C# try/catch - c# - c# tutorial - c# net
What is the use of try/catch statement in C# ?
- In C# programming, exception handling is performed by try/catch statement.
- The try block in C# is used to place the code that may throw exception.
- The catch block is used to handled the exception.
- The catch block must be preceded by try block.
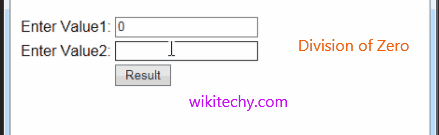
Try Catch Exception
C# example without try/catch
using System;
public class ExExample
{
public static void Main(string[] args)
{
int a = 10;
int b = 0;
int x = a/b;
Console.WriteLine("Rest of the code");
}
}
C# examples - Output :
Unhandled Exception: System.DivideByZeroException: Attempted to divide by zero.
Read Also
.net internship near me , dot net training course fees in chennai , dot net training institutes in chennaiC# try/catch example
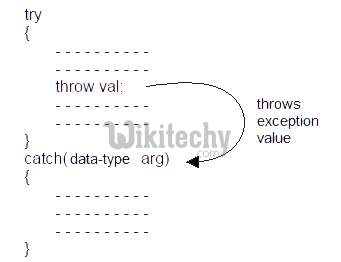
Try Catch Exception
using System;
public class ExExample
{
public static void Main(string[] args)
{
try
{
int a = 10;
int b = 0;
int x = a / b;
}
catch (Exception e) { Console.WriteLine(e); }
Console.WriteLine("Rest of the code");
}
}
C# examples - Output :
System.DivideByZeroException: Attempted to divide by zero.
Rest of the code