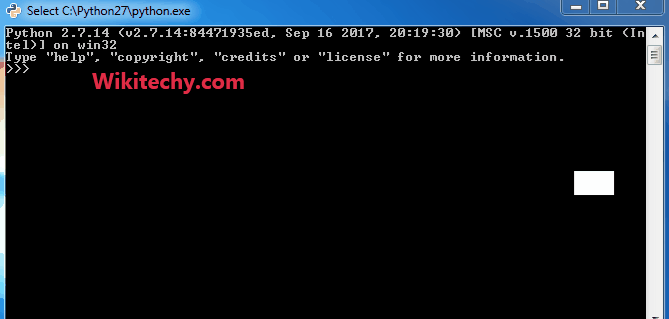
Learn Python - Python tutorial - python string split - Python examples - Python programs
Splitting a string by some delimiter is a very common task. For example, we have a comma separated list of items from a file and we want individual items in an array.
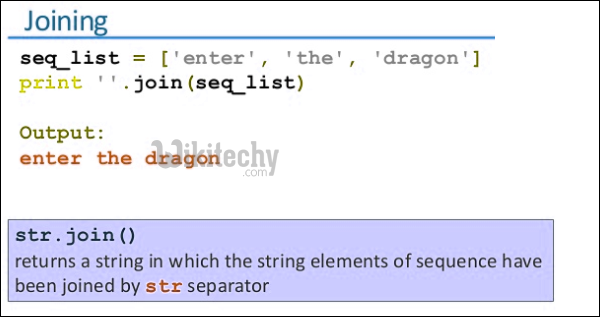
Almost all programming languages, provide function split a string by some delimiter.
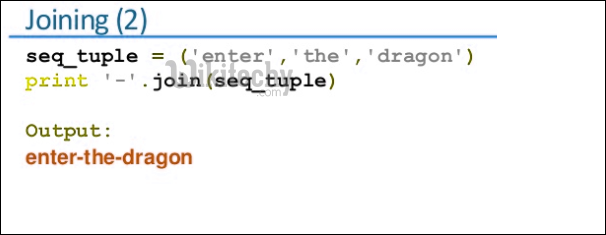
In C/C++:
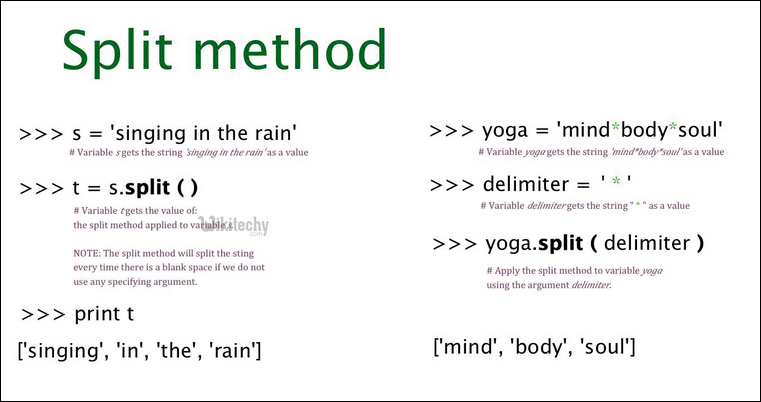
python - Sample - python code :
// Splits str[] according to given delimiters.
// and returns next token. It needs to be called
// in a loop to get all tokens. It returns NULL
// when there are no more tokens.
char * strtok(char str[], const char *delims);
// A C/C++ program for splitting a string
// using strtok()
#include
#include
int main()
{
char str[] = "Wiki-techy";
// Returns first token
char *token = strtok(str, "-");
// Keep printing tokens while one of the
// delimiters present in str[].
while (token != NULL)
{
printf("%s\n", token);
token = strtok(NULL, "-");
}
return 0;
}
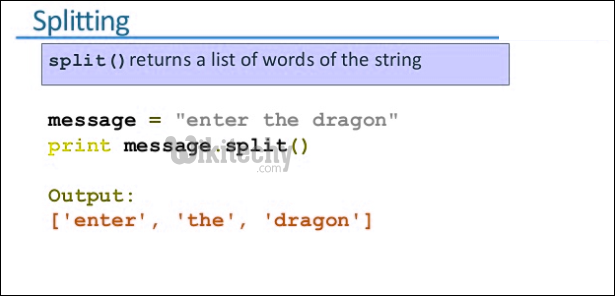
Output:
Wiki
techy
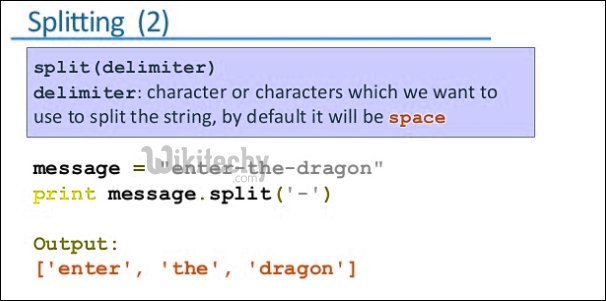
In Java :
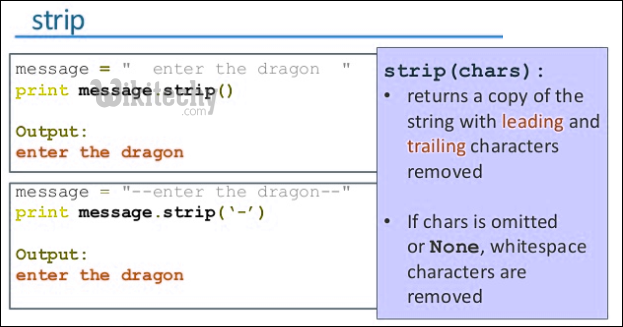
In Java, split() is a method in String class.
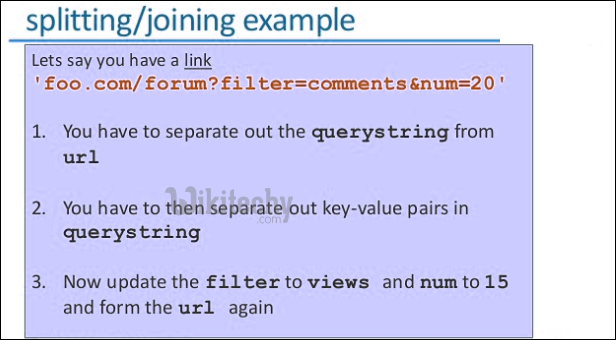
python - Sample - python code :
// expregexp is the delimiting regular expression;
// limit is the number of returned strings
public String[] split(String regexp, int limit);
// We can call split() without limit also
public String[] split(String regexp)
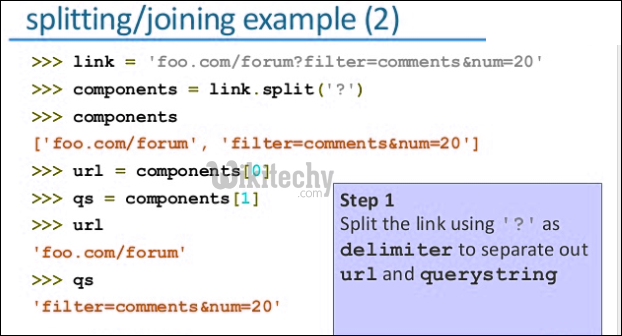
// A Java program for splitting a string
// using split()
import java.io.*;
public class Test
{
public static void main(String args[])
{
String Str = new String("Wikitechy-best-Website");
// Split above string in at-most two strings
for (String val: Str.split("-", 2))
System.out.println(val);
System.out.println("");
// Splits Str into all possible tokens
for (String val: Str.split("-"))
System.out.println(val);
}
}
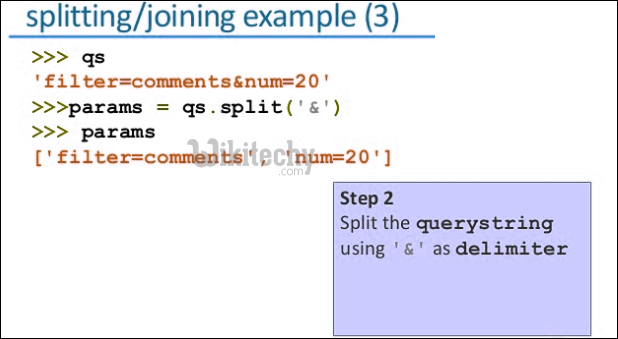
Output:
Wikitechy
best-Website
Wikitechy
best
Website
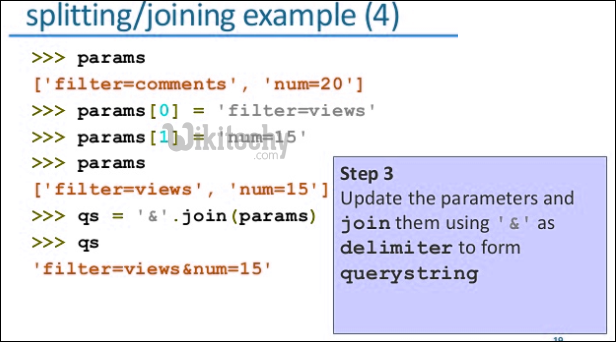
In Python:
// regexp is the delimiting regular expression; // limit is limit the number of splits to be made str.split(regexp = "", limit = string.count(str))
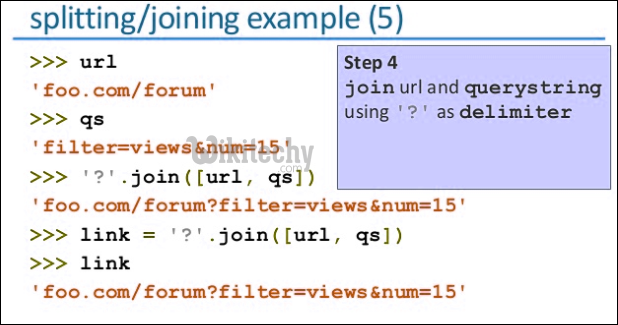
python - Sample - python code :
line = "Wiki1 \nWiki2 \nWiki3";
print line.split()
print line.split(' ', 1)
Output:
['Wiki1', 'Wiki2', 'Wiki3'] ['Wiki1', '\nWiki2 \nWiki3']