C# Filter Datagridview | C# Controls Datagridview Filter Data - c# - c# tutorial - c# net
What is Datagridview ?
- The DataGridView control is designed to be a complete solution for displaying tabular data with Windows Forms.
- The DataGridView control is highly configurable and extensible, and it provides many properties, methods, and events to customize its appearance and behavior.
- The DataGridView control provides TextBox, CheckBox, Image, Button, ComboBox and Link columns with the corresponding cell types.
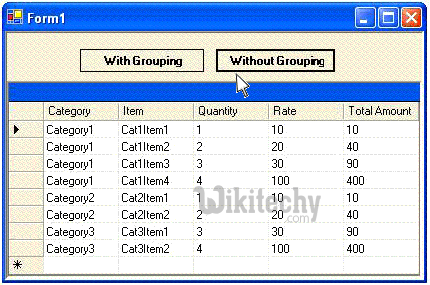
DataGridview Filter
Filter Data:
- In C#, the data filtering function support is built in the Data Grid Control.
- It allows users process data filtering in any columns.
- When a user’s click on the filter cell, the cell editor will be open and will allow users set the filter condition.
- In filter cell, when we clicking on the filter icon a menu will be open and provide filter conditions including greater than (>) contains, less than (<), equals to (=), and extra.
- Developers can also modify the filtering features in C# code in Windows Forms projects.
- Here in the below table is representing to Windows Forms:
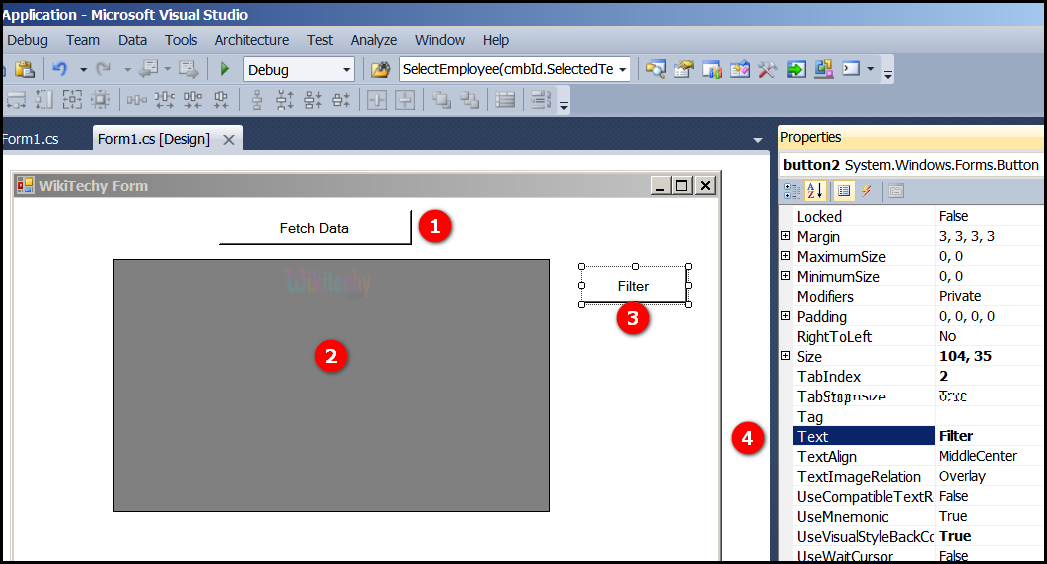
- Here Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Go to tool box and click on the DataGridview option the form will be open.
- Go to tool box and click on the button option and put the name as Filter in the button.
- Here the text field is specifying to enter the text in text field.
Syntax Example
DataView dv;
dv = new DataView(ds.Tables[0], "name = 'venkat' ", "name Desc",
DataViewRowState.CurrentRows);
dataGridView1.DataSource = dv;
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
DataSet ds = new DataSet();
private void button1_Click(object sender, EventArgs e)
{
string connectionString =
@"Data Source=VENKAT\KAASHIV;Initial Catalog=wikitechy;
user id = venkat;password=venkat";
string sql = "SELECT * FROM login_table";
SqlConnection connection = new SqlConnection(connectionString);
SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection);
connection.Open();
dataadapter.Fill(ds);
connection.Close();
dataGridView1.DataSource = ds.Tables[0];
}
private void button2_Click(object sender, EventArgs e)
{
DataView dv;
dv = new DataView(ds.Tables[0], "name = 'venkat' ",
"name Desc",DataViewRowState.CurrentRows);
dataGridView1.DataSource = dv;
}
}
}
C# Sample Code - C# Examples
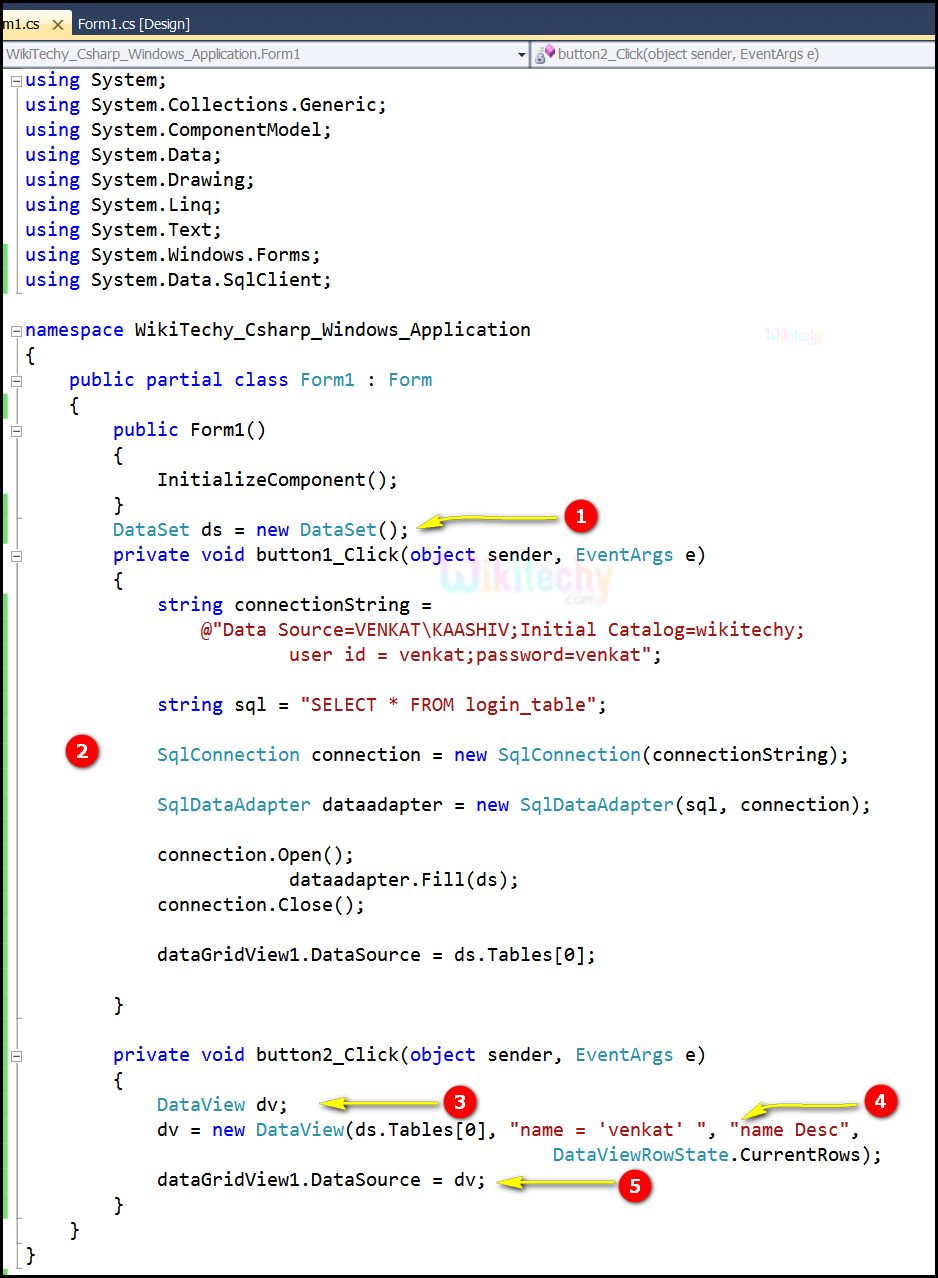
- Here DataSet ds = new DataSet(); specifies to Create a new instance of the DataSet class. The DataSet Object represents a complete set of data, including related tables, constraints, and relationships among the tables.
- Here SqlConnection connection = new SqlConnection (connectionString); this statement is used to acquire the SqlConnection as a resource.
- Here DataView dv; Used to filter in a DataTable, and additionally we can add new rows and modify the text in a DataTable.
- Here in this example dv = new DataView(ds.Tables[0], "name = 'venkat' ", "name Desc", DataViewRowState .CurrentRows); specifies to filter only "venkat" name in Dataset to Dataview method.
- dataGridView1.DataSource = dv; used to specify the data source of datagridview and the table which will be bind to. When we run the code a window will show with a datagridview that containing all the returning rows.
Sample C# examples - Output :
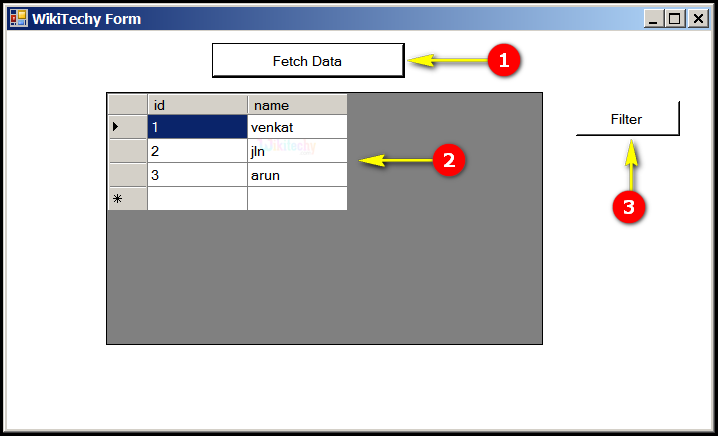
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the Id "1,2,3" and name "venkat, jln, arun".
- Here in this output table displays the button name as Filter.
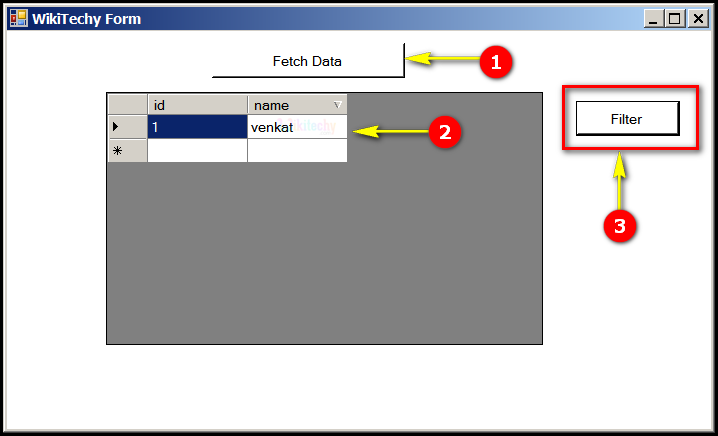
- Here in this output the Fetch Data button will display the data values from the sql and displays it by clicking it.
- Here in this output table displays the Id "1" and name "venkat".
- Here in this output we display Filter, the data filter in "venkat" row.