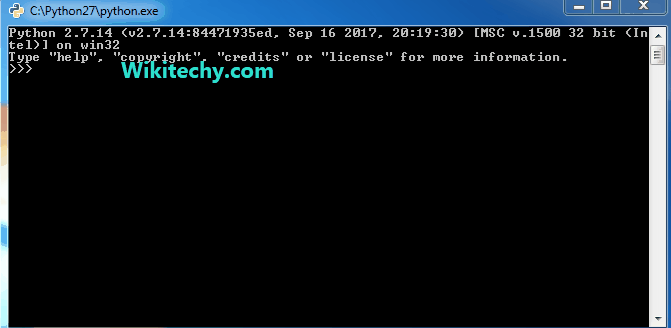
Learn Python - Python tutorial - python string template - Python examples - Python programs
In String module, Template Class allows us to create simplified syntax for output specification. The format uses placeholder names formed by $ with valid Python identifiers (alphanumeric characters and underscores). Surrounding the placeholder with braces allows it to be followed by more alphanumeric letters with no intervening spaces. Writing $$ creates a single escaped $:
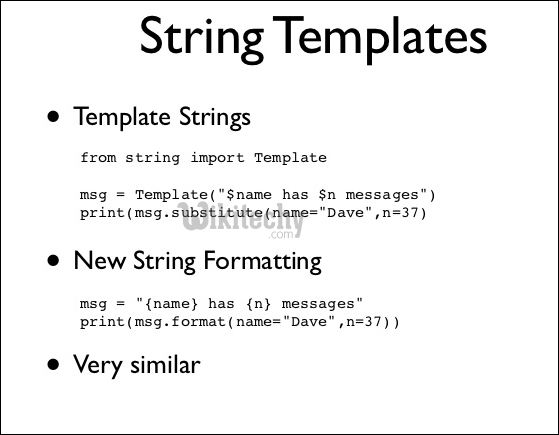
Below is a simple example.
# A Simple Python templaye example
from string import Template
# Create a template that has placeholder for value of x
t = Template('x is $x')
# Substitute value of x in above template
print (t.substitute({'x' : 1}))
python tutorial - Output :
x is 1
Following is another example where we import names and marks of students from a list and print them using template.
python - Sample - python code :
# A Python program to demonstrate working of string template
from string import Template
# List Student stores the name and marks of three students
Student = [('Ram',90), ('Ankit',78), ('Bob',92)]
# We are creating a basic structure to print the name and
# marks of the students.
t = Template('Hi $name, you have got $marks marks')
for i in Student:
print (t.substitute(name = i[0], marks = i[1]))
python tutorial - Output :
Hi Ram, you have got 90 marks
Hi Ankit, you have got 78 marks
Hi Bob, you have got 92 marks
The substitute() method raises a KeyError when a placeholder is not supplied in a dictionary or a keyword argument. For mail-merge style applications, user supplied data may be incomplete and the safe_substitute() method may be more appropriate — it will leave placeholders unchanged if data is missing:
Another application for template is separating program logic from the details of multiple output formats. This makes it possible to substitute custom templates for XML files, plain text reports, and HTML web reports.
Note that there are other ways also to print formatted output like %d for integer, %f for float, etc.,