C# Access database | Access database c# - c# - c# tutorial - c# net
How to Display data in datagridview from access database ?
- In C#, DataGridView control is one of the coolest features of Dot Net Framework.
- The DataGridView control supports the standard Windows Forms data binding model, so it will bind to a variety of data sources.
- In most conditions, however, we will fix to a BindingSource component which we will manage to the details of interacting with the data source.
- The BindingSource component can represents an any Windows Forms data source and it gives to great flexibility when choosing or modifying the location of our data.
- Here in the below table is representing to Windows Forms:
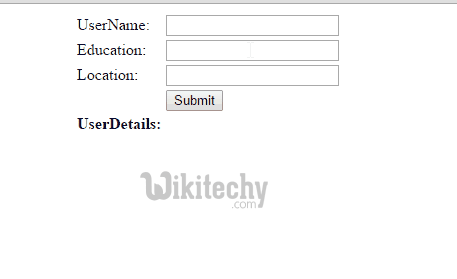
Access Database
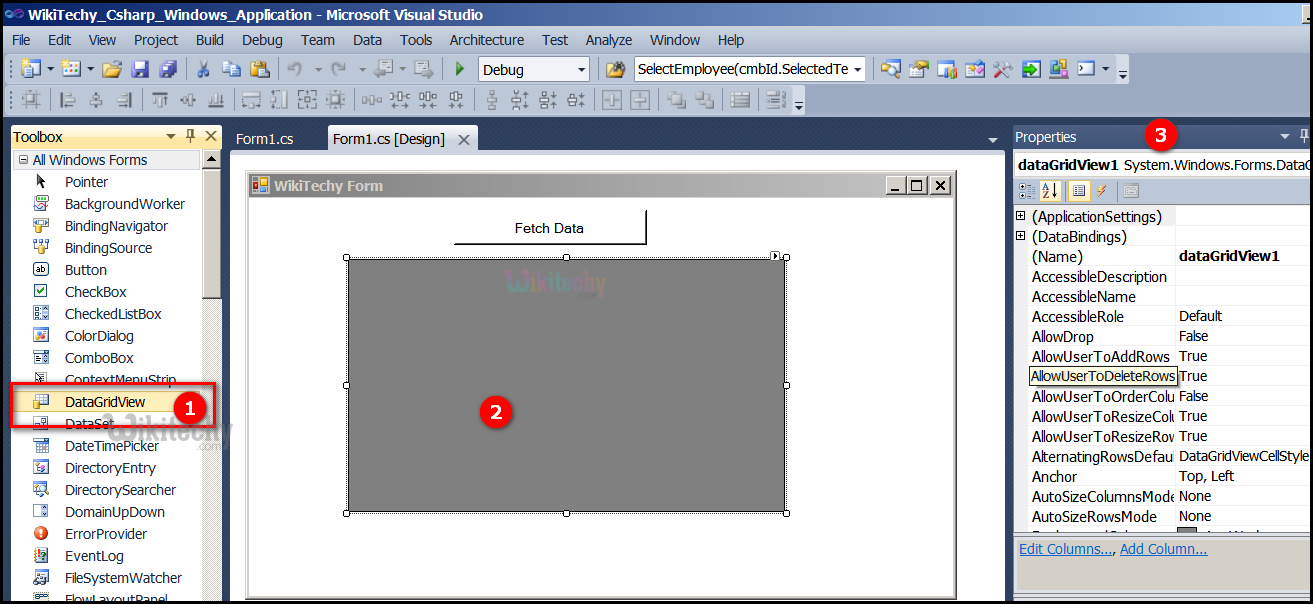
- The DataGridview specifies to displays data from SQL databases.
- Go to tool box and click on the DataGridview option the form will be open.
- Here the Properties window, specifies to properties for the form. Forms have various properties. For example, we can set the foreground and background color, title text that appears at the top of the form, size of the form, and other properties.
C# Sample Code - C# Examples:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
{
private void button1_Click(object sender, EventArgs e)
{
string connectionString =
@"Data Source=VENKAT\KAASHIV;Initial Catalog=wikitechy; user id = venkat;
password=venkat";
string sql = "SELECT * FROM login_table";
SqlConnection connection = new SqlConnection(connectionString);
SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection);
DataSet ds = new DataSet();
connection.Open();
dataadapter.Fill(ds);
connection.Close();
dataGridView1.DataSource = ds.Tables[0];
}
}
}
Read Also
dot net course qualification , dotnet institute in chennai , summer internship with stipendCode Explanation:
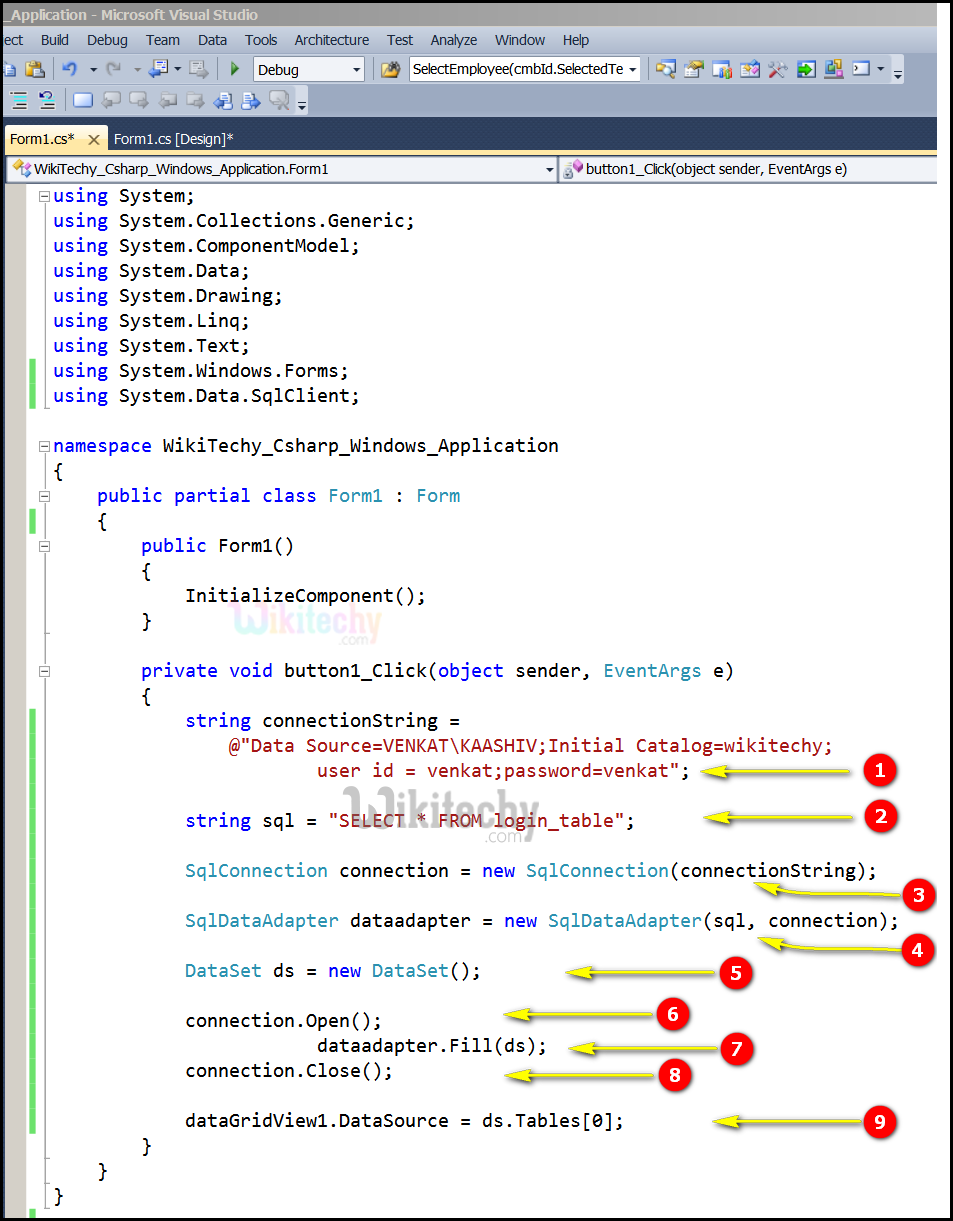
- Here string connectionString = @"Data Source=VENKAT\KAASHIV;Initial Catalog=wikitechy; user id = venkat;password=venkat"; connection string specifies that includes the source database name here the name is VENKAT\KAASHIV, and other parameters needed to establish the initial catalog connection is wikitechy. The default value is user id and password is venkat.
- Here in this example string sql = "SELECT * FROM login_table"; specifies to select the login_table in SQL server.
- Here SqlConnection connection = new SqlConnection (connectionString); this statement is used to acquire the SqlConnection as a resource.
- Here in this example SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection); specifies to initializes a new instance of the SqlDataAdapter class with a SelectCommand (sql) and a SqlConnection object(connection).
- Here DataSet ds = new DataSet(); specifies to Create a new instance of the DataSet class. The DataSet Object represents a complete set of data, including related tables, constraints, and relationships among the tables.
- Here in this example, connection.Open(); specifies to Open the SqlConnection.
- Here dataadapter.Fill(ds); specifies to fetches the data from User and fills in the DataSet ds.
- Here in this example connection.Close(); it is used to close the Database Connection.
- Here dataGridView1.DataSource = ds.Tables[0]; is used to specify the data source of datagridview and the table which will be bind to. When we run the code a window will show with a datagridview that containing all the returning rows.
Sample C# examples - Output :
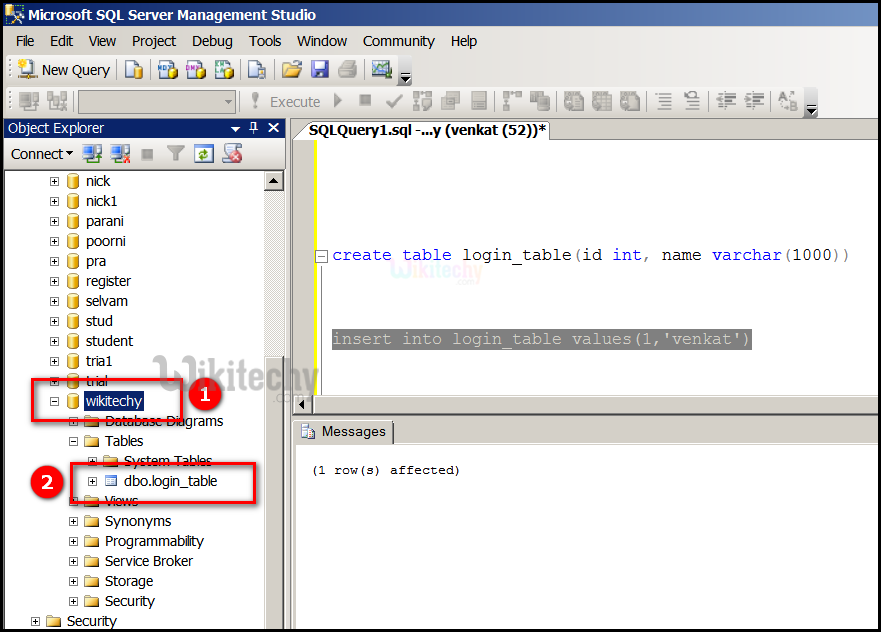
- Here in this output we display wikitechy which specifies to database name.
- Here in this output we display dbo.login_table which specifies to table name of the database. In that name of wikitechy database we created a table name as login_table.
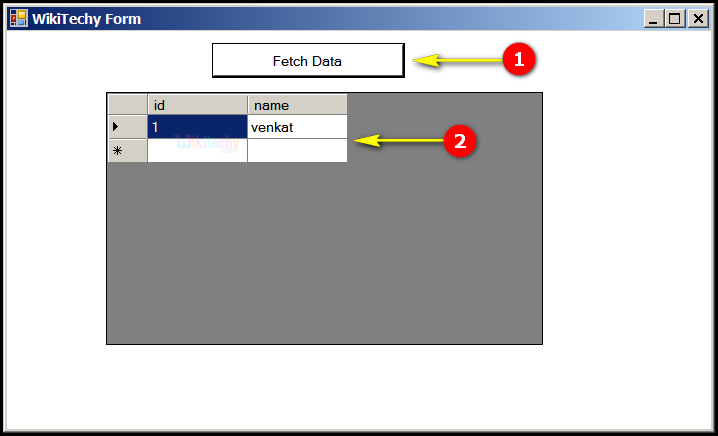
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the Id "1" and name "venkat".