C# array functions | C# array to string | C# Passing Array to Function - c# - c# tutorial - c# net
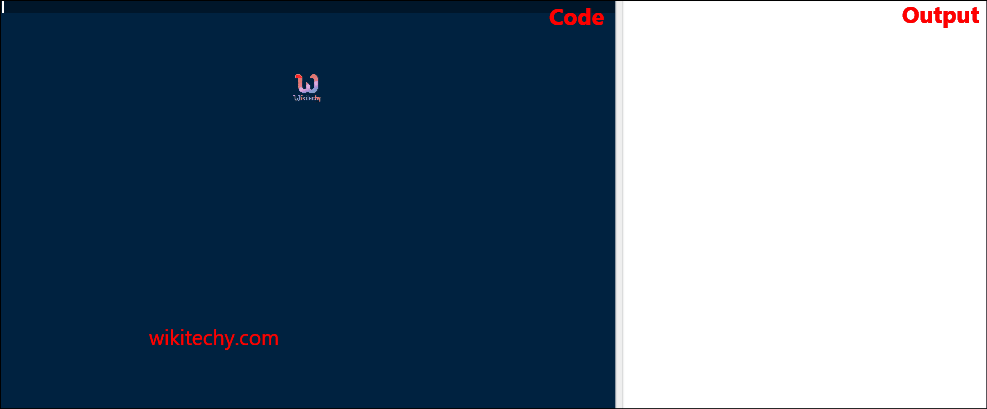
Learn c# - c# tutorial - C# Array Functions - c# examples - c# programs
How to passing array to function in C# ?
- In C#, to reuse the array logic, we can create function.
- To pass array to function in C#, we need to provide only array name.
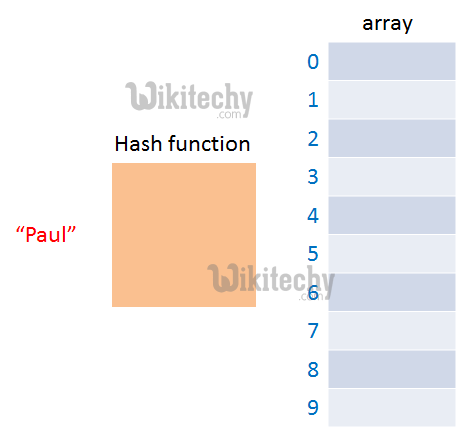
Passing Array Function
functionname(arrayname);//passing array
C# Passing Array to Function Example: print array elements
- Let's see an example of C# function which prints the array elements.
using System;
public class ArrayExample
{
static void printArray(int[] arr)
{
Console.WriteLine("Printing array elements:");
for (inti = 1; i<arr.Length; i++)
{
Console.WriteLine(arr[i]);
}
}
public static void Main(string[] args)
{
int[] arr1 = { 25, 10, 20, 15, 40, 50 };
int[] arr2 = { 12, 23, 44, 11, 54 };
printArray(arr1);//passing array to function
printArray(arr2);
}
}
C# examples - Output :
Printing array elements:
25
10
20
15
40
50
Printing array elements:
12
23
44
11
54
C# Passing Array to Function Example: Print minimum number
- Let's see an example of C# array which prints minimum number in an array using function.
using System;
public class ArrayExample
{
static void printMin(int[] arr)
{
int min = arr[0];
for (inti = 1; i<arr.Length; i++)
{
if (min >arr[i])
{
min = arr[i];
}
}
Console.WriteLine("Minimum element is: " + min);
}
public static void Main(string[] args)
{
int[] arr1 = { 25, 10, 20, 15, 40, 50 };
int[] arr2 = { 12, 23, 44, 11, 54 };
printMin(arr1);//passing array to function
printMin(arr2);
}
}
C# examples - Output :
Minimum element is: 10
Minimum element is: 11
C# Passing Array to Function Example: Print maximum number
- Let's see an example of C# array which prints maximum number in an array using function.
using System;
public class ArrayExample
{
static void printMax(int[] arr)
{
int max = arr[0];
for (inti = 1; i<arr.Length; i++)
{
if (max <arr[i])
{
max = arr[i];
}
}
Console.WriteLine("Maximum element is: " + max);
}
public static void Main(string[] args)
{
int[] arr1 = { 25, 10, 20, 15, 40, 50 };
int[] arr2 = { 12, 23, 64, 11, 54 };
printMax(arr1);//passing array to function
printMax(arr2);
}
}
C# examples - Output :
Maximum element is: 50
Maximum element is: 64