C# Dictionary - c# - c# tutorial - c# net
What is dictionary in C# ?
- C# Dictionary<TKey, TValue> class uses the concept of hashtable.
- It stores values on the basis of key.
- It contains unique keys only.
- By the help of key, we can easily search or remove elements.
- It is found in System.Collections.Generic namespace.
Syntax:
c# dictionary sample
Parameters :
- TKey - The type of the keys in the dictionary.
- TValue - The type of the values in the dictionary
Example
Dictionary<string, string>
Dictionary<string, int>
Adding Values to Dictionary:
- Add method in Dictionary takes two parameters, one for the key and one for the value.
Syntax:
public void Add(TKey key,TValue value)
Example
dictionary.Add("dozen",12);
- Key in a Dictionary should not be null, but a value can be, if TValue is a reference type.
Dictionary<string, int> dict = new Dictionary<string, int>();
dict.Add("one", 1);
dict.Add("two", 2);
dict.Add("three", 3);
dict.Add("four", 4);
Retrieve Key-Value pair from Dictionary:
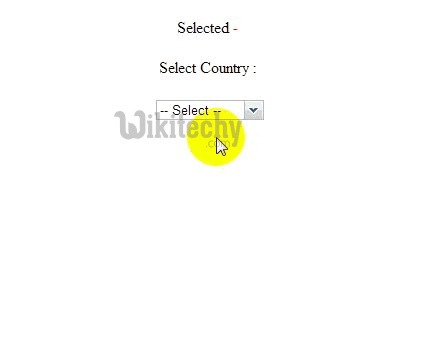
dictionary in csharp
- We can retrieve values from Dictionary using foreach loop
foreach (KeyValuePair<string, int> pair in dict)
{
MessageBox.Show(pair.Key.ToString ()+ " - " + pair.Value.ToString () );
}
C# Dictionary<TKey, TValue> example:
- Let's see an example of generic Dictionary<TKey, TValue> class that stores elements using Add() method and iterates elements using for-each loop.
- Here, we are using KeyValuePair class to get key and value
using System;
using System.Collections.Generic;
public class DictionaryExample
{
public static void Main(string[] args)
{
Dictionary<string, string> names = new Dictionary<string, string>();
names.Add("1","Sonoo");
names.Add("2","Peter");
names.Add("3","James");
names.Add("4","Ratan");
names.Add("5","Irfan");
foreach (KeyValuePair<string, string> kv in names)
{
Console.WriteLine(kv.Key+" "+kv.Value);
}
}
}
C# examples - Output :
1 Sonoo
2 Peter
3 James
4 Ratan
5 Irfan