C# Collections - c# - c# tutorial - c# net
What is C# Collections ?
- In C#, collection represents group of objects. By the help of collections, we can perform various operations on objects such as
- store object
- update object
- delete object
- retrieve object
- search object, and
- sort object
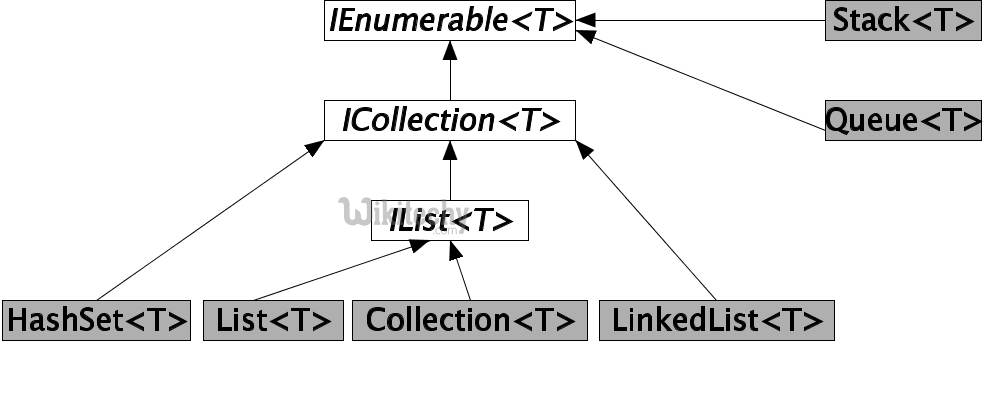
- In sort, all the data structure work can be performed by C# collections.
- We can store objects in array or collection.
- Collection has advantage over array.
- Array has size limit but objects stored in collection can grow or shrink dynamically.
- The System.Collections namespace includes following non-generic collections.
Non-generic Collections | Usage |
---|---|
ArrayList | ArrayList stores objects of any type like an array. However, there is no need to specify the size of the ArrayList like with an array as it grows automatically. |
SortedList | SortedList stores key and value pairs. It automatically arranges elements in ascending order of key by default. C# includes both, generic and non-generic SortedList collection. |
Stack | Stack stores the values in LIFO style (Last In First Out). It provides a Push() method to add a value and Pop() & Peek() methods to retrieve values. C# includes both, generic and non-generic Stack. |
Queue | Queue stores the values in FIFO style (First In First Out). It keeps the order in which the values were added. It provides an Enqueue() method to add values and a Dequeue() method to retrieve values from the collection. C# includes generic and non-generic Queue. |
Hashtable | Hashtable stores key and value pairs. It retrieves the values by comparing the hash value of the keys. |
BitArray | BitArray manages a compact array of bit values, which are represented as Booleans, where true indicates that the bit is on (1) and false indicates the bit is off (0). |
Types of Collections in C#:
- There are 3 ways to work with collections. The three namespaces are given below:
- System.Collections.Generic classes
- System.Collections classes (Now deprecated)
- System.Collections.Concurrent classes
System.Collections.Generic classes:
- The System.Collections.Generic namespace has following classes:
- List
- Stack
- Queue
- LinkedList
- HashSet
- SortedSet
- Dictionary
- SortedDictionary
- SortedList
System.Collections classes:
- These classes are legacy. It is suggested now to use System.Collections.Generic classes. The System.Collections namespace has following classes:
- ArrayList
- Stack
- Queue
- Hashtable
System.Collections.Concurrent classes:
- The System.Collections.Concurrent namespace provides classes for thread-safe operations.
- Now multiple threads will not create problem for accessing the collection items.
- The System.Collections.Concurrent namespace has following classes:
- ConcurrentBag
- BlockingCollection
- ConcurrentStack
- ConcurrentQueue
- ConcurrentDictionary
- Partitioner
- Partitioner
- OrderablePartitioner
Example 1:
/ Create a list of strings.
var salmons = new List<string>();
salmons.Add("chinook");
salmons.Add("coho");
salmons.Add("pink");
salmons.Add("sockeye");
// Iterate through the list.
foreach (var salmon in salmons)
{
Console.Write(salmon + " ");
}
C# examples - Output :
chinook coho pink sockeye
Example 2:
- The following example removes an element from the collection by specifying the object to remove.
// Create a list of strings by using a
// collection initializer.
var salmons = new List<string> { "chinook", "coho", "pink", "sockeye" };
// Remove an element from the list by specifying
// the object.
salmons.Remove("coho");
// Iterate through the list.
foreach (var salmon in salmons)
{
Console.Write(salmon + " ");
}
C# examples - Output :
chinook pink sockeye
- The following example removes elements from a generic list. Instead of a foreach statement, a for statement that iterates in descending order is used.
- This is because the RemoveAt method causes elements after a removed element to have a lower index value.