C# Datagridview Add Row | C# Controls Datagridview Add Row - c# - c# tutorial - c# net
What is Datagridview?
- The DataGridView control is designed to be a complete solution for displaying tabular data with Windows Forms.
- The DataGridView control is highly configurable and extensible, and it provides many properties, methods, and events to customize its appearance and behavior.
- The DataGridView control provides TextBox, CheckBox, Image, Button, ComboBox and Link columns with the corresponding cell types.
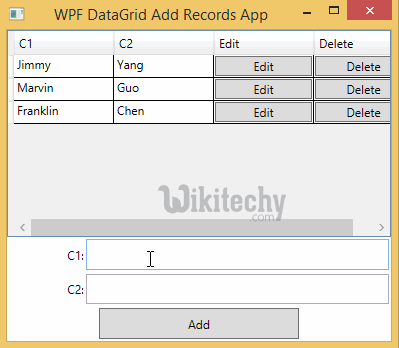
DataGridview Add Row
Add Row:
- In DataGridView Add Rows, rows can be added to a DataGridView.
- In c#, Rows cannot be programmatically added to the DataGridView's rows collection when the control is data-bound.
- Here in the below table is representing to Windows Forms:
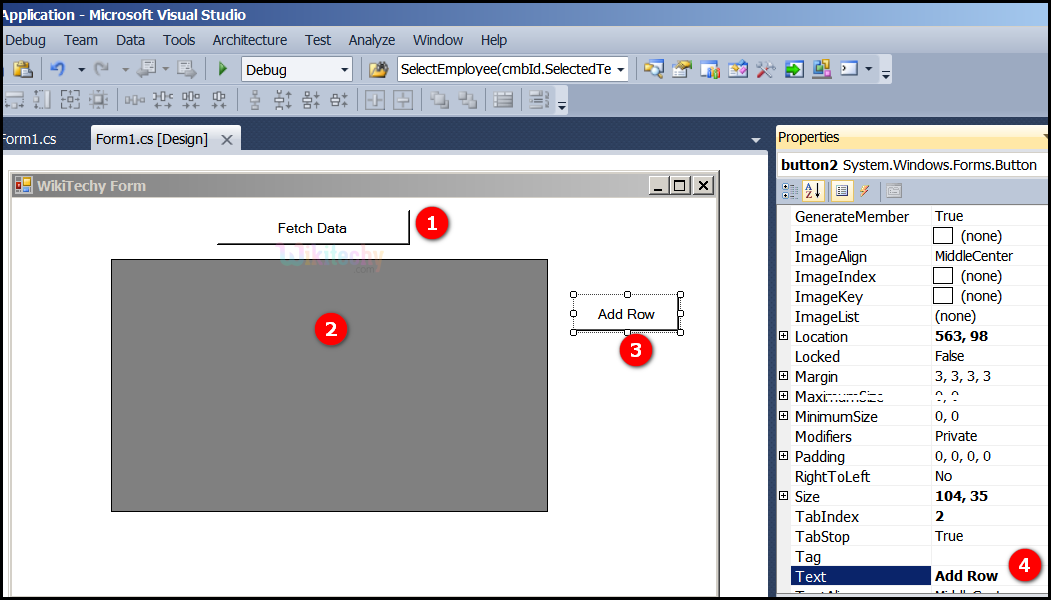
- Here Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Go to tool box and click on the DataGridview option the form will be open.
- Go to tool box and click on the button option and put the name as Add Row in the text button.
- Here the text field is specifying to enter the text in text field.
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
DataSet ds = new DataSet();
private void button2_Click(object sender, EventArgs e)
{
string connectionString =
@"Data Source=VENKAT\KAASHIV;Initial Catalog=wikitechy;
user id = venkat;password=venkat";
string sql = "SELECT * FROM login_table";
SqlConnection connection = new SqlConnection(connectionString);
SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection);
connection.Open();
dataadapter.Fill(ds);
connection.Close();
dataGridView1.DataSource = ds.Tables[0];
}
private void button2_Click(object sender, EventArgs e)
{
DataTable dataTable = (DataTable)dataGridView1.DataSource;
DataRow drToAdd = dataTable.NewRow();
drToAdd[0] = 4;
drToAdd[1] = "jacob";
dataTable.Rows.Add(drToAdd);
dataTable.AcceptChanges();
dataGridView1.DataSource = dataTable;
}
}
}
Code Explanation:
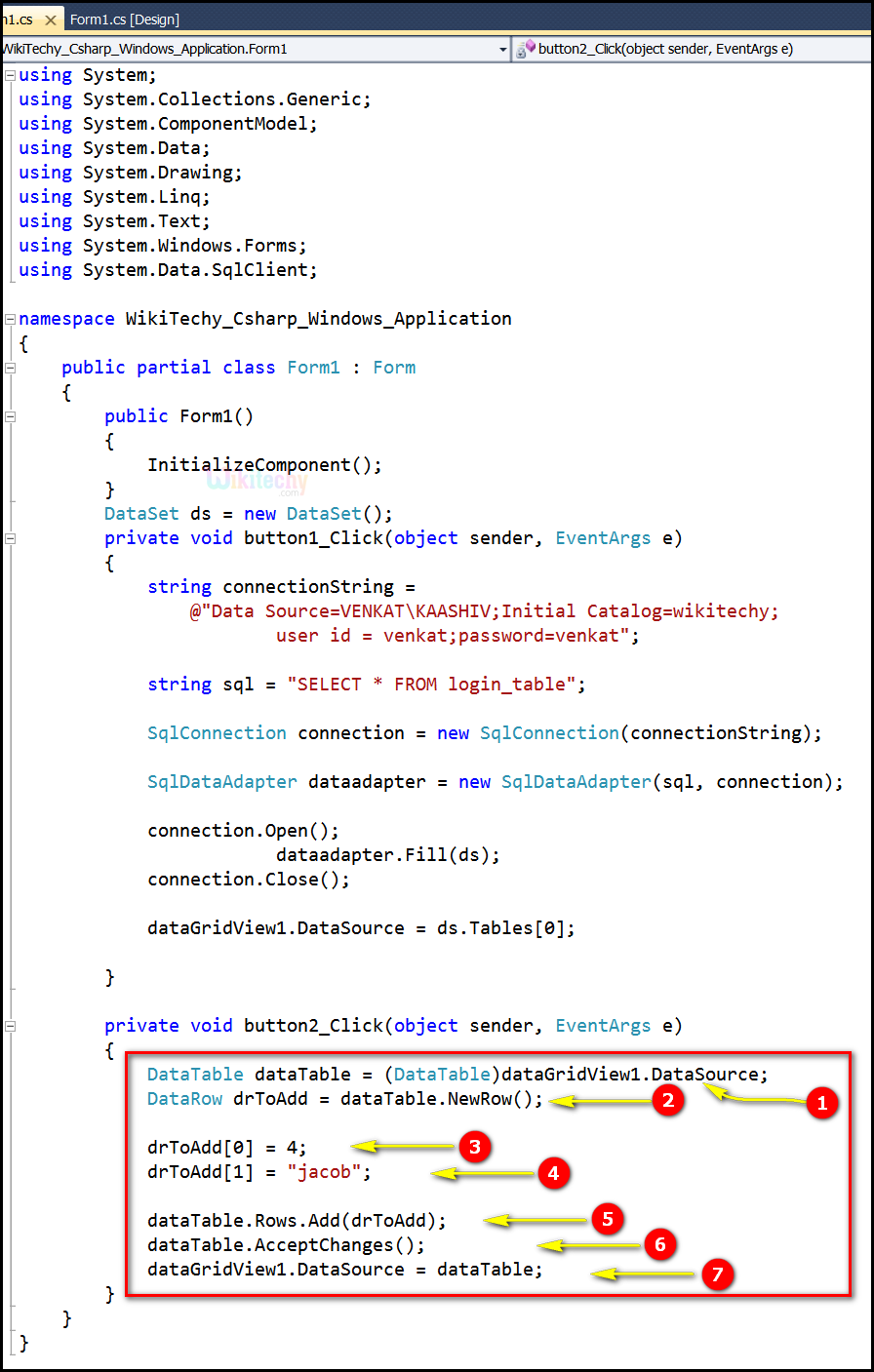
- Here DataTable dataTable = (DataTable) dataGridView1. DataSource; specifies to dataTable can stores the rows and columns of data.
- Here DataRow drToAdd = dataTable.NewRow(); specifies to Creates a new DataRow with the same schema as the table.
- Here in this example drToAdd[0] = 4; specifies to add the four rows in the dataTable.
- Here in this example drToAdd[1] = "jacob"; specifies to add Jacob new row in the DataTable.
- Here dataTable.Rows.Add(drToAdd); specifies to new row is add to DataTable.
- Here dataTable.AcceptChanges(); specifies to Commits all the changes complete to this table, then the last time AcceptChanges was called.
- Here dataGridView1.DataSource = dataTable; used to specify the data source of datagridview and the table which will be bind to. When we run the code a window will show with a datagridview that containing all the returning rows.
Sample Output
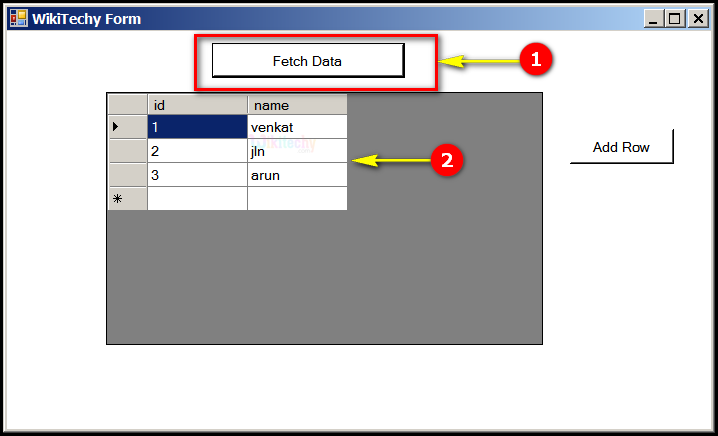
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the Id "1,2,3" and name "venkat, jln, arun".
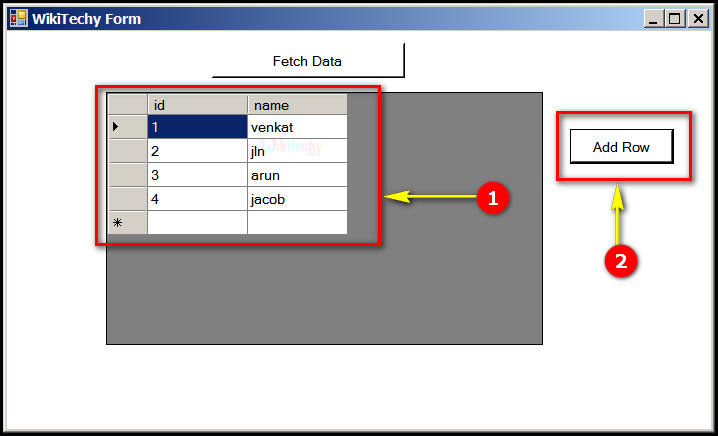
- Here in this output table displays the Id "1,2,3,4" and name "venkat, jln, arun, jacob".
- Here in this output we display Add Row, which is add the new row "jacob" in the DataTable.