C# Delegates | Delegates in C# with Examples
C# Delegates
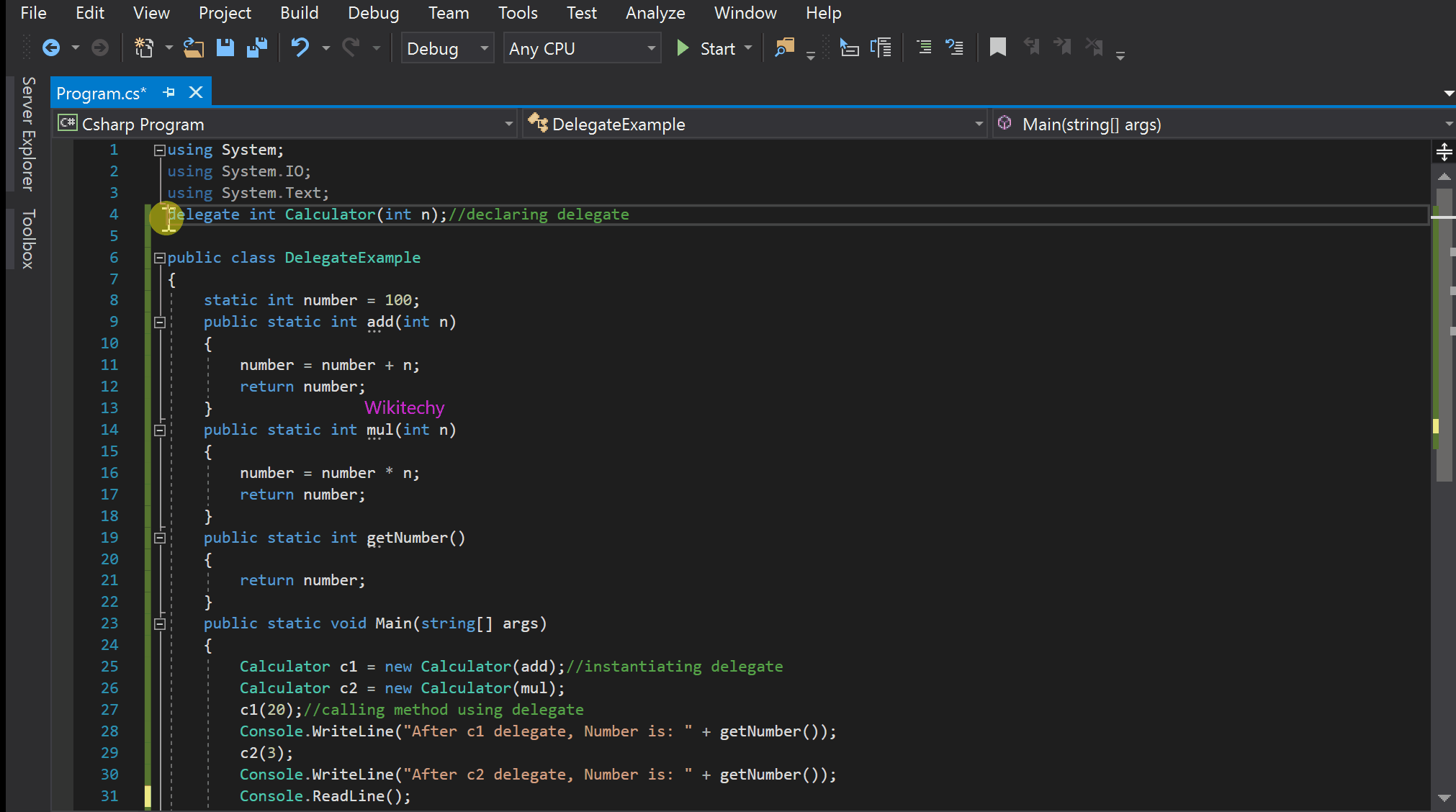
- In C#, delegate is a reference to the method and it works like function pointer in C and C++.
- It is secured, objected-oriented and type-safe than function pointer.
- Delegate encapsulates method only, for static method and it encapsulates method and instance both, for instance method.
- Internally a delegate declaration defines a class which is the derived class of System.Delegate.
Sample Code
using System;
using System.IO;
using System.Text;
delegate int Calculator(int n);//declaring delegate
public class DelegateExample
{
static int number = 100;
public static int add(int n)
{
number = number + n;
return number;
}
public static int mul(int n)
{
number = number * n;
return number;
}
public static int getNumber()
{
return number;
}
public static void Main(string[] args)
{
Calculator c1 = new Calculator(add);//instantiating delegate
Calculator c2 = new Calculator(mul);
c1(20);//calling method using delegate
Console.WriteLine("After c1 delegate, Number is: " + getNumber());
c2(3);
Console.WriteLine("After c2 delegate, Number is: " + getNumber());
Console.ReadLine();
}
}
Output
After c1 delegate, Number is: 120
After c2 delegate, Number is: 360