C# Readline Method - Console.ReadLine() Method in C#
C# Readline Method
- In C# Console.Writeline () is a method used to print the entire statement of a single line and transfer control to the next line of the console.
- Equally to the Console.WriteLine(), the Read Line () method is used to read the entire line of string or statement value from the user until the Enter key is pressed to transfer control to the next line.
Console.ReadLine() Method
- In C#, Console. ReadiLine () method is a commonly used method or function to take an input from the user until the enter key is pressed.
- It is a method that reads each line of string or values from a standard input stream.
- In C#, it is a predefined method of the Console class (System Namespace).
- This method reads and only returns the string from the stream output device (console) until a newline character is found.
- When we want to read a character or numeric value from the user, we need to convert the string to the appropriate data sets.
Syntax
public static string ReadLine ();
Sample Code
using System; // Define the System package
namespace ConsoleApp3 // Project name or Folder
{
class Program
{
static void Main(string[] args) // Defining the main function
{
string name; // string variable name
Console.WriteLine("Hello, what is your name?");
name = Console.ReadLine(); // takes an input from the user
Console.WriteLine("Hi! "+ name + " Welcome to the Wikitechy");
// print the output
}
}
}
Output
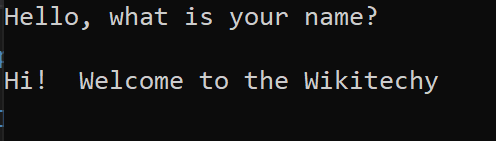
Example
- Write a program to print the user's first and last name using the Read Line () function in C#.
Sample Code
using System;
namespace ConsoleApp3
{
class Program2
{
static void Main(string[] args)
{
string fname, lname; // string variables
Console.Write("Please, Enter your first Name : ");
fname = Console.ReadLine(); // takes the first name from the user
// ReadLine() is a method of Console class to read a line from the standard input stream
Console.Write("Please, Enter Your Last Name : ");
lname = Console.ReadLine(); // takes the second name from the user
Console.WriteLine("Your Full Name is : " + fname + " " + lname);
}
}
}
Output

Read () Method
- In C#, Read () method is used to read a single character from the user and it is different from the Read Line () method because the read Line () method receives each line input from the user until the line is finished and control transfer to the next statement to read strings.
using System; // Define the System package
namespace ConsoleApp3
{
class Program4
{
static void Main(string[] args)
{
char ch;
Console.Write("Enter the characters "); // Cosole.Write() print the same line statement.
ch = Convert.ToChar(Console.Read()); // Read a single character from the user.
Console.WriteLine("You have entered the character " + ch); //print the complete line
}
}
}
Output

Read Key ()
- In C# Read Key () method is used to get the next character, or the user presses any key to exit the program.
- It holds the screen until the user presses any key from the keyboard and pressed key will be displayed on the console.
Sample Code
using System; // Define the System package
namespace ConsoleApp3
{
class Program5
{
static void Main(string[] args)
{
DateTime dt = DateTime.Now; // DateTime.Now() print the current time
Console.WriteLine(" The Current Date and Time is : " + dt);
Console.Write("Press any key or Enter to exit from the Console Screen");
Console.ReadKey(); // enter any key to exit from the console screen.
}
}
}
Output
