C# List Sort - c# - c# tutorial - c# net
What is C# List Sort ?
- C# SortedList,< TKey, TValue> is an array of key/value pairs.
- It stores values on the basis of key. The SortedList<TKey, TValue>class contains unique keys and maintains ascending order on the basis of key.
- By the help of key, we can easily search or remove elements. It is found in System.Collections.Generic namespace.
- It is like SortedDictionary<TKey, TValue> class.
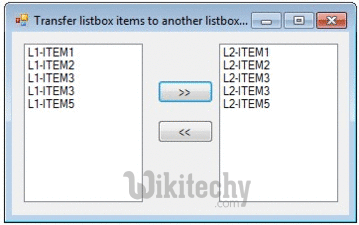
List
Constructors:
Name | Description |
---|---|
SortedList<TKey, TValue>() | Initializes a new instance of the SortedList<TKey, TValue> class that is empty, has the default initial capacity, and uses the default IComparer<T>. |
SortedList<TKey, TValue>(IComparer<TKey>) | Initializes a new instance of the SortedList<TKey, TValue> class that is empty, has the default initial capacity, and uses the specified IComparer<T>. |
SortedList<TKey, TValue>(IDictionary<TKey, TValue>) | Initializes a new instance of the SortedList<TKey, TValue> class that contains elements copied from the specified IDictionary<TKey, TValue>, has sufficient capacity to accommodate the number of elements copied, and uses the default IComparer<T>. |
SortedList<TKey, TValue> (IDictionary<TKey, TValue>, IComparer<TKey>) |
Initializes a new instance of the SortedList<TKey, TValue> class that contains elements copied from the specified IDictionary<TKey, TValue>, >br>has sufficient capacity to accommodate the number of elements copied, and uses the specified IComparer<T>. |
SortedList<TKey, TValue>(Int32) | Initializes a new instance of the SortedList<TKey, TValue> class that is empty, has the specified initial capacity, and uses the default IComparer<T>. |
SortedList<TKey, TValue>(Int32, IComparer<TKey>) | Initializes a new instance of the SortedList<TKey, TValue> class that is empty, has the specified initial capacity, and uses the specified IComparer<T>. |
Properties:
Name | Description |
---|---|
Capacity | Gets or sets the number of elements that the SortedList<TKey, TValue> can contain. |
Comparer | Gets the IComparer<T> for the sorted list |
Count | Gets the number of key/value pairs contained in the SortedList<TKey, TValue>. |
Item[TKey] | Gets or sets the value associated with the specified key. |
Keys | Gets a collection containing the keys in the SortedList<TKey, TValue>, in sorted order.. |
Values | Gets a collection containing the values in the SortedList<TKey, TValue>. |
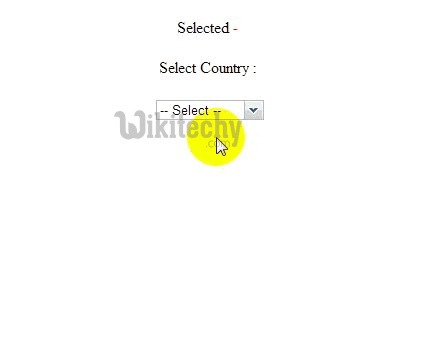
Dictionary in csharp
C# SortedList<TKey, TValue> vs SortedDictionary<TKey, TValue>
- SortedList<TKey, TValue> class uses less memory than SortedDictionary<TKey, TValue>.
- It is recommended to use SortedList<TKey, TValue> if you have to store and retrieve key/valye pairs.
- The SortedDictionary<TKey, TValue>class is faster than SortedList<TKey, TValue> class if you perform insertion and removal for unsorted data.
C# SortedList<TKey, TValue> Example:
- Let's see an example of generic SortedList<TKey, TValue> class that stores elements using Add() method and iterates elements using for-each loop.
- Here, we are using KeyValuePair class to get key and value.
using System;
using System.Collections.Generic;
public class SortedDictionaryExample
{
public static void Main(string[] args)
{
SortedList<string, string> names = new SortedList<string, string>();
names.Add("1","Sonoo");
names.Add("4","Peter");
names.Add("5","James");
names.Add("3","Ratan");
names.Add("2","Irfan");
foreach (KeyValuePair<string, string> kv in names)
{
Console.WriteLine(kv.Key+" "+kv.Value);
}
}
}
C# examples - Output :
1 Sonoo
2 Irfan
3 Ratan
4 Peter
5 James