For strings in python, boolean operators (and , or) work. Let us consider the two strings namely str1 and str2 and try boolean operators on them:
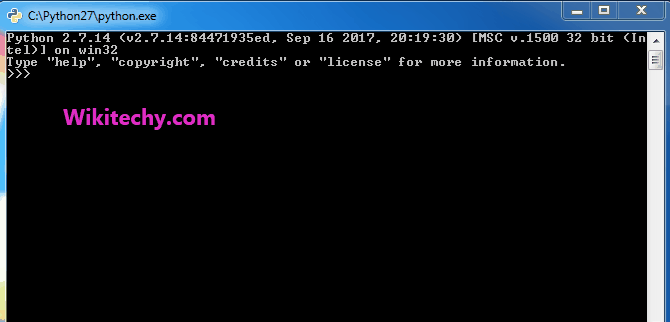
Learn Python - Python tutorial - python striprepr - Python examples - Python programs
python - Sample - python code :
str1 = ''
str2 = 'wiki'
# repr is used to print the string along with the quotes
print repr(str1 and str2) # Returns str1
print repr(str2 and str1) # Returns str1
print repr(str1 or str2) # Returns str2
print repr(str2 or str1) # Returns str2
str1 = 'techy'
print repr(str1 and str2) # Returns str2
print repr(str2 and str1) # Returns str1
print repr(str1 or str2) # Returns str1
print repr(str2 or str1) # Returns str2
Output:
''
''
'wiki'
'wiki'
'wiki'
'techy'
'techy'
'wiki'
The output of the boolean operations between the strings depends on following things:
- Python considers empty strings as having boolean value of ‘false’ and non-empty string as having boolean value of ‘true’.
- For ‘and’ operator if left value is true, then right value is checked and returned. If left value is false, then it is returned
- For ‘or’ operator if left value is true, then it is returned, otherwise if right value is false, then right value is returned.
Note that the bitwise operators (| , &) don’t work for strings.