Datetime in C# - Date and Time in C# - C# DateTime Examples
Datetime in C#
- In C# we used the Date Time when there is a need to work with the dates and times.
- We can format the date and time in different formats by the properties and methods of the Date Time.
- The value of the Date Time is between the 12:00:00 midnight, January 1 0001 and 11:59:59 PM, December 31, 9999 A.D.
- We have different ways to create the Date Time object and it has Time, Date, Culture, Localization, Milliseconds.
From Date Time create the Date and Time
Date Time DOB= new Date Time (19, 56, 8, 12, 8, 12, 23);
From String creation of Date Time
string DateString= "8/12/1956 7:10:24 AM";
DateTime dateFromString =
DateTime.Parse(DateString, System.Globalization.CultureInfo.InvariantCulture);
Console.WriteLine(dateFromString.ToString());
Empty Date Time
Date Time Emp Date Time= new Date Time ();
Just Date
Date Time Only Date= new Date Time (2002, 10, 18);
Date Time from Ticks
Date Time Only Time= new Date Time (10000000);
Localization with Date Time
Date Time Date Time with Kind = new Date Time (1976, 7, 10, 7, 10, 24, Date Time Kind.Local);
Date Time with date, time and milliseconds
Date Time With Milli seconds= new Date Time (2010, 12, 15, 5, 30, 45, 100);
Properties of Date Time in C#
- We can get the name of the day from the week with the help of the Day Of Week property.
- We will use Day Of Year property, to get the day of the year and we use Time Of Day property, to get time in a Date Time.
- Today property will return the object of the Date Time, which is having today's value and the value of time is 12:00:00. Now property will return the Date Time object, which is having the current date and time.
- The Utc property of Date Time will return the Coordinated Universal Time (UTC).
- The Kind property returns value where the representation of time is done by the instance, which is based on the local time, Coordinated Universal Time (UTC) and it also shows the unspecified default value.
We will show it with the code with the pattern in a table
Code | Pattern |
---|---|
"d" | Short date |
"D" | Long date |
"f" | Full date time. Short time. |
"F" | Full date time. Long Time. |
"g" | Generate date time. Long Time. |
"G" | General date time. Long Time. |
"M","m." | Month/day |
"O","o" | Round trip date/time. |
"R","r" | RFC1123 |
"s" | Sortable date time. |
"t" | Sort Time |
"T" | Long Time |
"u" | Universal sortable date time. |
"U" | Universal full date-time. |
"Y","y" | Year, Month |
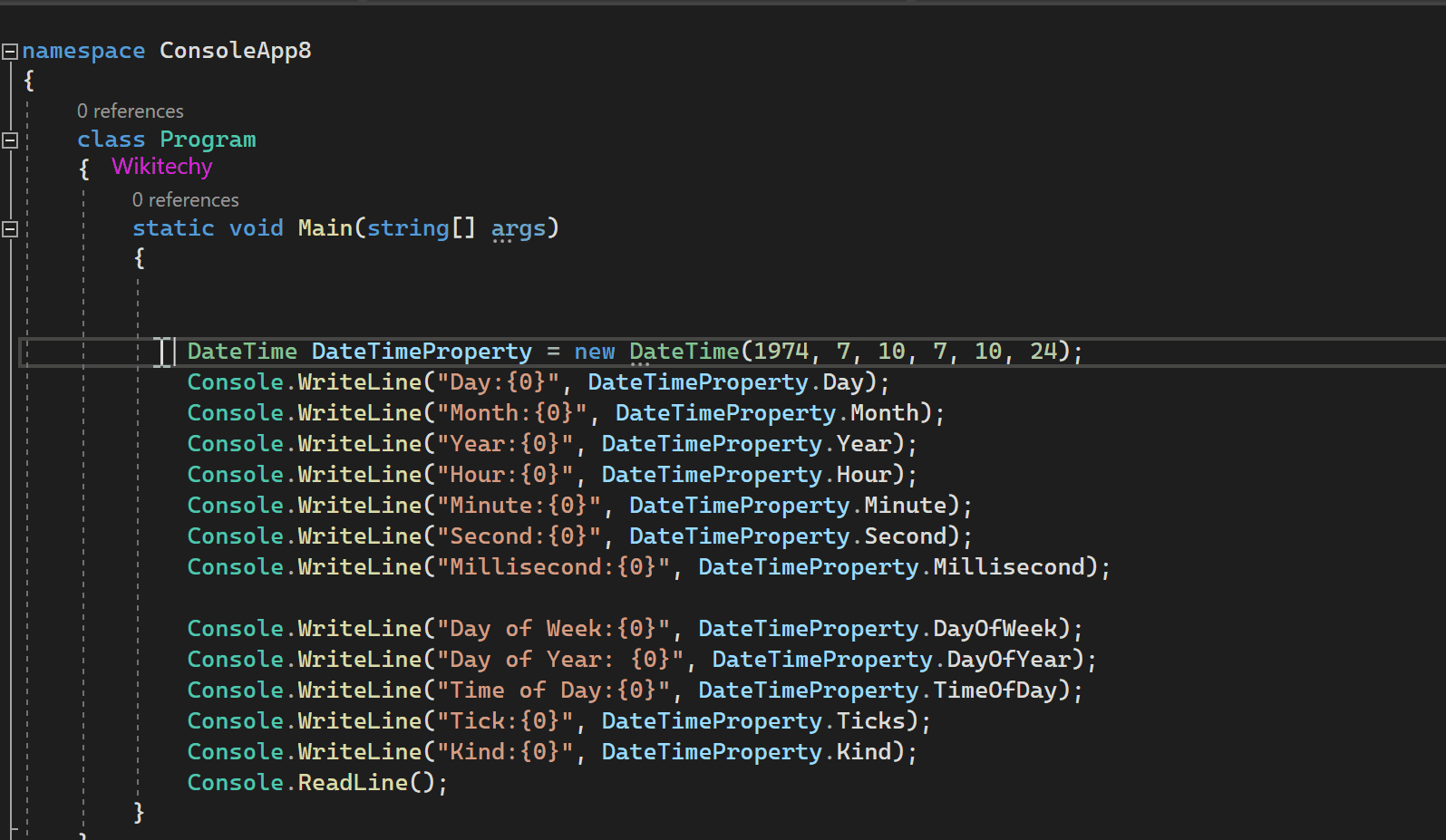
Sample Code
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp8
{
class Program
{
static void Main(string[] args)
{
DateTime DateTimeProperty = new DateTime(1974, 7, 10, 7, 10, 24);
Console.WriteLine("Day:{0}", DateTimeProperty.Day);
Console.WriteLine("Month:{0}", DateTimeProperty.Month);
Console.WriteLine("Year:{0}", DateTimeProperty.Year);
Console.WriteLine("Hour:{0}", DateTimeProperty.Hour);
Console.WriteLine("Minute:{0}", DateTimeProperty.Minute);
Console.WriteLine("Second:{0}", DateTimeProperty.Second);
Console.WriteLine("Millisecond:{0}", DateTimeProperty.Millisecond);
Console.WriteLine("Day of Week:{0}", DateTimeProperty.DayOfWeek);
Console.WriteLine("Day of Year: {0}", DateTimeProperty.DayOfYear);
Console.WriteLine("Time of Day:{0}", DateTimeProperty.TimeOfDay);
Console.WriteLine("Tick:{0}", DateTimeProperty.Ticks);
Console.WriteLine("Kind:{0}", DateTimeProperty.Kind);
Console.ReadLine();
}
}
}
Output
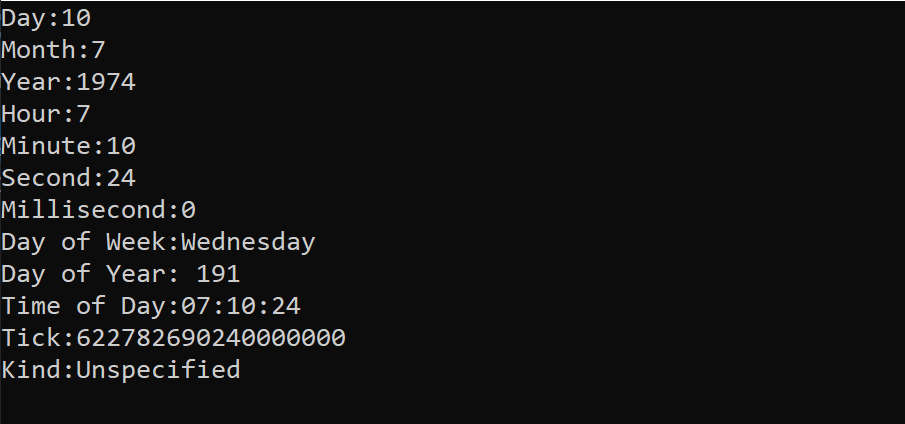