C# Regular Expression - Regular Expression in C#
C# Regular Expression - Regular Expression in C#
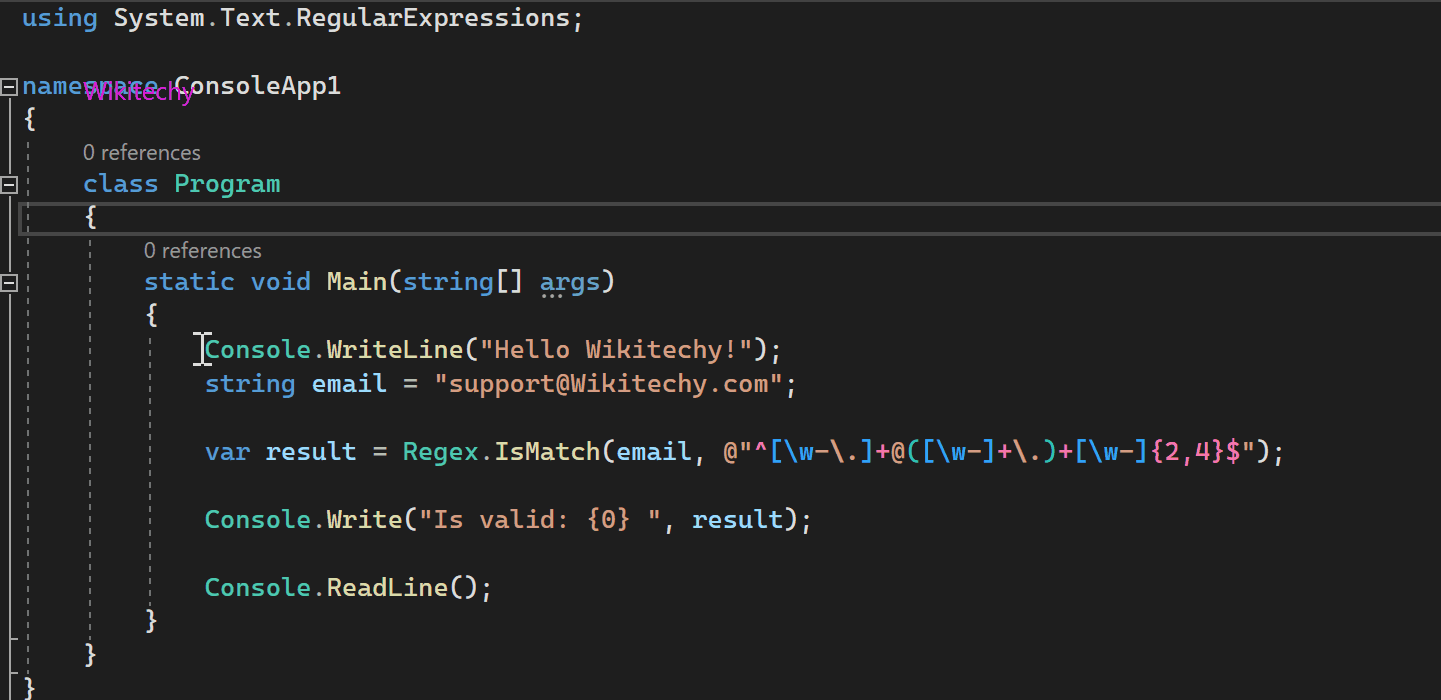
- In C#, Regular Expression is used for the parsing and validation of the given text to match with the defined pattern for example, an email address.
- The pattern can contain characters, operators, literals and constructs.
- Generally, we used the regular expression engine, to process the text with the regular Expression in .NET Framework.
- Regular Expression is shown by the Regex, in C#.
- We used the Regular Expression to check whether the given string matches the pattern or not.
- Regex or Regular Expression is a sequence of characters that defines the pattern.
- The pattern can contain literals, characters, numbers, operators, etc.
- We used the Patterns to search the strings or files and here we will see if the matches are found or not.
- Generally, the Regular Expressions are used for the parsing, finding the validations or strings, etc.
Regex Class
- In .NET Framework regex class shows the regular expression engine.
- This class is used to parse a large amount of text for finding the specific character pattern.
- We can use this class for the extraction, editing, for the replacement, or to delete the text of the substring.
- Regex Class has the string pattern as a parameter with the other optional parameter and System.Text.RegularExpressions namespace contains the Regex class.
Create a pattern for the word that starts with letter "M"
string pattern = @"\b[M]\w+";
Create a Regex
Regex rgex = new Regex(pattern);
Create a Long string
string authors = "Ramesh chand, Rakeshwar";
Get all matches
MatchCollection matchedAuthors = rg.Matches(authors);
To find the matches collection we will use the For loop // Print all matched authors
for (int count = 0; count < matchedAuthors.Count; count++)
Console.WriteLine(matchedAuthors[count]. Value);
For print all matched authors
for (int count = 0; count < matchedAuthors.Count; count++)
Console.WriteLine(matchedAuthors[count].Value);
Sample Code
using System;
using System.Text.RegularExpressions;
namespace ConsoleApp1
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello Wikitechy!");
string email = "support@Wikitechy.com";
var result = Regex.IsMatch(email, @"^[\w-\.]+@([\w-]+\.)+[\w-]{2,4}$");
Console.Write("Is valid: {0} ", result);
Console.ReadLine();
}
}
}
Output
