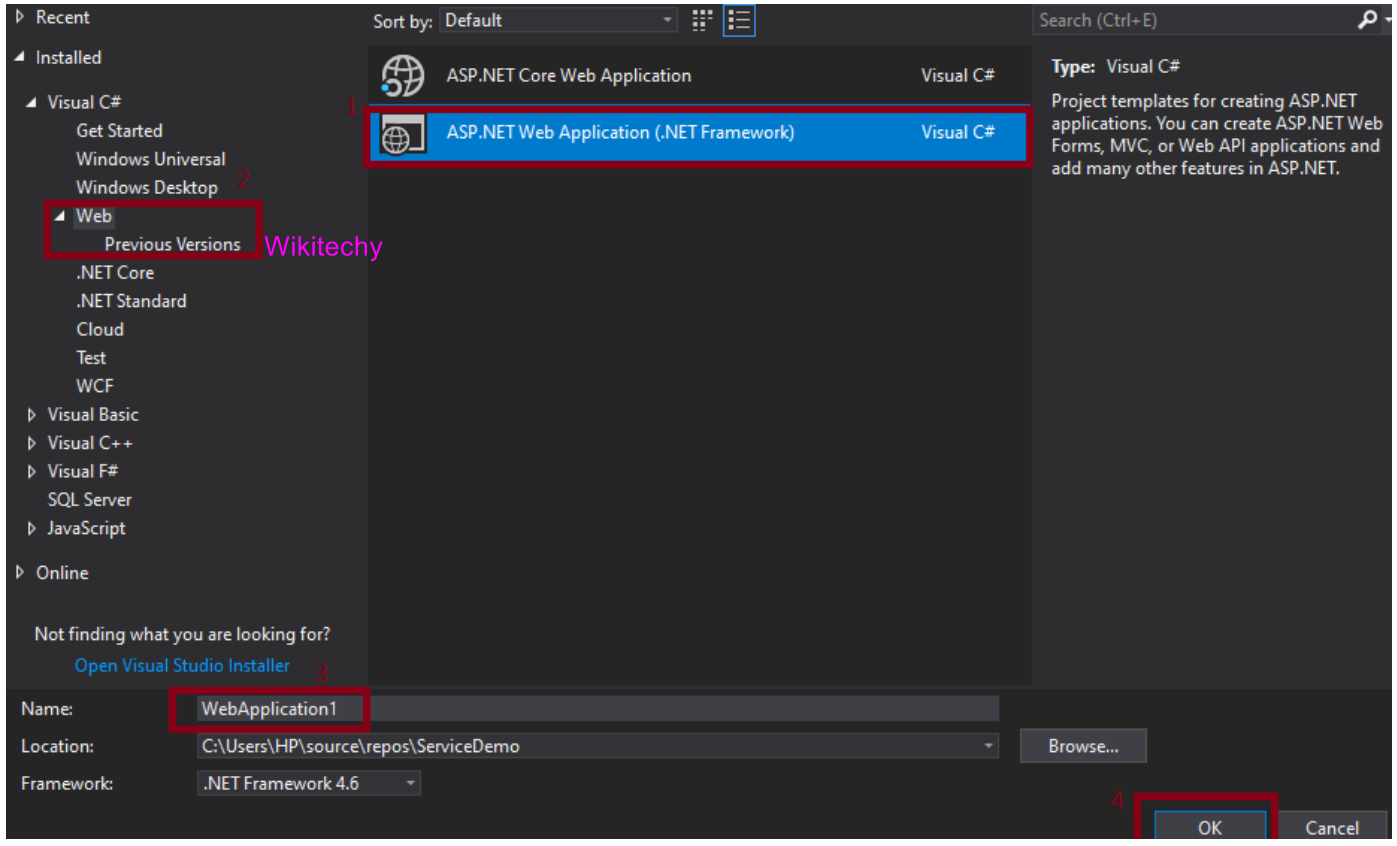
Step->3
- After this a new window will be shown us like as shown below
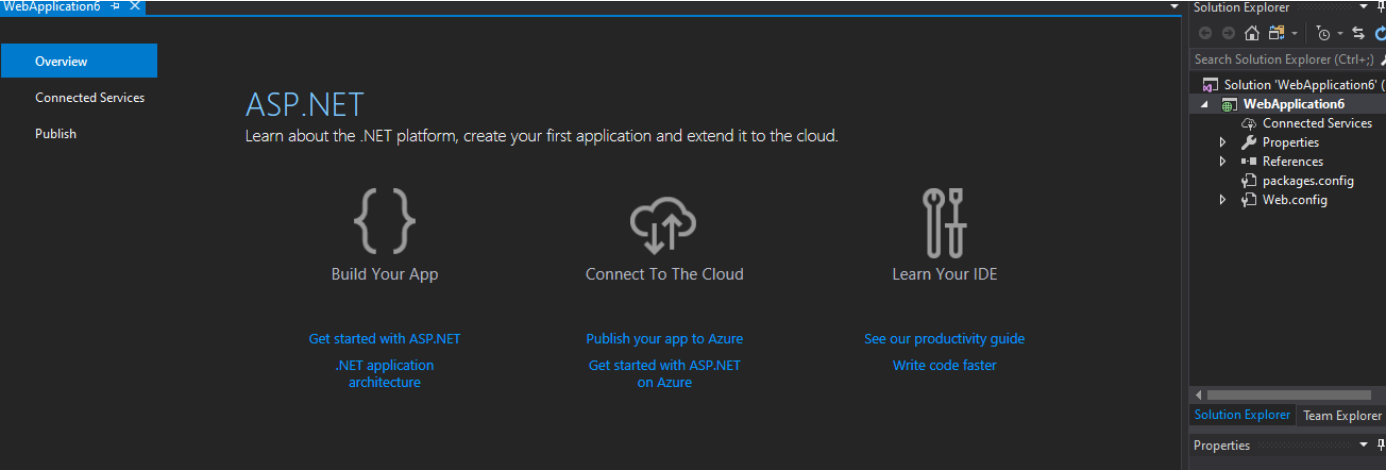
Step->4
- We will do the right click on the project name-> Click on Add-> Add New Item. As shown in the below screenshot:
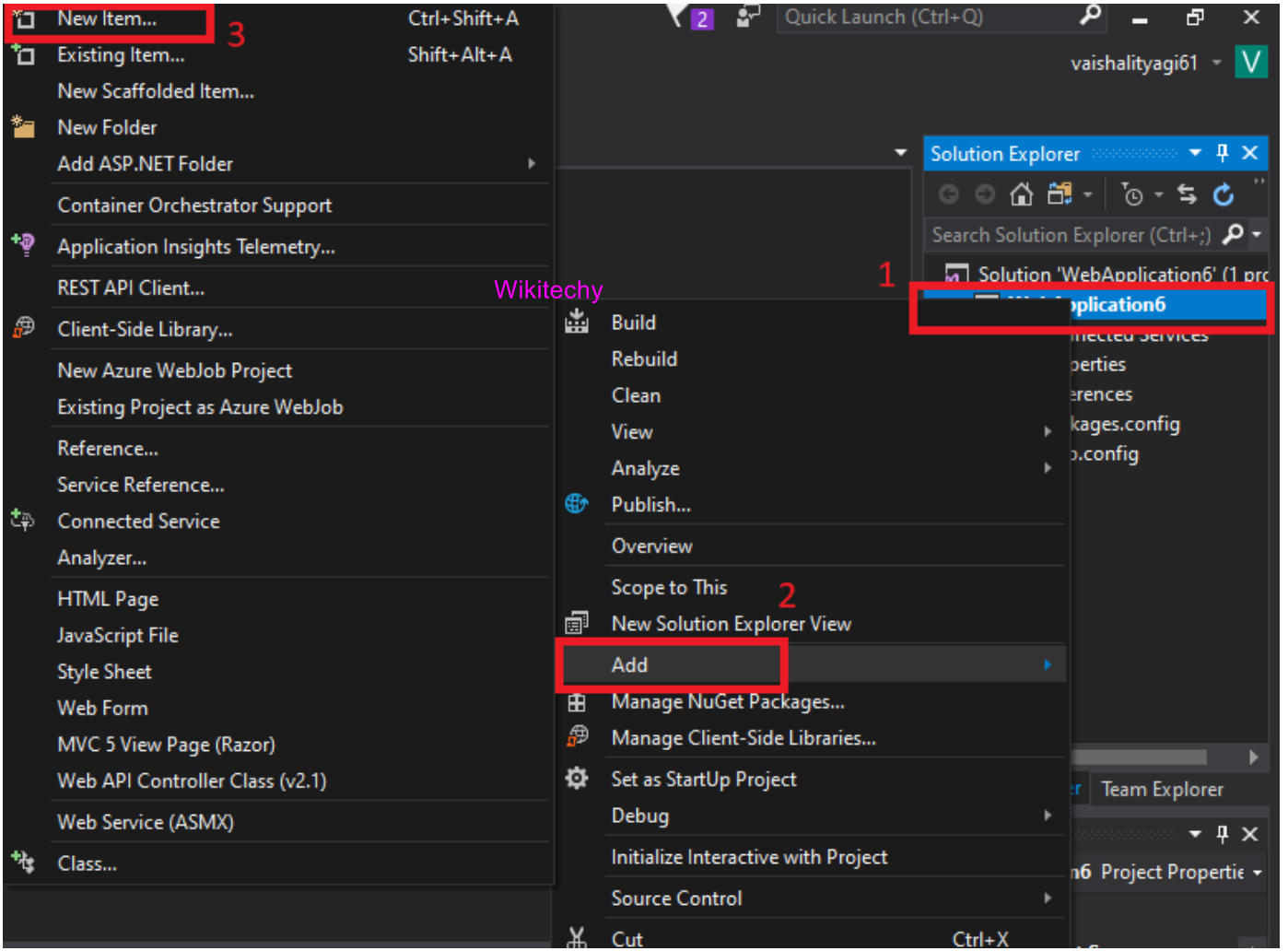
Step->5
- After this, a new window will appear. From where we have to click on the Web-> Select Web Service(.asmx page)-> Give a name to the Web Service as shown in the below screenshot:
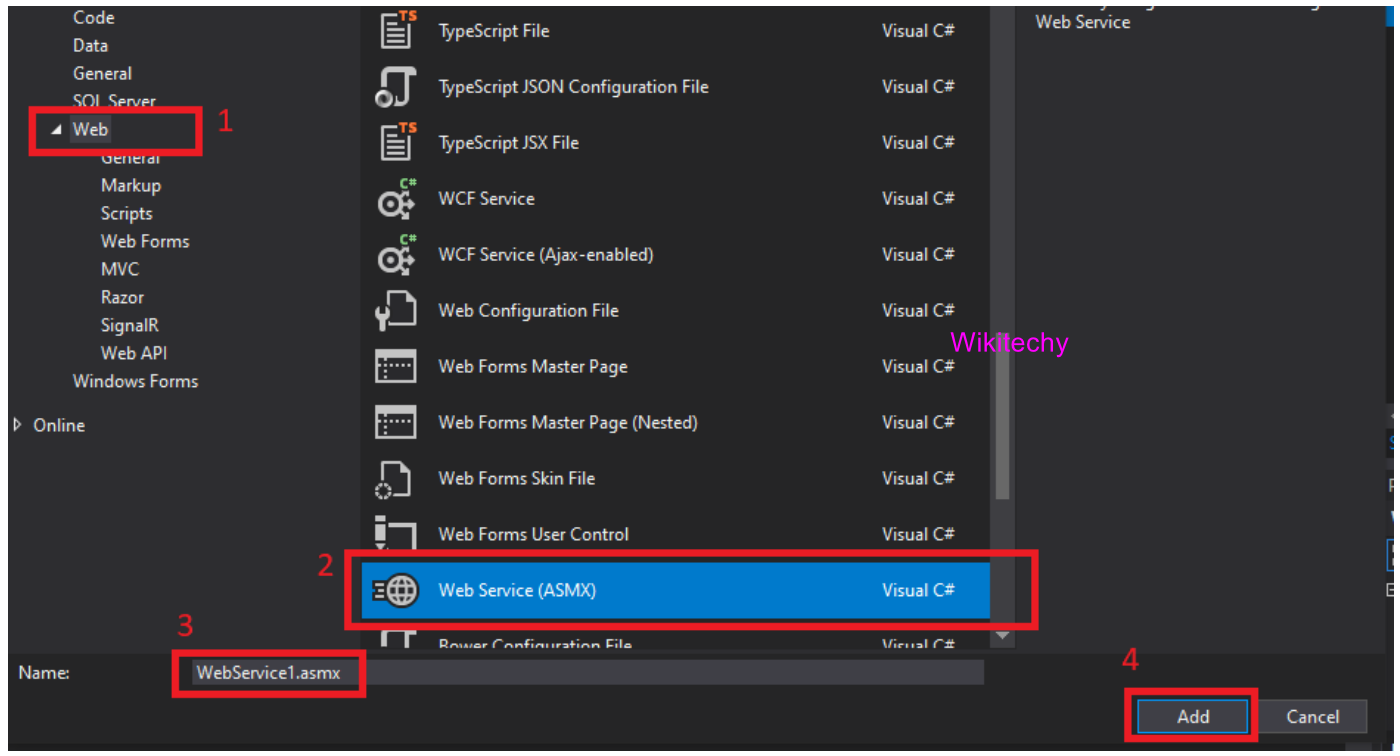
Step->6
- After adding the Web Service to the application, a new window will appear which is shown as below:
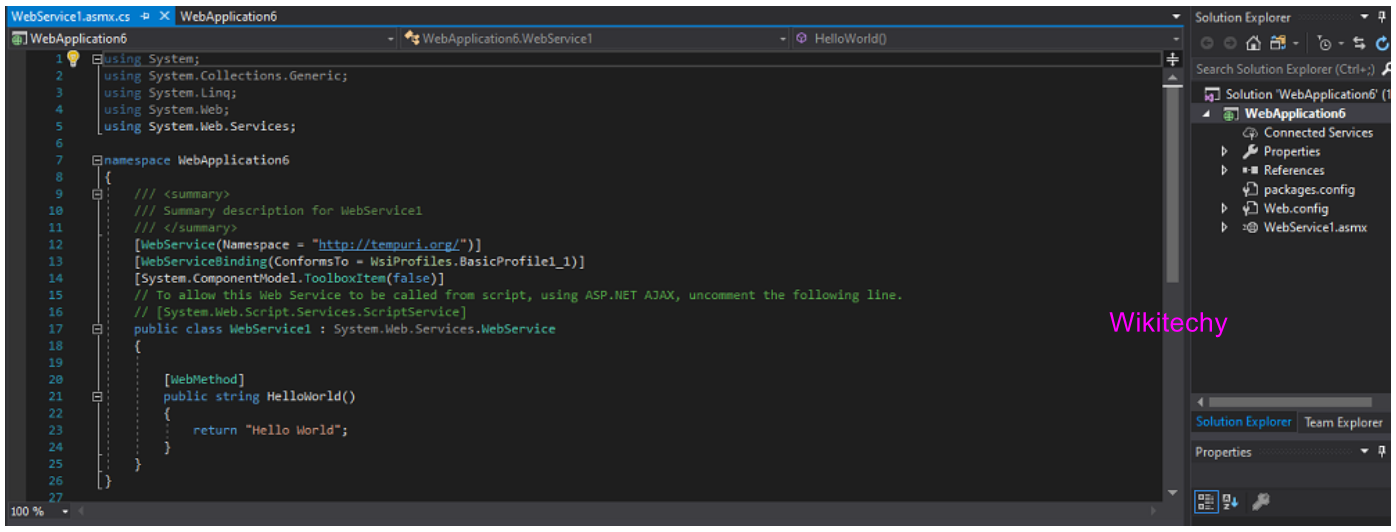
Consume the Web Service from the Client Script
- In any type of application, we can use web application and here we will create an application .Net web application.
Step->1
- Right-click on the Solution explorer->Add->New Project as shown in the below screenshot:
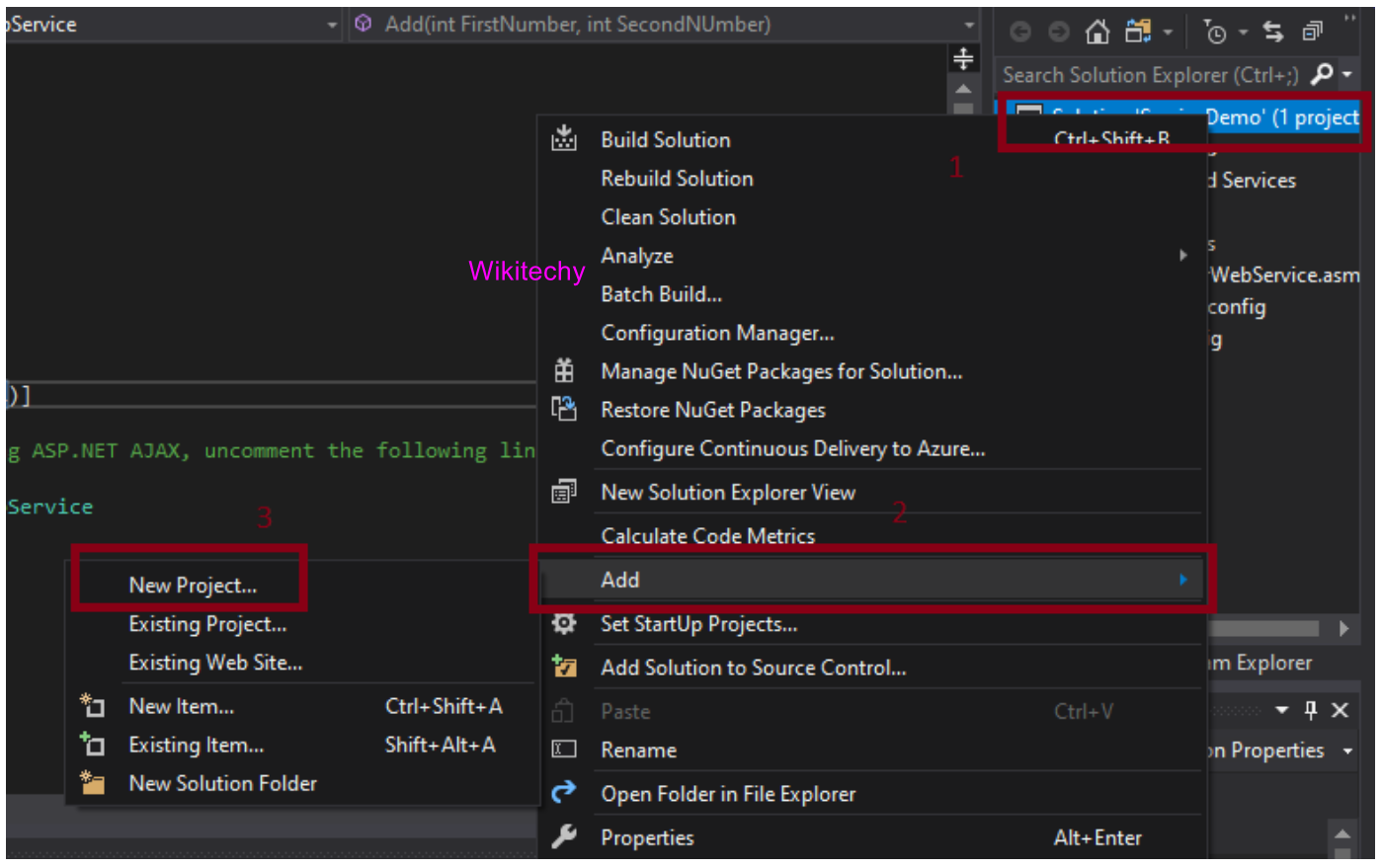
Step->2
- After clicking on the New Project, a new window will open as shown in the below screenshot:
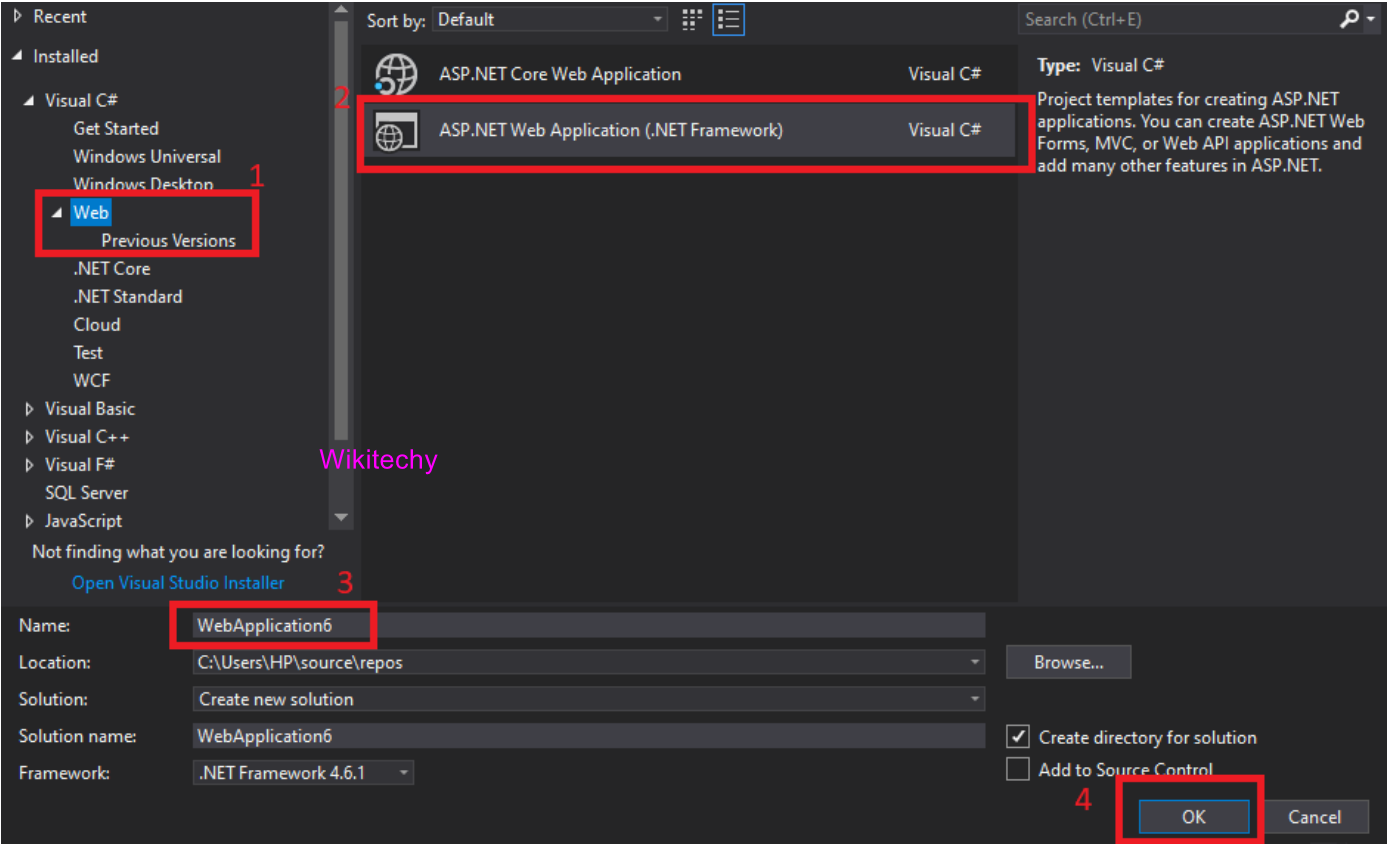
Step->3
- After this, to communicate with the web service, we have to create a proxy class. To create the proxy class, we have to follow the following steps:
Proxy Class
- To create the proxy class, we have to the right click on the References->Select Add Service Reference as shown in the below screenshot:
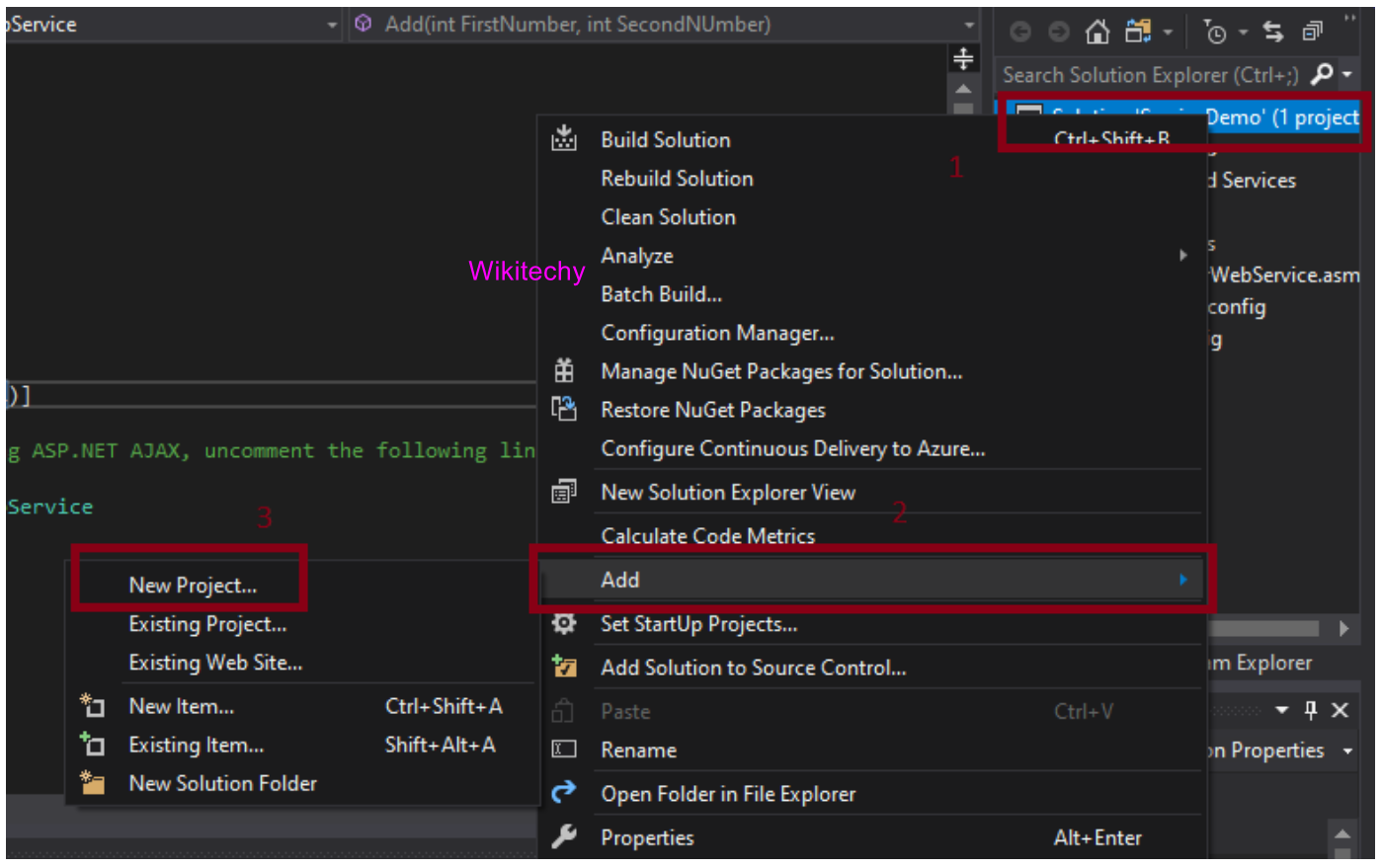
Step->4
- After clicking on the Add Service Reference, a new window will appear as shown in the below screenshot:
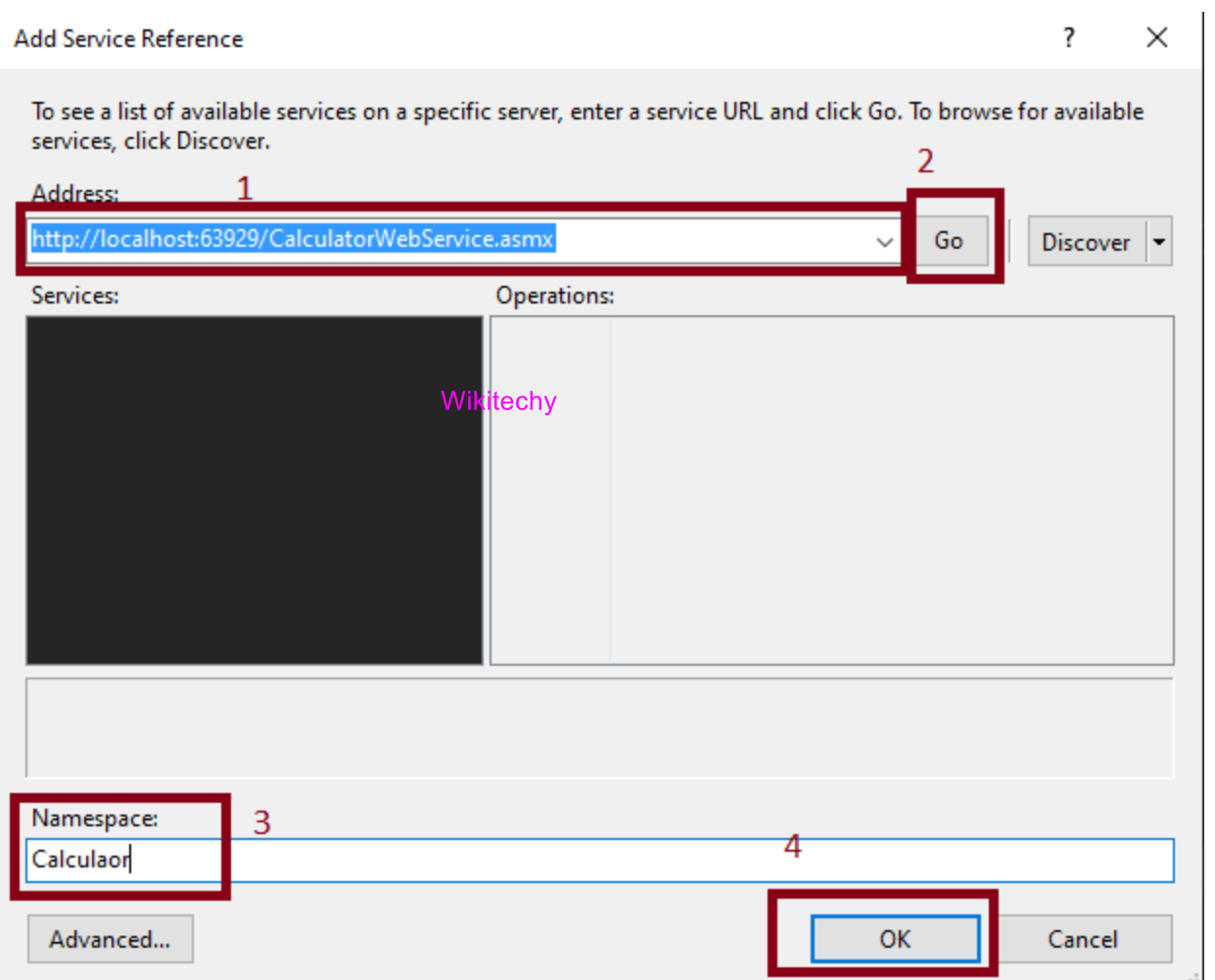
Implementing a web service
- As we can see here Visual Studio is unable to resolve “Service1” in the class property, since the class indicates a fully qualified name of the service and we renamed our Service1 class to MyService. So Visual Studio is unable to resolve it. So, now change the class property to “MyWebServiceDemo.MyService”.
- Change the “CodeBehind” property from “Service1.asmx.cs” to “MyService.asmx.cs” as we renamed the file also.
MyService.asmx
<%@ WebService Language="C#" CodeBehind="MyService.asmx.cs" Class="MyWebServiceDemo.MyService"%>
MyService.asmx.cs
using System.Web.Script.Serialization;
using System.Web.Services;
namespace MyWebServiceDemo
{
// Use "Namespace" attribute with an unique name,to make service uniquely discoverable
[WebService(Namespace = "http://tempuri.org/")]
// To indicate service confirms to "WsiProfiles.BasicProfile1_1" standard,
// if not, it will throw compile time error.
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
// To restrict this service from getting added as a custom tool to toolbox
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX
[System.Web.Script.Services.ScriptService]
public class MyService : WebService
{
[WebMethod]
public int SumOfNums(int First, int Second)
{
return First + Second;
}
}
}
Test a web service
- Let's run the project by hitting F5. The “http://localhost:56655/MyService.asmx” page will open that has a link for the Service description (the WSDL document, documentation for web service) another link for SumOfNums, which is for test page of SumOfNums method.
- Let's use method overloading of the OOP concept. Add the following WebMethod in MyService class:
[WebMethod]
public float SumOfNums(float First, float Second)
{
return First + Second;
}
- Hit F5 to run the application, you will get “Both Single SumOfNums(Single, Single) and Int32 SumOfNums(Int32, Int32) use the message name 'SumOfNums'.
- Use the MessageName property of the WebMethod custom attribute to specify unique message names for the methods” error message”.
- We just used method overloading concept, so why this error message? This is because these methods are not unique for a client application.
let's use the MessageName property of the WebMethod attribute as shown below:
[WebMethod (MessageName = "SumOfFloats")]
public float SumOfNums(float First, float Second)
{
return First + Second;
}
- Now, compile and run the application.
- Again it's showing some different error message “Service 'MyWebServiceDemo.
- MyService' does not conform to WS-I Basic Profile v1.1”.
- As, WsiProfiles.BasicProfile1_1 doesn't support method overloading we are getting this exception.
- Now, either remove this “[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]” attribute or make it “[WebServiceBinding(ConformsTo = WsiProfiles.None)]”.
For Example
using System.Web.Script.Serialization;
using System.Web.Services;
namespace MyWebServiceDemo
{
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.None)]
[System.ComponentModel.ToolboxItem(false)]
[System.Web.Script.Services.ScriptService]
public class MyService : WebService
{
[WebMethod]
public int SumOfNums(int First, int Second)
{
return First + Second;
}
[WebMethod(MessageName = "SumOfFloats")]
public float SumOfNums(float First, float Second)
{
return First + Second;
}
}
}
The WSDL document
- Web Services are self-describing, that means ASP.NET automatically provides all the information the client needs to consume a service as a WSDL document.
- The WSDL document tells a client what methods are present in a web service, what parameters and return values each method uses and how to communicate with them. It is an XML standard.
Host a Web Service
- Since we will add a reference to this service and consume it from various applications and the port number is supposed to change, let's host this service on IIS-Internet Information Server to have a specific address of a service.
- Open IIS, go to the default web sites node then select Add Application then provide an alias name (I gave it WebServiceForBlog) then browse to the physical location of your service for the physical path field then click “OK”.
- We can now browse with an alias name (like http://localhost/WebServiceForBlog/) to test if the application was hosted properly.
- We will get a “HTTP Error 403.14 – Forbidden” error since there is no default document set for this application.
- Add a “MyService.asmx” page as a default document.
- Now we can browse our service.
How to access a web service from a client application
MyService.asmx
<%@ WebService Language="C#" CodeBehind="MyService.asmx.cs" Class="MyWebServiceDemo.MyService" %>
MyService.asmx.cs
using System.Web.Script.Serialization;
using System.Web.Services;
namespace MyWebServiceDemo
{
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.None)]
[System.ComponentModel.ToolboxItem(false)]
[System.Web.Script.Services.ScriptService]
public class MyService
{
// Takes 2 int values & returns their summation
[WebMethod]
public int SumOfNums(int First, int Second)
{
return First + Second;
}
// Takes a stringified JSON object & returns an object of SumClass
[WebMethod(MessageName = "GetSumThroughObject")]
public SumClass SumOfNums(string JsonStr)
{
var ObjSerializer = new JavaScriptSerializer();
var ObjSumClass = ObjSerializer.Deserialize<SumClass>(JsonStr);
return new SumClass().GetSumClass(ObjSumClass.First, ObjSumClass.Second);
}
}
// Normal class, an instance of which will be returned by service
public class SumClass
{
public int First, Second, Sum;
public SumClass GetSumClass(int Num1, int Num2)
{
var ObjSum = new SumClass
{
Sum = Num1 + Num2,
};
return ObjSum;
}
}
}
- Compile this application, Since we will consume this service from other applications we need a fixed address for this service, the port numbers are supposed to vary.So, host the web service on IIS (refer to the previous article for hosting the web service).
- I hosted it with the virtual directory “WebServiceForBlog”.
How to consume a web service
<table>
<tr>
<td>First Number:</td>
<td><input type="text" id="Text1" runat="server" /></td>
</tr>
<tr>
<td>Second Number:</td>
<td><input type="text" id="Text2" runat="server" /></td>
</tr>
<tr>
<td><input type="button" onserverclick="AddNumber" value="Add" runat="server" /></td>
<td><div id="divSum" runat="server"></div>
<div id="divSumThroughJson" runat="server"></div></td>
</tr>
</table>
Home.aspx.cs
using System;
using ServiceConsumer.MyServiceReference;
namespace ServiceConsumer
{
public partial class Home : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{}
protected void AddNumber(object Sender, EventArgs E)
{
int Num1, Num2;
int.TryParse(txtFirstNum.Value, out Num1);
int.TryParse(txtSecondNum.Value, out Num2);
// creating object of MyService proxy class
var ObjMyService = new MyServiceSoapClient();
// Invoke service method through service proxy
divSum.InnerHtml = ObjMyService.SumOfNums(Num1, Num2).ToString();
var ObjSumClass = new SumClass { First = Num1, Second = Num2 };
var ObjSerializer = new JavaScriptSerializer();
var JsonStr = ObjSerializer.Serialize(ObjSumClass);
divSumThroughJson.InnerHtml =ObjMyServiceProxy.GetSumThroughObject(JsonStr).Sum.ToString();
}
}
}
How to consume a web service from a client script
- Add another project to your solution and named it “Consume Service from Client Script”.
Creating a JavaScript proxy
- JavaScript proxies can be automatically generated by using the ASP.NET AJAX ScriptManager control's Services property.
- We can define one or more services that a page can call asynchronously to send or receive data using the ASP.NET AJAX “ServiceReference” control and assigning the Web Service URL to the control's “Path” property.
Add a ScriptManager control like the following inside the form tag:
<asp:ScriptManager runat="server">
<Services>
<asp:ServiceReference Path="http://localhost/WebServiceForBlog/MyService.asmx"/>
</Services>
</asp:ScriptManager>
- Add an empty Web form to your project. I named it “Default.aspx”:
<body>
<form id="form1" runat="server">
<asp:ScriptManager runat="server">
<Services>
<asp:ServiceReference Path="http://localhost/WebServiceForBlog/MyService.asmx"/>
</Services>
</asp:ScriptManager>
<div>
<table>
<tr>
<td>First Number:</td>
<td><input type="text" id="txtFirstNum" /></td>
</tr>
<tr>
<td>Second Number:</td>
<td><input type="text" id="txtSecondNum" /></td>
</tr>
<tr>
<td><input type="button" onclick="AddNumber();" value="Add" /></td>
<td><div id="divSum1"></div><div id="divSum2"></div><div id="divSum3"></div><div id="divSum4"></div><div id="divSum5"></div></td>
</tr>
</table>
</div>
</form>
<script type="text/javascript" src="Scripts/jquery-1.7.1.js"></script>
<script type="text/javascript" src="Scripts/json2.js"></script>
<script type="text/javascript">
function AddNumber() {
var FirstNum = $('#txtFirstNum').val();
var SecondNum = $('#txtSecondNum').val();
$.ajax({
type: "POST",
url: "http://localhost/WebServiceForBlog/MyService.asmx/SumOfNums",
data: "First=" + FirstNum + "&Second=" + SecondNum,
// the data in form-encoded format, it would appear on a querystring
//contentType: "application/x-www-form-urlencoded; //charset=UTF-8"
//form encoding is the default one
dataType: "text",
crossDomain: true,
success: function (data) {
$('#divSum1').html(data);
}
});
// i/p in JSON format, response as JSON object
$.ajax({
type: "POST",
url: "http://localhost/WebServiceForBlog/MyService.asmx/SumOfNums",
data: "{First:" + FirstNum + ",Second:" + SecondNum + "}",
contentType:"application/json; charset=utf-8",// What I am ing
dataType: "json", // What I am expecting
crossDomain: true,
success: function (data) {
$('#divSum2').html(data.d);
}
});
// i/p as JSON object, response as plain text
$.ajax({
type: "POST",
url: "http://localhost/WebServiceForBlog/MyService.asmx/SumOfNums",
data: { First: FirstNum, Second: SecondNum },
contentType: "application/x-www-form-urlencoded; charset=UTF-8",
crossDomain: true,
dataType: "text",
success: function (data) {
$("#divSum3").html(data);
}
});
// Url encoded stringified JSON object as I/p & text response
var ObjSum = new Object();
ObjSum.First = FirstNum;
ObjSum.Second = SecondNum;
$.ajax({
type: "POST",
url: "http://localhost/WebServiceForBlog/MyService.asmx/GetSumThroughObject",
data: "JsonStr=" + JSON.stringify(ObjSum),
dataType: "text",
crossDomain: true,
success: function (data) {
$('#divSum4').html($(data).find('Sum').text());
}
});
// Call SOAP-XML web services directly
var SoapMessage = '<soap:Envelope xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/"> \
<soap:Body> \
<SumOfNums xmlns="http://tempuri.org/"> \
<First>'+FirstNum+'</First> \
<Second>'+SecondNum+'</Second> \
</SumOfNums> \
</soap:Body> \
</soap:Envelope>';
$.ajax({
url: "http://localhost/WebServiceForBlog/MyService.asmx?op=SumOfNums",
type: "POST",
dataType: "xml",
data: SoapMessage,
complete: ShowResult,
contentType: "text/xml; charset=utf-8"
});
}
function ShowResult(xmlHttpRequest, status) {
var SoapResponse = $(xmlHttpRequest.responseXML).find('SumOfNumsResult').text();
$('#divSum5').html(SoapResponse);
}
</script>
</body>
Call SOAP-XML Web Services directly
- In order to call the web service, we need to supply an XML message that matches the operation definition specified by the web service's WSDL.
- In the test page “http://localhost/WebServiceForBlog/MyService.asmx?op=SumOfNums” we can see a sample of SOAP 1.1/1.2 request and response format.
- From which we can get the operations schema.
- Now, we just need to exchange the type names for the operation's parameters, with their actual values.
- Copy the SOAP envelope to the Default.aspx page and replace the place holders (type names) with an actual value.
- A sample for this type of call is included in the code snippet above.
- The variable “SoapMessage” in the preceding example contains the complete XML message that we're going to send to the web service.
- On completion of the ajax request, we will call a call back method named “ShowResult”.
For Example
<configuration>
<system.web>
<webServices>
<protocols>
<!-- To disable SOAP 1.2 -->
<remove name="HttpSoap12"/>
<!-- To disable SOAP 1.1 -->
<remove name="HttpSoap"/>
</protocols>
</webServices>
</system.web>
</configuration>