C# Anonymous Function - Anonymous Method in C#
C# Anonymous Function
- Anonymous function is a type of function that does not has name and we can say that a function without name is known as anonymous function.
- In C# anonymous function consists of two types, they are:
- Lambda Expressions
- Anonymous Methods
C# Lambda Expressions
- Lambda expression is an anonymous function which we can use to create delegates and it is also helpful to write LINQ queries.
- Here, we can use lambda expression to create local functions that can be passed as an argument.
Syntax
(input-parameters) => expression
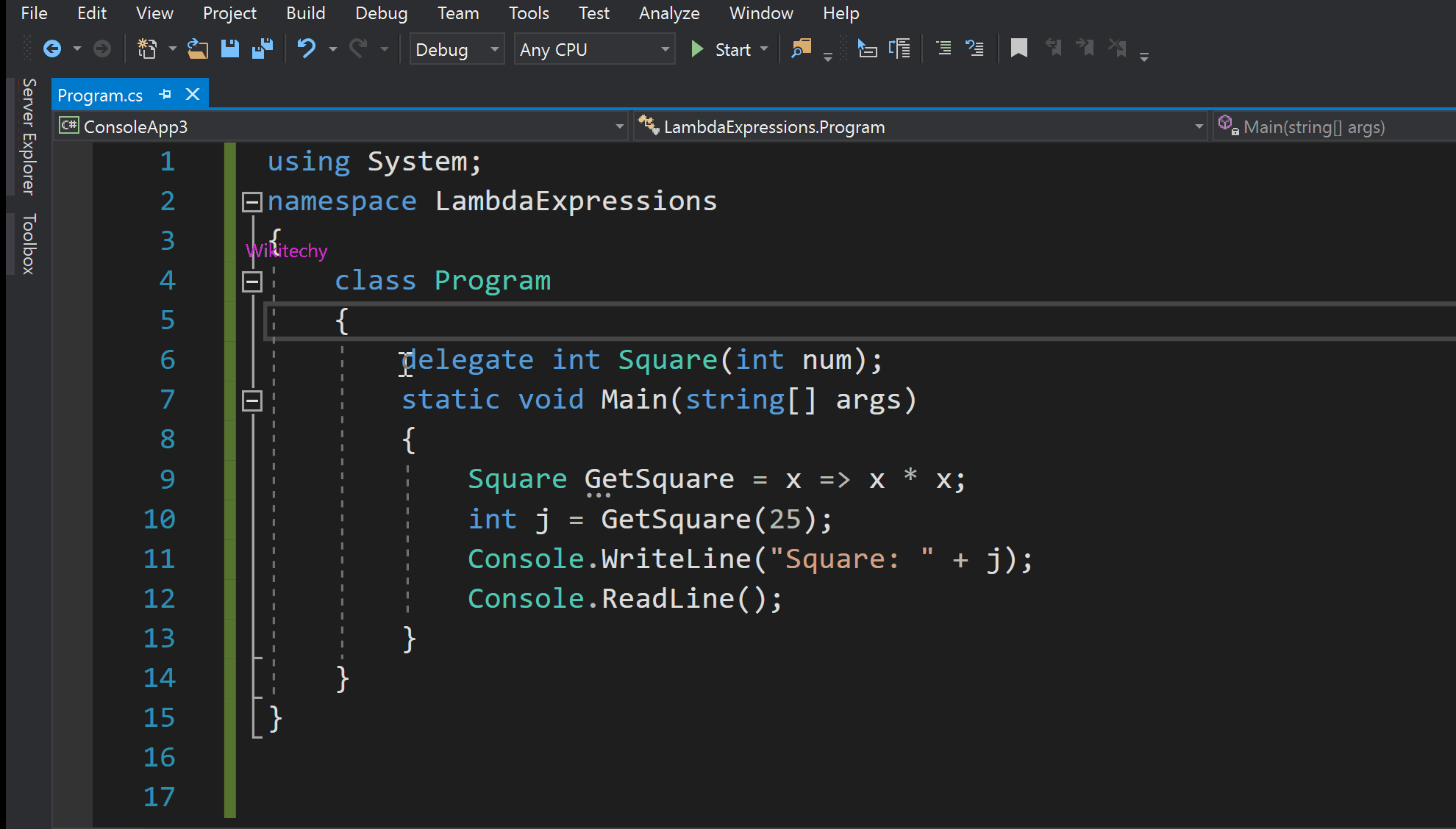
Sample Code
using System;
namespace LambdaExpressions
{
class Program
{
delegate int Square(int num);
static void Main(string[] args)
{
Square GetSquare = x => x * x;
int j = GetSquare(5);
Console.WriteLine("Square: "+j);
}
}
}
Output
Square : 25
C# Anonymous Methods
- Anonymous method provides the same functionality as lambda expression, except that it allows us to omit parameter list.
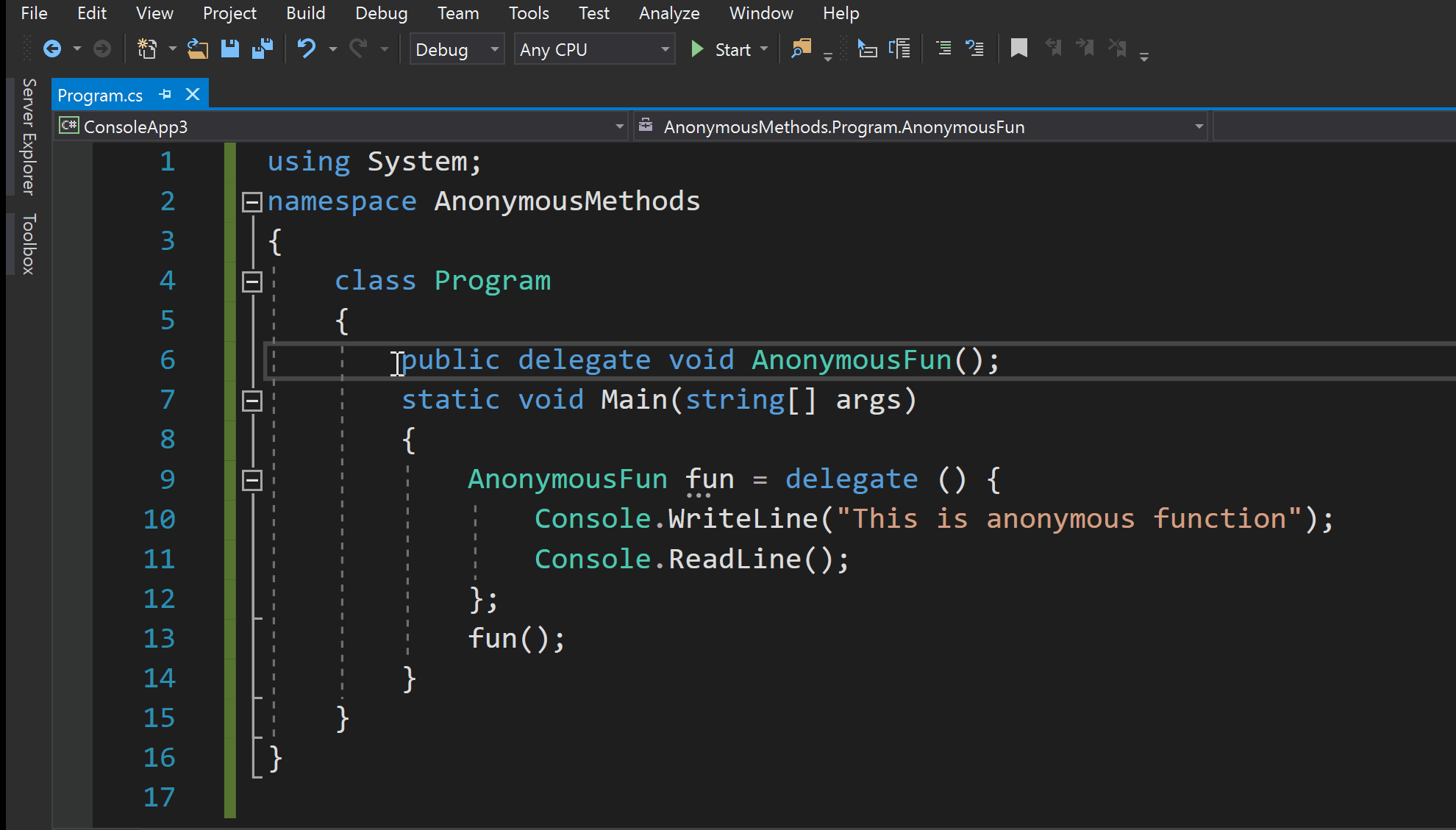
Sample Code
using System;
namespace AnonymousMethods
{
class Program
{
public delegate void AnonymousFun();
static void Main(string[] args)
{
AnonymousFun fun = delegate () {
Console.WriteLine("This is anonymous function");
};
fun();
}
}
}
Output
This is anonymous function