C# Datagridview | C# Controls Datagridview - c# - c# tutorial - c# net
What is the use of Datagridview in C# ?
- In C#, the DataGridView control available as a part of Windows Forms controls in Visual Studio 2008 is much more powerful than its previous versions.
- The DataGridView control provides several column types, enabling our users to enter and edit values in a variety of ways.
- If these column types do not meet our data-entry needs, we can create our own column types with cells that host controls of our choosing.
- In DataGridView control, we must define classes that derive from DataGridViewColumn and DataGridViewCell.
- Here in the below table is representing to Windows Forms:
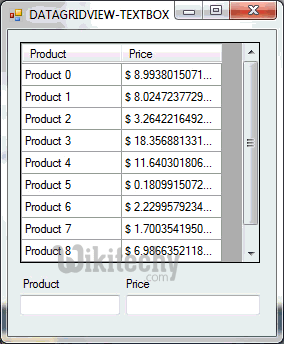
Datagridview
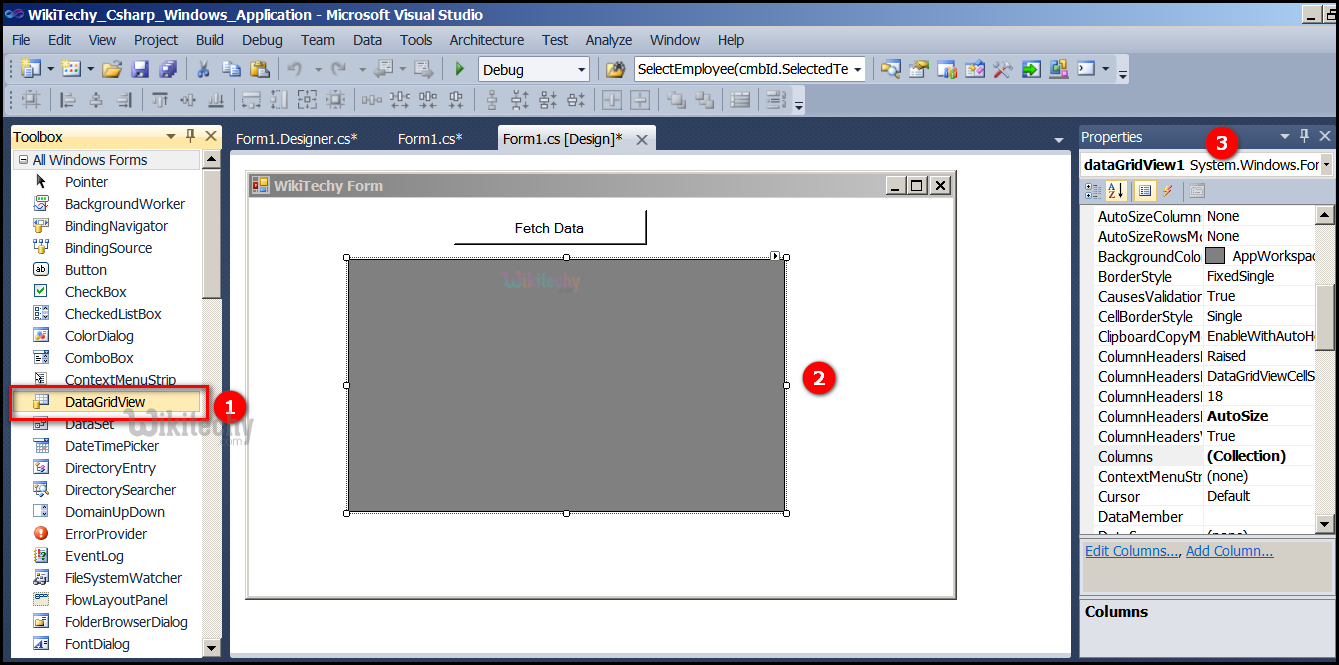
- The DataGridview specifies to displays data from SQL databases.
- Go to tool box and click on the DataGridview option the form will be open.
- Here the Properties window, specifies to properties for the form. Forms have various properties. For example, we can set the foreground and background color, title text that appears at the top of the form, size of the form, and other properties.
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Click(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
// create string array in c#
string[] items = new string[3];
items[0] = "venkat";
items[1] = "jln";
items[2] = "arun";
// string array to data grid
dataGridView1.DataSource = items;
}
}
}
Code Explanation:
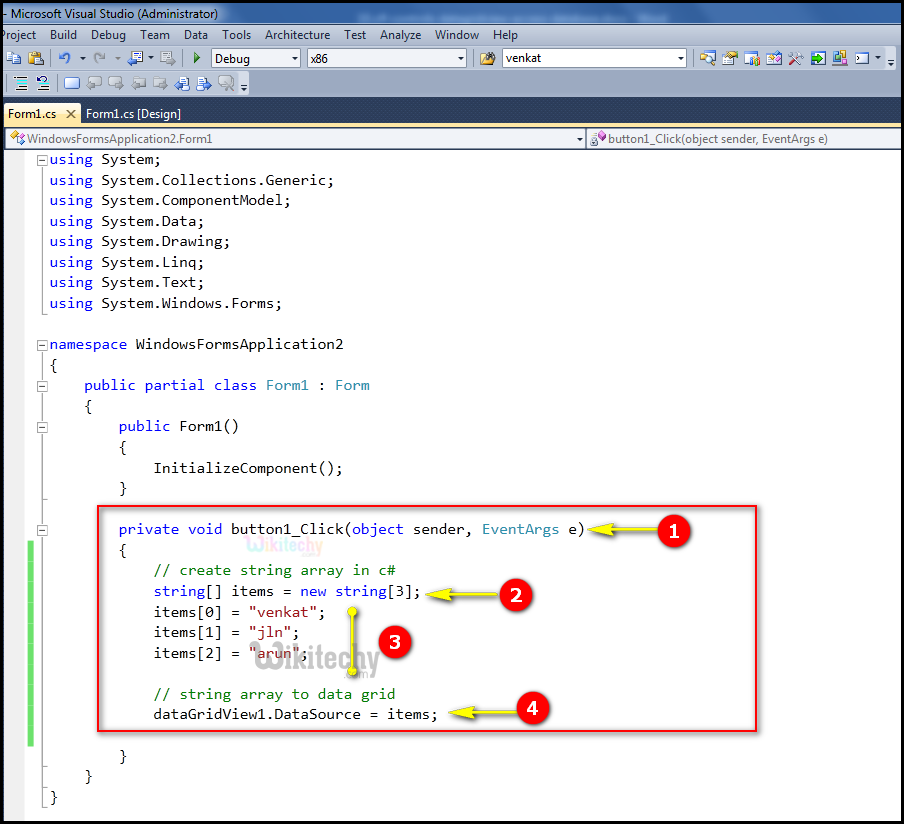
- Here private void button2_Click(object sender, EventArgs e) click event handler for button2 specifies, which is a normal Button control in Windows Forms. The two parameters, "object sender" and "EventArgs e" parameters of function of event of a button such as private void button2_Click(object sender, EventArgs e).
- Here string[] items = new string[3]; specifies to create a string array (0 to 3).
- Here in this example items[0] = "venkat"; items[1] = "jln";items[2] = "arun"; items "0,1,2" is an index values of array. "venkat, jln, arun" is specifies to string of array.
- Here dataGridView1.DataSource = items; specifies to string array to data grid that define the data source of datagridview and the table which will be bind to. When we run the code a window will show with a datagridview that containing all the returning rows.
Read Also
dotnet training in anna nagar chennai , advanced dotnet course syllabus , dotnet training onlineSample C# examples - Output :
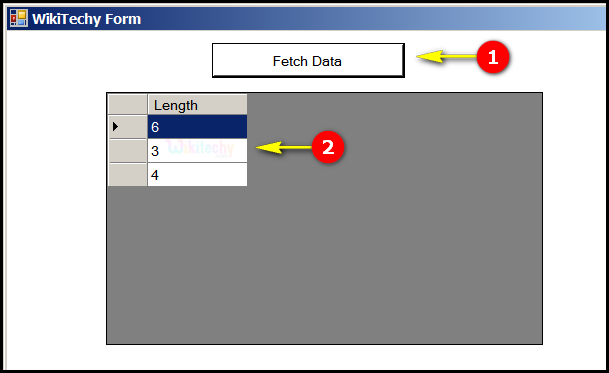
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the string Length "6,3,4".
- Accessing array will return the length of the array data and not the exact data itself.
- To access array data or bind array data in datagridview, we need to use datatable to bind
Data table:
- In data table, to Give definition and how data table can be created.
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Click(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
// create string array in c#
items[0] = "venkat";
items[1] = "jln";
items[2] = "arun";
DataTable dt = new DataTable();
dt.Columns.Add("Items");
foreach (string str in items)
{
DataRow dr = dt.NewRow();
dr[0] = str;
dt.Rows.Add(dr);
}
// string array to data grid
dataGridView1.DataSource = dt;
}
}
}
Code Explanation:
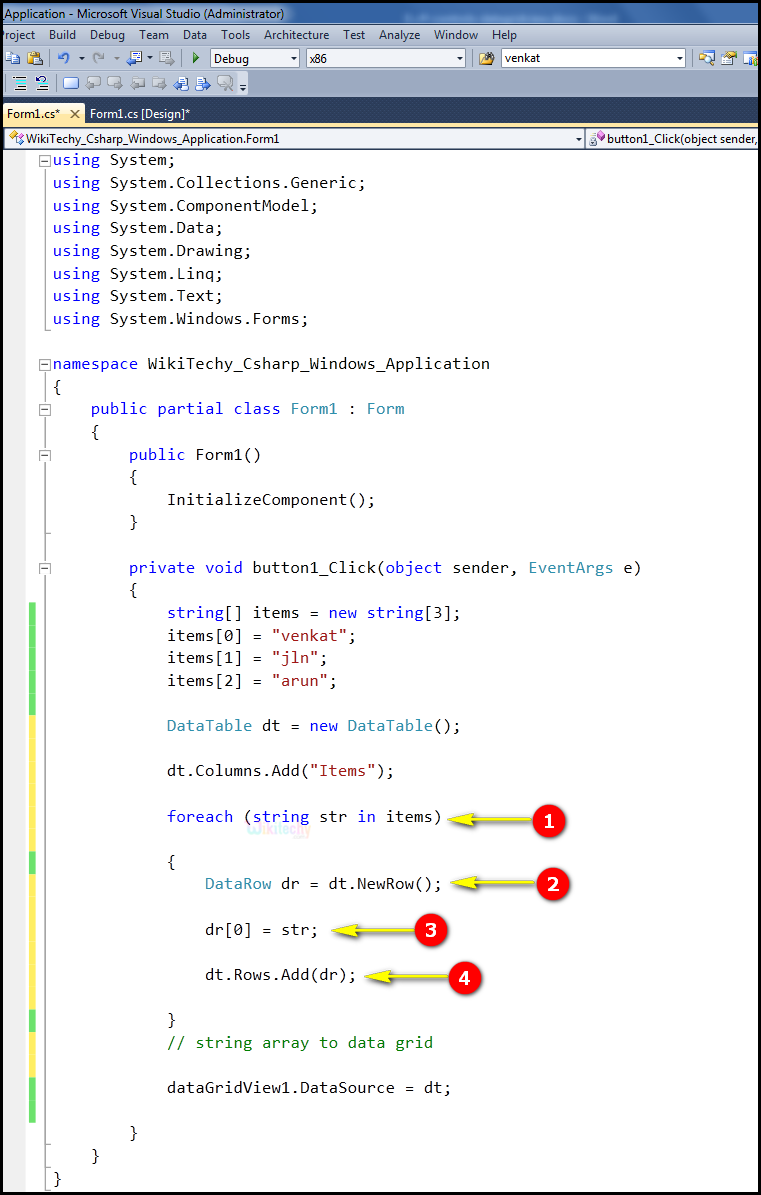
- Here in this example foreach (string str in items) specifies to Loop over strings items table.
- DataRow dr = dt.NewRow(); specifies to create a new row with the same schema of the table.
- dr[0] = str; Returns a string that represents the current object.
- dt.Rows.Add(dr); specifies to add new records into a dataset, a new data row must be created and added to the DataRow.
Sample C# examples - Output :
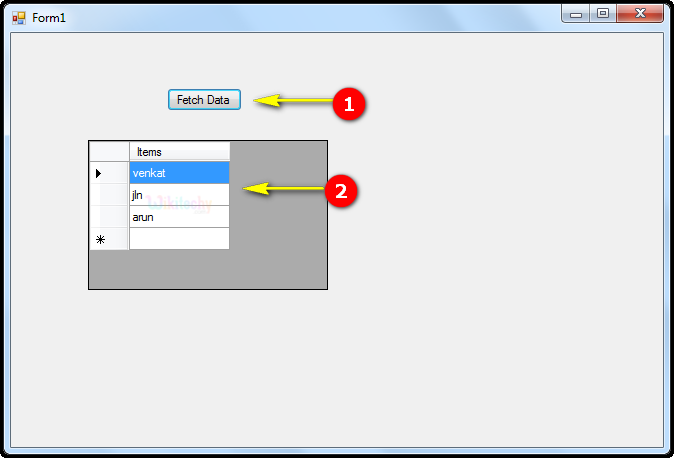
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the Id "1,2,3" and Items "venkat, jln, arun".
DataTable.LoadDataRow:
- We want to finds and updates a specific row. If no matching row is found, a new row is created which using the given values
public DataRow LoadDataRow(object[] values,
bool fAcceptChanges
)
Values:
- Type: System.Object[ ]
- Here System.Object[ ] is used to create a values of new row array in Data table.
fAcceptChanges:
- Type: System.Boolean
- Here System.Boolean is specify true to accept changes; otherwise false
- The LoadDataRow method takes an array of values and finds the matching value(s) in the primary key column(s).
- If a column has a default value, pass a null value in the array to set the default value for that column.
- Similarly, if a column has its AutoIncrement property set to true, pass a null value in the array to set the automatically generated value for the row.
- If the fAcceptChanges parameter is true or not specified in the row , the new data is added and then AcceptChanges is called to accept all changes in the DataTable; if the condition is false, newly added rows are marked as insertions, and changes to existing rows are marked as modifications.
C# Sample Code - C# Examples:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Click(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
// create string array in c#
string[] items = new string[1];
items[0] = "venkat";
DataTable dt = new DataTable();
dt.Columns.Add("Items");
dt.BeginLoadData();
DataRow dr = dt.LoadDataRow(items, true);
dt.EndLoadData();
// string array to data grid view
dataGridView1.DataSource = dt;
}
}
}
Code Explanation:
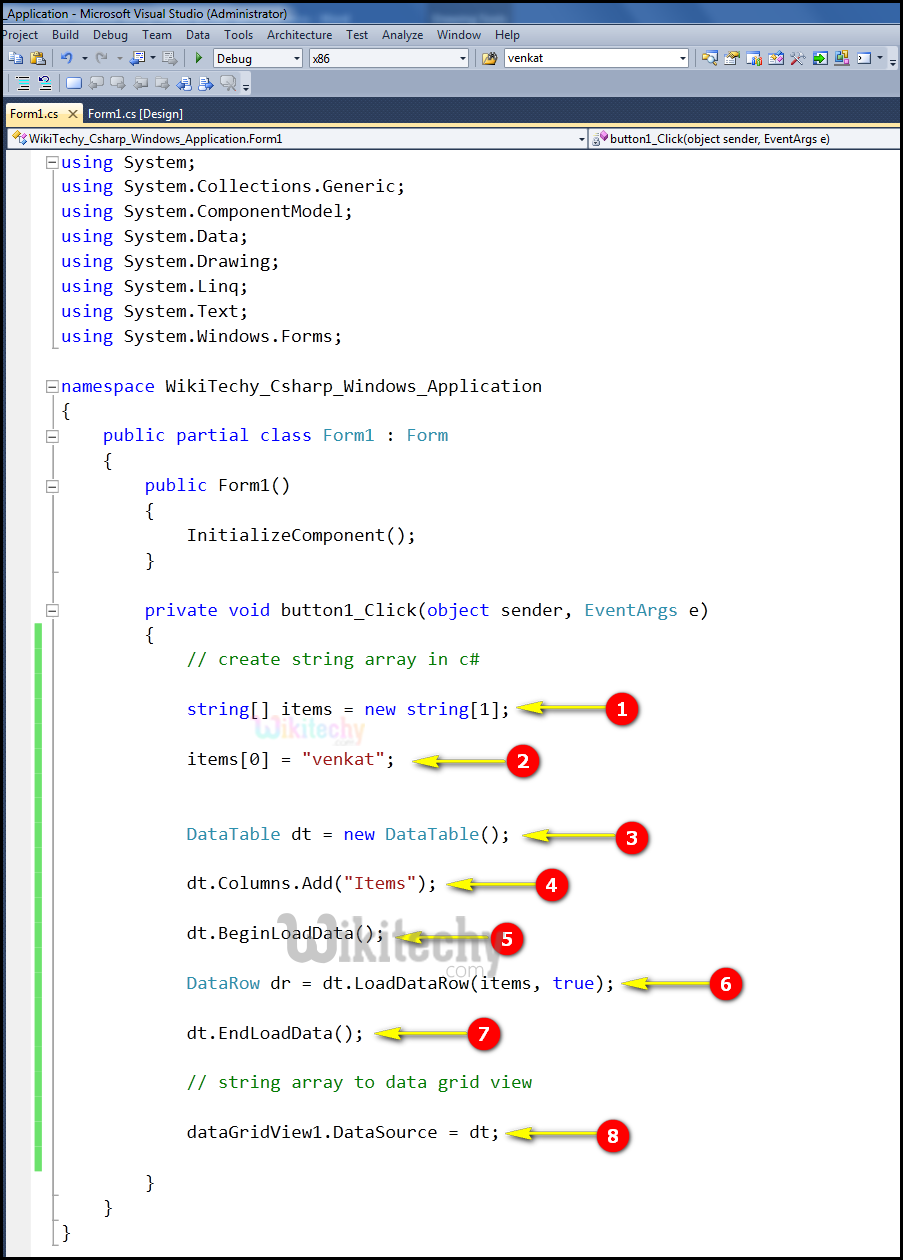
- Here string[] items = new string[1]; specifies to create a string array.
- Here in this example items[0] = "venkat"; items "0" is an index values of array. "venkat" is specifies to string of array.
- Here in this example DataTable dt = new DataTable(); specifies to initializes a new instance of the DataTable.
- Here in this example dt.Columns.Add("Items"); specifies to add new items in the table.
- Here dt.BeginLoadData(); specifies to BeginLoadData turns of constraint checking, so we can add the rows in table.
- Here DataRow dr = dt.LoadDataRow(items, true); specifies to finds and updates a specific row. If no matching row is found, a new row is created using the given values.
- Here dt.EndLoadData(); specifies to end the data.
- Here dataGridView1.DataSource = dt; used to specify the data source of datagridview and the table which will be bind to. When we run the code a window will show with a datagridview that containing all the returning rows.
Sample C# examples - Output :
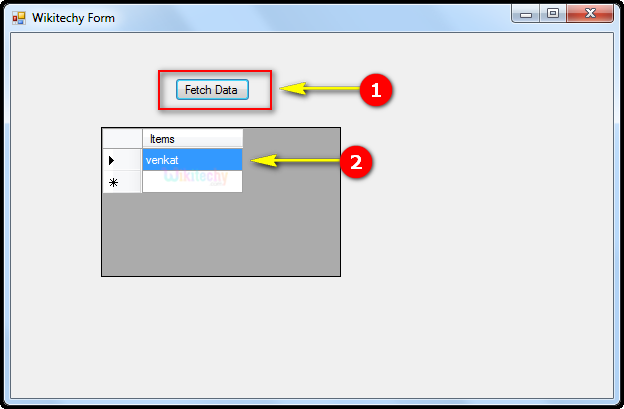
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the Id "1" and Items "venkat".