C# SortedSet - c# - c# tutorial - c# net
What is Sortedset in C# ?
- C# SortedSet class can be used to store, remove or view elements.
- It maintains ascending order and does not store duplicate elements.
- It is suggested to use SortedSet class if you have to store unique elements and maintain ascending order.
- It is found in System.Collections.Generic namespace.
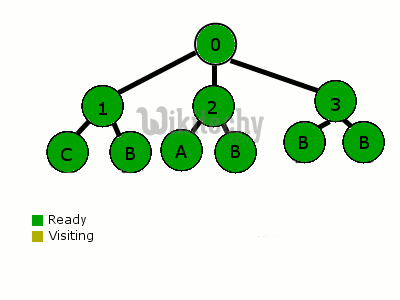
Sorting
Constructors:
Name | Description |
---|---|
SortedSet<T>() | Initializes a new instance of the SortedSet<T> class |
SortedSet<T>(IComparer<T>) | Initializes a new instance of the SortedSet<T> class that uses a specified comparer. |
SortedSet<T>(IEnumerable<T>) | Initializes a new instance of the SortedSet<T> class that contains elements copied from a specified enumerable collection. |
SortedSet<T>(IEnumerable<T>, IComparer<T>) | Initializes a new instance of the SortedSet<T> class that contains elements copied from a specified enumerable collection and that uses a specified comparer. |
SortedSet<T>(SerializationInfo, StreamingContext) | Initializes a new instance of the SortedSet<T> class that contains serialized data. |
Properties:
Name | Description |
---|---|
Comparer | Gets the IComparer<T> object that is used to order the values in the SortedSet<T>. |
Count | Gets the number of elements in the SortedSet<T>.. |
Max | Gets the maximum value in the SortedSet<T>, as defined by the comparer. |
Min | Gets the minimum value in the SortedSet<T>, as defined by the comparer |
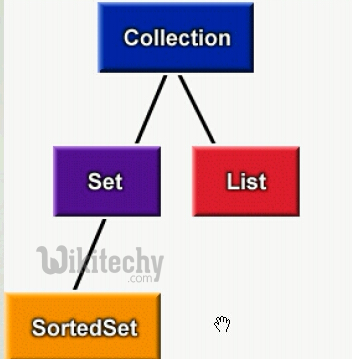
Csharp sorted set
C# SortedSet<T> example:
- Let's see an example of generic SortedSet<T> class that stores elements using Add() method and iterates elements using for-each loop.
using System;
using System.Collections.Generic;
public class SortedSetExample
{
public static void Main(string[] args)
{
// Create a set of strings
var names = new SortedSet<string>();
names.Add("Sonoo");
names.Add("Ankit");
names.Add("Peter");
names.Add("Irfan");
names.Add("Ankit");//will not be added
// Iterate SortedSet elements using foreach loop
foreach (var name in names)
{
Console.WriteLine(name);
}
}
}
C# examples - Output :
Ankit
Irfan
Peter
Sonoo
C# SortedSet<T> example 2
- Let's see another example of generic SortedSet<T> class that stores elements using Collection initializer.
using System;
using System.Collections.Generic;
public class SortedSetExample
{
public static void Main(string[] args)
{
// Create a set of strings
var names = new SortedSet<string>(){"Sonoo", "Ankit", "Peter", "Irfan"};
// Iterate SortedSet elements using foreach loop
foreach (var name in names)
{
Console.WriteLine(name);
}
}
}
C# examples - Output :
Ankit
Irfan
Peter
Sonoo