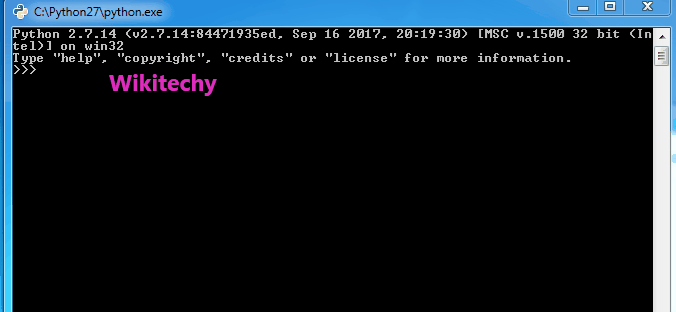
Learn Python - Python tutorial - python data types - Python examples - Python programs
Strings in Python
A string is a sequence of characters. It can be declared in python by using double quotes. Strings are immutable, i.e., they cannot be changed.
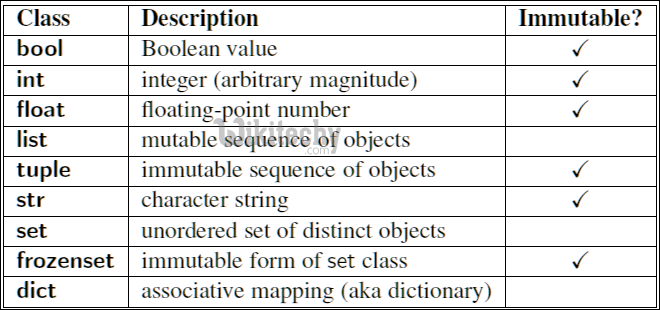
python - Sample - python code :
# Assigning string to a variable
a = "This is a string"
print a
click below button to copy the code. By Python tutorial team
Lists in Python
Lists are one of the most powerful tools in python. They are just like the arrays declared in other languages. But the most powerful thing is that list need not be always homogenous. A single list can contain strings, integers, as well as objects. Lists can also be used for implementing stacks and queues. Lists are mutable, i.e., they can be altered once declared.
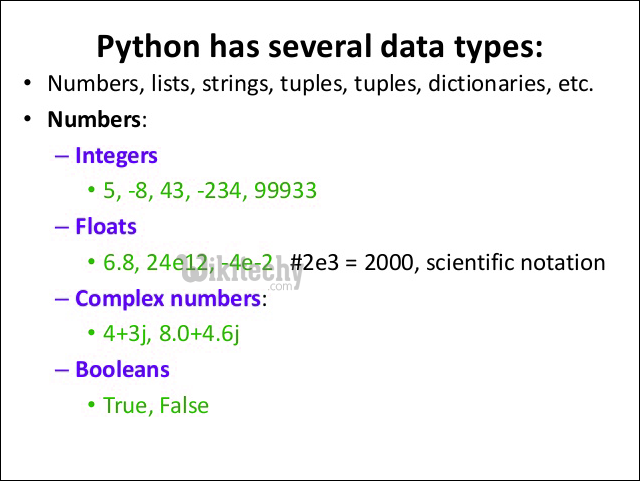
python - Sample - python code :
# Declaring a list
L = [1, "a" , "string" , 1+2]
print L
L.append(6)
print L
L.pop()
print L
print L[1]
click below button to copy the code. By Python tutorial team
python programming - Output :
[1, 'a', 'string', 3]
[1, 'a', 'string', 3, 6]
[1, 'a', 'string', 3]
a
Tuples in Python
A tuple is a sequence of immutable Python objects. Tuples are just like lists with the exception that tuples cannot be changed once declared. Tuples are usually faster than lists.
python - Sample - python code :
tup = (1, "a", "string", 1+2)
print tup
print tup[1]
click below button to copy the code. By Python tutorial team
python programming - Output :
(1, 'a', 'string', 3)
a
Iterations in Python
Iterations or looping can be performed in python by ‘for’ and ‘while’ loops. Apart from iterating upon a particular condition, we can also iterate on strings, lists, and tuples.
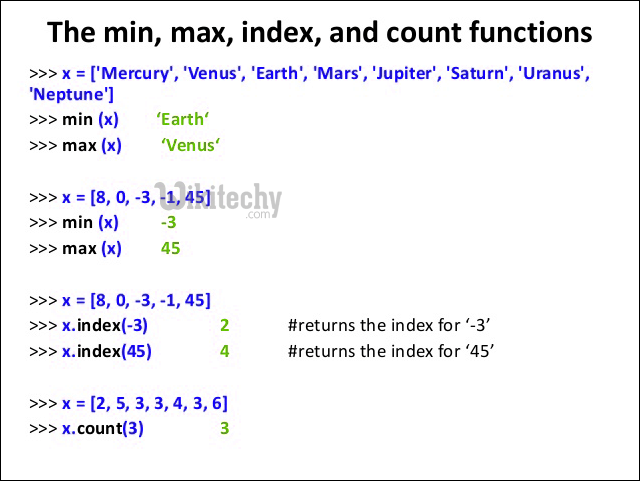
Example1: Iteration by while loop for a condition
python - Sample - python code :
i = 1
while (i < 10):
i += 1
print i,
click below button to copy the code. By Python tutorial team
python programming - Output :
2 3 4 5 6 7 8 9 10
Example 2: Iteration by for loop on string
python - Sample - python code :
s = "Hello World"
for i in s :
print i
click below button to copy the code. By Python tutorial team
python programming - Output :
H
e
l
l
o
W
o
r
l
d
Example 3: Iteration by for loop on list
python - Sample - python code :
L = [1, 4, 5, 7, 8, 9]
for i in L:
print i,
click below button to copy the code. By Python tutorial team
python programming - Output :
1 4 5 7 8 9
Example 4 : Iteration by for loop for range
python - Sample - python code :
for i in range(0, 10):
print i,
click below button to copy the code. By Python tutorial team
python programming - Output :
0 1 2 3 4 5 6 7 8 9