python tutorial - Python coding interview questions - learn python - python programming
python interview questions :41
How do you compile Python C/C++ extensions for different OS/versions of Python?
- We use Virtual Machines and a Hudson server.
- We have a Virtual Machine for each architecture we support (generally compiling doesn't stretch the resources allocated to them, so a VM is fine). I guess the configuration of each VM could be managed by something like Puppet or Chef to ensure it is a suitable build environment. You could of course use real machines if you have the hardware, but you'd want to avoid using machines which people are actually using (e.g. developer workstations).
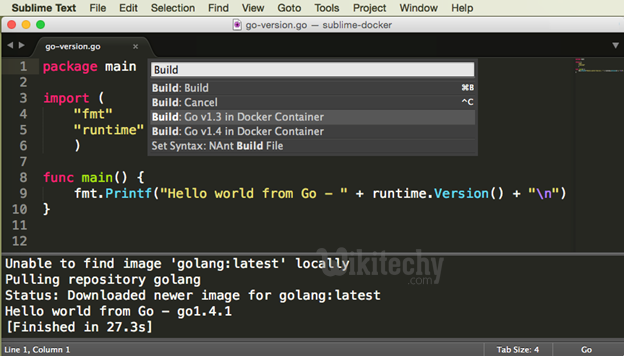
Learn python - python tutorial - python-extensions - python examples - python programs
- We then use a multi-configuration project in Hudson to setup a build matrix. The build matrix allows us to (with a single click) build multiple python versions, on multiple architectures, but in theory you can build every and any combination you can setup in your matrix. Of course you could use Jenkins instead.
python interview questions :42
Why isn't __getattr__ invoked when attr == '__str__'?
- It's because the base class object already implements a default __str__ method, and our __getattr__ function is only called for missing attributes. To fix the example as it is we must use the __getattribute__ method instead, but beware of the dangers.
class GetAttr(object):
def __getattribute__(self, attr):
print('getattr: ' + attr)
if attr == '__str__':
return lambda: '[Getattr str]'
else:
return lambda *args: None
A better and more readable solution would be to simply override the __str__ method explicitly.
class GetAttr(object):
def __getattr__(self, attr):
print('getattr: ' + attr)
return lambda *args: None
def __str__(self):
return '[Getattr str]'
click below button to copy the code. By Python tutorial team
python interview questions :43
Which tool in Python will you use to find bugs if any?
- Pylint and Pychecker. Pylint verifies that a module satisfies all the coding standards or not. Pychecker is a static analysis tool that helps find out bugs in the course code.
python interview questions :44
How are arguments passed in Python- by reference or by value?
- The answer to this question is neither of these because passing semantics in Python are completely different. In all cases, Python passes arguments by value where all values are references to objects.
python interview questions :45
Explain the usage of decorators?
- Decorators in Python are used to modify or inject code in functions or classes. Using decorators, you can wrap a class or function method call so that a piece of code can be executed before or after the execution of the original code.
- Decorators can be used to check for permissions, modify or track the arguments passed to a method, logging the calls to a specific method, etc.
python interview questions :46
How can you check whether a pandas data frame is empty or not?
- The attribute df.empty is used to check whether a data frame is empty or not.
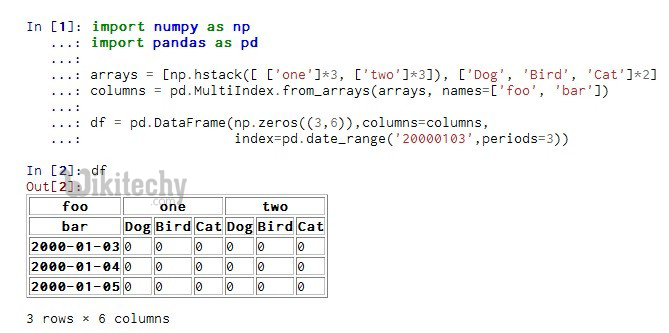
Learn python - python tutorial - data-frame - python examples - python programs
python interview questions :47
What will be the output of the below Python code def multipliers ()?
return [lambda x: i * x for i in range (4)]
print [m (2) for m in multipliers ()]
click below button to copy the code. By Python tutorial team
output:
[6, 6,6,6].
- The reason for this is that because of late binding the value of the variable i is looked up when any of the functions returned by multipliers.
python interview questions :48
What do you mean by list comprehension?
- The process of creating a list while performing some operation on the data so that it can be accessed using an iterator is referred to as List Comprehension.
[ord (j) for j in string.ascii_uppercase]
click below button to copy the code. By Python tutorial team
output
[65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90]
python interview questions :49
What will be the output of the below code word = ‘aeioubcdfg'?
print word [:3] + word [3:]
click below button to copy the code. By Python tutorial team
output:
‘aeioubcdfg'.
- In string slicing when the indices of both the slices collide and a “+” operator is applied on the string it concatenates them.
sss list= [‘a’,’e’,’i’,’o’,’u’]
print list [8:]
click below button to copy the code. By Python tutorial team
output :
empty list [].
- Most of the people might confuse the answer with an index error because the code is attempting to access a member in the list whose index exceeds the total number of members in the list.
- The reason being the code is trying to access the slice of a list at a starting index which is greater than the number of members in the list.
python interview questions :50
What will be the output of the below code:def foo (i= [])?
i.append (1)
return i
>>> foo ()
>>> foo ()
click below button to copy the code. By Python tutorial team
output :
[1]
[1, 1]
- Argument to the function foo is evaluated only once when the function is defined.
- However, since it is a list, on every all the list is modified by appending a 1 to it.